diff --git a/build/build.ps1 b/build/build.ps1
deleted file mode 100644
index 932fbef..0000000
--- a/build/build.ps1
+++ /dev/null
@@ -1,11 +0,0 @@
-param($version, $suffix, $env='release', [switch]$push=$false)
-
-$fullVersion = $version # -join($version, '-', $suffix)
-$outFolder = ".\$fullVersion"
-$packageProj = "..\src\Our.Umbraco.LinkedPages\Our.Umbraco.LinkedPages.csproj"
-
-dotnet pack $packageProj -c $env -o $outFolder /p:ContinuousIntegrationBuild=true,version=$fullVersion
-
-if ($push) {
- .\nuget.exe push "$outFolder\*.nupkg" -ApiKey AzureDevOps -src https://pkgs.dev.azure.com/jumoo/Public/_packaging/nightly/nuget/v3/index.json
-}
\ No newline at end of file
diff --git a/dist/build-package.ps1 b/dist/build-package.ps1
new file mode 100644
index 0000000..0d000ad
--- /dev/null
+++ b/dist/build-package.ps1
@@ -0,0 +1,40 @@
+param(
+ # Version to build
+ [Parameter(Mandatory)]
+ [string]
+ [Alias("v")]
+ $version,
+
+ # Optional suffix for the version
+ [string]
+ $suffix,
+
+ # configuration to build against
+ [string]
+ $config = 'release',
+
+ # push to azure nightly feed ?
+ [switch]
+ $push = $false
+)
+
+$versionString = $version
+if (![string]::IsNullOrWhiteSpace($suffix)) {
+ $versionString = -join($version, '-', $suffix)
+}
+
+$package = "Our.Umbraco.LinkedPages";
+$project = "..\src\$package\$package.csproj"
+$outFolder = ".\$versionString"
+$buildParams = "ContinuousIntegrationBuild=true,version=$versionString"
+
+# pack the thing
+dotnet pack $project -c $config -o $outFolder /p:$buildParams
+
+if ($push) {
+ # push to a nightly .
+ $feedUrl = "https://pkgs.dev.azure.com/jumoo/Public/_packaging/nightly/nuget/v3/index.json";
+ &nuget.exe push "$outfolder\*.nupkg" -ApiKey AzureDevOps -src $feedUrl
+}
+
+XCOPY "$outfolder\*.nupkg" "c:\source\localgit" /y
\ No newline at end of file
diff --git a/src/Our.Umbraco.LinkedPages/App_Plugins/LinkedPages/package.manifest b/src/Our.Umbraco.LinkedPages/App_Plugins/LinkedPages/package.manifest
deleted file mode 100644
index 8fb56de..0000000
--- a/src/Our.Umbraco.LinkedPages/App_Plugins/LinkedPages/package.manifest
+++ /dev/null
@@ -1,9 +0,0 @@
-{
- "javascript": [
- "~/App_Plugins/LinkedPages/linkedPagesDialogController.js",
- "~/App_Plugins/LinkedPages/linkedPagesService.js"
- ],
- "css": [
- "~/App_Plugins/LinkedPages/linkedpages.css"
- ]
-}
\ No newline at end of file
diff --git a/src/Our.Umbraco.LinkedPages/Controllers/LinkedPagesApiController.cs b/src/Our.Umbraco.LinkedPages/Controllers/LinkedPagesApiController.cs
index b0f68f1..81bbbd5 100644
--- a/src/Our.Umbraco.LinkedPages/Controllers/LinkedPagesApiController.cs
+++ b/src/Our.Umbraco.LinkedPages/Controllers/LinkedPagesApiController.cs
@@ -2,7 +2,6 @@
using System.Collections.Generic;
using System.Linq;
-#if NETCOREAPP
using Microsoft.AspNetCore.Mvc;
using Umbraco.Cms.Core;
@@ -11,149 +10,139 @@
using Umbraco.Cms.Core.Services;
using Umbraco.Cms.Web.BackOffice.Controllers;
using Umbraco.Extensions;
-#else
-using System.Web.Http;
-using Umbraco.Core;
-using Umbraco.Core.Models;
-using Umbraco.Core.Models.Entities;
-using Umbraco.Core.Services;
-using Umbraco.Web.Editors;
-#endif
+namespace Our.Umbraco.LinkedPages.Controllers;
-namespace Our.Umbraco.LinkedPages.Controllers
+public class LinkedPagesApiController : UmbracoAuthorizedJsonController
{
- public class LinkedPagesApiController : UmbracoAuthorizedJsonController
+ private readonly IRelationService _relationService;
+ private readonly IEntityService _entityService;
+ private readonly LinkedPagesConfig _config;
+
+ private string defaultRelationType = Constants.Conventions.RelationTypes.RelateDocumentOnCopyAlias;
+ private int relationTypeId = 0;
+ private int[] _ignoredTypeIds;
+
+ public LinkedPagesApiController(
+ IRelationService relationService,
+ IEntityService entityService,
+ LinkedPagesConfig config)
{
- private readonly IRelationService _relationService;
- private readonly IEntityService _entityService;
- private readonly LinkedPagesConfig _config;
-
- private string defaultRelationType = Constants.Conventions.RelationTypes.RelateDocumentOnCopyAlias;
- private int relationTypeId = 0;
- private int[] _ignoredTypeIds;
-
- public LinkedPagesApiController(
- IRelationService relationService,
- IEntityService entityService,
- LinkedPagesConfig config)
- {
- _relationService = relationService;
- _entityService = entityService;
- _config = config;
+ _relationService = relationService;
+ _entityService = entityService;
+ _config = config;
- _ignoredTypeIds = GetIgnoredTypeIds();
- }
+ _ignoredTypeIds = GetIgnoredTypeIds();
+ }
- private int[] GetIgnoredTypeIds()
- {
- var ignore = _config.ignoredTypes.ToDelimitedList();
- var types = _relationService.GetAllRelationTypes();
+ private int[] GetIgnoredTypeIds()
+ {
+ var ignore = _config.ignoredTypes.ToDelimitedList();
+ var types = _relationService.GetAllRelationTypes();
- return types.Where(x => ignore.InvariantContains(x.Alias))
- .Select(x => x.Id)
- .ToArray();
- }
+ return types.Where(x => ignore.InvariantContains(x.Alias))
+ .Select(x => x.Id)
+ .ToArray();
+ }
- ///
- /// API endpoint - used for discovery in ServerVariablesParser.
- ///
- [HttpGet]
- public bool GetApi() => true;
+ ///
+ /// API endpoint - used for discovery in ServerVariablesParser.
+ ///
+ [HttpGet]
+ public bool GetApi() => true;
- [HttpGet]
- public IEnumerable GetChildLinks(int id)
- {
- var relations = _relationService.GetByParentId(id);
- if (!relations.Any())
- return Enumerable.Empty();
+ [HttpGet]
+ public IEnumerable GetChildLinks(int id)
+ {
+ var relations = _relationService.GetByParentId(id);
+ if (!relations.Any())
+ return Enumerable.Empty();
- return GetRelations(relations, true);
- }
+ return GetRelations(relations, true);
+ }
- [HttpGet]
- public IEnumerable GetParentLinks(int id)
- {
- var relations = _relationService.GetByChildId(id);
- if (!relations.Any())
- return Enumerable.Empty();
+ [HttpGet]
+ public IEnumerable GetParentLinks(int id)
+ {
+ var relations = _relationService.GetByChildId(id);
+ if (!relations.Any())
+ return Enumerable.Empty();
- return GetRelations(relations, false);
- }
+ return GetRelations(relations, false);
+ }
- [HttpPost]
- public IEnumerable CreateLink(int parent, int child)
- {
- var parentNode = _entityService.Get(parent);
- var childNode = _entityService.Get(child);
+ [HttpPost]
+ public IEnumerable CreateLink(int parent, int child)
+ {
+ var parentNode = _entityService.Get(parent);
+ var childNode = _entityService.Get(child);
- if (parentNode == null || childNode == null)
- throw new KeyNotFoundException();
+ if (parentNode == null || childNode == null)
+ throw new KeyNotFoundException();
- var relationType = _relationService.GetRelationTypeByAlias(defaultRelationType);
- if (relationType == null)
- throw new ApplicationException($"Cannot create relation of type {defaultRelationType}");
+ var relationType = _relationService.GetRelationTypeByAlias(defaultRelationType);
+ if (relationType == null)
+ throw new ApplicationException($"Cannot create relation of type {defaultRelationType}");
- var relation = new Relation(parent, child, relationType);
- _relationService.Save(relation);
+ var relation = new Relation(parent, child, relationType);
+ _relationService.Save(relation);
- return GetChildLinks(parent);
- }
+ return GetChildLinks(parent);
+ }
- [HttpDelete]
- public IEnumerable RemoveLink(int id, int currentPage)
- {
- var relation = _relationService.GetById(id);
- if (relation == null)
- throw new ArgumentOutOfRangeException($"Cannot find relation with id {id}");
+ [HttpDelete]
+ public IEnumerable RemoveLink(int id, int currentPage)
+ {
+ var relation = _relationService.GetById(id);
+ if (relation == null)
+ throw new ArgumentOutOfRangeException($"Cannot find relation with id {id}");
- _relationService.Delete(relation);
+ _relationService.Delete(relation);
- return GetChildLinks(currentPage);
- }
+ return GetChildLinks(currentPage);
+ }
- private IEnumerable GetRelations(IEnumerable relations, bool linkChild)
+ private IEnumerable GetRelations(IEnumerable relations, bool linkChild)
+ {
+ foreach (var relation in relations.Where(x => !_ignoredTypeIds.Contains(x.RelationTypeId)))
{
- foreach(var relation in relations.Where(x => !_ignoredTypeIds.Contains(x.RelationTypeId)))
+ if (relationTypeId == 0 || relation.RelationType.Id == this.relationTypeId)
{
- if (relationTypeId == 0 || relation.RelationType.Id == this.relationTypeId)
+ var nodeId = linkChild ? relation.ChildId : relation.ParentId;
+ var node = _entityService.Get(nodeId);
+ if (node == null) continue;
+
+ yield return new LinkedPageInfo
{
- var nodeId = linkChild ? relation.ChildId : relation.ParentId;
- var node = _entityService.Get(nodeId);
- if (node == null) continue;
-
- yield return new LinkedPageInfo
- {
- RelationId = relation.Id,
- PageId = nodeId,
- Name = node.Name,
- Path = GetContentPath(node),
- RelationType = relation.RelationType.Alias,
- RelationTypeId = relation.RelationTypeId
- };
- }
+ RelationId = relation.Id,
+ PageId = nodeId,
+ Name = node.Name,
+ Path = GetContentPath(node),
+ RelationType = relation.RelationType.Alias,
+ RelationTypeId = relation.RelationTypeId
+ };
}
}
+ }
- private string GetContentPath(IEntitySlim node)
- {
- if (node == null) return string.Empty;
+ private string GetContentPath(IEntitySlim node)
+ {
+ if (node == null) return string.Empty;
- var path = string.Empty;
- if (node.ParentId > -1)
- {
- var parent = _entityService.GetParent(node.Id);
- if (parent != null)
- path += GetContentPath(parent);
- }
+ var path = string.Empty;
+ if (node.ParentId > -1)
+ {
+ var parent = _entityService.GetParent(node.Id);
+ if (parent != null)
+ path += GetContentPath(parent);
+ }
- if (!string.IsNullOrWhiteSpace(path))
- return path + " > " + node.Name;
+ if (!string.IsNullOrWhiteSpace(path))
+ return path + " > " + node.Name;
- return node.Name;
- }
+ return node.Name;
}
}
diff --git a/src/Our.Umbraco.LinkedPages/LinikedAction.cs b/src/Our.Umbraco.LinkedPages/LinikedAction.cs
index 05a785b..0d066b4 100644
--- a/src/Our.Umbraco.LinkedPages/LinikedAction.cs
+++ b/src/Our.Umbraco.LinkedPages/LinikedAction.cs
@@ -1,30 +1,18 @@
-using System;
-using System.Collections.Generic;
-using System.Linq;
-using System.Text;
-using System.Threading.Tasks;
+using Umbraco.Cms.Core.Actions;
+namespace Our.Umbraco.LinkedPages;
-#if NETCOREAPP
-using Umbraco.Cms.Core.Actions;
-#else
-using Umbraco.Web.Actions;
-#endif
-
-namespace Our.Umbraco.LinkedPages
+public class LinikedAction : IAction
{
- public class LinikedAction : IAction
- {
- public char Letter => LinkedPages.ActionLetter[0];
+ public char Letter => LinkedPages.ActionLetter[0];
- public bool ShowInNotifier => true;
+ public bool ShowInNotifier => true;
- public bool CanBePermissionAssigned => true;
+ public bool CanBePermissionAssigned => true;
- public string Icon => "link";
+ public string Icon => "link";
- public string Alias => LinkedPages.Alias;
+ public string Alias => LinkedPages.Alias;
- public string Category => LinkedPages.Category;
- }
+ public string Category => LinkedPages.Category;
}
diff --git a/src/Our.Umbraco.LinkedPages/LinkPagedNotificationHandler.cs b/src/Our.Umbraco.LinkedPages/LinkPagedNotificationHandler.cs
index ecae57b..9530d6f 100644
--- a/src/Our.Umbraco.LinkedPages/LinkPagedNotificationHandler.cs
+++ b/src/Our.Umbraco.LinkedPages/LinkPagedNotificationHandler.cs
@@ -1,10 +1,4 @@
-//
-// Notification handlers - NETCORE only - handle server variables and the menu
-//
-//
-#if NETCOREAPP
-
-using System;
+using System;
using System.Collections.Generic;
using System.Linq;
@@ -20,71 +14,68 @@
using Umbraco.Cms.Core.Services;
using Umbraco.Extensions;
-namespace Our.Umbraco.LinkedPages
+namespace Our.Umbraco.LinkedPages;
+
+public class LinkPagedNotificationHandler :
+ INotificationHandler,
+ INotificationHandler
{
- public class LinkPagedNotificationHandler :
- INotificationHandler,
- INotificationHandler
+ private readonly LinkedPagesConfig _config;
+ private readonly LinkGenerator _linkGenerator;
+ private readonly IEntityService _entityService;
+ private readonly IUserService _userService;
+ private readonly IBackOfficeSecurityAccessor _backOfficeSecurityAccessor;
+
+ public LinkPagedNotificationHandler(
+ LinkedPagesConfig config,
+ LinkGenerator linkGenerator,
+ IEntityService entityService,
+ IUserService userService,
+ IBackOfficeSecurityAccessor backOfficeSercurityAccessor)
{
- private readonly LinkedPagesConfig _config;
- private readonly LinkGenerator _linkGenerator;
- private readonly IEntityService _entityService;
- private readonly IUserService _userService;
- private readonly IBackOfficeSecurityAccessor _backOfficeSecurityAccessor;
+ _config = config;
+ _linkGenerator = linkGenerator;
+ _entityService = entityService;
+ _userService = userService;
+ _backOfficeSecurityAccessor = backOfficeSercurityAccessor;
+ }
- public LinkPagedNotificationHandler(
- LinkedPagesConfig config,
- LinkGenerator linkGenerator,
- IEntityService entityService,
- IUserService userService,
- IBackOfficeSecurityAccessor backOfficeSercurityAccessor)
+ public void Handle(ServerVariablesParsingNotification notification)
+ {
+ notification.ServerVariables.Add(LinkedPages.Variables.Name, new Dictionary
{
- _config = config;
- _linkGenerator = linkGenerator;
- _entityService = entityService;
- _userService = userService;
- _backOfficeSecurityAccessor = backOfficeSercurityAccessor;
- }
+ { LinkedPages.Variables.ApiRoute, _linkGenerator.GetUmbracoApiServiceBaseUrl(c => c.GetApi()) },
+ { LinkedPages.Variables.ShowRelationType, _config.ShowType },
+ { LinkedPages.Variables.RelationTypeAlias, _config.RelationType },
+ { LinkedPages.Variables.IgnoredTypes, _config.ignoredTypes }
+ });
+ }
+
+ public void Handle(MenuRenderingNotification notification)
+ {
+ if (notification.TreeAlias != Constants.Trees.Content) return;
+
+ var currentUser = _backOfficeSecurityAccessor.BackOfficeSecurity.CurrentUser;
+ var showMenu = currentUser.Groups.Any(x => x.Alias.InvariantContains(Constants.Security.AdminGroupAlias));
- public void Handle(ServerVariablesParsingNotification notification)
+ if (!showMenu && int.TryParse(notification.NodeId, out int nodeId))
{
- notification.ServerVariables.Add(LinkedPages.Variables.Name, new Dictionary
- {
- { LinkedPages.Variables.ApiRoute, _linkGenerator.GetUmbracoApiServiceBaseUrl(c => c.GetApi()) },
- { LinkedPages.Variables.ShowRelationType, _config.ShowType },
- { LinkedPages.Variables.RelationTypeAlias, _config.RelationType },
- { LinkedPages.Variables.IgnoredTypes, _config.ignoredTypes }
- });
+ var permissions = _userService.GetPermissions(currentUser, nodeId);
+ showMenu = permissions.Any(x => x.AssignedPermissions.Contains(LinkedPages.ActionLetter));
}
- public void Handle(MenuRenderingNotification notification)
+ if (showMenu)
{
- if (notification.TreeAlias != Constants.Trees.Content) return;
-
- var currentUser = _backOfficeSecurityAccessor.BackOfficeSecurity.CurrentUser;
- var showMenu = currentUser.Groups.Any(x => x.Alias.InvariantContains(Constants.Security.AdminGroupAlias));
- if (!showMenu && int.TryParse(notification.NodeId, out int nodeId))
+ var item = new MenuItem("linkedPages", "Linked Page")
{
- var permissions = _userService.GetPermissions(currentUser, nodeId);
- showMenu = permissions.Any(x => x.AssignedPermissions.Contains(LinkedPages.ActionLetter));
- }
+ Icon = "link",
+ SeparatorBefore = true
+ };
- if (showMenu)
- {
-
- var item = new MenuItem("linkedPages", "Linked Page")
- {
- Icon = "link",
- SeparatorBefore = true
- };
+ item.AdditionalData.Add("actionView", LinkedPages.ActionView);
- item.AdditionalData.Add("actionView", LinkedPages.ActionView);
-
- notification.Menu.Items.Insert(notification.Menu.Items.Count - 1, item);
- }
+ notification.Menu.Items.Insert(notification.Menu.Items.Count - 1, item);
}
}
-}
-
-#endif
\ No newline at end of file
+}
\ No newline at end of file
diff --git a/src/Our.Umbraco.LinkedPages/LinkedPages.cs b/src/Our.Umbraco.LinkedPages/LinkedPages.cs
index b68e5ce..d066664 100644
--- a/src/Our.Umbraco.LinkedPages/LinkedPages.cs
+++ b/src/Our.Umbraco.LinkedPages/LinkedPages.cs
@@ -1,29 +1,22 @@
-using System;
-using System.Collections.Generic;
-using System.Linq;
-using System.Text;
-using System.Threading.Tasks;
+namespace Our.Umbraco.LinkedPages;
-namespace Our.Umbraco.LinkedPages
+public class LinkedPages
{
- public class LinkedPages
- {
- public const string Alias = "linkPages";
- public const string Category = "structure";
+ public const string Alias = "linkPages";
+ public const string Category = "structure";
- public const string ProductName = "Our.Umbraco.LinkedPages";
- public const string ActionLetter = "l";
+ public const string ProductName = "Our.Umbraco.LinkedPages";
+ public const string ActionLetter = "l";
- public const string ActionView = "/App_Plugins/LinkedPages/linkedDialog.html";
+ public const string ActionView = "/App_Plugins/LinkedPages/linkedDialog.html";
- public static class Variables
- {
- public const string Name = "LinkedPages";
+ public static class Variables
+ {
+ public const string Name = "LinkedPages";
- public const string ApiRoute = "LinkedPageApi";
- public const string ShowRelationType = "showRelationType";
- public const string RelationTypeAlias = "relationTypeAlias";
- public const string IgnoredTypes = "ignoredTypes";
- }
+ public const string ApiRoute = "LinkedPageApi";
+ public const string ShowRelationType = "showRelationType";
+ public const string RelationTypeAlias = "relationTypeAlias";
+ public const string IgnoredTypes = "ignoredTypes";
}
}
diff --git a/src/Our.Umbraco.LinkedPages/LinkedPagesBoot.cs b/src/Our.Umbraco.LinkedPages/LinkedPagesBoot.cs
index 45bc9af..3847eb1 100644
--- a/src/Our.Umbraco.LinkedPages/LinkedPagesBoot.cs
+++ b/src/Our.Umbraco.LinkedPages/LinkedPagesBoot.cs
@@ -1,36 +1,59 @@
-#if NETCOREAPP
+using System.Collections.Generic;
+using System.Linq;
+
using Microsoft.Extensions.DependencyInjection;
using Umbraco.Cms.Core.Composing;
using Umbraco.Cms.Core.DependencyInjection;
+using Umbraco.Cms.Core.Manifest;
using Umbraco.Cms.Core.Notifications;
-using Umbraco.Extensions;
-#else
-using Umbraco.Core;
-using Umbraco.Core.Composing;
-#endif
-namespace Our.Umbraco.LinkedPages
+namespace Our.Umbraco.LinkedPages;
+
+public class LinkedPagesBoot : IComposer
{
- public class LinkedPagesBoot : IComposer
+ public void Compose(IUmbracoBuilder builder)
{
-#if NETCOREAPP
- public void Compose(IUmbracoBuilder builder)
- {
- builder.Services.AddSingleton();
+ builder.AddLinkedPages();
+ }
+}
+
+public static class LinkedPagesBuilderExtensions
+{
+ public static IUmbracoBuilder AddLinkedPages(this IUmbracoBuilder builder)
+ {
+ if (builder.Services.Any(x => x.ServiceType == typeof(LinkedPagesConfig)))
+ return builder;
- builder.AddNotificationHandler();
- builder.AddNotificationHandler();
+ builder.Services.AddSingleton();
+ builder.AddNotificationHandler();
+ builder.AddNotificationHandler();
- }
-#else
- public void Compose(Composition composition)
- {
- composition.RegisterUnique();
+ if (!builder.ManifestFilters().Has())
+ builder.ManifestFilters().Append();
- composition.Components().Append();
- }
-#endif
+ return builder;
}
+}
+internal class LinkedPagesManifestFilter : IManifestFilter
+{
+ public void Filter(List manifests)
+ {
+ manifests.Add(new PackageManifest
+ {
+ PackageName = LinkedPages.ProductName,
+ Version = typeof(LinkedPages).Assembly.GetName().Version.ToString(3),
+ AllowPackageTelemetry = true,
+ Scripts = new[]
+ {
+ "/App_Plugins/LinkedPages/linkedPagesDialogController.js",
+ "/App_Plugins/LinkedPages/linkedPagesService.js"
+ },
+ Stylesheets = new[]
+ {
+ "/App_Plugins/LinkedPages/linkedpages.css"
+ }
+ });
+ }
}
diff --git a/src/Our.Umbraco.LinkedPages/LinkedPagesComponent.cs b/src/Our.Umbraco.LinkedPages/LinkedPagesComponent.cs
deleted file mode 100644
index 63d2c97..0000000
--- a/src/Our.Umbraco.LinkedPages/LinkedPagesComponent.cs
+++ /dev/null
@@ -1,91 +0,0 @@
-//
-// Component is only used in .netframework app to hook into menu and server variable parser.
-//
-#if NETFRAMEWORK
-
-using Our.Umbraco.LinkedPages.Controllers;
-using System.Collections.Generic;
-using System.Configuration;
-using System.Web.Routing;
-using System.Web;
-using System;
-
-using Umbraco.Core.Composing;
-using Umbraco.Web.JavaScript;
-using Umbraco.Web.Trees;
-using Umbraco.Core;
-using System.Web.Mvc;
-using Umbraco.Web;
-using System.Linq;
-using Umbraco.Web.Models.Trees;
-
-namespace Our.Umbraco.LinkedPages
-{
- public class LinkedPagesComponent : IComponent
- {
- private readonly LinkedPagesConfig _config;
-
- public LinkedPagesComponent(LinkedPagesConfig config)
- {
- _config = config;
- }
-
- public void Initialize()
- {
- ContentTreeController.MenuRendering += ContentTreeController_MenuRendering;
- ServerVariablesParser.Parsing += ServerVariablesParser_Parsing;
- }
-
- private void ServerVariablesParser_Parsing(object sender, System.Collections.Generic.Dictionary e)
- {
- if (HttpContext.Current == null)
- throw new InvalidOperationException("This method requires an HttpContext");
-
-
- var urlHelper = new UrlHelper(new RequestContext(
- new HttpContextWrapper(HttpContext.Current), new RouteData()));
-
- e.Add(LinkedPages.Variables.Name, new Dictionary
- {
- { LinkedPages.Variables.ApiRoute, urlHelper.GetUmbracoApiServiceBaseUrl( c => c.GetApi()) },
- { LinkedPages.Variables.ShowRelationType, _config.ShowType },
- { LinkedPages.Variables.RelationTypeAlias, _config.RelationType },
- { LinkedPages.Variables.IgnoredTypes, _config.ignoredTypes }
- });
- }
-
- private void ContentTreeController_MenuRendering(TreeControllerBase sender, MenuRenderingEventArgs e)
- {
- // only the content tree and not the root.
- if (!sender.TreeAlias.InvariantEquals("content") || e.NodeId == "-1") return;
-
- bool showMenu = sender.Security.CurrentUser.Groups.Any(x => x.Alias.InvariantContains(Constants.Security.AdminGroupAlias));
- if (!showMenu && int.TryParse(e.NodeId, out int nodeId))
- {
- var permissions = sender.Services.UserService
- .GetPermissions(sender.Security.CurrentUser, nodeId);
-
- showMenu = permissions.Any(x => x.AssignedPermissions.Contains(LinkedPages.ActionLetter));
- }
-
- if (showMenu)
- {
- var linkedPagedItem = new MenuItem("linkedPages", "Linked Pages")
- {
- Icon = "link",
- SeparatorBefore = true
- };
-
- linkedPagedItem.AdditionalData.Add("actionView", UriUtility.ToAbsolute(LinkedPages.ActionView));
-
- e.Menu.Items.Insert(e.Menu.Items.Count - 1, linkedPagedItem);
- }
- }
-
- public void Terminate()
- {
- }
- }
-
-}
-#endif
\ No newline at end of file
diff --git a/src/Our.Umbraco.LinkedPages/LinkedPagesConfig.cs b/src/Our.Umbraco.LinkedPages/LinkedPagesConfig.cs
index 537c1d4..5ccea0b 100644
--- a/src/Our.Umbraco.LinkedPages/LinkedPagesConfig.cs
+++ b/src/Our.Umbraco.LinkedPages/LinkedPagesConfig.cs
@@ -1,46 +1,34 @@
-#if NETCOREAPP
-using Microsoft.Extensions.Configuration;
+using Microsoft.Extensions.Configuration;
+
using Umbraco.Extensions;
-#else
-using System.Configuration;
-using Umbraco.Core;
-#endif
+namespace Our.Umbraco.LinkedPages;
-namespace Our.Umbraco.LinkedPages
+public class LinkedPagesConfig
{
- public class LinkedPagesConfig
- {
-#if NETCOREAPP
- private readonly IConfiguration _config;
+ private readonly IConfiguration _config;
- public LinkedPagesConfig(IConfiguration configuration)
- {
- _config = configuration;
- }
-#endif
+ public LinkedPagesConfig(IConfiguration configuration)
+ {
+ _config = configuration;
+ }
- public string RelationType => GetConfigValue("LinkedPages:RelationType", string.Empty);
- public bool ShowType => GetConfigValue("LinkedPages:ShowType", true);
+ public string RelationType => GetConfigValue("LinkedPages:RelationType", string.Empty);
+ public bool ShowType => GetConfigValue("LinkedPages:ShowType", true);
- public string ignoredTypes => GetConfigValue("LinkedPages:Ignore", "umbMedia,umbDocument");
+ public string ignoredTypes => GetConfigValue("LinkedPages:Ignore", "umbMedia,umbDocument");
- private TResult GetConfigValue(string path, TResult defaultValue)
+ private TResult GetConfigValue(string path, TResult defaultValue)
+ {
+ var value = _config[path];
+ if (value != null)
{
-#if NETCOREAPP
- var value = _config[path];
-#else
- var value = ConfigurationManager.AppSettings[path.Replace(":", ".")];
-#endif
- if (value != null)
- {
- var attempt = value.TryConvertTo();
- if (attempt) return attempt.Result;
- }
-
- return defaultValue;
-
+ var attempt = value.TryConvertTo();
+ if (attempt) return attempt.Result;
}
+
+ return defaultValue;
+
}
}
diff --git a/src/Our.Umbraco.LinkedPages/Models/LinkedPageInfo.cs b/src/Our.Umbraco.LinkedPages/Models/LinkedPageInfo.cs
index 0da5a37..ee0c93a 100644
--- a/src/Our.Umbraco.LinkedPages/Models/LinkedPageInfo.cs
+++ b/src/Our.Umbraco.LinkedPages/Models/LinkedPageInfo.cs
@@ -1,17 +1,16 @@
-using Newtonsoft.Json.Serialization;
using Newtonsoft.Json;
+using Newtonsoft.Json.Serialization;
+
+namespace Our.Umbraco.LinkedPages;
-namespace Our.Umbraco.LinkedPages
+[JsonObject(NamingStrategyType = typeof(CamelCaseNamingStrategy))]
+public class LinkedPageInfo
{
- [JsonObject(NamingStrategyType = typeof(CamelCaseNamingStrategy))]
- public class LinkedPageInfo
- {
- public int RelationId { get; set; }
- public int PageId { get; set; }
- public string Name { get; set; }
- public string Path { get; set; }
- public int RelationTypeId { get; set; }
- public string RelationType { get; set; }
- }
+ public int RelationId { get; set; }
+ public int PageId { get; set; }
+ public string Name { get; set; }
+ public string Path { get; set; }
+ public int RelationTypeId { get; set; }
+ public string RelationType { get; set; }
}
diff --git a/src/Our.Umbraco.LinkedPages/Our.Umbraco.LinkedPages.csproj b/src/Our.Umbraco.LinkedPages/Our.Umbraco.LinkedPages.csproj
index d4fc52a..51a4420 100644
--- a/src/Our.Umbraco.LinkedPages/Our.Umbraco.LinkedPages.csproj
+++ b/src/Our.Umbraco.LinkedPages/Our.Umbraco.LinkedPages.csproj
@@ -1,7 +1,7 @@
-
+
- net60;net472
+ net6.0
content
Our.Umbraco.LinkedPages
@@ -10,34 +10,29 @@
View and Manage relations between pages in Umbraco v8 & v9
umbraco umbraco-marketplace
+ https://github.com/KevinJump/Our.Umbraco.LinkedPages
+ https://github.com/KevinJump/Our.Umbraco.LinkedPages
+
+ App_Plugins
README.md
+ logo.png
-
-
-
-
-
+
+
-
-
-
+
+
+
-
-
- true
- Always
-
-
True
buildTransitive
-
diff --git a/src/Our.Umbraco.LinkedPages/build/Our.Umbraco.LinkedPages.targets b/src/Our.Umbraco.LinkedPages/build/Our.Umbraco.LinkedPages.targets
index e45a005..4b3cebb 100644
--- a/src/Our.Umbraco.LinkedPages/build/Our.Umbraco.LinkedPages.targets
+++ b/src/Our.Umbraco.LinkedPages/build/Our.Umbraco.LinkedPages.targets
@@ -1,21 +1,6 @@
-
- $(MSBuildThisFileDirectory)..\content\App_Plugins\LinkedPages\**\*.*
-
-
-
-
-
-
-
-
-
-
-
+
diff --git a/src/Our.Umbraco.LinkedPages/docs/README.md b/src/Our.Umbraco.LinkedPages/docs/README.md
index 49d19bc..7fc0694 100644
--- a/src/Our.Umbraco.LinkedPages/docs/README.md
+++ b/src/Our.Umbraco.LinkedPages/docs/README.md
@@ -8,22 +8,9 @@ From here you can quickly and easily see the related items for any page.
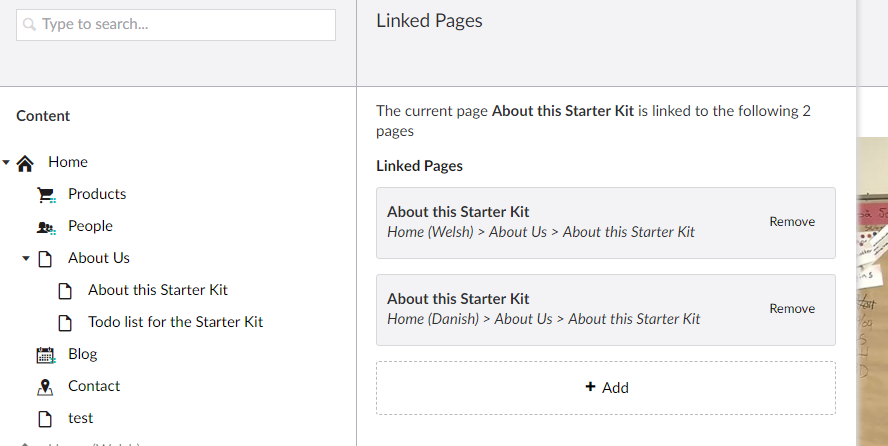
-
# Config
you can control what relationships are displayed in the linked pages dialog via settings in web.config / app settings
-
-
-### Web.Config
-
-```
-
- ...
-
-
-
-
-```
### appsettings.json
diff --git a/src/Our.Umbraco.LinkedPages/logo.png b/src/Our.Umbraco.LinkedPages/logo.png
new file mode 100644
index 0000000..569b0f5
Binary files /dev/null and b/src/Our.Umbraco.LinkedPages/logo.png differ
diff --git a/src/Our.Umbraco.LinkedPages/App_Plugins/LinkedPages/lang/en-US.xml b/src/Our.Umbraco.LinkedPages/wwwroot/LinkedPages/lang/en-US.xml
similarity index 100%
rename from src/Our.Umbraco.LinkedPages/App_Plugins/LinkedPages/lang/en-US.xml
rename to src/Our.Umbraco.LinkedPages/wwwroot/LinkedPages/lang/en-US.xml
diff --git a/src/Our.Umbraco.LinkedPages/App_Plugins/LinkedPages/linkedDialog.html b/src/Our.Umbraco.LinkedPages/wwwroot/LinkedPages/linkedDialog.html
similarity index 100%
rename from src/Our.Umbraco.LinkedPages/App_Plugins/LinkedPages/linkedDialog.html
rename to src/Our.Umbraco.LinkedPages/wwwroot/LinkedPages/linkedDialog.html
diff --git a/src/Our.Umbraco.LinkedPages/App_Plugins/LinkedPages/linkedPagesDialogController.js b/src/Our.Umbraco.LinkedPages/wwwroot/LinkedPages/linkedPagesDialogController.js
similarity index 100%
rename from src/Our.Umbraco.LinkedPages/App_Plugins/LinkedPages/linkedPagesDialogController.js
rename to src/Our.Umbraco.LinkedPages/wwwroot/LinkedPages/linkedPagesDialogController.js
diff --git a/src/Our.Umbraco.LinkedPages/App_Plugins/LinkedPages/linkedPagesService.js b/src/Our.Umbraco.LinkedPages/wwwroot/LinkedPages/linkedPagesService.js
similarity index 100%
rename from src/Our.Umbraco.LinkedPages/App_Plugins/LinkedPages/linkedPagesService.js
rename to src/Our.Umbraco.LinkedPages/wwwroot/LinkedPages/linkedPagesService.js
diff --git a/src/Our.Umbraco.LinkedPages/App_Plugins/LinkedPages/linkedpages.css b/src/Our.Umbraco.LinkedPages/wwwroot/LinkedPages/linkedpages.css
similarity index 100%
rename from src/Our.Umbraco.LinkedPages/App_Plugins/LinkedPages/linkedpages.css
rename to src/Our.Umbraco.LinkedPages/wwwroot/LinkedPages/linkedpages.css
diff --git a/umbraco-marketplace.json b/umbraco-marketplace.json
new file mode 100644
index 0000000..67d84f1
--- /dev/null
+++ b/umbraco-marketplace.json
@@ -0,0 +1,32 @@
+{
+ "$schema": "https://marketplace.umbraco.com/umbraco-marketplace-schema.json",
+ "AuthorDetails": {
+ "Name": "Jumoo",
+ "Description": "Jumoo are a software development company based in the UK",
+ "Url": "https://jumoo.co.uk",
+ "ImageUrl": "https://jumoo.co.uk/img/ju_square.jpg"
+ },
+ "Category": "Editor Tools",
+ "PackageType": "Package",
+ "LicenseTypes": ["Free"],
+ "Tags": [ "translation", "relations" ],
+ "PackagesByAuthor": [
+ "uSync",
+ "uSync.Complete",
+ "Jumoo.TranslationManger",
+ "Our.Umbraco.BackOfficeThemes",
+ "Our.Umbraco.LinkedPages",
+ "Our.Umbraco.MaintenanceMode"
+ ],
+ "RelatedPackages": [
+ {
+ "PackageId": "Jumoo.TranslationManager",
+ "Description": "Translation tools for Umbraco"
+ }
+ ],
+
+ "Title": "Linked Pages",
+ "Description": "Quickly manage relationship links between pages within Umbraco",
+ "DiscussionForumUrl": "https://our.umbraco.com/packages/backoffice-extensions/linked-pages/linked-pages-feedback/",
+ "IssueTrackerUrl": "https://github.com/KevinJump/Our.Umbraco.LinkedPages/issues"
+}
\ No newline at end of file