diff --git a/README.md b/README.md
index 368dc9a9..7c1278fe 100644
--- a/README.md
+++ b/README.md
@@ -1,13 +1,15 @@
  
+[
](https://apt.izzysoft.de/fdroid/index/apk/com.nextcloud_cookbook_flutter)
# Nextcloud Cookbook Mobile Client written in Flutter
-This project aims to provide a mobile client for both Android and IOs for the nextcloud plugin cookbook (https://github.com/nextcloud/cookbook)
+This project aims to provide a mobile client for both Android and IOs for the nextcloud app cookbook (https://github.com/nextcloud/cookbook)
+
+It works best with an Nextcloud installation >= 17
## Screenshots
-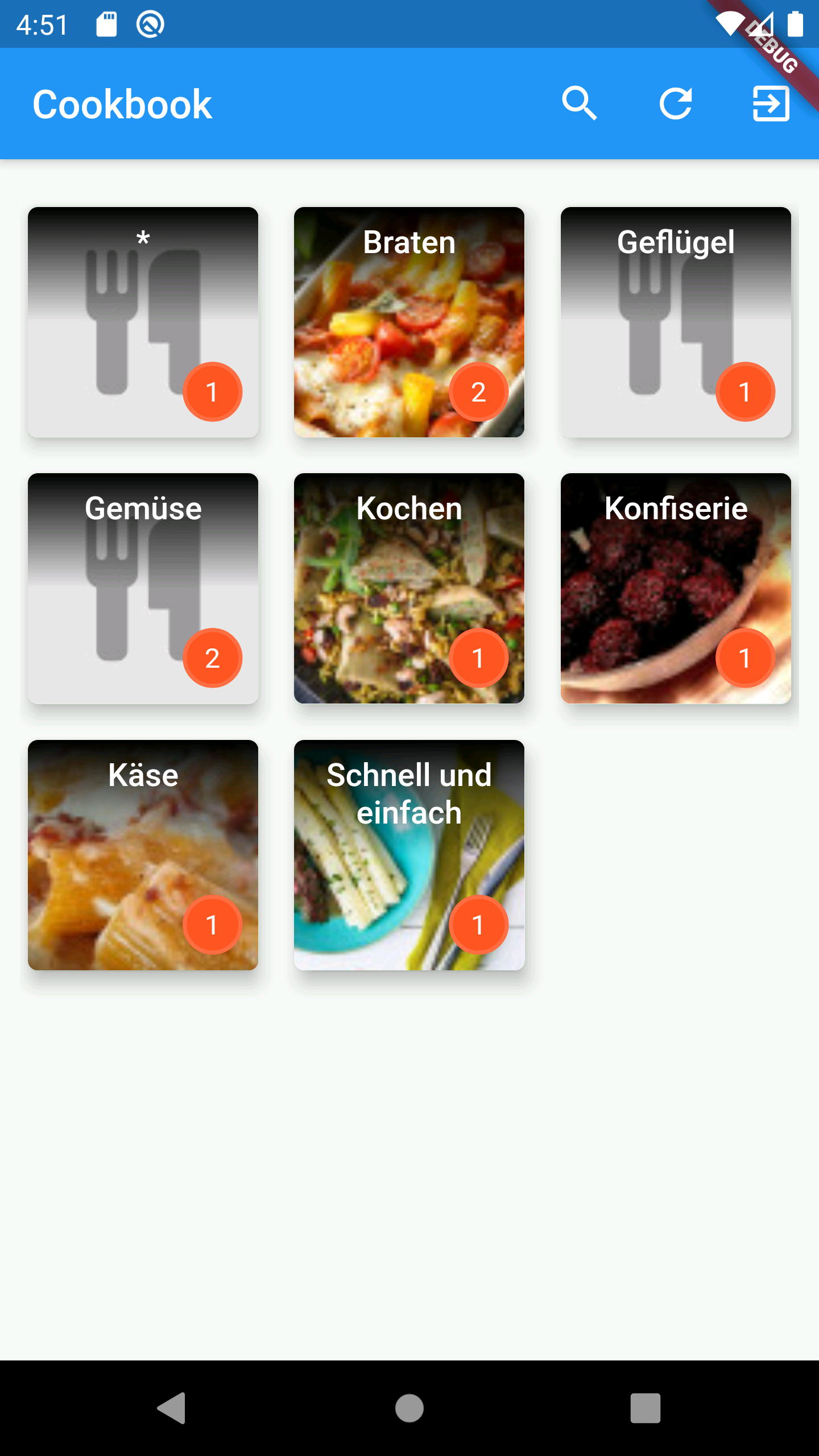
-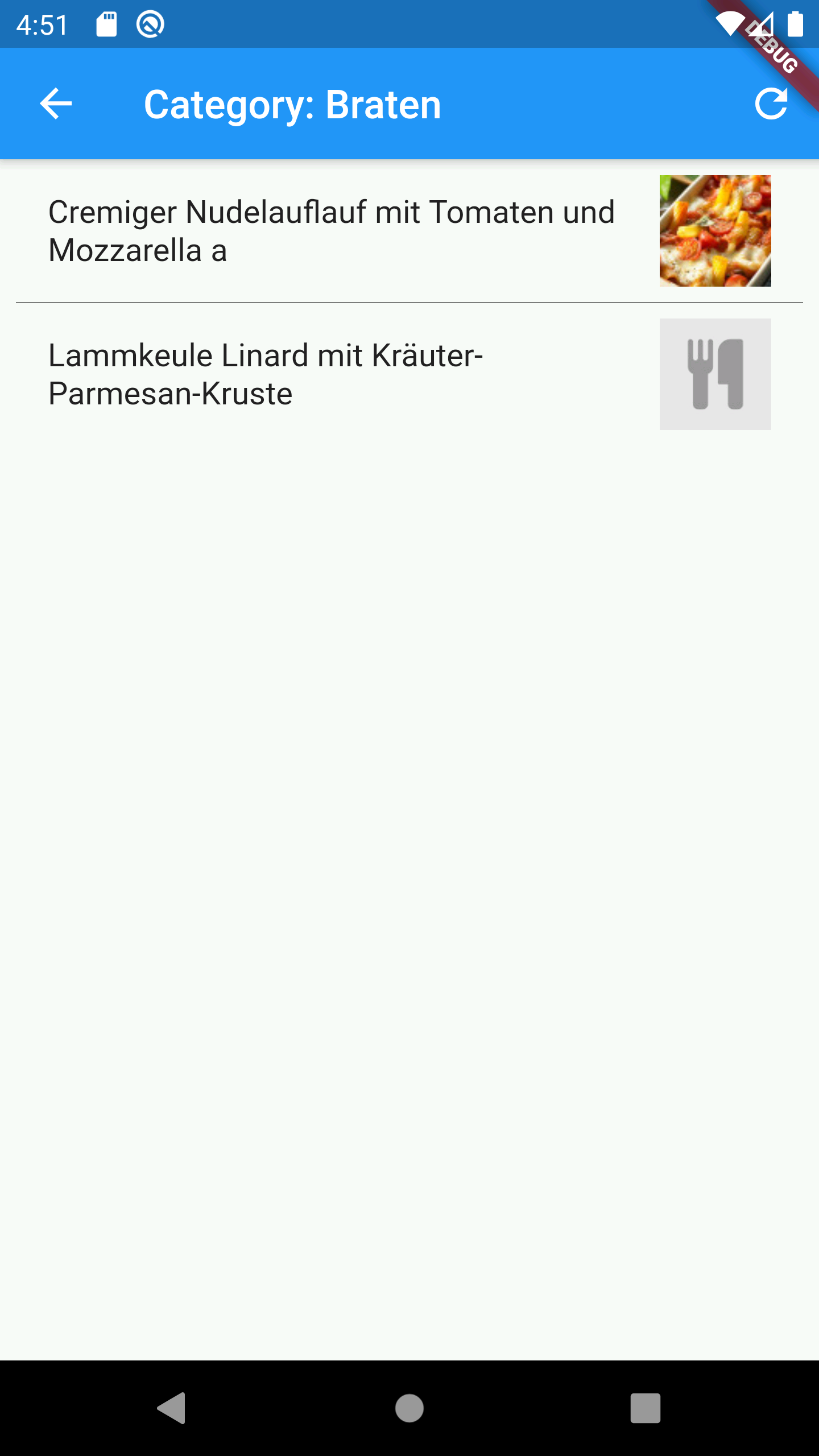
-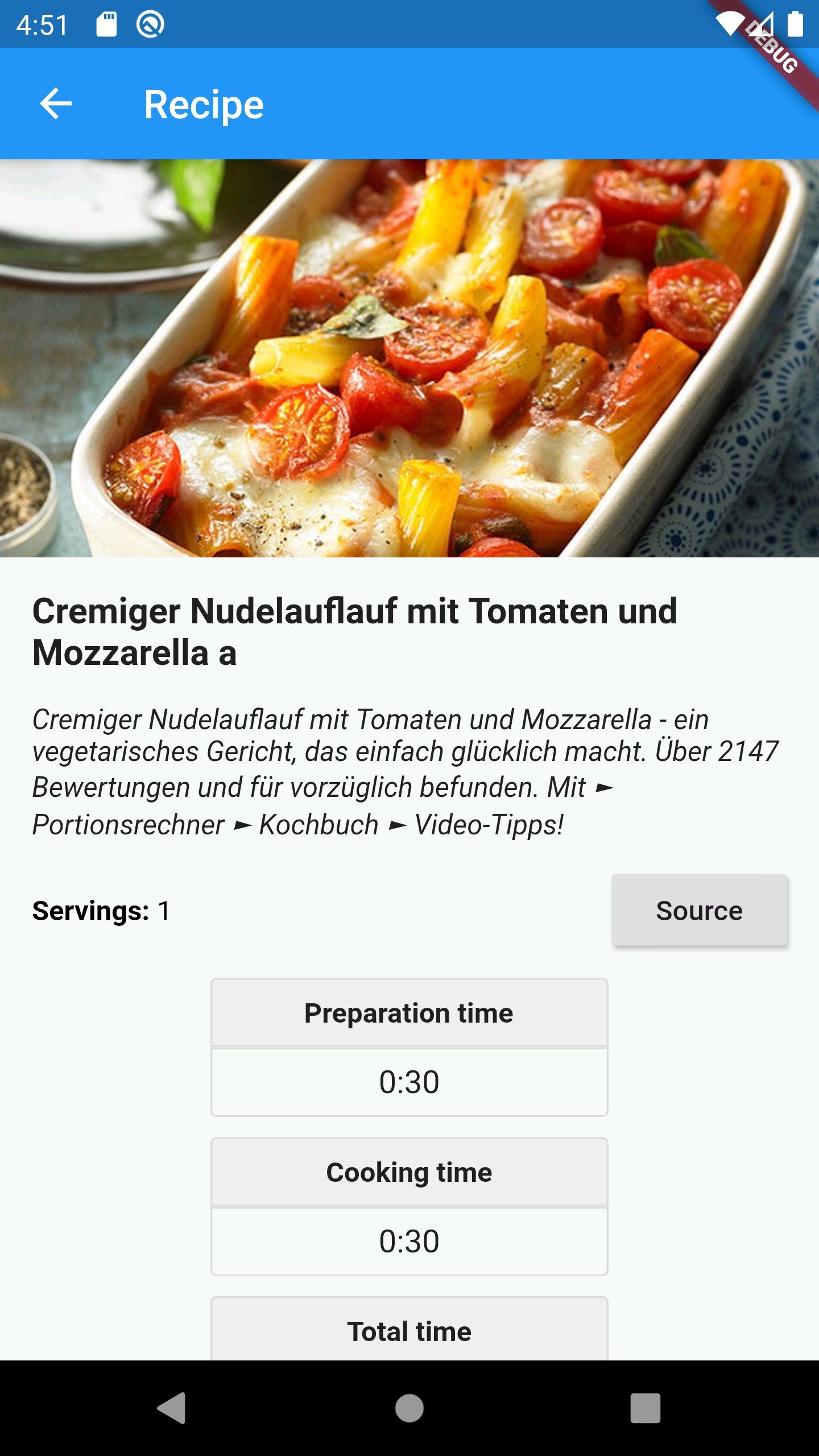
-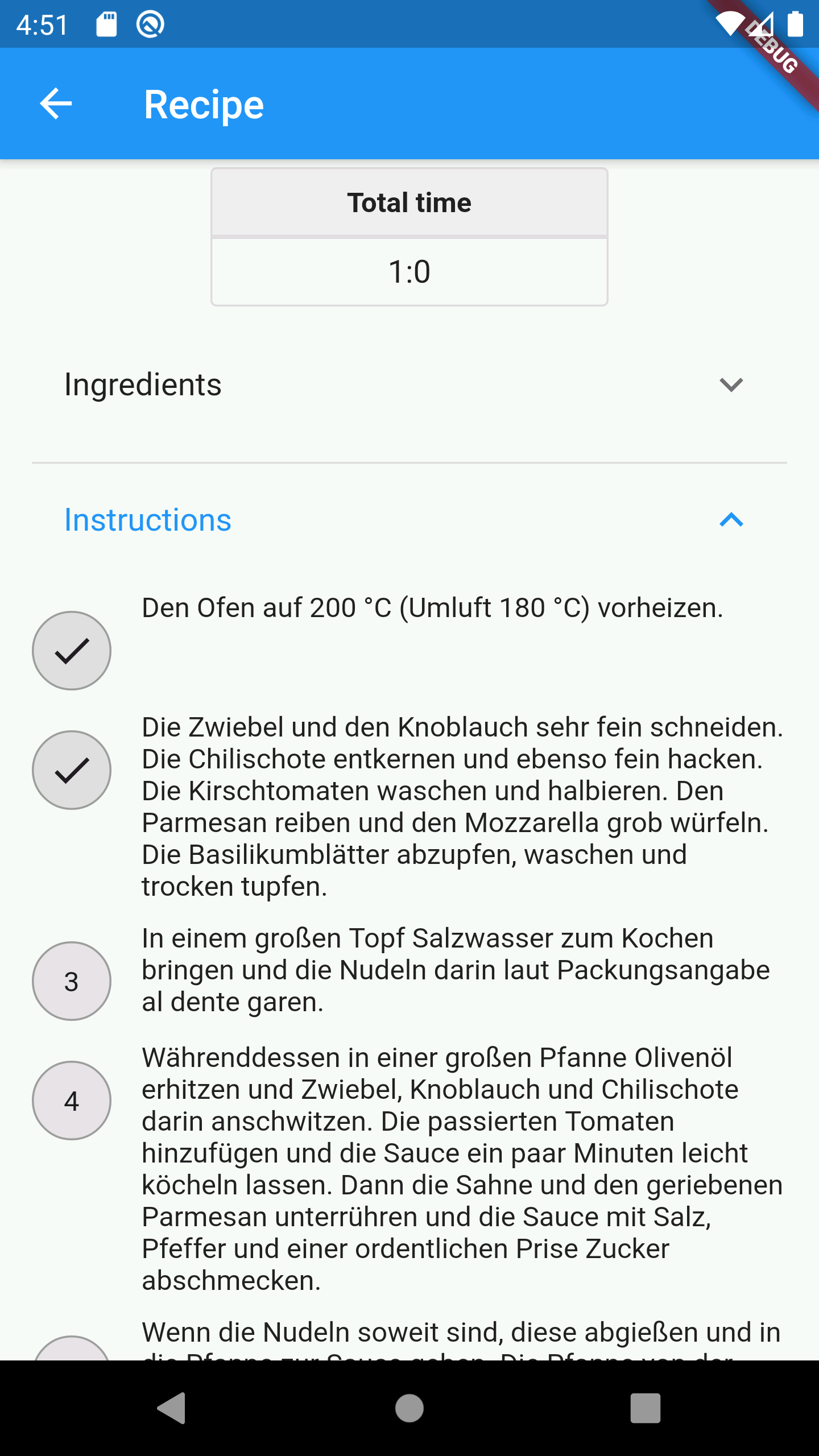
+
+
+
diff --git a/assets/IzzyOnDroid.png b/assets/IzzyOnDroid.png
new file mode 100644
index 00000000..af5bf5bd
Binary files /dev/null and b/assets/IzzyOnDroid.png differ
diff --git a/lib/src/blocs/login/login_bloc.dart b/lib/src/blocs/login/login_bloc.dart
index ddf699af..4d2d0782 100644
--- a/lib/src/blocs/login/login_bloc.dart
+++ b/lib/src/blocs/login/login_bloc.dart
@@ -1,10 +1,10 @@
import 'dart:async';
-import 'package:meta/meta.dart';
import 'package:bloc/bloc.dart';
+import 'package:meta/meta.dart';
import 'package:nextcloud_cookbook_flutter/src/blocs/authentication/authentication.dart';
-import '../../services/user_repository.dart';
+import '../../services/user_repository.dart';
import 'login.dart';
class LoginBloc extends Bloc {
@@ -23,9 +23,8 @@ class LoginBloc extends Bloc {
yield LoginLoading();
try {
- final appAuthentication = await userRepository.authenticate(
- serverUrl: event.serverURL
- );
+ final appAuthentication =
+ await userRepository.authenticate(event.serverURL);
authenticationBloc.add(LoggedIn(appAuthentication: appAuthentication));
yield LoginInitial();
diff --git a/lib/src/blocs/login/login_event.dart b/lib/src/blocs/login/login_event.dart
index d3b3a6df..8b48215f 100644
--- a/lib/src/blocs/login/login_event.dart
+++ b/lib/src/blocs/login/login_event.dart
@@ -1,8 +1,11 @@
-import 'package:meta/meta.dart';
import 'package:equatable/equatable.dart';
+import 'package:meta/meta.dart';
abstract class LoginEvent extends Equatable {
const LoginEvent();
+
+ @override
+ List