*
- * 当用户使用自己的缓存驱动时,直接实例化对象后可直接设置 AccessToekn
+ * 当用户使用自己的缓存驱动时,直接实例化对象后可直接设置 AccessToken
* - 多用于分布式项目时保持 AccessToken 统一
- * - 使用此方法后就由用户来保证传入的 AccessToekn 为有效 AccessToekn
+ * - 使用此方法后就由用户来保证传入的 AccessToken 为有效 AccessToken
*/
- public function setAccessToken($access_token)
+ public function setAccessToken($accessToken)
{
- if (!is_string($access_token)) {
+ if (!is_string($accessToken)) {
throw new InvalidArgumentException("Invalid AccessToken type, need string.");
}
$cache = $this->config->get('appid') . '_access_token';
- Tools::setCache($cache, $this->access_token = $access_token);
+ Tools::setCache($cache, $this->access_token = $accessToken);
}
/**
@@ -159,22 +161,21 @@ public function delAccessToken()
* 以GET获取接口数据并转为数组
* @param string $url 接口地址
* @return array
- * @throws InvalidResponseException
+ * @throws \WeChat\Exceptions\InvalidResponseException
* @throws \WeChat\Exceptions\LocalCacheException
*/
protected function httpGetForJson($url)
{
try {
return Tools::json2arr(Tools::get($url));
- } catch (InvalidResponseException $e) {
+ } catch (InvalidResponseException $exception) {
if (isset($this->currentMethod['method']) && empty($this->isTry)) {
- if (in_array($e->getCode(), ['40014', '40001', '41001', '42001'])) {
- $this->delAccessToken();
- $this->isTry = true;
+ if (in_array($exception->getCode(), ['40014', '40001', '41001', '42001'])) {
+ [$this->delAccessToken(), $this->isTry = true];
return call_user_func_array([$this, $this->currentMethod['method']], $this->currentMethod['arguments']);
}
}
- throw new InvalidResponseException($e->getMessage(), $e->getCode());
+ throw new InvalidResponseException($exception->getMessage(), $exception->getCode());
}
}
@@ -184,19 +185,21 @@ protected function httpGetForJson($url)
* @param array $data 请求数据
* @param bool $buildToJson
* @return array
- * @throws InvalidResponseException
+ * @throws \WeChat\Exceptions\InvalidResponseException
* @throws \WeChat\Exceptions\LocalCacheException
*/
protected function httpPostForJson($url, array $data, $buildToJson = true)
{
try {
- return Tools::json2arr(Tools::post($url, $buildToJson ? Tools::arr2json($data) : $data));
- } catch (InvalidResponseException $e) {
- if (!$this->isTry && in_array($e->getCode(), ['40014', '40001', '41001', '42001'])) {
+ $options = [];
+ if ($buildToJson) $options['headers'] = ['Content-Type: application/json'];
+ return Tools::json2arr(Tools::post($url, $buildToJson ? Tools::arr2json($data) : $data, $options));
+ } catch (InvalidResponseException $exception) {
+ if (!$this->isTry && in_array($exception->getCode(), ['40014', '40001', '41001', '42001'])) {
[$this->delAccessToken(), $this->isTry = true];
return call_user_func_array([$this, $this->currentMethod['method']], $this->currentMethod['arguments']);
}
- throw new InvalidResponseException($e->getMessage(), $e->getCode());
+ throw new InvalidResponseException($exception->getMessage(), $exception->getCode());
}
}
@@ -205,17 +208,15 @@ protected function httpPostForJson($url, array $data, $buildToJson = true)
* @param string $url 接口地址
* @param string $method 当前接口方法
* @param array $arguments 请求参数
- * @return mixed
+ * @return string
* @throws \WeChat\Exceptions\InvalidResponseException
* @throws \WeChat\Exceptions\LocalCacheException
*/
protected function registerApi(&$url, $method, $arguments = [])
{
$this->currentMethod = ['method' => $method, 'arguments' => $arguments];
- if (empty($this->access_token)) {
- $this->access_token = $this->getAccessToken();
- }
- return $url = str_replace('ACCESS_TOKEN', $this->access_token, $url);
+ if (empty($this->access_token)) $this->access_token = $this->getAccessToken();
+ return $url = str_replace('ACCESS_TOKEN', urlencode($this->access_token), $url);
}
/**
@@ -224,7 +225,7 @@ protected function registerApi(&$url, $method, $arguments = [])
* @param array $data POST提交接口参数
* @param bool $isBuildJson
* @return array
- * @throws InvalidResponseException
+ * @throws \WeChat\Exceptions\InvalidResponseException
* @throws \WeChat\Exceptions\LocalCacheException
*/
public function callPostApi($url, array $data, $isBuildJson = true)
@@ -237,7 +238,7 @@ public function callPostApi($url, array $data, $isBuildJson = true)
* 接口通用GET请求方法
* @param string $url 接口URL
* @return array
- * @throws InvalidResponseException
+ * @throws \WeChat\Exceptions\InvalidResponseException
* @throws \WeChat\Exceptions\LocalCacheException
*/
public function callGetApi($url)
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Contracts/BasicWePay.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Contracts/BasicWePay.php
index 55a70800456..5697485ad53 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Contracts/BasicWePay.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Contracts/BasicWePay.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
namespace WeChat\Contracts;
@@ -90,12 +92,13 @@ public static function instance(array $config)
/**
* 获取微信支付通知
+ * @param string $xml
* @return array
- * @throws InvalidResponseException
+ * @throws \WeChat\Exceptions\InvalidResponseException
*/
- public function getNotify()
+ public function getNotify($xml = '')
{
- $data = Tools::xml2arr(file_get_contents('php://input'));
+ $data = Tools::xml2arr(empty($xml) ? Tools::getRawInput() : $xml);
if (isset($data['sign']) && $this->getPaySign($data) === $data['sign']) {
return $data;
}
@@ -122,7 +125,10 @@ public function getPaySign(array $data, $signType = 'MD5', $buff = '')
{
ksort($data);
if (isset($data['sign'])) unset($data['sign']);
- foreach ($data as $k => $v) $buff .= "{$k}={$v}&";
+ foreach ($data as $k => $v) {
+ if ('' === $v || null === $v) continue;
+ $buff .= "{$k}={$v}&";
+ }
$buff .= ("key=" . $this->config->get('mch_key'));
if (strtoupper($signType) === 'MD5') {
return strtoupper(md5($buff));
@@ -134,7 +140,7 @@ public function getPaySign(array $data, $signType = 'MD5', $buff = '')
* 转换短链接
* @param string $longUrl 需要转换的URL,签名用原串,传输需URLencode
* @return array
- * @throws InvalidResponseException
+ * @throws \WeChat\Exceptions\InvalidResponseException
* @throws \WeChat\Exceptions\LocalCacheException
*/
public function shortUrl($longUrl)
@@ -143,34 +149,32 @@ public function shortUrl($longUrl)
return $this->callPostApi($url, ['long_url' => $longUrl]);
}
-
/**
* 数组直接转xml数据输出
* @param array $data
* @param bool $isReturn
- * @return string
+ * @return string|void
*/
public function toXml(array $data, $isReturn = false)
{
$xml = Tools::arr2xml($data);
- if ($isReturn) {
- return $xml;
- }
+ if ($isReturn) return $xml;
echo $xml;
}
/**
- * 以Post请求接口
+ * 以 Post 请求接口
* @param string $url 请求
* @param array $data 接口参数
* @param bool $isCert 是否需要使用双向证书
* @param string $signType 数据签名类型 MD5|SHA256
* @param bool $needSignType 是否需要传签名类型参数
+ * @param bool $needNonceStr
* @return array
- * @throws InvalidResponseException
+ * @throws \WeChat\Exceptions\InvalidResponseException
* @throws \WeChat\Exceptions\LocalCacheException
*/
- protected function callPostApi($url, array $data, $isCert = false, $signType = 'HMAC-SHA256', $needSignType = true)
+ protected function callPostApi($url, array $data, $isCert = false, $signType = 'HMAC-SHA256', $needSignType = true, $needNonceStr = true)
{
$option = [];
if ($isCert) {
@@ -192,7 +196,8 @@ protected function callPostApi($url, array $data, $isCert = false, $signType = '
}
}
$params = $this->params->merge($data);
- $needSignType && ($params['sign_type'] = strtoupper($signType));
+ if (!$needNonceStr) unset($params['nonce_str']);
+ if ($needSignType) $params['sign_type'] = strtoupper($signType);
$params['sign'] = $this->getPaySign($params, $signType);
$result = Tools::xml2arr(Tools::post($url, Tools::arr2xml($params), $option));
if ($result['return_code'] !== 'SUCCESS') {
@@ -200,4 +205,4 @@ protected function callPostApi($url, array $data, $isCert = false, $signType = '
}
return $result;
}
-}
\ No newline at end of file
+}
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Contracts/BasicWeWork.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Contracts/BasicWeWork.php
new file mode 100644
index 00000000000..bde60b6705f
--- /dev/null
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Contracts/BasicWeWork.php
@@ -0,0 +1,43 @@
+access_token) return $this->access_token;
+ $ckey = $this->config->get('appid') . '_access_token';
+ if ($this->access_token = Tools::getCache($ckey)) return $this->access_token;
+ list($appid, $secret) = [$this->config->get('appid'), $this->config->get('appsecret')];
+ $result = Tools::json2arr(Tools::get("https://qyapi.weixin.qq.com/cgi-bin/gettoken?corpid={$appid}&corpsecret={$secret}"));
+ if (isset($result['access_token']) && $result['access_token']) Tools::setCache($ckey, $result['access_token'], 7000);
+ return $this->access_token = $result['access_token'];
+ }
+
+}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Contracts/DataArray.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Contracts/DataArray.php
index c932c7daf5a..628da33215e 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Contracts/DataArray.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Contracts/DataArray.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
namespace WeChat\Contracts;
@@ -51,7 +53,7 @@ public function set($offset, $value)
/**
* 获取配置项参数
* @param string|null $offset
- * @return array|string|null
+ * @return array|string|null|mixed
*/
public function get($offset = null)
{
@@ -76,7 +78,9 @@ public function merge(array $data, $append = false)
* 设置配置项值
* @param string $offset
* @param string|array|null|integer $value
+ * @return void
*/
+ #[\ReturnTypeWillChange]
public function offsetSet($offset, $value)
{
if (is_null($offset)) {
@@ -91,6 +95,7 @@ public function offsetSet($offset, $value)
* @param string $offset
* @return bool
*/
+ #[\ReturnTypeWillChange]
public function offsetExists($offset)
{
return isset($this->config[$offset]);
@@ -99,7 +104,9 @@ public function offsetExists($offset)
/**
* 清理配置项
* @param string|null $offset
+ * @return void
*/
+ #[\ReturnTypeWillChange]
public function offsetUnset($offset = null)
{
if (is_null($offset)) {
@@ -112,13 +119,12 @@ public function offsetUnset($offset = null)
/**
* 获取配置项参数
* @param string|null $offset
- * @return array|string|null
+ * @return mixed
*/
+ #[\ReturnTypeWillChange]
public function offsetGet($offset = null)
{
- if (is_null($offset)) {
- return $this->config;
- }
+ if (is_null($offset)) return $this->config;
return isset($this->config[$offset]) ? $this->config[$offset] : null;
}
}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Contracts/DataError.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Contracts/DataError.php
index 51cd9a2eea0..7f064a6d7e5 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Contracts/DataError.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Contracts/DataError.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
namespace WeChat\Contracts;
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Contracts/MyCurlFile.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Contracts/MyCurlFile.php
index e81e3da46c8..5284e0e5ba3 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Contracts/MyCurlFile.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Contracts/MyCurlFile.php
@@ -1,5 +1,19 @@
filename = Tools::pushFile($this->tempname, base64_decode($this->content));
if (class_exists('CURLFile')) {
return new \CURLFile($this->filename, $this->mimetype, $this->postname);
+ } else {
+ return "@{$this->tempname};filename={$this->postname};type={$this->mimetype}";
}
- return "@{$this->tempname};filename={$this->postname};type={$this->mimetype}";
}
/**
- * 类销毁处理
+ * 通用销毁函数清理缓存文件
+ * 提前删除过期因此放到了网络请求之后
*/
public function __destruct()
{
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Contracts/Tools.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Contracts/Tools.php
index 60a435d5ce3..f408b80256b 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Contracts/Tools.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Contracts/Tools.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
namespace WeChat\Contracts;
@@ -63,13 +65,25 @@ public static function createNoncestr($length = 32, $str = "")
return $str;
}
+ /**
+ * 获取输入对象
+ * @return false|mixed|string
+ */
+ public static function getRawInput()
+ {
+ if (empty($GLOBALS['HTTP_RAW_POST_DATA'])) {
+ return file_get_contents('php://input');
+ } else {
+ return $GLOBALS['HTTP_RAW_POST_DATA'];
+ }
+ }
/**
* 根据文件后缀获取文件类型
* @param string|array $ext 文件后缀
* @param array $mine 文件后缀MINE信息
* @return string
- * @throws LocalCacheException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public static function getExtMine($ext, $mine = [])
{
@@ -83,7 +97,7 @@ public static function getExtMine($ext, $mine = [])
/**
* 获取所有文件扩展的类型
* @return array
- * @throws LocalCacheException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
private static function getMines()
{
@@ -99,21 +113,22 @@ private static function getMines()
/**
* 创建CURL文件对象
- * @param $filename
+ * @param mixed $filename
* @param string $mimetype
* @param string $postname
* @return \CURLFile|string
- * @throws LocalCacheException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public static function createCurlFile($filename, $mimetype = null, $postname = null)
{
if (is_string($filename) && file_exists($filename)) {
if (is_null($postname)) $postname = basename($filename);
if (is_null($mimetype)) $mimetype = self::getExtMine(pathinfo($filename, 4));
- if (function_exists('curl_file_create')) {
- return curl_file_create($filename, $mimetype, $postname);
+ if (class_exists('CURLFile')) {
+ return new \CURLFile($filename, $mimetype, $postname);
+ } else {
+ return "@{$filename};filename={$postname};type={$mimetype}";
}
- return "@{$filename};filename={$postname};type={$mimetype}";
}
return $filename;
}
@@ -158,20 +173,35 @@ private static function _arr2xml($data, $content = '')
*/
public static function xml2arr($xml)
{
- $entity = libxml_disable_entity_loader(true);
- $data = (array)simplexml_load_string($xml, 'SimpleXMLElement', LIBXML_NOCDATA);
- libxml_disable_entity_loader($entity);
+ if (PHP_VERSION_ID < 80000) {
+ $backup = libxml_disable_entity_loader(true);
+ $data = (array)simplexml_load_string($xml, 'SimpleXMLElement', LIBXML_NOCDATA);
+ libxml_disable_entity_loader($backup);
+ } else {
+ $data = (array)simplexml_load_string($xml, 'SimpleXMLElement', LIBXML_NOCDATA);
+ }
return json_decode(json_encode($data), true);
}
+ /**
+ * 解析XML文本内容
+ * @param string $xml
+ * @return array|false
+ */
+ public static function xml3arr($xml)
+ {
+ $state = xml_parse($parser = xml_parser_create(), $xml, true);
+ return xml_parser_free($parser) && $state ? self::xml2arr($xml) : false;
+ }
+
/**
* 数组转xml内容
* @param array $data
- * @return null|string|string
+ * @return null|string
*/
public static function arr2json($data)
{
- $json = json_encode(self::buildEnEmojiData($data), JSON_UNESCAPED_UNICODE);
+ $json = json_encode($data, JSON_UNESCAPED_UNICODE);
return $json === '[]' ? '{}' : $json;
}
@@ -241,7 +271,7 @@ public static function emojiDecode($content)
* 解析JSON内容到数组
* @param string $json
* @return array
- * @throws InvalidResponseException
+ * @throws \WeChat\Exceptions\InvalidResponseException
*/
public static function json2arr($json)
{
@@ -261,7 +291,7 @@ public static function json2arr($json)
* @param array $query GET数
* @param array $options
* @return boolean|string
- * @throws LocalCacheException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public static function get($url, $query = [], $options = [])
{
@@ -275,7 +305,7 @@ public static function get($url, $query = [], $options = [])
* @param array $data POST数据
* @param array $options
* @return boolean|string
- * @throws LocalCacheException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public static function post($url, $data = [], $options = [])
{
@@ -289,7 +319,7 @@ public static function post($url, $data = [], $options = [])
* @param string $url 请求方法
* @param array $options 请求参数[headers,data,ssl_cer,ssl_key]
* @return boolean|string
- * @throws LocalCacheException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public static function doRequest($method, $url, $options = [])
{
@@ -342,13 +372,18 @@ public static function doRequest($method, $url, $options = [])
private static function _buildHttpData($data, $build = true)
{
if (!is_array($data)) return $data;
- foreach ($data as $key => $value) if (is_object($value) && $value instanceof \CURLFile) {
+ foreach ($data as $key => $value) if ($value instanceof \CURLFile) {
$build = false;
} elseif (is_object($value) && isset($value->datatype) && $value->datatype === 'MY_CURL_FILE') {
$build = false;
$mycurl = new MyCurlFile((array)$value);
$data[$key] = $mycurl->get();
- array_push(self::$cache_curl, $mycurl->tempname);
+ self::$cache_curl[] = $mycurl->tempname;
+ } elseif (is_array($value) && isset($value['datatype']) && $value['datatype'] === 'MY_CURL_FILE') {
+ $build = false;
+ $mycurl = new MyCurlFile($value);
+ $data[$key] = $mycurl->get();
+ self::$cache_curl[] = $mycurl->tempname;
} elseif (is_string($value) && class_exists('CURLFile', false) && stripos($value, '@') === 0) {
if (($filename = realpath(trim($value, '@'))) && file_exists($filename)) {
$build = false;
@@ -363,7 +398,7 @@ private static function _buildHttpData($data, $build = true)
* @param string $name 文件名称
* @param string $content 文件内容
* @return string
- * @throws LocalCacheException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public static function pushFile($name, $content)
{
@@ -383,7 +418,7 @@ public static function pushFile($name, $content)
* @param string $value 缓存内容
* @param int $expired 缓存时间(0表示永久缓存)
* @return string
- * @throws LocalCacheException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public static function setCache($name, $value = '', $expired = 3600)
{
@@ -409,7 +444,7 @@ public static function getCache($name)
return call_user_func_array(self::$cache_callable['get'], func_get_args());
}
$file = self::_getCacheName($name);
- if (file_exists($file) && ($content = file_get_contents($file))) {
+ if (file_exists($file) && is_file($file) && ($content = file_get_contents($file))) {
$data = unserialize($content);
if (isset($data['expired']) && (intval($data['expired']) === 0 || intval($data['expired']) >= time())) {
return $data['value'];
@@ -430,7 +465,7 @@ public static function delCache($name)
return call_user_func_array(self::$cache_callable['del'], func_get_args());
}
$file = self::_getCacheName($name);
- return file_exists($file) ? unlink($file) : true;
+ return !file_exists($file) || @unlink($file);
}
/**
@@ -447,4 +482,4 @@ private static function _getCacheName($name)
file_exists(self::$cache_path) || mkdir(self::$cache_path, 0755, true);
return self::$cache_path . $name;
}
-}
\ No newline at end of file
+}
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Custom.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Custom.php
index 6d030914007..bfca666fafa 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Custom.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Custom.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
namespace WeChat;
@@ -34,56 +36,49 @@ class Custom extends BasicWeChat
*/
public function addAccount($kf_account, $nickname)
{
- $data = ['kf_account' => $kf_account, 'nickname' => $nickname];
$url = "https://api.weixin.qq.com/customservice/kfaccount/add?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, $data);
+ return $this->callPostApi($url, ['kf_account' => $kf_account, 'nickname' => $nickname]);
}
/**
* 修改客服帐号
- * @param string $kf_account 客服账号
+ * @param string $kfAccount 客服账号
* @param string $nickname 客服昵称
* @return array
* @throws Exceptions\InvalidResponseException
* @throws Exceptions\LocalCacheException
*/
- public function updateAccount($kf_account, $nickname)
+ public function updateAccount($kfAccount, $nickname)
{
- $data = ['kf_account' => $kf_account, 'nickname' => $nickname];
$url = "https://api.weixin.qq.com/customservice/kfaccount/update?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, $data);
+ return $this->callPostApi($url, ['kf_account' => $kfAccount, 'nickname' => $nickname]);
}
/**
* 删除客服帐号
- * @param string $kf_account 客服账号
+ * @param string $kfAccount 客服账号
* @return array
* @throws Exceptions\InvalidResponseException
* @throws Exceptions\LocalCacheException
*/
- public function deleteAccount($kf_account)
+ public function deleteAccount($kfAccount)
{
- $data = ['kf_account' => $kf_account];
$url = "https://api.weixin.qq.com/customservice/kfaccount/del?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, $data);
+ return $this->callPostApi($url, ['kf_account' => $kfAccount]);
}
/**
* 邀请绑定客服帐号
- * @param string $kf_account 完整客服帐号,格式为:帐号前缀@公众号微信号
+ * @param string $kfAccount 完整客服帐号,格式为:帐号前缀@公众号微信号
* @param string $invite_wx 接收绑定邀请的客服微信号
* @return array
* @throws Exceptions\InvalidResponseException
* @throws Exceptions\LocalCacheException
*/
- public function inviteWorker($kf_account, $invite_wx)
+ public function inviteWorker($kfAccount, $invite_wx)
{
$url = 'https://api.weixin.qq.com/customservice/kfaccount/inviteworker?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->callPostApi($url, ['kf_account' => $kf_account, 'invite_wx' => $invite_wx]);
+ return $this->callPostApi($url, ['kf_account' => $kfAccount, 'invite_wx' => $invite_wx]);
}
/**
@@ -95,8 +90,7 @@ public function inviteWorker($kf_account, $invite_wx)
public function getAccountList()
{
$url = "https://api.weixin.qq.com/cgi-bin/customservice/getkflist?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpGetForJson($url);
+ return $this->callGetApi($url);
}
/**
@@ -109,9 +103,8 @@ public function getAccountList()
*/
public function uploadHeadimg($kf_account, $image)
{
- $url = "http://api.weixin.qq.com/customservice/kfaccount/uploadheadimg?access_token=ACCESS_TOKEN&kf_account={$kf_account}";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, ['media' => Tools::createCurlFile($image)]);
+ $url = "https://api.weixin.qq.com/customservice/kfaccount/uploadheadimg?access_token=ACCESS_TOKEN&kf_account={$kf_account}";
+ return $this->callPostApi($url, ['media' => Tools::createCurlFile($image)], false);
}
/**
@@ -124,8 +117,7 @@ public function uploadHeadimg($kf_account, $image)
public function send(array $data)
{
$url = "https://api.weixin.qq.com/cgi-bin/message/custom/send?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, $data);
+ return $this->callPostApi($url, $data);
}
/**
@@ -139,8 +131,7 @@ public function send(array $data)
public function typing($openid, $command = 'Typing')
{
$url = "https://api.weixin.qq.com/cgi-bin/message/custom/typing?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, ['touser' => $openid, 'command' => $command]);
+ return $this->callPostApi($url, ['touser' => $openid, 'command' => $command]);
}
/**
@@ -153,8 +144,7 @@ public function typing($openid, $command = 'Typing')
public function massSendAll(array $data)
{
$url = "https://api.weixin.qq.com/cgi-bin/message/mass/sendall?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, $data);
+ return $this->callPostApi($url, $data);
}
/**
@@ -167,8 +157,7 @@ public function massSendAll(array $data)
public function massSend(array $data)
{
$url = "https://api.weixin.qq.com/cgi-bin/message/mass/send?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, $data);
+ return $this->callPostApi($url, $data);
}
/**
@@ -184,8 +173,7 @@ public function massDelete($msg_id, $article_idx = null)
$data = ['msg_id' => $msg_id];
is_null($article_idx) || $data['article_idx'] = $article_idx;
$url = "https://api.weixin.qq.com/cgi-bin/message/mass/delete?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, $data);
+ return $this->callPostApi($url, $data);
}
/**
@@ -198,22 +186,20 @@ public function massDelete($msg_id, $article_idx = null)
public function massPreview(array $data)
{
$url = "https://api.weixin.qq.com/cgi-bin/message/mass/preview?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, $data);
+ return $this->callPostApi($url, $data);
}
/**
* 查询群发消息发送状态【订阅号与服务号认证后均可用】
- * @param integer $msg_id 群发消息后返回的消息id
+ * @param integer $msgId 群发消息后返回的消息id
* @return array
* @throws Exceptions\InvalidResponseException
* @throws Exceptions\LocalCacheException
*/
- public function massGet($msg_id)
+ public function massGet($msgId)
{
$url = "https://api.weixin.qq.com/cgi-bin/message/mass/get?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, ['msg_id' => $msg_id]);
+ return $this->callPostApi($url, ['msg_id' => $msgId]);
}
/**
@@ -225,8 +211,7 @@ public function massGet($msg_id)
public function massGetSeed()
{
$url = "https://api.weixin.qq.com/cgi-bin/message/mass/speed/get?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, []);
+ return $this->callPostApi($url, []);
}
/**
@@ -239,9 +224,6 @@ public function massGetSeed()
public function massSetSeed($speed)
{
$url = "https://api.weixin.qq.com/cgi-bin/message/mass/speed/set?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, ['speed' => $speed]);
+ return $this->callPostApi($url, ['speed' => $speed]);
}
-
-
}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Draft.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Draft.php
new file mode 100644
index 00000000000..0a097afe924
--- /dev/null
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Draft.php
@@ -0,0 +1,124 @@
+callPostApi($url, ['articles' => $articles]);
+ }
+
+ /**
+ * 获取草稿
+ * @param string $mediaId
+ * @param string $outType 返回处理函数
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function get($mediaId, $outType = null)
+ {
+ $url = "https://api.weixin.qq.com/cgi-bin/draft/get?access_token=ACCESS_TOKEN";
+ return $this->callPostApi($url, ['media_id' => $mediaId]);
+ }
+
+ /**
+ * 删除草稿
+ * @param string $mediaId
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function delete($mediaId)
+ {
+ $url = "https://api.weixin.qq.com/cgi-bin/draft/delete?access_token=ACCESS_TOKEN";
+ return $this->callPostApi($url, ['media_id' => $mediaId]);
+ }
+
+ /**
+ * 新增图文素材
+ * @param array $data 文件名称
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function addNews($data)
+ {
+ $url = "https://api.weixin.qq.com/cgi-bin/material/add_news?access_token=ACCESS_TOKEN";
+ return $this->callPostApi($url, $data);
+ }
+
+ /**
+ * 修改草稿
+ * @param string $media_id 要修改的图文消息的id
+ * @param int $index 要更新的文章在图文消息中的位置(多图文消息时,此字段才有意义),第一篇为0
+ * @param $articles
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function update($media_id, $index, $articles)
+ {
+ $url = "https://api.weixin.qq.com/cgi-bin/draft/update?access_token=ACCESS_TOKEN";
+ $data = ['media_id' => $media_id, 'index' => $index, 'articles' => $articles];
+ return $this->callPostApi($url, $data);
+ }
+
+ /**
+ * 获取草稿总数
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function getCount()
+ {
+ $url = "https://api.weixin.qq.com/cgi-bin/draft/count?access_token=ACCESS_TOKEN";
+ return $this->callGetApi($url);
+ }
+
+ /**
+ * 获取草稿列表
+ * @param int $offset 从全部素材的该偏移位置开始返回,0表示从第一个素材返回
+ * @param int $count 返回素材的数量,取值在1到20之间
+ * @param int $noContent 1 表示不返回 content 字段,0 表示正常返回,默认为 0
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function batchGet($offset = 0, $count = 20, $noContent = 0)
+ {
+ $url = "https://api.weixin.qq.com/cgi-bin/draft/batchget?access_token=ACCESS_TOKEN";
+ return $this->callPostApi($url, ['no_content' => $noContent, 'offset' => $offset, 'count' => $count]);
+ }
+}
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Exceptions/InvalidArgumentException.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Exceptions/InvalidArgumentException.php
index 993c4e0083a..1c38e67f612 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Exceptions/InvalidArgumentException.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Exceptions/InvalidArgumentException.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
namespace WeChat\Exceptions;
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Exceptions/InvalidDecryptException.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Exceptions/InvalidDecryptException.php
index f45c0581e85..cc0d6e5325c 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Exceptions/InvalidDecryptException.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Exceptions/InvalidDecryptException.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
namespace WeChat\Exceptions;
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Exceptions/InvalidInstanceException.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Exceptions/InvalidInstanceException.php
index e4c6816f82c..e9a3c157d8c 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Exceptions/InvalidInstanceException.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Exceptions/InvalidInstanceException.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
namespace WeChat\Exceptions;
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Exceptions/InvalidResponseException.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Exceptions/InvalidResponseException.php
index c3cb8926735..5e3e9bcb07f 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Exceptions/InvalidResponseException.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Exceptions/InvalidResponseException.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
namespace WeChat\Exceptions;
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Exceptions/LocalCacheException.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Exceptions/LocalCacheException.php
index 35be83a8dcb..14ebc71d83b 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Exceptions/LocalCacheException.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Exceptions/LocalCacheException.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
namespace WeChat\Exceptions;
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Freepublish.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Freepublish.php
new file mode 100644
index 00000000000..1cd96142577
--- /dev/null
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Freepublish.php
@@ -0,0 +1,98 @@
+callPostApi($url, ['media_id' => $mediaId]);
+ }
+
+ /**
+ * 发布状态轮询接口
+ * @param mixed $publishId
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function get($publishId)
+ {
+ $url = "https://api.weixin.qq.com/cgi-bin/freepublish/get?access_token=ACCESS_TOKEN";
+ return $this->callPostApi($url, ['publish_id' => $publishId]);
+ }
+
+ /**
+ * 删除发布
+ * 发布成功之后,随时可以通过该接口删除。此操作不可逆,请谨慎操作。
+ * @param mixed $articleId 成功发布时返回的 article_id
+ * @param int $index 要删除的文章在图文消息中的位置,第一篇编号为1,该字段不填或填0会删除全部文章
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function delete($articleId, $index = 0)
+ {
+ $url = "https://api.weixin.qq.com/cgi-bin/freepublish/delete?access_token=ACCESS_TOKEN";
+ return $this->callPostApi($url, ['article_id' => $articleId, 'index' => $index]);
+ }
+
+ /**
+ * 通过 article_id 获取已发布文章
+ * @param mixed $articleId 要获取的草稿的article_id
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function getArticle($articleId)
+ {
+ $url = "https://api.weixin.qq.com/cgi-bin/freepublish/getarticle?access_token=ACCESS_TOKEN";
+ return $this->callPostApi($url, ['article_id' => $articleId]);
+ }
+
+ /**
+ * 获取成功发布列表
+ * @param int $offset 从全部素材的该偏移位置开始返回,0表示从第一个素材返回
+ * @param int $count 返回素材的数量,取值在1到20之间
+ * @param int $noContent 1 表示不返回 content 字段,0 表示正常返回,默认为 0
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function batchGet($offset = 0, $count = 20, $noContent = 0)
+ {
+ $url = "https://api.weixin.qq.com/cgi-bin/freepublish/batchget?access_token=ACCESS_TOKEN";
+ return $this->callPostApi($url, ['no_content' => $noContent, 'offset' => $offset, 'count' => $count]);
+ }
+}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Limit.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Limit.php
index 6ccc6f48830..05973c99b2b 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Limit.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Limit.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
namespace WeChat;
@@ -27,13 +29,12 @@ class Limit extends BasicWeChat
/**
* 公众号调用或第三方平台帮公众号调用对公众号的所有api调用(包括第三方帮其调用)次数进行清零
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function clearQuota()
{
$url = 'https://api.weixin.qq.com/cgi-bin/clear_quota?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, ['appid' => $this->config->get('appid')]);
}
@@ -42,27 +43,24 @@ public function clearQuota()
* @param string $action 执行的检测动作
* @param string $operator 指定平台从某个运营商进行检测
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function ping($action = 'all', $operator = 'DEFAULT')
{
$url = 'https://api.weixin.qq.com/cgi-bin/callback/check?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, ['action' => $action, 'check_operator' => $operator]);
}
/**
* 获取微信服务器IP地址
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function getCallbackIp()
{
$url = 'https://api.weixin.qq.com/cgi-bin/getcallbackip?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpGetForJson($url);
+ return $this->callGetApi($url);
}
-
}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Media.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Media.php
index 8c7ca02d238..22feec275a1 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Media.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Media.php
@@ -3,19 +3,21 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
namespace WeChat;
-use WeChat\Contracts\Tools;
use WeChat\Contracts\BasicWeChat;
+use WeChat\Contracts\Tools;
use WeChat\Exceptions\InvalidResponseException;
/**
@@ -30,8 +32,8 @@ class Media extends BasicWeChat
* @param string $filename 文件名称
* @param string $type 媒体文件类型(image|voice|video|thumb)
* @return array
- * @throws Exceptions\LocalCacheException
- * @throws InvalidResponseException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function add($filename, $type = 'image')
{
@@ -39,8 +41,7 @@ public function add($filename, $type = 'image')
throw new InvalidResponseException('Invalid Media Type.', '0');
}
$url = "https://api.weixin.qq.com/cgi-bin/media/upload?access_token=ACCESS_TOKEN&type={$type}";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, ['media' => Tools::createCurlFile($filename)], false);
+ return $this->callPostApi($url, ['media' => Tools::createCurlFile($filename)], false);
}
/**
@@ -48,13 +49,14 @@ public function add($filename, $type = 'image')
* @param string $media_id
* @param string $outType 返回处理函数
* @return array|string
- * @throws Exceptions\LocalCacheException
- * @throws InvalidResponseException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function get($media_id, $outType = null)
{
$url = "https://api.weixin.qq.com/cgi-bin/media/get?access_token=ACCESS_TOKEN&media_id={$media_id}";
$this->registerApi($url, __FUNCTION__, func_get_args());
+ if ($outType == 'url') return $url;
$result = Tools::get($url);
if (is_array($json = json_decode($result, true))) {
if (!$this->isTry && isset($json['errcode']) && in_array($json['errcode'], ['40014', '40001', '41001', '42001'])) {
@@ -70,14 +72,13 @@ public function get($media_id, $outType = null)
* 新增图文素材
* @param array $data 文件名称
* @return array
- * @throws Exceptions\LocalCacheException
- * @throws InvalidResponseException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function addNews($data)
{
$url = "https://api.weixin.qq.com/cgi-bin/material/add_news?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, $data);
+ return $this->callPostApi($url, $data);
}
/**
@@ -86,39 +87,36 @@ public function addNews($data)
* @param int $index 要更新的文章在图文消息中的位置(多图文消息时,此字段才有意义),第一篇为0
* @param array $news 文章内容
* @return array
- * @throws Exceptions\LocalCacheException
- * @throws InvalidResponseException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function updateNews($media_id, $index, $news)
{
- $data = ['media_id' => $media_id, 'index' => $index, 'articles' => $news];
$url = "https://api.weixin.qq.com/cgi-bin/material/update_news?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, $data);
+ return $this->callPostApi($url, ['media_id' => $media_id, 'index' => $index, 'articles' => $news]);
}
/**
* 上传图文消息内的图片获取URL
- * @param string $filename
+ * @param mixed $filename
* @return array
- * @throws Exceptions\LocalCacheException
- * @throws InvalidResponseException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function uploadImg($filename)
{
$url = "https://api.weixin.qq.com/cgi-bin/media/uploadimg?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, ['media' => Tools::createCurlFile($filename)], false);
+ return $this->callPostApi($url, ['media' => Tools::createCurlFile($filename)], false);
}
/**
* 新增其他类型永久素材
- * @param string $filename 文件名称
+ * @param mixed $filename 文件名称
* @param string $type 媒体文件类型(image|voice|video|thumb)
* @param array $description 包含素材的描述信息
* @return array
- * @throws Exceptions\LocalCacheException
- * @throws InvalidResponseException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function addMaterial($filename, $type = 'image', $description = [])
{
@@ -126,8 +124,7 @@ public function addMaterial($filename, $type = 'image', $description = [])
throw new InvalidResponseException('Invalid Media Type.', '0');
}
$url = "https://api.weixin.qq.com/cgi-bin/material/add_material?access_token=ACCESS_TOKEN&type={$type}";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, ['media' => Tools::createCurlFile($filename), 'description' => Tools::arr2json($description)], false);
+ return $this->callPostApi($url, ['media' => Tools::createCurlFile($filename), 'description' => Tools::arr2json($description)], false);
}
/**
@@ -135,13 +132,14 @@ public function addMaterial($filename, $type = 'image', $description = [])
* @param string $media_id
* @param null|string $outType 输出类型
* @return array|string
- * @throws Exceptions\LocalCacheException
- * @throws InvalidResponseException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function getMaterial($media_id, $outType = null)
{
$url = "https://api.weixin.qq.com/cgi-bin/material/get_material?access_token=ACCESS_TOKEN";
$this->registerApi($url, __FUNCTION__, func_get_args());
+ if ($outType == 'url') return $url;
$result = Tools::post($url, ['media_id' => $media_id]);
if (is_array($json = json_decode($result, true))) {
if (!$this->isTry && isset($json['errcode']) && in_array($json['errcode'], ['40014', '40001', '41001', '42001'])) {
@@ -155,29 +153,27 @@ public function getMaterial($media_id, $outType = null)
/**
* 删除永久素材
- * @param string $media_id
+ * @param string $mediaId
* @return array
- * @throws Exceptions\LocalCacheException
- * @throws InvalidResponseException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
- public function delMaterial($media_id)
+ public function delMaterial($mediaId)
{
$url = "https://api.weixin.qq.com/cgi-bin/material/del_material?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, ['media_id' => $media_id]);
+ return $this->httpPostForJson($url, ['media_id' => $mediaId]);
}
/**
* 获取素材总数
* @return array
- * @throws Exceptions\LocalCacheException
- * @throws InvalidResponseException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function getMaterialCount()
{
$url = "https://api.weixin.qq.com/cgi-bin/material/get_materialcount?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpGetForJson($url);
+ return $this->callGetApi($url);
}
/**
@@ -186,8 +182,8 @@ public function getMaterialCount()
* @param int $offset
* @param int $count
* @return array
- * @throws Exceptions\LocalCacheException
- * @throws InvalidResponseException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function batchGetMaterial($type = 'image', $offset = 0, $count = 20)
{
@@ -195,7 +191,6 @@ public function batchGetMaterial($type = 'image', $offset = 0, $count = 20)
throw new InvalidResponseException('Invalid Media Type.', '0');
}
$url = "https://api.weixin.qq.com/cgi-bin/material/batchget_material?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, ['type' => $type, 'offset' => $offset, 'count' => $count]);
+ return $this->callPostApi($url, ['type' => $type, 'offset' => $offset, 'count' => $count]);
}
}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Menu.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Menu.php
index c006a8eae02..4f31c770dc1 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Menu.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Menu.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
namespace WeChat;
@@ -27,83 +29,76 @@ class Menu extends BasicWeChat
/**
* 自定义菜单查询接口
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function get()
{
$url = "https://api.weixin.qq.com/cgi-bin/menu/get?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpGetForJson($url);
+ return $this->callGetApi($url);
}
/**
* 自定义菜单删除接口
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function delete()
{
$url = "https://api.weixin.qq.com/cgi-bin/menu/delete?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpGetForJson($url);
+ return $this->callGetApi($url);
}
/**
* 自定义菜单创建
* @param array $data
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function create(array $data)
{
$url = "https://api.weixin.qq.com/cgi-bin/menu/create?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, $data);
+ return $this->callPostApi($url, $data);
}
/**
* 创建个性化菜单
* @param array $data
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function addConditional(array $data)
{
$url = "https://api.weixin.qq.com/cgi-bin/menu/addconditional?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, $data);
+ return $this->callPostApi($url, $data);
}
/**
* 删除个性化菜单
* @param string $menuid
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function delConditional($menuid)
{
$url = "https://api.weixin.qq.com/cgi-bin/menu/delconditional?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, ['menuid' => $menuid]);
+ return $this->callPostApi($url, ['menuid' => $menuid]);
}
/**
* 测试个性化菜单匹配结果
* @param string $openid
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function tryConditional($openid)
{
$url = "https://api.weixin.qq.com/cgi-bin/menu/trymatch?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, ['user_id' => $openid]);
+ return $this->callPostApi($url, ['user_id' => $openid]);
}
-
}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Oauth.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Oauth.php
index a8be2729e60..0dd5b132c9c 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Oauth.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Oauth.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
namespace WeChat;
@@ -40,15 +42,16 @@ public function getOauthRedirect($redirect_url, $state = '', $scope = 'snsapi_ba
/**
* 通过 code 获取 AccessToken 和 openid
- * @return bool|array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @param string $code 授权Code值,不传则取GET参数
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
- public function getOauthAccessToken()
+ public function getOauthAccessToken($code = '')
{
$appid = $this->config->get('appid');
$appsecret = $this->config->get('appsecret');
- $code = isset($_GET['code']) ? $_GET['code'] : '';
+ $code = $code ? $code : (isset($_GET['code']) ? $_GET['code'] : '');
$url = "https://api.weixin.qq.com/sns/oauth2/access_token?appid={$appid}&secret={$appsecret}&code={$code}&grant_type=authorization_code";
return $this->httpGetForJson($url);
}
@@ -56,9 +59,9 @@ public function getOauthAccessToken()
/**
* 刷新AccessToken并续期
* @param string $refresh_token
- * @return bool|array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function getOauthRefreshToken($refresh_token)
{
@@ -69,31 +72,30 @@ public function getOauthRefreshToken($refresh_token)
/**
* 检验授权凭证(access_token)是否有效
- * @param string $access_token 网页授权接口调用凭证,注意:此access_token与基础支持的access_token不同
+ * @param string $accessToken 网页授权接口调用凭证,注意:此access_token与基础支持的access_token不同
* @param string $openid 用户的唯一标识
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
- public function checkOauthAccessToken($access_token, $openid)
+ public function checkOauthAccessToken($accessToken, $openid)
{
- $url = "https://api.weixin.qq.com/sns/auth?access_token={$access_token}&openid={$openid}";
+ $url = "https://api.weixin.qq.com/sns/auth?access_token={$accessToken}&openid={$openid}";
return $this->httpGetForJson($url);
}
/**
* 拉取用户信息(需scope为 snsapi_userinfo)
- * @param string $access_token 网页授权接口调用凭证,注意:此access_token与基础支持的access_token不同
+ * @param string $accessToken 网页授权接口调用凭证,注意:此access_token与基础支持的access_token不同
* @param string $openid 用户的唯一标识
* @param string $lang 返回国家地区语言版本,zh_CN 简体,zh_TW 繁体,en 英语
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
- public function getUserInfo($access_token, $openid, $lang = 'zh_CN')
+ public function getUserInfo($accessToken, $openid, $lang = 'zh_CN')
{
- $url = "https://api.weixin.qq.com/sns/userinfo?access_token={$access_token}&openid={$openid}&lang={$lang}";
+ $url = "https://api.weixin.qq.com/sns/userinfo?access_token={$accessToken}&openid={$openid}&lang={$lang}";
return $this->httpGetForJson($url);
}
-
}
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Pay.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Pay.php
index dcc87cede65..59fac873cb0 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Pay.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Pay.php
@@ -3,19 +3,20 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
namespace WeChat;
use WeChat\Contracts\BasicWePay;
-use WeChat\Exceptions\InvalidResponseException;
use WePay\Bill;
use WePay\Order;
use WePay\Refund;
@@ -34,8 +35,8 @@ class Pay extends BasicWePay
* 统一下单
* @param array $options
* @return array
- * @throws Exceptions\LocalCacheException
- * @throws InvalidResponseException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function createOrder(array $options)
{
@@ -46,8 +47,8 @@ public function createOrder(array $options)
* 刷卡支付
* @param array $options
* @return array
- * @throws Exceptions\LocalCacheException
- * @throws InvalidResponseException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function createMicropay($options)
{
@@ -88,8 +89,8 @@ public function createParamsForRuleQrc($product_id)
* 查询订单
* @param array $options
* @return array
- * @throws Exceptions\LocalCacheException
- * @throws InvalidResponseException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function queryOrder(array $options)
{
@@ -100,8 +101,8 @@ public function queryOrder(array $options)
* 关闭订单
* @param string $out_trade_no 商户订单号
* @return array
- * @throws Exceptions\LocalCacheException
- * @throws InvalidResponseException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function closeOrder($out_trade_no)
{
@@ -112,8 +113,8 @@ public function closeOrder($out_trade_no)
* 申请退款
* @param array $options
* @return array
- * @throws Exceptions\LocalCacheException
- * @throws InvalidResponseException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function createRefund(array $options)
{
@@ -124,8 +125,8 @@ public function createRefund(array $options)
* 查询退款
* @param array $options
* @return array
- * @throws Exceptions\LocalCacheException
- * @throws InvalidResponseException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function queryRefund(array $options)
{
@@ -136,8 +137,8 @@ public function queryRefund(array $options)
* 交易保障
* @param array $options
* @return array
- * @throws Exceptions\LocalCacheException
- * @throws InvalidResponseException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function report(array $options)
{
@@ -148,8 +149,8 @@ public function report(array $options)
* 授权码查询openid
* @param string $authCode 扫码支付授权码,设备读取用户微信中的条码或者二维码信息
* @return array
- * @throws Exceptions\LocalCacheException
- * @throws InvalidResponseException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function queryAuthCode($authCode)
{
@@ -161,8 +162,8 @@ public function queryAuthCode($authCode)
* @param array $options 静音参数
* @param null|string $outType 输出类型
* @return bool|string
- * @throws Exceptions\LocalCacheException
- * @throws InvalidResponseException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function billDownload(array $options, $outType = null)
{
@@ -173,8 +174,8 @@ public function billDownload(array $options, $outType = null)
* 拉取订单评价数据
* @param array $options
* @return array
- * @throws Exceptions\LocalCacheException
- * @throws InvalidResponseException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function billCommtent(array $options)
{
@@ -185,8 +186,8 @@ public function billCommtent(array $options)
* 企业付款到零钱
* @param array $options
* @return array
- * @throws Exceptions\LocalCacheException
- * @throws InvalidResponseException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function createTransfers(array $options)
{
@@ -197,8 +198,8 @@ public function createTransfers(array $options)
* 查询企业付款到零钱
* @param string $partner_trade_no 商户调用企业付款API时使用的商户订单号
* @return array
- * @throws Exceptions\LocalCacheException
- * @throws InvalidResponseException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function queryTransfers($partner_trade_no)
{
@@ -209,9 +210,9 @@ public function queryTransfers($partner_trade_no)
* 企业付款到银行卡
* @param array $options
* @return array
- * @throws Exceptions\LocalCacheException
- * @throws Exceptions\InvalidDecryptException
- * @throws Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\InvalidDecryptException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function createTransfersBank(array $options)
{
@@ -222,8 +223,8 @@ public function createTransfersBank(array $options)
* 商户企业付款到银行卡操作进行结果查询
* @param string $partner_trade_no 商户订单号,需保持唯一
* @return array
- * @throws Exceptions\LocalCacheException
- * @throws InvalidResponseException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function queryTransFresBank($partner_trade_no)
{
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Product.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Product.php
index 74eb3eed4ac..7c6fc443540 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Product.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Product.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
namespace WeChat;
@@ -29,15 +31,14 @@ class Product extends BasicWeChat
* @param string $keystr 商品编码内容
* @param string $status 设置发布状态。on为提交审核,off为取消发布
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function modStatus($keystandard, $keystr, $status = 'on')
{
- $data = ['keystandard' => $keystandard, 'keystr' => $keystr, 'status' => $status];
$url = "https://api.weixin.qq.com/scan/product/modstatus?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, $data);
+ $data = ['keystandard' => $keystandard, 'keystr' => $keystr, 'status' => $status];
+ return $this->callPostApi($url, $data);
}
/**
@@ -45,15 +46,13 @@ public function modStatus($keystandard, $keystr, $status = 'on')
* @param array $openids 测试人员的openid列表
* @param array $usernames 测试人员的微信号列表
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function setTestWhiteList(array $openids = [], array $usernames = [])
{
- $data = ['openid' => $openids, 'username' => $usernames];
$url = "https://api.weixin.qq.com/scan/testwhitelist/set?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, $data);
+ return $this->callPostApi($url, ['openid' => $openids, 'username' => $usernames]);
}
/**
@@ -63,16 +62,15 @@ public function setTestWhiteList(array $openids = [], array $usernames = [])
* @param integer $qrcode_size 二维码的尺寸(整型),数值代表边长像素数,不填写默认值为100
* @param array $extinfo 由商户自定义传入,建议仅使用大小写字母、数字及-_().*这6个常用字符
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function getQrcode($keystandard, $keystr, $qrcode_size, $extinfo = [])
{
+ $url = "https://api.weixin.qq.com/scan/product/getqrcode?access_token=ACCESS_TOKEN";
$data = ['keystandard' => $keystandard, 'keystr' => $keystr, 'qrcode_size' => $qrcode_size];
empty($extinfo) || $data['extinfo'] = $extinfo;
- $url = "https://api.weixin.qq.com/scan/product/getqrcode?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, $data);
+ return $this->callPostApi($url, $data);
}
/**
@@ -80,16 +78,15 @@ public function getQrcode($keystandard, $keystr, $qrcode_size, $extinfo = [])
* @param string $keystandard 商品编码标准
* @param string $keystr 商品编码内容
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function getProduct($keystandard, $keystr)
{
+ $url = "https://api.weixin.qq.com/scan/product/get?access_token=ACCESS_TOKEN";
$data = ['keystandard' => $keystandard, 'keystr' => $keystr];
empty($extinfo) || $data['extinfo'] = $extinfo;
- $url = "https://api.weixin.qq.com/scan/product/get?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, $data);
+ return $this->callPostApi($url, $data);
}
/**
@@ -99,32 +96,29 @@ public function getProduct($keystandard, $keystr)
* @param null|string $status 支持按状态拉取。on为发布状态,off为未发布状态,check为审核中状态,reject为审核未通过状态,all为所有状态
* @param string $keystr 支持按部分编码内容拉取。填写该参数后,可将编码内容中包含所传参数的商品信息拉出。类似关键词搜索
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function getProductList($offset, $limit = 10, $status = null, $keystr = '')
{
+ $url = "https://api.weixin.qq.com/scan/product/get?access_token=ACCESS_TOKEN";
$data = ['offset' => $offset, 'limit' => $limit];
is_null($status) || $data['status'] = $status;
empty($keystr) || $data['keystr'] = $keystr;
- $url = "https://api.weixin.qq.com/scan/product/get?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, $data);
+ return $this->callPostApi($url, $data);
}
-
/**
* 更新商品信息
* @param array $data
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function updateProduct(array $data)
{
$url = "https://api.weixin.qq.com/scan/product/update?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, $data);
+ return $this->callPostApi($url, $data);
}
/**
@@ -132,29 +126,26 @@ public function updateProduct(array $data)
* @param string $keystandard 商品编码标准
* @param string $keystr 商品编码内容
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function clearProduct($keystandard, $keystr)
{
$url = "https://api.weixin.qq.com/scan/product/clear?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, ['keystandard' => $keystandard, 'keystr' => $keystr]);
+ return $this->callPostApi($url, ['keystandard' => $keystandard, 'keystr' => $keystr]);
}
-
/**
* 检查wxticket参数
* @param string $ticket
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function scanTicketCheck($ticket)
{
$url = "https://api.weixin.qq.com/scan/scanticket/check?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, ['ticket' => $ticket]);
+ return $this->callPostApi($url, ['ticket' => $ticket]);
}
/**
@@ -163,15 +154,13 @@ public function scanTicketCheck($ticket)
* @param string $keystr 商品编码内容
* @param string $extinfo 调用“获取商品二维码接口”时传入的extinfo,为标识参数
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function clearScanticket($keystandard, $keystr, $extinfo)
{
- $data = ['keystandard' => $keystandard, 'keystr' => $keystr, 'extinfo' => $extinfo];
$url = "https://api.weixin.qq.com/scan/scanticket/check?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, $data);
+ $data = ['keystandard' => $keystandard, 'keystr' => $keystr, 'extinfo' => $extinfo];
+ return $this->callPostApi($url, $data);
}
-
}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Prpcrypt/ErrorCode.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Prpcrypt/ErrorCode.php
new file mode 100644
index 00000000000..d11e4eb7931
--- /dev/null
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Prpcrypt/ErrorCode.php
@@ -0,0 +1,53 @@
+ '处理成功',
+ '40001' => '校验签名失败',
+ '40002' => '解析xml失败',
+ '40003' => '计算签名失败',
+ '40004' => '不合法的AESKey',
+ '40005' => '校验AppID失败',
+ '40006' => 'AES加密失败',
+ '40007' => 'AES解密失败',
+ '40008' => '公众平台发送的xml不合法',
+ '40009' => 'Base64编码失败',
+ '40010' => 'Base64解码失败',
+ '40011' => '公众帐号生成回包xml失败',
+ ];
+
+ /**
+ * 获取错误消息内容
+ * @param string $code 错误代码
+ * @return bool
+ */
+ public static function getErrText($code)
+ {
+ if (isset(self::$errCode[$code])) {
+ return self::$errCode[$code];
+ }
+ return false;
+ }
+
+}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Prpcrypt/PKCS7Encoder.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Prpcrypt/PKCS7Encoder.php
new file mode 100644
index 00000000000..bac5ab04daa
--- /dev/null
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Prpcrypt/PKCS7Encoder.php
@@ -0,0 +1,46 @@
+ PKCS7Encoder::$blockSize) {
+ $pad = 0;
+ }
+ return substr($text, 0, strlen($text) - $pad);
+ }
+
+}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Contracts/Prpcrypt.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Prpcrypt/Prpcrypt.php
similarity index 51%
rename from upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Contracts/Prpcrypt.php
rename to upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Prpcrypt/Prpcrypt.php
index 2b93c5038a5..cec9af76243 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Contracts/Prpcrypt.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Prpcrypt/Prpcrypt.php
@@ -3,57 +3,16 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2022 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
// +----------------------------------------------------------------------
// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
-/**
- * PKCS7算法 - 加解密
- * Class PKCS7Encoder
- */
-class PKCS7Encoder
-{
-
- public static $blockSize = 32;
-
- /**
- * 对需要加密的明文进行填充补位
- * @param string $text 需要进行填充补位操作的明文
- * @return string 补齐明文字符串
- */
- function encode($text)
- {
- $amount_to_pad = PKCS7Encoder::$blockSize - (strlen($text) % PKCS7Encoder::$blockSize);
- if ($amount_to_pad == 0) {
- $amount_to_pad = PKCS7Encoder::$blockSize;
- }
- list($pad_chr, $tmp) = [chr($amount_to_pad), ''];
- for ($index = 0; $index < $amount_to_pad; $index++) {
- $tmp .= $pad_chr;
- }
- return $text . $tmp;
- }
-
- /**
- * 对解密后的明文进行补位删除
- * @param string $text 解密后的明文
- * @return string 删除填充补位后的明文
- */
- function decode($text)
- {
- $pad = ord(substr($text, -1));
- if ($pad < 1 || $pad > PKCS7Encoder::$blockSize) {
- $pad = 0;
- }
- return substr($text, 0, strlen($text) - $pad);
- }
-
-}
+namespace WeChat\Prpcrypt;
/**
* 公众号消息 - 加解密
@@ -88,7 +47,7 @@ public function encrypt($text, $appid)
$text = $pkcEncoder->encode($random . pack("N", strlen($text)) . $text . $appid);
$encrypted = openssl_encrypt($text, 'AES-256-CBC', substr($this->key, 0, 32), OPENSSL_ZERO_PADDING, $iv);
return [ErrorCode::$OK, $encrypted];
- } catch (Exception $e) {
+ } catch (\Exception $e) {
return [ErrorCode::$EncryptAESError, null];
}
}
@@ -103,7 +62,7 @@ public function decrypt($encrypted)
try {
$iv = substr($this->key, 0, 16);
$decrypted = openssl_decrypt($encrypted, 'AES-256-CBC', substr($this->key, 0, 32), OPENSSL_ZERO_PADDING, $iv);
- } catch (Exception $e) {
+ } catch (\Exception $e) {
return [ErrorCode::$DecryptAESError, null];
}
try {
@@ -116,7 +75,7 @@ public function decrypt($encrypted)
$len_list = unpack("N", substr($content, 0, 4));
$xml_len = $len_list[1];
return [0, substr($content, 4, $xml_len), substr($content, $xml_len + 4)];
- } catch (Exception $e) {
+ } catch (\Exception $e) {
return [ErrorCode::$IllegalBuffer, null];
}
}
@@ -137,53 +96,3 @@ function getRandomStr($str = "")
}
}
-
-/**
- * 仅用作类内部使用
- * 不用于官方API接口的errCode码
- * Class ErrorCode
- */
-class ErrorCode
-{
-
- public static $OK = 0;
- public static $ParseXmlError = 40002;
- public static $IllegalAesKey = 40004;
- public static $IllegalBuffer = 40008;
- public static $EncryptAESError = 40006;
- public static $DecryptAESError = 40007;
- public static $EncodeBase64Error = 40009;
- public static $DecodeBase64Error = 40010;
- public static $GenReturnXmlError = 40011;
- public static $ValidateAppidError = 40005;
- public static $ComputeSignatureError = 40003;
- public static $ValidateSignatureError = 40001;
- public static $errCode = [
- '0' => '处理成功',
- '40001' => '校验签名失败',
- '40002' => '解析xml失败',
- '40003' => '计算签名失败',
- '40004' => '不合法的AESKey',
- '40005' => '校验AppID失败',
- '40006' => 'AES加密失败',
- '40007' => 'AES解密失败',
- '40008' => '公众平台发送的xml不合法',
- '40009' => 'Base64编码失败',
- '40010' => 'Base64解码失败',
- '40011' => '公众帐号生成回包xml失败',
- ];
-
- /**
- * 获取错误消息内容
- * @param string $code 错误代码
- * @return bool
- */
- public static function getErrText($code)
- {
- if (isset(self::$errCode[$code])) {
- return self::$errCode[$code];
- }
- return false;
- }
-
-}
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Qrcode.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Qrcode.php
index 3b6251101b8..19abd49f4b7 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Qrcode.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Qrcode.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
namespace WeChat;
@@ -29,8 +31,8 @@ class Qrcode extends BasicWeChat
* @param string|integer $scene 场景
* @param int $expire_seconds 有效时间
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function create($scene, $expire_seconds = 0)
{
@@ -46,8 +48,7 @@ public function create($scene, $expire_seconds = 0)
$data['action_name'] = is_integer($scene) ? 'QR_LIMIT_SCENE' : 'QR_LIMIT_STR_SCENE';
}
$url = "https://api.weixin.qq.com/cgi-bin/qrcode/create?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, $data);
+ return $this->callPostApi($url, $data);
}
/**
@@ -57,21 +58,19 @@ public function create($scene, $expire_seconds = 0)
*/
public function url($ticket)
{
- return "https://mp.weixin.qq.com/cgi-bin/showqrcode?ticket={$ticket}";
+ return "https://mp.weixin.qq.com/cgi-bin/showqrcode?ticket=" . urlencode($ticket);
}
/**
* 长链接转短链接接口
* @param string $longUrl 需要转换的长链接
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function shortUrl($longUrl)
{
$url = "https://api.weixin.qq.com/cgi-bin/shorturl?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, ['action' => 'long2short', 'long_url' => $longUrl]);
+ return $this->callPostApi($url, ['action' => 'long2short', 'long_url' => $longUrl]);
}
-
}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Receive.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Receive.php
index 7f4d0700635..edf9920218f 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Receive.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Receive.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
namespace WeChat;
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Scan.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Scan.php
index ad272eb4333..ffd2e8acf04 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Scan.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Scan.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
namespace WeChat;
@@ -26,28 +28,26 @@ class Scan extends BasicWeChat
/**
* 获取商户信息
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function getMerchantInfo()
{
$url = "https://api.weixin.qq.com/scan/merchantinfo/get?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpGetForJson($url);
+ return $this->callGetApi($url);
}
/**
* 创建商品
* @param array $data
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function addProduct(array $data)
{
$url = "https://api.weixin.qq.com/scan/product/create?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, $data);
+ return $this->callPostApi($url, $data);
}
/**
@@ -56,15 +56,14 @@ public function addProduct(array $data)
* @param string $keystr 商品编码内容
* @param string $status 设置发布状态。on为提交审核,off为取消发布
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function modProduct($keystandard, $keystr, $status = 'on')
{
- $data = ['keystandard' => $keystandard, 'keystr' => $keystr, 'status' => $status];
$url = "https://api.weixin.qq.com/scan/product/modstatus?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, $data);
+ $data = ['keystandard' => $keystandard, 'keystr' => $keystr, 'status' => $status];
+ return $this->callPostApi($url, $data);
}
/**
@@ -72,15 +71,13 @@ public function modProduct($keystandard, $keystr, $status = 'on')
* @param array $openids 测试人员的openid列表
* @param array $usernames 测试人员的微信号列表
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function setTestWhiteList($openids = [], $usernames = [])
{
- $data = ['openid' => $openids, 'username' => $usernames];
$url = "https://api.weixin.qq.com/scan/product/modstatus?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, $data);
+ return $this->callPostApi($url, ['openid' => $openids, 'username' => $usernames]);
}
/**
@@ -90,16 +87,15 @@ public function setTestWhiteList($openids = [], $usernames = [])
* @param null|string $extinfo
* @param integer $qrcode_size
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function getQrc($keystandard, $keystr, $extinfo = null, $qrcode_size = 64)
{
+ $url = "https://api.weixin.qq.com/scan/product/getqrcode?access_token=ACCESS_TOKEN";
$data = ['keystandard' => $keystandard, 'keystr' => $keystr, 'qrcode_size' => $qrcode_size];
is_null($extinfo) || $data['extinfo'] = $extinfo;
- $url = "https://api.weixin.qq.com/scan/product/getqrcode?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, $data);
+ return $this->callPostApi($url, $data);
}
/**
@@ -107,14 +103,13 @@ public function getQrc($keystandard, $keystr, $extinfo = null, $qrcode_size = 64
* @param string $keystandard 商品编码标准
* @param string $keystr 商品编码内容
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function getProductInfo($keystandard, $keystr)
{
$url = "https://api.weixin.qq.com/scan/product/get?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, ['keystandard' => $keystandard, 'keystr' => $keystr]);
+ return $this->callPostApi($url, ['keystandard' => $keystandard, 'keystr' => $keystr]);
}
/**
@@ -124,31 +119,29 @@ public function getProductInfo($keystandard, $keystr)
* @param string $status 支持按状态拉取。on为发布状态,off为未发布状态,check为审核中状态,reject为审核未通过状态,all为所有状态。
* @param string $keystr 支持按部分编码内容拉取。填写该参数后,可将编码内容中包含所传参数的商品信息拉出。类似关键词搜索。
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function getProductList($offset = 1, $limit = 10, $status = null, $keystr = null)
{
+ $url = "https://api.weixin.qq.com/scan/product/getlist?access_token=ACCESS_TOKEN";
$data = ['offset' => $offset, 'limit' => $limit];
is_null($status) || $data['status'] = $status;
is_null($keystr) || $data['keystr'] = $keystr;
- $url = "https://api.weixin.qq.com/scan/product/getlist?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, $data);
+ return $this->callPostApi($url, $data);
}
/**
* 更新商品信息
* @param array $data
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function updateProduct(array $data)
{
$url = "https://api.weixin.qq.com/scan/product/update?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, $data);
+ return $this->callPostApi($url, $data);
}
/**
@@ -156,28 +149,26 @@ public function updateProduct(array $data)
* @param string $keystandard 商品编码标准
* @param string $keystr 商品编码内容
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function clearProduct($keystandard, $keystr)
{
$url = "https://api.weixin.qq.com/scan/product/clear?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, ['keystandard' => $keystandard, 'keystr' => $keystr]);
+ return $this->callPostApi($url, ['keystandard' => $keystandard, 'keystr' => $keystr]);
}
/**
* 检查wxticket参数
* @param string $ticket
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function checkTicket($ticket)
{
$url = "https://api.weixin.qq.com/scan/scanticket/check?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, ['ticket' => $ticket]);
+ return $this->callPostApi($url, ['ticket' => $ticket]);
}
/**
@@ -186,14 +177,12 @@ public function checkTicket($ticket)
* @param string $keystr 商品编码内容
* @param string $extinfo 调用“获取商品二维码接口”时传入的extinfo,为标识参数
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function clearScanTicket($keystandard, $keystr, $extinfo)
{
$url = "https://api.weixin.qq.com/scan/scanticket/check?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, ['keystandard' => $keystandard, 'keystr' => $keystr, 'extinfo' => $extinfo]);
+ return $this->callPostApi($url, ['keystandard' => $keystandard, 'keystr' => $keystr, 'extinfo' => $extinfo]);
}
-
}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Script.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Script.php
index 145e51945e1..4bdf4af9c4f 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Script.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Script.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
namespace WeChat;
@@ -44,8 +46,8 @@ public function delTicket($type = 'jsapi', $appid = null)
* @param string $type TICKET类型(wx_card|jsapi)
* @param string $appid 强制指定有效APPID
* @return string
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function getTicket($type = 'jsapi', $appid = null)
{
@@ -60,7 +62,7 @@ public function getTicket($type = 'jsapi', $appid = null)
throw new InvalidResponseException('Invalid Resoponse Ticket.', '0');
}
$ticket = $result['ticket'];
- Tools::setCache($cache_name, $ticket, 5000);
+ Tools::setCache($cache_name, $ticket, 7000);
}
return $ticket;
}
@@ -70,15 +72,23 @@ public function getTicket($type = 'jsapi', $appid = null)
* @param string $url 网页的URL
* @param string $appid 用于多个appid时使用(可空)
* @param string $ticket 强制指定ticket
+ * @param array $jsApiList 需初始化的 jsApiList
* @return array
- * @throws Exceptions\LocalCacheException
- * @throws InvalidResponseException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
- public function getJsSign($url, $appid = null, $ticket = null)
+ public function getJsSign($url, $appid = null, $ticket = null, $jsApiList = null)
{
list($url,) = explode('#', $url);
is_null($ticket) && $ticket = $this->getTicket('jsapi');
is_null($appid) && $appid = $this->config->get('appid');
+ is_null($jsApiList) && $jsApiList = [
+ 'updateAppMessageShareData', 'updateTimelineShareData', 'onMenuShareTimeline', 'onMenuShareAppMessage', 'onMenuShareQQ', 'onMenuShareWeibo', 'onMenuShareQZone',
+ 'startRecord', 'stopRecord', 'onVoiceRecordEnd', 'playVoice', 'pauseVoice', 'stopVoice', 'onVoicePlayEnd', 'uploadVoice', 'downloadVoice',
+ 'chooseImage', 'previewImage', 'uploadImage', 'downloadImage', 'translateVoice', 'getNetworkType', 'openLocation', 'getLocation',
+ 'hideOptionMenu', 'showOptionMenu', 'hideMenuItems', 'showMenuItems', 'hideAllNonBaseMenuItem', 'showAllNonBaseMenuItem',
+ 'closeWindow', 'scanQRCode', 'chooseWXPay', 'openProductSpecificView', 'addCard', 'chooseCard', 'openCard',
+ ];
$data = ["url" => $url, "timestamp" => '' . time(), "jsapi_ticket" => $ticket, "noncestr" => Tools::createNoncestr(16)];
return [
'debug' => false,
@@ -86,13 +96,7 @@ public function getJsSign($url, $appid = null, $ticket = null)
"nonceStr" => $data['noncestr'],
"timestamp" => $data['timestamp'],
"signature" => $this->getSignature($data, 'sha1'),
- 'jsApiList' => [
- 'updateAppMessageShareData', 'updateTimelineShareData', 'onMenuShareTimeline', 'onMenuShareAppMessage', 'onMenuShareQQ', 'onMenuShareWeibo', 'onMenuShareQZone',
- 'startRecord', 'stopRecord', 'onVoiceRecordEnd', 'playVoice', 'pauseVoice', 'stopVoice', 'onVoicePlayEnd', 'uploadVoice', 'downloadVoice',
- 'chooseImage', 'previewImage', 'uploadImage', 'downloadImage', 'translateVoice', 'getNetworkType', 'openLocation', 'getLocation',
- 'hideOptionMenu', 'showOptionMenu', 'hideMenuItems', 'showMenuItems', 'hideAllNonBaseMenuItem', 'showAllNonBaseMenuItem',
- 'closeWindow', 'scanQRCode', 'chooseWXPay', 'openProductSpecificView', 'addCard', 'chooseCard', 'openCard',
- ],
+ 'jsApiList' => $jsApiList,
];
}
@@ -107,7 +111,7 @@ protected function getSignature($data, $method = "sha1", $params = [])
{
ksort($data);
if (!function_exists($method)) return false;
- foreach ($data as $k => $v) array_push($params, "{$k}={$v}");
+ foreach ($data as $k => $v) $params[] = "{$k}={$v}";
return $method(join('&', $params));
}
}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Shake.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Shake.php
index 2d259581cde..5794c3a82ba 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Shake.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Shake.php
@@ -3,20 +3,21 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
namespace WeChat;
-
-use WeChat\Contracts\Tools;
use WeChat\Contracts\BasicWeChat;
+use WeChat\Contracts\Tools;
/**
* 揺一揺周边
@@ -29,27 +30,25 @@ class Shake extends BasicWeChat
* 申请开通功能
* @param array $data
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function register(array $data)
{
$url = "https://api.weixin.qq.com/shakearound/account/register?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, $data);
+ return $this->callPostApi($url, $data);
}
/**
* 查询审核状态
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function auditStatus()
{
$url = "https://api.weixin.qq.com/shakearound/account/auditstatus?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpGetForJson($url);
+ return $this->callGetApi($url);
}
/**
@@ -59,8 +58,8 @@ public function auditStatus()
* @param null|string $comment 备注,不超过15个汉字或30个英文字母
* @param null|string $poi_id 设备关联的门店ID,关联门店后,在门店1KM的范围内有优先摇出信息的机会。
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function createApply($quantity, $apply_reason, $comment = null, $poi_id = null)
{
@@ -68,120 +67,111 @@ public function createApply($quantity, $apply_reason, $comment = null, $poi_id =
is_null($poi_id) || $data['poi_id'] = $poi_id;
is_null($comment) || $data['comment'] = $comment;
$url = "https://api.weixin.qq.com/shakearound/device/applyid?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, $data);
+ return $this->callPostApi($url, $data);
}
/**
* 查询设备ID申请审核状态
* @param integer $applyId 批次ID,申请设备ID时所返回的批次ID
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function getApplyStatus($applyId)
{
$url = "https://api.weixin.qq.com/shakearound/device/applyid?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, ['apply_id' => $applyId]);
+ return $this->callPostApi($url, ['apply_id' => $applyId]);
}
/**
* 编辑设备信息
* @param array $data
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function updateApply(array $data)
{
$url = "https://api.weixin.qq.com/shakearound/device/update?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, $data);
+ return $this->callPostApi($url, $data);
}
/**
* 配置设备与门店的关联关系
* @param array $data
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function bindLocation(array $data)
{
$url = "https://api.weixin.qq.com/shakearound/device/bindlocation?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, $data);
+ return $this->callPostApi($url, $data);
}
/**
* 查询设备列表
* @param array $data
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function search(array $data)
{
$url = "https://api.weixin.qq.com/shakearound/device/search?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, $data);
+ return $this->callPostApi($url, $data);
}
/**
* 页面管理
* @param array $data
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function createPage(array $data)
{
$url = "https://api.weixin.qq.com/shakearound/page/add?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, $data);
+ return $this->callPostApi($url, $data);
}
/**
* 编辑页面信息
* @param array $data
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function updatePage(array $data)
{
$url = "https://api.weixin.qq.com/shakearound/page/update?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, $data);
+ return $this->callPostApi($url, $data);
}
/**
* 查询页面列表
* @param array $data
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function searchPage(array $data)
{
$url = "https://api.weixin.qq.com/shakearound/page/search?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, $data);
+ return $this->callPostApi($url, $data);
}
/**
* 删除页面
- * @param integer page_id 指定页面的id
+ * @param integer $pageId 指定页面的id
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
- public function deletePage($page_id)
+ public function deletePage($pageId)
{
$url = "https://api.weixin.qq.com/shakearound/page/delete?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, ['page_id' => $page_id]);
+ return $this->callPostApi($url, ['page_id' => $pageId]);
}
/**
@@ -189,116 +179,108 @@ public function deletePage($page_id)
* @param string $filename 图片名字
* @param string $type Icon:摇一摇页面展示的icon图;License:申请开通摇一摇周边功能时需上传的资质文件;若不传type,则默认type=icon
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function upload($filename, $type = 'icon')
{
$url = "https://api.weixin.qq.com/shakearound/material/add?access_token=ACCESS_TOKEN&type={$type}";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, ['media' => Tools::createCurlFile($filename)]);
+ return $this->callPostApi($url, ['media' => Tools::createCurlFile($filename)], false);
}
/**
* 配置设备与页面的关联关系
* @param array $data
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function bindPage(array $data)
{
$url = "https://api.weixin.qq.com/shakearound/device/bindpage?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, $data);
+ return $this->callPostApi($url, $data);
}
/**
* 查询设备与页面的关联关系
* @param array $data
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function queryPage(array $data)
{
$url = "https://api.weixin.qq.com/shakearound/relation/search?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, $data);
+ return $this->callPostApi($url, $data);
}
/**
* 以设备为维度的数据统计接口
* @param array $data
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function totalDevice(array $data)
{
$url = "https://api.weixin.qq.com/shakearound/statistics/device?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, $data);
+ return $this->callPostApi($url, $data);
}
/**
* 批量查询设备统计数据接口
* @param integer $date 指定查询日期时间戳,单位为秒
- * @param integer $page_index 指定查询的结果页序号;返回结果按摇周边人数降序排序,每50条记录为一页
+ * @param integer $pageIndex 指定查询的结果页序号;返回结果按摇周边人数降序排序,每50条记录为一页
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
- public function totalDeviceList($date, $page_index = 1)
+ public function totalDeviceList($date, $pageIndex = 1)
{
$url = "https://api.weixin.qq.com/shakearound/statistics/devicelist?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, ['date' => $date, 'page_index' => $page_index]);
+ return $this->callPostApi($url, ['date' => $date, 'page_index' => $pageIndex]);
}
/**
* 以页面为维度的数据统计接口
- * @param integer $page_id 指定页面的设备ID
- * @param integer $begin_date 起始日期时间戳,最长时间跨度为30天,单位为秒
- * @param integer $end_date 结束日期时间戳,最长时间跨度为30天,单位为秒
+ * @param integer $pageId 指定页面的设备ID
+ * @param integer $beginDate 起始日期时间戳,最长时间跨度为30天,单位为秒
+ * @param integer $endDate 结束日期时间戳,最长时间跨度为30天,单位为秒
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
- public function totalPage($page_id, $begin_date, $end_date)
+ public function totalPage($pageId, $beginDate, $endDate)
{
$url = "https://api.weixin.qq.com/shakearound/statistics/page?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, ['page_id' => $page_id, 'begin_date' => $begin_date, 'end_date' => $end_date]);
+ return $this->callPostApi($url, ['page_id' => $pageId, 'begin_date' => $beginDate, 'end_date' => $endDate]);
}
/**
* 编辑分组信息
- * @param integer $group_id 分组唯一标识,全局唯一
- * @param string $group_name 分组名称,不超过100汉字或200个英文字母
+ * @param integer $groupId 分组唯一标识,全局唯一
+ * @param string $groupName 分组名称,不超过100汉字或200个英文字母
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
- public function updateGroup($group_id, $group_name)
+ public function updateGroup($groupId, $groupName)
{
$url = "https://api.weixin.qq.com/shakearound/device/group/update?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, ['group_id' => $group_id, 'group_name' => $group_name]);
+ return $this->callPostApi($url, ['group_id' => $groupId, 'group_name' => $groupName]);
}
/**
* 删除分组
- * @param integer $group_id 分组唯一标识,全局唯一
+ * @param integer $groupId 分组唯一标识,全局唯一
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
- public function deleteGroup($group_id)
+ public function deleteGroup($groupId)
{
$url = "https://api.weixin.qq.com/shakearound/device/group/delete?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, ['group_id' => $group_id]);
+ return $this->callPostApi($url, ['group_id' => $groupId]);
}
/**
@@ -306,14 +288,13 @@ public function deleteGroup($group_id)
* @param integer $begin 分组列表的起始索引值
* @param integer $count 待查询的分组数量,不能超过1000个
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function getGroupList($begin = 0, $count = 10)
{
$url = "https://api.weixin.qq.com/shakearound/device/group/getlist?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, ['begin' => $begin, 'count' => $count]);
+ return $this->callPostApi($url, ['begin' => $begin, 'count' => $count]);
}
@@ -323,42 +304,38 @@ public function getGroupList($begin = 0, $count = 10)
* @param integer $begin 分组里设备的起始索引值
* @param integer $count 待查询的分组里设备的数量,不能超过1000个
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function getGroupDetail($group_id, $begin = 0, $count = 100)
{
$url = "https://api.weixin.qq.com/shakearound/device/group/getdetail?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, ['group_id' => $group_id, 'begin' => $begin, 'count' => $count]);
+ return $this->callPostApi($url, ['group_id' => $group_id, 'begin' => $begin, 'count' => $count]);
}
/**
* 添加设备到分组
* @param array $data
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function addDeviceGroup(array $data)
{
$url = "https://api.weixin.qq.com/shakearound/device/group/adddevice?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, $data);
+ return $this->callPostApi($url, $data);
}
/**
* 从分组中移除设备
* @param array $data
* @return array
- * @throws Exceptions\InvalidResponseException
- * @throws Exceptions\LocalCacheException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
*/
public function deleteDeviceGroup(array $data)
{
$url = "https://api.weixin.qq.com/shakearound/device/group/deletedevice?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, $data);
+ return $this->callPostApi($url, $data);
}
-
}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Tags.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Tags.php
index a13c79f79f6..d0e9fef72b6 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Tags.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Tags.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
namespace WeChat;
@@ -31,8 +33,7 @@ class Tags extends BasicWeChat
public function getTags()
{
$url = "https://api.weixin.qq.com/cgi-bin/tags/get?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpGetForJson($url);
+ return $this->callGetApi($url);
}
/**
@@ -45,8 +46,7 @@ public function getTags()
public function createTags($name)
{
$url = "https://api.weixin.qq.com/cgi-bin/tags/create?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, ['tag' => ['name' => $name]]);
+ return $this->callPostApi($url, ['tag' => ['name' => $name]]);
}
/**
@@ -60,8 +60,7 @@ public function createTags($name)
public function updateTags($id, $name)
{
$url = "https://api.weixin.qq.com/cgi-bin/tags/update?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, ['tag' => ['name' => $name, 'id' => $id]]);
+ return $this->callPostApi($url, ['tag' => ['name' => $name, 'id' => $id]]);
}
/**
@@ -74,8 +73,7 @@ public function updateTags($id, $name)
public function deleteTags($tagId)
{
$url = 'https://api.weixin.qq.com/cgi-bin/tags/delete?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, ['tag' => ['id' => $tagId]]);
+ return $this->callPostApi($url, ['tag' => ['id' => $tagId]]);
}
/**
@@ -89,8 +87,7 @@ public function deleteTags($tagId)
public function batchTagging(array $openids, $tagId)
{
$url = 'https://api.weixin.qq.com/cgi-bin/tags/members/batchtagging?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, ['openid_list' => $openids, 'tagid' => $tagId]);
+ return $this->callPostApi($url, ['openid_list' => $openids, 'tagid' => $tagId]);
}
/**
@@ -104,8 +101,7 @@ public function batchTagging(array $openids, $tagId)
public function batchUntagging(array $openids, $tagId)
{
$url = 'https://api.weixin.qq.com/cgi-bin/tags/members/batchuntagging?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, ['openid_list' => $openids, 'tagid' => $tagId]);
+ return $this->callPostApi($url, ['openid_list' => $openids, 'tagid' => $tagId]);
}
/**
@@ -118,7 +114,6 @@ public function batchUntagging(array $openids, $tagId)
public function getUserTagId($openid)
{
$url = 'https://api.weixin.qq.com/cgi-bin/tags/getidlist?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, ['openid' => $openid]);
+ return $this->callPostApi($url, ['openid' => $openid]);
}
}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Template.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Template.php
index eb50ab4a23c..0410d2922a5 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Template.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Template.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
namespace WeChat;
@@ -25,17 +27,16 @@ class Template extends BasicWeChat
{
/**
* 设置所属行业
- * @param string $industry_id1 公众号模板消息所属行业编号
- * @param string $industry_id2 公众号模板消息所属行业编号
+ * @param string $industryId1 公众号模板消息所属行业编号
+ * @param string $industryId2 公众号模板消息所属行业编号
* @return array
* @throws Exceptions\InvalidResponseException
* @throws Exceptions\LocalCacheException
*/
- public function setIndustry($industry_id1, $industry_id2)
+ public function setIndustry($industryId1, $industryId2)
{
$url = "https://api.weixin.qq.com/cgi-bin/template/api_set_industry?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, ['industry_id1' => $industry_id1, 'industry_id2' => $industry_id2]);
+ return $this->callPostApi($url, ['industry_id1' => $industryId1, 'industry_id2' => $industryId2]);
}
/**
@@ -47,22 +48,21 @@ public function setIndustry($industry_id1, $industry_id2)
public function getIndustry()
{
$url = "https://api.weixin.qq.com/cgi-bin/template/get_industry?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpGetForJson($url);
+ return $this->callGetApi($url);
}
/**
* 获得模板ID
- * @param string $tpl_id 板库中模板的编号,有“TM**”和“OPENTMTM**”等形式
+ * @param string $templateIdShort 板库中模板的编号,有“TM**”和“OPENTMTM**”等形式
+ * @param array $keywordNameList 选用的类目模板的关键词
* @return array
* @throws Exceptions\InvalidResponseException
* @throws Exceptions\LocalCacheException
*/
- public function addTemplate($tpl_id)
+ public function addTemplate($templateIdShort, $keywordNameList = [])
{
$url = "https://api.weixin.qq.com/cgi-bin/template/api_add_template?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, ['template_id_short' => $tpl_id]);
+ return $this->callPostApi($url, ['template_id_short' => $templateIdShort, 'keyword_name_list' => $keywordNameList]);
}
/**
@@ -74,22 +74,20 @@ public function addTemplate($tpl_id)
public function getAllPrivateTemplate()
{
$url = "https://api.weixin.qq.com/cgi-bin/template/get_all_private_template?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpGetForJson($url);
+ return $this->callGetApi($url);
}
/**
* 删除模板ID
- * @param string $tpl_id 公众帐号下模板消息ID
+ * @param string $tplId 公众帐号下模板消息ID
* @return array
* @throws Exceptions\InvalidResponseException
* @throws Exceptions\LocalCacheException
*/
- public function delPrivateTemplate($tpl_id)
+ public function delPrivateTemplate($tplId)
{
$url = "https://api.weixin.qq.com/cgi-bin/template/del_private_template?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, ['template_id' => $tpl_id]);
+ return $this->callPostApi($url, ['template_id' => $tplId]);
}
/**
@@ -102,9 +100,6 @@ public function delPrivateTemplate($tpl_id)
public function send(array $data)
{
$url = "https://api.weixin.qq.com/cgi-bin/message/template/send?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, $data);
+ return $this->callPostApi($url, $data);
}
-
-
}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/User.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/User.php
index 2156cf7b28b..a69294b2d32 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/User.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/User.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
namespace WeChat;
@@ -35,8 +37,7 @@ class User extends BasicWeChat
public function updateMark($openid, $remark)
{
$url = 'https://api.weixin.qq.com/cgi-bin/user/info/updateremark?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, ['openid' => $openid, 'remark' => $remark]);
+ return $this->callPostApi($url, ['openid' => $openid, 'remark' => $remark]);
}
/**
@@ -50,8 +51,7 @@ public function updateMark($openid, $remark)
public function getUserInfo($openid, $lang = 'zh_CN')
{
$url = "https://api.weixin.qq.com/cgi-bin/user/info?access_token=ACCESS_TOKEN&openid={$openid}&lang={$lang}";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpGetForJson($url);
+ return $this->callGetApi($url);
}
/**
@@ -69,8 +69,7 @@ public function getBatchUserInfo(array $openids, $lang = 'zh_CN')
foreach ($openids as $openid) {
$data['user_list'][] = ['openid' => $openid, 'lang' => $lang];
}
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, $data);
+ return $this->callPostApi($url, $data);
}
/**
@@ -83,37 +82,34 @@ public function getBatchUserInfo(array $openids, $lang = 'zh_CN')
public function getUserList($next_openid = '')
{
$url = "https://api.weixin.qq.com/cgi-bin/user/get?access_token=ACCESS_TOKEN&next_openid={$next_openid}";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpGetForJson($url);
+ return $this->callGetApi($url);
}
/**
* 获取标签下粉丝列表
* @param integer $tagid 标签ID
- * @param string $next_openid 第一个拉取的OPENID
+ * @param string $nextOpenid 第一个拉取的OPENID
* @return array
* @throws Exceptions\InvalidResponseException
* @throws Exceptions\LocalCacheException
*/
- public function getUserListByTag($tagid, $next_openid = '')
+ public function getUserListByTag($tagid, $nextOpenid = '')
{
$url = 'https://api.weixin.qq.com/cgi-bin/user/tag/get?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, ['tagid' => $tagid, 'next_openid' => $next_openid]);
+ return $this->callPostApi($url, ['tagid' => $tagid, 'next_openid' => $nextOpenid]);
}
/**
* 获取公众号的黑名单列表
- * @param string $begin_openid
+ * @param string $beginOpenid
* @return array
* @throws Exceptions\InvalidResponseException
* @throws Exceptions\LocalCacheException
*/
- public function getBlackList($begin_openid = '')
+ public function getBlackList($beginOpenid = '')
{
$url = "https://api.weixin.qq.com/cgi-bin/tags/members/getblacklist?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, ['begin_openid' => $begin_openid]);
+ return $this->callPostApi($url, ['begin_openid' => $beginOpenid]);
}
/**
@@ -126,8 +122,7 @@ public function getBlackList($begin_openid = '')
public function batchBlackList(array $openids)
{
$url = "https://api.weixin.qq.com/cgi-bin/tags/members/batchblacklist?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, ['openid_list' => $openids]);
+ return $this->callPostApi($url, ['openid_list' => $openids]);
}
/**
@@ -140,8 +135,6 @@ public function batchBlackList(array $openids)
public function batchUnblackList(array $openids)
{
$url = "https://api.weixin.qq.com/cgi-bin/tags/members/batchunblacklist?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->httpPostForJson($url, ['openid_list' => $openids]);
+ return $this->callPostApi($url, ['openid_list' => $openids]);
}
-
}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Wifi.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Wifi.php
index ff22afffc84..c877532bd44 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Wifi.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeChat/Wifi.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
namespace WeChat;
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Crypt.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Crypt.php
index 38fe360d2b0..24f5e7542e3 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Crypt.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Crypt.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
namespace WeMini;
@@ -18,6 +20,7 @@
use WeChat\Contracts\Tools;
use WeChat\Exceptions\InvalidDecryptException;
use WeChat\Exceptions\InvalidResponseException;
+use WXBizDataCrypt;
/**
@@ -33,12 +36,12 @@ class Crypt extends BasicWeChat
* @param string $iv
* @param string $sessionKey
* @param string $encryptedData
- * @return bool
+ * @return bool|array
*/
public function decode($iv, $sessionKey, $encryptedData)
{
require_once __DIR__ . DIRECTORY_SEPARATOR . 'crypt' . DIRECTORY_SEPARATOR . 'wxBizDataCrypt.php';
- $pc = new \WXBizDataCrypt($this->config->get('appid'), $sessionKey);
+ $pc = new WXBizDataCrypt($this->config->get('appid'), $sessionKey);
$errCode = $pc->decryptData($encryptedData, $iv, $data);
if ($errCode == 0) {
return json_decode($data, true);
@@ -66,8 +69,8 @@ public function session($code)
* @param string $iv 加密算法的初始向量
* @param string $encryptedData 加密数据( encryptedData )
* @return array
- * @throws InvalidDecryptException
- * @throws InvalidResponseException
+ * @throws \WeChat\Exceptions\InvalidDecryptException
+ * @throws \WeChat\Exceptions\InvalidResponseException
* @throws \WeChat\Exceptions\LocalCacheException
*/
public function userInfo($code, $iv, $encryptedData)
@@ -83,6 +86,20 @@ public function userInfo($code, $iv, $encryptedData)
return array_merge($result, $userinfo);
}
+ /**
+ * 通过授权码换取手机号
+ * @param string $code
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function getPhoneNumber($code)
+ {
+ $url = 'https://api.weixin.qq.com/wxa/business/getuserphonenumber?access_token=ACCESS_TOKEN';
+ $this->registerApi($url, __FUNCTION__, func_get_args());
+ return $this->httpPostForJson($url, ['code' => $code], true);
+ }
+
/**
* 用户支付完成后,获取该用户的 UnionId
* @param string $openid 支付用户唯一标识
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Delivery.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Delivery.php
index 6d6d6aff5cc..21fa777c612 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Delivery.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Delivery.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
namespace WeMini;
@@ -34,7 +36,6 @@ class Delivery extends BasicWeChat
public function abnormalConfirm($data)
{
$url = 'https://api.weixin.qq.com/cgi-bin/express/local/business/order/confirm_return?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, $data, true);
}
@@ -48,7 +49,6 @@ public function abnormalConfirm($data)
public function addOrder($data)
{
$url = 'https://api.weixin.qq.com/cgi-bin/express/local/business/order/add?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, $data, true);
}
@@ -62,7 +62,6 @@ public function addOrder($data)
public function addTip($data)
{
$url = 'https://api.weixin.qq.com/cgi-bin/express/local/business/order/addtips?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, $data, true);
}
@@ -76,7 +75,6 @@ public function addTip($data)
public function cancelOrder($data)
{
$url = 'https://api.weixin.qq.com/cgi-bin/express/local/business/order/cancel?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, $data, true);
}
@@ -90,7 +88,6 @@ public function cancelOrder($data)
public function getAllImmeDelivery($data)
{
$url = 'https://api.weixin.qq.com/cgi-bin/express/local/business/delivery/getall?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, $data, true);
}
@@ -104,7 +101,6 @@ public function getAllImmeDelivery($data)
public function getBindAccount($data)
{
$url = 'https://api.weixin.qq.com/cgi-bin/express/local/business/shop/get?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, $data, true);
}
@@ -118,7 +114,6 @@ public function getBindAccount($data)
public function getOrder($data)
{
$url = 'https://api.weixin.qq.com/cgi-bin/express/local/business/order/get?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, $data, true);
}
@@ -132,7 +127,6 @@ public function getOrder($data)
public function mockUpdateOrder($data)
{
$url = 'https://api.weixin.qq.com/cgi-bin/express/local/business/test_update_order?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, $data, true);
}
@@ -146,7 +140,6 @@ public function mockUpdateOrder($data)
public function preAddOrder($data)
{
$url = 'https://api.weixin.qq.com/cgi-bin/express/local/business/order/pre_add?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, $data, true);
}
@@ -160,7 +153,6 @@ public function preAddOrder($data)
public function preCancelOrder($data)
{
$url = 'https://api.weixin.qq.com/cgi-bin/express/local/business/order/precancel?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, $data, true);
}
@@ -174,7 +166,6 @@ public function preCancelOrder($data)
public function reOrder($data)
{
$url = 'https://api.weixin.qq.com/cgi-bin/express/local/business/order/readd?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, $data, true);
}
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Guide.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Guide.php
new file mode 100644
index 00000000000..c63327d5eb7
--- /dev/null
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Guide.php
@@ -0,0 +1,527 @@
+callPostApi($url, $data, true);
+ }
+
+ /**
+ * 服务号删除导购
+ * @param array $data
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function delGuideAcct($data)
+ {
+ $url = 'https://api.weixin.qq.com/cgi-bin/guide/delguideacct?access_token=ACCESS_TOKEN';
+ return $this->callPostApi($url, $data, true);
+ }
+
+ /**
+ * 服务号获取导购信息
+ * @param array $data
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function getGuideAcct($data)
+ {
+ $url = 'https://api.weixin.qq.com/cgi-bin/guide/getguideacct?access_token=ACCESS_TOKEN';
+ return $this->callPostApi($url, $data, true);
+ }
+
+ /**
+ * 获取服务号的敏感词信息与自动回复信息
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function getGuideAcctConfig()
+ {
+ $url = 'https://api.weixin.qq.com/cgi-bin/guide/getguideacctconfig?access_token=ACCESS_TOKEN';
+ return $this->callPostApi($url, [], true);
+ }
+
+ /**
+ * 服务号拉取导购列表
+ * @param integer $page
+ * @param integer $num
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function getGuideAcctList($page = 0, $num = 10)
+ {
+ $url = 'https://api.weixin.qq.com/cgi-bin/guide/getguideacctconfig?access_token=ACCESS_TOKEN';
+ return $this->callPostApi($url, ['page' => $page, 'num' => $num], true);
+ }
+
+ /**
+ * 获取导购聊天记录
+ * @param array $data
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function getGuideBuyerChatRecord($data)
+ {
+ $url = 'https://api.weixin.qq.com/cgi-bin/guide/getguideacct?access_token=ACCESS_TOKEN';
+ return $this->callPostApi($url, $data, true);
+ }
+
+ /**
+ * 获取导购快捷回复信息
+ * @param array $data
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function getGuideConfig($data)
+ {
+ $url = 'https://api.weixin.qq.com/cgi-bin/guide/getguideconfig?access_token=ACCESS_TOKEN';
+ return $this->callPostApi($url, $data, true);
+ }
+
+ /**
+ * 生成导购二维码
+ * @param array $data
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function guideCreateQrCode($data)
+ {
+ $url = 'https://api.weixin.qq.com/cgi-bin/guide/guidecreateqrcode?access_token=ACCESS_TOKEN';
+ return $this->callPostApi($url, $data, true);
+ }
+
+ /**
+ * @param array $data
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function pushShowWxaPathMenu($data)
+ {
+ $url = 'https://api.weixin.qq.com/cgi-bin/guide/pushshowwxapathmenu?access_token=ACCESS_TOKEN';
+ return $this->callPostApi($url, $data, true);
+ }
+
+ /**
+ * 为服务号设置敏感词与自动回复
+ * @param array $data
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function setGuideAcctConfig($data)
+ {
+ $url = 'https://api.weixin.qq.com/cgi-bin/guide/setguideacctconfig?access_token=ACCESS_TOKEN';
+ return $this->callPostApi($url, $data, true);
+ }
+
+ /**
+ * 设置导购快捷回复信息
+ * @param array $data
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function setGuideConfig($data)
+ {
+ $url = 'https://api.weixin.qq.com/cgi-bin/guide/setguideconfig?access_token=ACCESS_TOKEN';
+ return $this->callPostApi($url, $data, true);
+ }
+
+ /**
+ * 更新导购昵称或者头像
+ * @param array $data
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function updateGuideAcct($data)
+ {
+ $url = 'https://api.weixin.qq.com/cgi-bin/guide/setguideconfig?access_token=ACCESS_TOKEN';
+ return $this->callPostApi($url, $data, true);
+ }
+
+ /**
+ * 添加展示标签信息
+ * @param array $data
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function addGuideBuyerDisplayTag($data)
+ {
+ $url = 'https://api.weixin.qq.com/cgi-bin/guide/addguidebuyerdisplaytag?access_token=ACCESS_TOKEN';
+ return $this->callPostApi($url, $data, true);
+ }
+
+ /**
+ * 为粉丝添加可查询标签
+ * @param array $data
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function addGuideBuyerTag($data)
+ {
+ $url = 'https://api.weixin.qq.com/cgi-bin/guide/addguidebuyertag?access_token=ACCESS_TOKEN';
+ return $this->callPostApi($url, $data, true);
+ }
+
+ /**
+ * 添加标签可选值
+ * @param array $data
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function addGuideTagOption($data)
+ {
+ $url = 'https://api.weixin.qq.com/cgi-bin/guide/addguidetagoption?access_token=ACCESS_TOKEN';
+ return $this->callPostApi($url, $data, true);
+ }
+
+ /**
+ * 删除粉丝标签
+ * @param array $data
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function delGuideBuyerTag($data)
+ {
+ $url = 'https://api.weixin.qq.com/cgi-bin/guide/delguidebuyertag?access_token=ACCESS_TOKEN';
+ return $this->callPostApi($url, $data, true);
+ }
+
+ /**
+ * 查询展示标签信息
+ * @param array $data
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function getGuideBuyerDisplayTag($data)
+ {
+ $url = 'https://api.weixin.qq.com/cgi-bin/guide/getguidebuyerdisplaytag?access_token=ACCESS_TOKEN';
+ return $this->callPostApi($url, $data, true);
+ }
+
+ /**
+ * 查询粉丝标签
+ * @param array $data
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function getGuideBuyerTag($data)
+ {
+ $url = 'https://api.weixin.qq.com/cgi-bin/guide/getguidebuyertag?access_token=ACCESS_TOKEN';
+ return $this->callPostApi($url, $data, true);
+ }
+
+ /**
+ * 查询标签可选值信息
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function getGuideTagOption()
+ {
+ $url = 'https://api.weixin.qq.com/cgi-bin/guide/getguidetagoption?access_token=ACCESS_TOKEN';
+ return $this->callPostApi($url, [], true);
+ }
+
+ /**
+ * 新建可查询标签类型,支持新建4类可查询标签
+ * @param array $data
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function newGuideTagOption($data)
+ {
+ $url = 'https://api.weixin.qq.com/cgi-bin/guide/newguidetagoption?access_token=ACCESS_TOKEN';
+ return $this->callPostApi($url, $data, true);
+ }
+
+ /**
+ * 根据标签值筛选粉丝
+ * @param array $data
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function queryGuideBuyerByTag($data)
+ {
+ $url = 'https://api.weixin.qq.com/cgi-bin/guide/queryguidebuyerbytag?access_token=ACCESS_TOKEN';
+ $this->registerApi($url, __FUNCTION__, func_get_args());
+ return $this->callPostApi($url, $data, true);
+ }
+
+ /**
+ * 为服务号导购添加粉丝
+ * @param array $data
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function addGuideBuyerRelation($data)
+ {
+ $url = 'https://api.weixin.qq.com/cgi-bin/guide/addguidebuyerrelation?access_token=ACCESS_TOKEN';
+ $this->registerApi($url, __FUNCTION__, func_get_args());
+ return $this->callPostApi($url, $data, true);
+ }
+
+ /**
+ * 删除导购的粉丝
+ * @param array $data
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function delGuideBuyerRelation($data)
+ {
+ $url = 'https://api.weixin.qq.com/cgi-bin/guide/delguidebuyerrelation?access_token=ACCESS_TOKEN';
+ $this->registerApi($url, __FUNCTION__, func_get_args());
+ return $this->callPostApi($url, $data, true);
+ }
+
+ /**
+ * 查询某一个粉丝与导购的绑定关系
+ * @param array $data
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function getGuideBuyerRelation($data)
+ {
+ $url = 'https://api.weixin.qq.com/cgi-bin/guide/getguidebuyerrelation?access_token=ACCESS_TOKEN';
+ $this->registerApi($url, __FUNCTION__, func_get_args());
+ return $this->callPostApi($url, $data, true);
+ }
+
+ /**
+ * 通过粉丝信息查询该粉丝与导购的绑定关系
+ * @param string $openid
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function getGuideBuyerRelationByBuyer($openid)
+ {
+ $url = 'https://api.weixin.qq.com/cgi-bin/guide/getguidebuyerrelation?access_token=ACCESS_TOKEN';
+ $this->registerApi($url, __FUNCTION__, func_get_args());
+ return $this->callPostApi($url, ['openid' => $openid], true);
+ }
+
+ /**
+ * 拉取导购的粉丝列表
+ * @param array $data
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function getGuideBuyerRelationList($data)
+ {
+ $url = 'https://api.weixin.qq.com/cgi-bin/guide/getguidebuyerrelationlist?access_token=ACCESS_TOKEN';
+ $this->registerApi($url, __FUNCTION__, func_get_args());
+ return $this->callPostApi($url, $data, true);
+ }
+
+ /**
+ * 将粉丝从一个导购迁移到另外一个导购下
+ * @param array $data
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function rebindGuideAcctForBuyer($data)
+ {
+ $url = 'https://api.weixin.qq.com/cgi-bin/guide/rebindguideacctforbuyer?access_token=ACCESS_TOKEN';
+ $this->registerApi($url, __FUNCTION__, func_get_args());
+ return $this->callPostApi($url, $data, true);
+ }
+
+ /**
+ * 更新粉丝昵称
+ * @param array $data
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function updateGuideBuyerRelation($data)
+ {
+ $url = 'https://api.weixin.qq.com/cgi-bin/guide/updateguidebuyerrelation?access_token=ACCESS_TOKEN';
+ $this->registerApi($url, __FUNCTION__, func_get_args());
+ return $this->callPostApi($url, $data, true);
+ }
+
+ /**
+ * 删除小程序卡片素材
+ * @param array $data
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function delGuideCardMaterial($data)
+ {
+ $url = 'https://api.weixin.qq.com/cgi-bin/guide/delguidecardmaterial?access_token=ACCESS_TOKEN';
+ $this->registerApi($url, __FUNCTION__, func_get_args());
+ return $this->callPostApi($url, $data, true);
+ }
+
+ /**
+ * 删除图片素材
+ * @param array $data
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function delGuideImageMaterial($data)
+ {
+ $url = 'https://api.weixin.qq.com/cgi-bin/guide/delguideimagematerial?access_token=ACCESS_TOKEN';
+ $this->registerApi($url, __FUNCTION__, func_get_args());
+ return $this->callPostApi($url, $data, true);
+ }
+
+ /**
+ * 删除文字素材
+ * @param array $data
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function delGuideWordMaterial($data)
+ {
+ $url = 'https://api.weixin.qq.com/cgi-bin/guide/delguidewordmaterial?access_token=ACCESS_TOKEN';
+ $this->registerApi($url, __FUNCTION__, func_get_args());
+ return $this->callPostApi($url, $data, true);
+ }
+
+ /**
+ * 获取小程序卡片素材信息
+ * @param integer $type
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function getGuideCardMaterial($type = 0)
+ {
+ $url = 'https://api.weixin.qq.com/cgi-bin/guide/getguidecardmaterial?access_token=ACCESS_TOKEN';
+ $this->registerApi($url, __FUNCTION__, func_get_args());
+ return $this->callPostApi($url, ['type' => $type], true);
+ }
+
+ /**
+ * 获取图片素材信息
+ * @param integer $type 操作类型
+ * @param integer $start 分页查询,起始位置
+ * @param integer $num 分页查询,查询个数
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function getGuideImageMaterial($type = 0, $start = 0, $num = 10)
+ {
+ $url = 'https://api.weixin.qq.com/cgi-bin/guide/getguideimagematerial?access_token=ACCESS_TOKEN';
+ $this->registerApi($url, __FUNCTION__, func_get_args());
+ return $this->callPostApi($url, ['type' => $type, 'start' => $start, 'num' => $num], true);
+ }
+
+ /**
+ * 获取文字素材信息
+ * @param integer $type 操作类型
+ * @param integer $start 分页查询,起始位置
+ * @param integer $num 分页查询,查询个数
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function getGuideWordMaterial($type = 0, $start = 0, $num = 10)
+ {
+ $url = 'https://api.weixin.qq.com/cgi-bin/guide/getguidewordmaterial?access_token=ACCESS_TOKEN';
+ $this->registerApi($url, __FUNCTION__, func_get_args());
+ return $this->callPostApi($url, ['type' => $type, 'start' => $start, 'num' => $num], true);
+ }
+
+ /**
+ * 添加小程序卡片素材
+ * @param array $data
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function setGuideCardMaterial($data)
+ {
+ $url = 'https://api.weixin.qq.com/cgi-bin/guide/setguidecardmaterial?access_token=ACCESS_TOKEN';
+ $this->registerApi($url, __FUNCTION__, func_get_args());
+ return $this->callPostApi($url, $data, true);
+ }
+
+ /**
+ * 添加图片素材
+ * @param array $data
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function setGuideImageMaterial($data)
+ {
+ $url = 'https://api.weixin.qq.com/cgi-bin/guide/setguideimagematerial?access_token=ACCESS_TOKEN';
+ $this->registerApi($url, __FUNCTION__, func_get_args());
+ return $this->callPostApi($url, $data, true);
+ }
+
+ /**
+ * 为服务号添加文字素材
+ * @param array $data
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function setGuideWordMaterial($data)
+ {
+ $url = 'https://api.weixin.qq.com/cgi-bin/guide/setguidewordmaterial?access_token=ACCESS_TOKEN';
+ $this->registerApi($url, __FUNCTION__, func_get_args());
+ return $this->callPostApi($url, $data, true);
+ }
+}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Image.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Image.php
index 16db57544f6..d2185ce3d76 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Image.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Image.php
@@ -3,19 +3,20 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
namespace WeMini;
use WeChat\Contracts\BasicWeChat;
-use WeChat\Exceptions\InvalidResponseException;
/**
* 小程序图像处理
@@ -30,13 +31,12 @@ class Image extends BasicWeChat
* @param string $img_url 要检测的图片 url,传这个则不用传 img 参数。
* @param string $img form-data 中媒体文件标识,有filename、filelength、content-type等信息,传这个则不用穿 img_url
* @return array
- * @throws InvalidResponseException
+ * @throws \WeChat\Exceptions\InvalidResponseException
* @throws \WeChat\Exceptions\LocalCacheException
*/
public function aiCrop($img_url, $img)
{
- $url = "https://api.weixin.qq.com/cv/img/aicrop?access_token=ACCESS_TOCKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
+ $url = "https://api.weixin.qq.com/cv/img/aicrop?access_token=ACCESS_TOKEN";
return $this->callPostApi($url, ['img_url' => $img_url, 'img' => $img], true);
}
@@ -45,13 +45,12 @@ public function aiCrop($img_url, $img)
* @param string $img_url 要检测的图片 url,传这个则不用传 img 参数。
* @param string $img form-data 中媒体文件标识,有filename、filelength、content-type等信息,传这个则不用穿 img_url
* @return array
- * @throws InvalidResponseException
+ * @throws \WeChat\Exceptions\InvalidResponseException
* @throws \WeChat\Exceptions\LocalCacheException
*/
public function scanQRCode($img_url, $img)
{
- $url = "https://api.weixin.qq.com/cv/img/qrcode?img_url=ENCODE_URL&access_token=ACCESS_TOCKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
+ $url = "https://api.weixin.qq.com/cv/img/qrcode?img_url=ENCODE_URL&access_token=ACCESS_TOKEN";
return $this->callPostApi($url, ['img_url' => $img_url, 'img' => $img], true);
}
@@ -60,13 +59,12 @@ public function scanQRCode($img_url, $img)
* @param string $img_url 要检测的图片 url,传这个则不用传 img 参数
* @param string $img form-data 中媒体文件标识,有filename、filelength、content-type等信息,传这个则不用穿 img_url
* @return array
- * @throws InvalidResponseException
+ * @throws \WeChat\Exceptions\InvalidResponseException
* @throws \WeChat\Exceptions\LocalCacheException
*/
public function superresolution($img_url, $img)
{
- $url = "https://api.weixin.qq.com/cv/img/qrcode?img_url=ENCODE_URL&access_token=ACCESS_TOCKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
+ $url = "https://api.weixin.qq.com/cv/img/qrcode?img_url=ENCODE_URL&access_token=ACCESS_TOKEN";
return $this->callPostApi($url, ['img_url' => $img_url, 'img' => $img], true);
}
}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Live.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Live.php
new file mode 100644
index 00000000000..089fa25f5ba
--- /dev/null
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Live.php
@@ -0,0 +1,171 @@
+callPostApi($url, $data, true);
+ }
+
+ /**
+ * 获取直播房间列表
+ * @param integer $start 起始拉取房间
+ * @param integer $limit 每次拉取的个数上限
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function getLiveList($start = 0, $limit = 10)
+ {
+ $url = 'https://api.weixin.qq.com/wxa/business/getliveinfo?access_token=ACCESS_TOKEN';
+ return $this->callPostApi($url, ['start' => $start, 'limit' => $limit], true);
+ }
+
+ /**
+ * 获取回放源视频
+ * @param array $data
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function getLiveInfo($data = [])
+ {
+ $url = 'https://api.weixin.qq.com/wxa/business/getliveinfo?access_token=ACCESS_TOKEN';
+ return $this->callPostApi($url, $data, true);
+ }
+
+ /**
+ * 直播间导入商品
+ * @param array $data
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function addLiveGoods($data = [])
+ {
+ $url = 'https://api.weixin.qq.com/wxaapi/broadcast/room/addgoods?access_token=ACCESS_TOKEN';
+ return $this->callPostApi($url, $data, true);
+ }
+
+ /**
+ * 商品添加并提审
+ * @param array $data
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function addGoods($data)
+ {
+ $url = "https://api.weixin.qq.com/wxaapi/broadcast/goods/add?access_token=ACCESS_TOKEN";
+ return $this->callPostApi($url, $data, true);
+ }
+
+ /**
+ * 商品撤回审核
+ * @param array $data
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function resetAuditGoods($data)
+ {
+ $url = "https://api.weixin.qq.com/wxaapi/broadcast/goods/resetaudit?access_token=ACCESS_TOKEN";
+ return $this->callPostApi($url, $data, true);
+ }
+
+ /**
+ * 重新提交审核
+ * @param array $data
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function auditGoods($data)
+ {
+ $url = "https://api.weixin.qq.com/wxaapi/broadcast/goods/audit?access_token=ACCESS_TOKEN";
+ return $this->callPostApi($url, $data, true);
+ }
+
+ /**
+ * 删除商品
+ * @param array $data
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function deleteGoods($data)
+ {
+ $url = "https://api.weixin.qq.com/wxaapi/broadcast/goods/delete?access_token=ACCESS_TOKEN";
+ return $this->callPostApi($url, $data, true);
+ }
+
+ /**
+ * 更新商品
+ * @param array $data
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function updateGoods($data)
+ {
+ $url = "https://api.weixin.qq.com/wxaapi/broadcast/goods/update?access_token=ACCESS_TOKEN";
+ return $this->callPostApi($url, $data, true);
+ }
+
+ /**
+ * 获取商品状态
+ * @param array $data
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function stateGoods($data)
+ {
+ $url = "https://api.weixin.qq.com/wxa/business/getgoodswarehouse?access_token=ACCESS_TOKEN";
+ return $this->callPostApi($url, $data, true);
+ }
+
+ /**
+ * 获取商品列表
+ * @param array $data
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function getGoods($data)
+ {
+ $url = "https://api.weixin.qq.com/wxaapi/broadcast/goods/getapproved?access_token=ACCESS_TOKEN";
+ return $this->callPostApi($url, $data, true);
+ }
+}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Logistics.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Logistics.php
index dc048bb5e19..876ab97909d 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Logistics.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Logistics.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
namespace WeMini;
@@ -33,7 +35,6 @@ class Logistics extends BasicWeChat
public function addOrder($data)
{
$url = 'https://api.weixin.qq.com/cgi-bin/express/business/order/add?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, $data, true);
}
@@ -47,7 +48,6 @@ public function addOrder($data)
public function cancelOrder($data)
{
$url = 'https://api.weixin.qq.com/cgi-bin/express/business/order/cancel?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, $data, true);
}
@@ -60,7 +60,6 @@ public function cancelOrder($data)
public function getAllDelivery()
{
$url = 'https://api.weixin.qq.com/cgi-bin/express/business/delivery/getall?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callGetApi($url);
}
@@ -74,7 +73,6 @@ public function getAllDelivery()
public function getOrder($data)
{
$url = 'https://api.weixin.qq.com/cgi-bin/express/business/order/get?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, $data, true);
}
@@ -88,7 +86,6 @@ public function getOrder($data)
public function getPath($data)
{
$url = 'https://api.weixin.qq.com/cgi-bin/express/business/path/get?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, $data, true);
}
@@ -101,7 +98,6 @@ public function getPath($data)
public function getPrinter()
{
$url = 'https://api.weixin.qq.com/cgi-bin/express/business/printer/getall?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callGetApi($url);
}
@@ -115,7 +111,6 @@ public function getPrinter()
public function getQuota($data)
{
$url = 'https://api.weixin.qq.com/cgi-bin/express/business/path/get?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, $data, true);
}
@@ -129,7 +124,6 @@ public function getQuota($data)
public function testUpdateOrder($data)
{
$url = 'https://api.weixin.qq.com/cgi-bin/express/business/test_update_order?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, $data, true);
}
@@ -143,7 +137,6 @@ public function testUpdateOrder($data)
public function updatePrinter($data)
{
$url = 'https://api.weixin.qq.com/cgi-bin/express/business/printer/update?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, $data, true);
}
@@ -157,7 +150,6 @@ public function updatePrinter($data)
public function getContact($data)
{
$url = 'https://api.weixin.qq.com/cgi-bin/express/delivery/contact/get?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, $data, true);
}
@@ -171,7 +163,6 @@ public function getContact($data)
public function previewTemplate($data)
{
$url = 'https://api.weixin.qq.com/cgi-bin/express/delivery/template/preview?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, $data, true);
}
@@ -185,7 +176,6 @@ public function previewTemplate($data)
public function updateBusiness($data)
{
$url = 'https://api.weixin.qq.com/cgi-bin/express/delivery/service/business/update?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, $data, true);
}
@@ -199,8 +189,6 @@ public function updateBusiness($data)
public function updatePath($data)
{
$url = 'https://api.weixin.qq.com/cgi-bin/express/delivery/path/update?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, $data, true);
}
-
}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Message.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Message.php
index fdb06a4c620..1ef90f14968 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Message.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Message.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
namespace WeMini;
@@ -33,7 +35,6 @@ class Message extends BasicWeChat
public function createActivityId($data)
{
$url = 'https://api.weixin.qq.com/cgi-bin/message/wxopen/activityid/create?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, $data, true);
}
@@ -47,7 +48,6 @@ public function createActivityId($data)
public function setUpdatableMsg($data)
{
$url = 'https://api.weixin.qq.com/cgi-bin/message/wxopen/updatablemsg/send?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, $data, true);
}
@@ -61,7 +61,6 @@ public function setUpdatableMsg($data)
public function uniformSend($data)
{
$url = 'https://api.weixin.qq.com/cgi-bin/message/wxopen/template/uniform_send?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, $data, true);
}
}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Newtmpl.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Newtmpl.php
index 80ae2ace0bb..a88ce631e00 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Newtmpl.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Newtmpl.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
namespace WeMini;
@@ -33,7 +35,6 @@ class Newtmpl extends BasicWeChat
public function addCategory($data)
{
$url = 'https://api.weixin.qq.com/cgi-bin/wxopen/addcategory?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, $data, true);
}
@@ -46,7 +47,6 @@ public function addCategory($data)
public function getCategory()
{
$url = 'https://api.weixin.qq.com/wxaapi/newtmpl/getcategory?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callGetApi($url);
}
@@ -59,7 +59,6 @@ public function getCategory()
public function deleteCategory()
{
$url = 'https://api.weixin.qq.com/cgi-bin/wxopen/deletecategory?access_token=TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, [], true);
}
@@ -73,8 +72,8 @@ public function deleteCategory()
public function getPubTemplateTitleList($ids)
{
$url = 'https://api.weixin.qq.com/wxaapi/newtmpl/getpubtemplatetitles?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->callPostApi($url, ['ids'=>$ids,'start' => '0', 'limit' => '30'], true);
+ $url .= '&' . http_build_query(['ids' => $ids, 'start' => '0', 'limit' => '30']);
+ return $this->callGetApi($url);
}
/**
@@ -87,8 +86,8 @@ public function getPubTemplateTitleList($ids)
public function getPubTemplateKeyWordsById($tid)
{
$url = 'https://api.weixin.qq.com/wxaapi/newtmpl/getpubtemplatekeywords?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->callPostApi($url, ['tid' => $tid], true);
+ $url .= '&' . http_build_query(['tid' => $tid]);
+ return $this->callGetApi($url);
}
/**
@@ -103,7 +102,6 @@ public function getPubTemplateKeyWordsById($tid)
public function addTemplate($tid, array $kidList, $sceneDesc = '')
{
$url = 'https://api.weixin.qq.com/wxaapi/newtmpl/addtemplate?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, ['tid' => $tid, 'kidList' => $kidList, 'sceneDesc' => $sceneDesc], false);
}
@@ -116,8 +114,7 @@ public function addTemplate($tid, array $kidList, $sceneDesc = '')
public function getTemplateList()
{
$url = 'https://api.weixin.qq.com/wxaapi/newtmpl/gettemplate?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->callPostApi($url, [], true);
+ return $this->callGetApi($url);
}
/**
@@ -130,7 +127,6 @@ public function getTemplateList()
public function delTemplate($priTmplId)
{
$url = 'https://api.weixin.qq.com/wxaapi/newtmpl/deltemplate?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, ['priTmplId' => $priTmplId], true);
}
@@ -144,8 +140,6 @@ public function delTemplate($priTmplId)
public function send(array $data)
{
$url = 'https://api.weixin.qq.com/cgi-bin/message/subscribe/send?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, $data, true);
}
-
}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Ocr.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Ocr.php
index 22b5048d7a0..57ca618e340 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Ocr.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Ocr.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
namespace WeMini;
@@ -32,9 +34,8 @@ class Ocr extends BasicWeChat
*/
public function bankcard($data)
{
- $url = 'https://api.weixin.qq.com/cv/ocr/bankcard?access_token=ACCESS_TOCKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->callPostApi($url, $data, true);
+ $url = 'https://api.weixin.qq.com/cv/ocr/bankcard?access_token=ACCESS_TOKEN';
+ return $this->callPostApi($url, $data, false);
}
/**
@@ -46,9 +47,8 @@ public function bankcard($data)
*/
public function businessLicense($data)
{
- $url = 'https://api.weixin.qq.com/cv/ocr/bizlicense?access_token=ACCESS_TOCKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->callPostApi($url, $data, true);
+ $url = 'https://api.weixin.qq.com/cv/ocr/bizlicense?access_token=ACCESS_TOKEN';
+ return $this->callPostApi($url, $data, false);
}
/**
@@ -60,9 +60,8 @@ public function businessLicense($data)
*/
public function driverLicense($data)
{
- $url = 'https://api.weixin.qq.com/cv/ocr/drivinglicense?access_token=ACCESS_TOCKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->callPostApi($url, $data, true);
+ $url = 'https://api.weixin.qq.com/cv/ocr/drivinglicense?access_token=ACCESS_TOKEN';
+ return $this->callPostApi($url, $data, false);
}
/**
@@ -74,9 +73,8 @@ public function driverLicense($data)
*/
public function idcard($data)
{
- $url = 'https://api.weixin.qq.com/cv/ocr/idcard?access_token=ACCESS_TOCKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->callPostApi($url, $data, true);
+ $url = 'https://api.weixin.qq.com/cv/ocr/idcard?access_token=ACCESS_TOKEN';
+ return $this->callPostApi($url, $data, false);
}
/**
@@ -88,9 +86,8 @@ public function idcard($data)
*/
public function printedText($data)
{
- $url = 'https://api.weixin.qq.com/cv/ocr/comm?access_token=ACCESS_TOCKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->callPostApi($url, $data, true);
+ $url = 'https://api.weixin.qq.com/cv/ocr/comm?access_token=ACCESS_TOKEN';
+ return $this->callPostApi($url, $data, false);
}
/**
@@ -102,9 +99,7 @@ public function printedText($data)
*/
public function vehicleLicense($data)
{
- $url = 'https://api.weixin.qq.com/cv/ocr/driving?access_token=ACCESS_TOCKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->callPostApi($url, $data, true);
+ $url = 'https://api.weixin.qq.com/cv/ocr/driving?access_token=ACCESS_TOKEN';
+ return $this->callPostApi($url, $data, false);
}
-
}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Operation.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Operation.php
new file mode 100644
index 00000000000..8708b24360a
--- /dev/null
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Operation.php
@@ -0,0 +1,41 @@
+callPostApi($url, $data, true);
+ }
+}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Plugs.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Plugs.php
index 6110e89cdc3..a8f9a7e528a 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Plugs.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Plugs.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
namespace WeMini;
@@ -33,7 +35,6 @@ class Plugs extends BasicWeChat
public function apply($plugin_appid)
{
$url = 'https://api.weixin.qq.com/wxa/plugin?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, ['action' => 'apply', 'plugin_appid' => $plugin_appid], true);
}
@@ -46,7 +47,6 @@ public function apply($plugin_appid)
public function getList()
{
$url = 'https://api.weixin.qq.com/wxa/plugin?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, ['action' => 'list'], true);
}
@@ -60,7 +60,6 @@ public function getList()
public function unbind($plugin_appid)
{
$url = 'https://api.weixin.qq.com/wxa/plugin?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, ['action' => 'unbind', 'plugin_appid' => $plugin_appid], true);
}
@@ -75,7 +74,6 @@ public function unbind($plugin_appid)
public function devplugin($data)
{
$url = 'https://api.weixin.qq.com/wxa/devplugin?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, $data, true);
}
@@ -90,7 +88,6 @@ public function devplugin($data)
public function devApplyList($page = 1, $num = 10)
{
$url = 'https://api.weixin.qq.com/wxa/plugin?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
$data = ['action' => 'dev_apply_list', 'page' => $page, 'num' => $num];
return $this->callPostApi($url, $data, true);
}
@@ -105,8 +102,6 @@ public function devApplyList($page = 1, $num = 10)
public function devAgree($action = 'dev_agree')
{
$url = 'https://api.weixin.qq.com/wxa/plugin?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, ['action' => $action], true);
}
-
}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Poi.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Poi.php
index c81ef5d2d0e..800a39bd571 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Poi.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Poi.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
namespace WeMini;
@@ -36,7 +38,6 @@ class Poi extends BasicWeChat
public function addBearByPoi($related_name, $related_credential, $related_address, $related_proof_material)
{
$url = 'https://api.weixin.qq.com/wxa/addnearbypoi?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
$data = [
'related_name' => $related_name, 'related_credential' => $related_credential,
'related_address' => $related_address, 'related_proof_material' => $related_proof_material,
@@ -55,7 +56,6 @@ public function addBearByPoi($related_name, $related_credential, $related_addres
public function getNearByPoiList($page = 1, $page_rows = 1000)
{
$url = "https://api.weixin.qq.com/wxa/getnearbypoilist?page={$page}&page_rows={$page_rows}&access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callGetApi($url);
}
@@ -69,7 +69,6 @@ public function getNearByPoiList($page = 1, $page_rows = 1000)
public function delNearByPoiList($poi_id)
{
$url = "https://api.weixin.qq.com/wxa/delnearbypoi?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, ['poi_id' => $poi_id], true);
}
@@ -84,8 +83,6 @@ public function delNearByPoiList($poi_id)
public function setNearByPoiShowStatus($poi_id, $status)
{
$url = "https://api.weixin.qq.com/wxa/setnearbypoishowstatus?access_token=ACCESS_TOKEN";
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, ['poi_id' => $poi_id, 'status' => $status], true);
}
-
}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Qrcode.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Qrcode.php
index 4c238b34d80..2bb26106cfb 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Qrcode.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Qrcode.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
namespace WeMini;
@@ -18,40 +20,40 @@
use WeChat\Contracts\Tools;
/**
- * 微信小程序二维码管理
+ * 微信小程序二维码
* Class Qrcode
* @package WeMini
*/
class Qrcode extends BasicWeChat
{
+ /**
+ * 默认线条颜色
+ * @var string[]
+ */
+ private $lineColor = ["r" => "0", "g" => "0", "b" => "0"];
+
/**
* 获取小程序码(永久有效)
* 接口A: 适用于需要的码数量较少的业务场景
* @param string $path 不能为空,最大长度 128 字节
* @param integer $width 二维码的宽度
- * @param bool $auto_color 自动配置线条颜色,如果颜色依然是黑色,则说明不建议配置主色调
- * @param array $line_color auto_color 为 false 时生效
- * @param boolean $is_hyaline 是否需要透明底色
- * @param null|string $outType 输出类型
- * @return array|string
+ * @param bool $autoColor 自动配置线条颜色,如果颜色依然是黑色,则说明不建议配置主色调
+ * @param null|array $lineColor auto_color 为 false 时生效
+ * @param boolean $isHyaline 透明底色
+ * @param string|null $outType 输出类型
+ * @param array $extra 其他参数
+ * @return string|array
* @throws \WeChat\Exceptions\InvalidResponseException
* @throws \WeChat\Exceptions\LocalCacheException
*/
- public function createMiniPath($path, $width = 430, $auto_color = false, $line_color = ["r" => "0", "g" => "0", "b" => "0"], $is_hyaline = true, $outType = null)
+ public function createMiniPath($path, $width = 430, $autoColor = false, $lineColor = null, $isHyaline = true, $outType = null, array $extra = [])
{
$url = 'https://api.weixin.qq.com/wxa/getwxacode?access_token=ACCESS_TOKEN';
$this->registerApi($url, __FUNCTION__, func_get_args());
- $data = ['path' => $path, 'width' => $width, 'auto_color' => $auto_color, 'line_color' => $line_color, 'is_hyaline' => $is_hyaline];
- $result = Tools::post($url, Tools::arr2json($data));
- if (is_array($json = json_decode($result, true))) {
- if (!$this->isTry && isset($json['errcode']) && in_array($json['errcode'], ['40014', '40001', '41001', '42001'])) {
- [$this->delAccessToken(), $this->isTry = true];
- return call_user_func_array([$this, $this->currentMethod['method']], $this->currentMethod['arguments']);
- }
- return Tools::json2arr($result);
- }
- return is_null($outType) ? $result : $outType($result);
+ $lineColor = empty($lineColor) ? $this->lineColor : $lineColor;
+ $data = ['path' => $path, 'width' => $width, 'auto_color' => $autoColor, 'line_color' => $lineColor, 'is_hyaline' => $isHyaline];
+ return $this->parseResult(Tools::post($url, Tools::arr2json(array_merge($data, $extra))), $outType);
}
/**
@@ -60,28 +62,23 @@ public function createMiniPath($path, $width = 430, $auto_color = false, $line_c
* @param string $scene 最大32个可见字符,只支持数字
* @param string $page 必须是已经发布的小程序存在的页面
* @param integer $width 二维码的宽度
- * @param bool $auto_color 自动配置线条颜色,如果颜色依然是黑色,则说明不建议配置主色调
- * @param array $line_color auto_color 为 false 时生效
- * @param boolean $is_hyaline 是否需要透明底色
+ * @param bool $autoColor 自动配置线条颜色,如果颜色依然是黑色,则说明不建议配置主色调
+ * @param null|array $lineColor auto_color 为 false 时生效
+ * @param bool $isHyaline 是否需要透明底色
* @param null|string $outType 输出类型
+ * @param array $extra 其他参数
* @return array|string
* @throws \WeChat\Exceptions\InvalidResponseException
* @throws \WeChat\Exceptions\LocalCacheException
*/
- public function createMiniScene($scene, $page, $width = 430, $auto_color = false, $line_color = ["r" => "0", "g" => "0", "b" => "0"], $is_hyaline = true, $outType = null)
+ public function createMiniScene($scene, $page = '', $width = 430, $autoColor = false, $lineColor = null, $isHyaline = true, $outType = null, array $extra = [])
{
$url = 'https://api.weixin.qq.com/wxa/getwxacodeunlimit?access_token=ACCESS_TOKEN';
- $data = ['scene' => $scene, 'width' => $width, 'auto_color' => $auto_color, 'page' => $page, 'line_color' => $line_color, 'is_hyaline' => $is_hyaline];
$this->registerApi($url, __FUNCTION__, func_get_args());
- $result = Tools::post($url, Tools::arr2json($data));
- if (is_array($json = json_decode($result, true))) {
- if (!$this->isTry && isset($json['errcode']) && in_array($json['errcode'], ['40014', '40001', '41001', '42001'])) {
- [$this->delAccessToken(), $this->isTry = true];
- return call_user_func_array([$this, $this->currentMethod['method']], $this->currentMethod['arguments']);
- }
- return Tools::json2arr($result);
- }
- return is_null($outType) ? $result : $outType($result);
+ $lineColor = empty($lineColor) ? $this->lineColor : $lineColor;
+ $data = ['scene' => $scene, 'width' => $width, 'page' => $page, 'auto_color' => $autoColor, 'line_color' => $lineColor, 'is_hyaline' => $isHyaline, 'check_path' => false];
+ if (empty($page)) unset($data['page']);
+ return $this->parseResult(Tools::post($url, Tools::arr2json(array_merge($data, $extra))), $outType);
}
/**
@@ -89,7 +86,7 @@ public function createMiniScene($scene, $page, $width = 430, $auto_color = false
* 接口C:适用于需要的码数量较少的业务场景
* @param string $path 不能为空,最大长度 128 字节
* @param integer $width 二维码的宽度
- * @param null|string $outType 输出类型
+ * @param string|null $outType 输出类型
* @return array|string
* @throws \WeChat\Exceptions\InvalidResponseException
* @throws \WeChat\Exceptions\LocalCacheException
@@ -98,15 +95,26 @@ public function createDefault($path, $width = 430, $outType = null)
{
$url = 'https://api.weixin.qq.com/cgi-bin/wxaapp/createwxaqrcode?access_token=ACCESS_TOKEN';
$this->registerApi($url, __FUNCTION__, func_get_args());
- $result = Tools::post($url, Tools::arr2json(['path' => $path, 'width' => $width]));
+ return $this->parseResult(Tools::post($url, Tools::arr2json(['path' => $path, 'width' => $width])), $outType);
+ }
+
+ /**
+ * 解释接口数据
+ * @param bool|string $result
+ * @param null|string $outType
+ * @return array|mixed
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ */
+ private function parseResult($result, $outType)
+ {
if (is_array($json = json_decode($result, true))) {
if (!$this->isTry && isset($json['errcode']) && in_array($json['errcode'], ['40014', '40001', '41001', '42001'])) {
[$this->delAccessToken(), $this->isTry = true];
return call_user_func_array([$this, $this->currentMethod['method']], $this->currentMethod['arguments']);
}
return Tools::json2arr($result);
+ } else {
+ return is_null($outType) ? $result : $outType($result);
}
- return is_null($outType) ? $result : $outType($result);
}
-
}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Scheme.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Scheme.php
new file mode 100644
index 00000000000..909c4a38b42
--- /dev/null
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Scheme.php
@@ -0,0 +1,80 @@
+callPostApi($url, $data, true);
+ }
+
+ /**
+ * 查询 URL-Scheme
+ * @param string $scheme
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function query($scheme)
+ {
+ $url = 'https://api.weixin.qq.com/wxa/queryscheme?access_token=ACCESS_TOKEN';
+ return $this->callPostApi($url, ['scheme' => $scheme], true);
+ }
+
+ /**
+ * 创建 URL-Link
+ * @param array $data
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function urlLink($data)
+ {
+ $url = "https://api.weixin.qq.com/wxa/generate_urllink?access_token=ACCESS_TOKEN";
+ return $this->callPostApi($url, $data, true);
+ }
+
+ /**
+ * 查询 URL-Link
+ * @param string $urllink
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function urlQuery($urllink)
+ {
+ $url = 'https://api.weixin.qq.com/wxa/query_urllink?access_token=ACCESS_TOKEN';
+ return $this->callPostApi($url, ['url_link' => $urllink], true);
+ }
+}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Search.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Search.php
new file mode 100644
index 00000000000..2368c5e45a3
--- /dev/null
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Search.php
@@ -0,0 +1,40 @@
+callPostApi($url, ['pages' => $pages], true);
+ }
+}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Security.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Security.php
index 5b1901fa4ea..0a5e7315bc8 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Security.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Security.php
@@ -3,19 +3,20 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
namespace WeMini;
use WeChat\Contracts\BasicWeChat;
-use WeChat\Exceptions\InvalidResponseException;
/**
* 小程序内容安全
@@ -35,8 +36,7 @@ class Security extends BasicWeChat
public function imgSecCheck($media)
{
$url = 'https://api.weixin.qq.com/wxa/img_sec_check?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->callPostApi($url, ['media' => $media], true);
+ return $this->callPostApi($url, ['media' => $media], false);
}
/**
@@ -44,13 +44,12 @@ public function imgSecCheck($media)
* @param string $media_url
* @param string $media_type
* @return array
- * @throws InvalidResponseException
+ * @throws \WeChat\Exceptions\InvalidResponseException
* @throws \WeChat\Exceptions\LocalCacheException
*/
public function mediaCheckAsync($media_url, $media_type)
{
$url = 'https://api.weixin.qq.com/wxa/media_check_async?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, ['media_url' => $media_url, 'media_type' => $media_type], true);
}
@@ -58,13 +57,12 @@ public function mediaCheckAsync($media_url, $media_type)
* 检查一段文本是否含有违法违规内容
* @param string $content
* @return array
- * @throws InvalidResponseException
+ * @throws \WeChat\Exceptions\InvalidResponseException
* @throws \WeChat\Exceptions\LocalCacheException
*/
public function msgSecCheck($content)
{
$url = 'https://api.weixin.qq.com/wxa/msg_sec_check?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, ['content' => $content], true);
}
}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Shipping.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Shipping.php
new file mode 100644
index 00000000000..b797d662eb4
--- /dev/null
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Shipping.php
@@ -0,0 +1,118 @@
+callPostApi($url, $data, true);
+ }
+
+ /**
+ * 发货信息合单录入接口
+ * @param array $data
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function combined($data)
+ {
+ $url = 'https://api.weixin.qq.com/wxa/sec/order/upload_combined_shipping_info?access_token=ACCESS_TOKEN';
+ return $this->callPostApi($url, $data, true);
+ }
+
+ /**
+ * 查询订单发货状态
+ * @param array $data
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function query($data)
+ {
+ $url = 'https://api.weixin.qq.com/wxa/sec/order/get_order?access_token=ACCESS_TOKEN';
+ return $this->callPostApi($url, $data, true);
+ }
+
+ /**
+ * 查询订单列表
+ * @param array $data
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function qlist($data)
+ {
+ $url = 'https://api.weixin.qq.com/wxa/sec/order/get_order_list?access_token=ACCESS_TOKEN';
+ return $this->callPostApi($url, $data, true);
+ }
+
+ /**
+ * 确认收货提醒接口
+ * @param array $data
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function confirm($data)
+ {
+ $url = 'https://api.weixin.qq.com/wxa/sec/order/notify_confirm_receive?access_token=ACCESS_TOKEN';
+ return $this->callPostApi($url, $data, true);
+ }
+
+ /**
+ * 消息跳转路径设置接口
+ * @param array $data
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function setJump($data)
+ {
+ $url = 'https://api.weixin.qq.com/wxa/sec/order/set_msg_jump_path?access_token=ACCESS_TOKEN';
+ return $this->callPostApi($url, $data, true);
+ }
+
+ /**
+ * 查询小程序是否已开通发货信息管理服务
+ * @param array $data
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function isTrade($data)
+ {
+ $url = 'https://api.weixin.qq.com/wxa/sec/order/is_trade_managed?access_token=ACCESS_TOKEN';
+ return $this->callPostApi($url, $data, true);
+ }
+
+ /**
+ * 查询小程序是否已完成交易结算管理确认
+ * @param array $data
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function isCompleted($data)
+ {
+ $url = 'https://api.weixin.qq.com/wxa/sec/order/is_trade_management_confirmation_completed?access_token=ACCESS_TOKEN';
+ return $this->callPostApi($url, $data, true);
+ }
+}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Soter.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Soter.php
index 789056896cc..37dc54cc3bb 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Soter.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Soter.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
namespace WeMini;
@@ -33,8 +35,6 @@ class Soter extends BasicWeChat
public function verifySignature($data)
{
$url = 'https://api.weixin.qq.com/cgi-bin/soter/verify_signature?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, $data, true);
}
-
}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Template.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Template.php
index 516d407416d..074d8fc73b6 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Template.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Template.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
namespace WeMini;
@@ -33,7 +35,6 @@ class Template extends BasicWeChat
public function getTemplateLibraryList()
{
$url = 'https://api.weixin.qq.com/cgi-bin/wxopen/template/library/list?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, ['offset' => '0', 'count' => '20'], true);
}
@@ -47,7 +48,6 @@ public function getTemplateLibraryList()
public function getTemplateLibrary($template_id)
{
$url = 'https://api.weixin.qq.com/cgi-bin/wxopen/template/library/get?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, ['id' => $template_id], true);
}
@@ -62,7 +62,6 @@ public function getTemplateLibrary($template_id)
public function addTemplate($template_id, array $keyword_id_list)
{
$url = 'https://api.weixin.qq.com/cgi-bin/wxopen/template/add?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, ['id' => $template_id, 'keyword_id_list' => $keyword_id_list], true);
}
@@ -75,7 +74,6 @@ public function addTemplate($template_id, array $keyword_id_list)
public function getTemplateList()
{
$url = 'https://api.weixin.qq.com/cgi-bin/wxopen/template/list?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, ['offset' => '0', 'count' => '20'], true);
}
@@ -89,7 +87,6 @@ public function getTemplateList()
public function delTemplate($template_id)
{
$url = 'https://api.weixin.qq.com/cgi-bin/wxopen/template/del?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, ['template_id' => $template_id], true);
}
@@ -103,8 +100,6 @@ public function delTemplate($template_id)
public function send(array $data)
{
$url = 'https://api.weixin.qq.com/cgi-bin/message/wxopen/template/send?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, $data, true);
}
-
}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Total.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Total.php
index 9d55a6b5b75..ad09ff9019f 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Total.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/Total.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
namespace WeMini;
@@ -25,32 +27,30 @@ class Total extends BasicWeChat
{
/**
* 数据分析接口
- * @param string $begin_date 开始日期
- * @param string $end_date 结束日期,限定查询1天数据,end_date允许设置的最大值为昨日
+ * @param string $beginDate 开始日期
+ * @param string $endDate 结束日期,限定查询1天数据,end_date允许设置的最大值为昨日
* @return array
* @throws \WeChat\Exceptions\InvalidResponseException
* @throws \WeChat\Exceptions\LocalCacheException
*/
- public function getWeanalysisAppidDailySummarytrend($begin_date, $end_date)
+ public function getWeanalysisAppidDailySummarytrend($beginDate, $endDate)
{
$url = 'https://api.weixin.qq.com/datacube/getweanalysisappiddailysummarytrend?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->callPostApi($url, ['begin_date' => $begin_date, 'end_date' => $end_date], true);
+ return $this->callPostApi($url, ['begin_date' => $beginDate, 'end_date' => $endDate], true);
}
/**
* 访问分析
- * @param string $begin_date 开始日期
- * @param string $end_date 结束日期,限定查询1天数据,end_date允许设置的最大值为昨日
+ * @param string $beginDate 开始日期
+ * @param string $endDate 结束日期,限定查询1天数据,end_date允许设置的最大值为昨日
* @return array
* @throws \WeChat\Exceptions\InvalidResponseException
* @throws \WeChat\Exceptions\LocalCacheException
*/
- public function getWeanalysisAppidDailyVisittrend($begin_date, $end_date)
+ public function getWeanalysisAppidDailyVisittrend($beginDate, $endDate)
{
$url = 'https://api.weixin.qq.com/datacube/getweanalysisappiddailyvisittrend?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
- return $this->callPostApi($url, ['begin_date' => $begin_date, 'end_date' => $end_date], true);
+ return $this->callPostApi($url, ['begin_date' => $beginDate, 'end_date' => $endDate], true);
}
/**
@@ -64,7 +64,6 @@ public function getWeanalysisAppidDailyVisittrend($begin_date, $end_date)
public function getWeanalysisAppidWeeklyVisittrend($begin_date, $end_date)
{
$url = 'https://api.weixin.qq.com/datacube/getweanalysisappidweeklyvisittrend?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, ['begin_date' => $begin_date, 'end_date' => $end_date], true);
}
@@ -79,7 +78,6 @@ public function getWeanalysisAppidWeeklyVisittrend($begin_date, $end_date)
public function getWeanalysisAppidMonthlyVisittrend($begin_date, $end_date)
{
$url = 'https://api.weixin.qq.com/datacube/getweanalysisappidmonthlyvisittrend?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, ['begin_date' => $begin_date, 'end_date' => $end_date], true);
}
@@ -94,7 +92,6 @@ public function getWeanalysisAppidMonthlyVisittrend($begin_date, $end_date)
public function getWeanalysisAppidVisitdistribution($begin_date, $end_date)
{
$url = 'https://api.weixin.qq.com/datacube/getweanalysisappidvisitdistribution?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, ['begin_date' => $begin_date, 'end_date' => $end_date], true);
}
@@ -109,7 +106,6 @@ public function getWeanalysisAppidVisitdistribution($begin_date, $end_date)
public function getWeanalysisAppidDailyRetaininfo($begin_date, $end_date)
{
$url = 'https://api.weixin.qq.com/datacube/getweanalysisappiddailyretaininfo?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, ['begin_date' => $begin_date, 'end_date' => $end_date], true);
}
@@ -124,7 +120,6 @@ public function getWeanalysisAppidDailyRetaininfo($begin_date, $end_date)
public function getWeanalysisAppidWeeklyRetaininfo($begin_date, $end_date)
{
$url = 'https://api.weixin.qq.com/datacube/getweanalysisappidweeklyretaininfo?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, ['begin_date' => $begin_date, 'end_date' => $end_date], true);
}
@@ -139,7 +134,6 @@ public function getWeanalysisAppidWeeklyRetaininfo($begin_date, $end_date)
public function getWeanalysisAppidMonthlyRetaininfo($begin_date, $end_date)
{
$url = 'https://api.weixin.qq.com/datacube/getweanalysisappidmonthlyretaininfo?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, ['begin_date' => $begin_date, 'end_date' => $end_date], true);
}
@@ -154,7 +148,6 @@ public function getWeanalysisAppidMonthlyRetaininfo($begin_date, $end_date)
public function getWeanalysisAppidVisitPage($begin_date, $end_date)
{
$url = 'https://api.weixin.qq.com/datacube/getweanalysisappidvisitpage?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, ['begin_date' => $begin_date, 'end_date' => $end_date], true);
}
@@ -169,8 +162,6 @@ public function getWeanalysisAppidVisitPage($begin_date, $end_date)
public function getWeanalysisAppidUserportrait($begin_date, $end_date)
{
$url = 'https://api.weixin.qq.com/datacube/getweanalysisappiduserportrait?access_token=ACCESS_TOKEN';
- $this->registerApi($url, __FUNCTION__, func_get_args());
return $this->callPostApi($url, ['begin_date' => $begin_date, 'end_date' => $end_date], true);
}
-
}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/crypt/wxBizDataCrypt.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/crypt/wxBizDataCrypt.php
index d969638efff..abc80abe467 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/crypt/wxBizDataCrypt.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WeMini/crypt/wxBizDataCrypt.php
@@ -33,24 +33,25 @@ public function __construct($appid, $sessionKey)
public function decryptData($encryptedData, $iv, &$data)
{
if (strlen($this->sessionKey) != 24) {
- return \ErrorCode::$IllegalAesKey;
+ return ErrorCode::$IllegalAesKey;
}
$aesKey = base64_decode($this->sessionKey);
if (strlen($iv) != 24) {
- return \ErrorCode::$IllegalIv;
+ return ErrorCode::$IllegalIv;
}
$aesIV = base64_decode($iv);
$aesCipher = base64_decode($encryptedData);
$result = openssl_decrypt($aesCipher, "AES-128-CBC", $aesKey, 1, $aesIV);
$dataObj = json_decode($result);
if ($dataObj == null) {
- return \ErrorCode::$IllegalBuffer;
+ return ErrorCode::$IllegalBuffer;
}
- if ($dataObj->watermark->appid != $this->appid) {
- return \ErrorCode::$IllegalBuffer;
+ // 兼容新版本无 watermark 的情况
+ if (isset($dataObj->watermark) && $dataObj->watermark->appid != $this->appid) {
+ return ErrorCode::$IllegalBuffer;
}
$data = $result;
- return \ErrorCode::$OK;
+ return ErrorCode::$OK;
}
}
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WePay/Bill.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WePay/Bill.php
index 4c853c700e5..721fff3599a 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WePay/Bill.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WePay/Bill.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
namespace WePay;
@@ -30,7 +32,7 @@ class Bill extends BasicWePay
* @param array $options 静音参数
* @param null|string $outType 输出类型
* @return bool|string
- * @throws InvalidResponseException
+ * @throws \WeChat\Exceptions\InvalidResponseException
* @throws \WeChat\Exceptions\LocalCacheException
*/
public function download(array $options, $outType = null)
@@ -39,7 +41,7 @@ public function download(array $options, $outType = null)
$params = $this->params->merge($options);
$params['sign'] = $this->getPaySign($params, 'MD5');
$result = Tools::post('https://api.mch.weixin.qq.com/pay/downloadbill', Tools::arr2xml($params));
- if (($jsonData = Tools::xml2arr($result))) {
+ if (is_array($jsonData = Tools::xml3arr($result))) {
if ($jsonData['return_code'] !== 'SUCCESS') {
throw new InvalidResponseException($jsonData['return_msg'], '0');
}
@@ -52,7 +54,7 @@ public function download(array $options, $outType = null)
* 拉取订单评价数据
* @param array $options
* @return array
- * @throws InvalidResponseException
+ * @throws \WeChat\Exceptions\InvalidResponseException
* @throws \WeChat\Exceptions\LocalCacheException
*/
public function comment(array $options)
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WePay/Coupon.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WePay/Coupon.php
index 92ca5c06f3f..00db86f4f9c 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WePay/Coupon.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WePay/Coupon.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
namespace WePay;
@@ -33,7 +35,7 @@ class Coupon extends BasicWePay
public function create(array $options)
{
$url = "https://api.mch.weixin.qq.com/mmpaymkttransfers/send_coupon";
- return $this->callPostApi($url, $options, true);
+ return $this->callPostApi($url, $options, true, 'MD5');
}
/**
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WePay/Custom.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WePay/Custom.php
new file mode 100644
index 00000000000..58e729f7e69
--- /dev/null
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WePay/Custom.php
@@ -0,0 +1,69 @@
+callPostApi($url, $options, false, 'MD5', false, false);
+ }
+
+ /**
+ * 订单附加信息查询接口
+ * @param array $options
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function get(array $options = [])
+ {
+ $url = 'https://api.mch.weixin.qq.com/cgi-bin/mch/customs/customdeclarequery';
+ return $this->callPostApi($url, $options, false, 'MD5', true, false);
+ }
+
+
+ /**
+ * 订单附加信息重推接口
+ * @param array $options
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function reset(array $options = [])
+ {
+ $url = 'https://api.mch.weixin.qq.com/cgi-bin/mch/newcustoms/customdeclareredeclare';
+ return $this->callPostApi($url, $options, false, 'MD5', true, false);
+ }
+
+}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WePay/Order.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WePay/Order.php
index 1d93288fe6f..44165658a32 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WePay/Order.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WePay/Order.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
namespace WePay;
@@ -155,7 +157,7 @@ public function reverse(array $options)
public function queryAuthCode($authCode)
{
$url = 'https://api.mch.weixin.qq.com/tools/authcodetoopenid';
- return $this->callPostApi($url, ['auth_code' => $authCode]);
+ return $this->callPostApi($url, ['auth_code' => $authCode], false, 'MD5', false);
}
/**
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WePay/ProfitSharing.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WePay/ProfitSharing.php
new file mode 100644
index 00000000000..f1a93536688
--- /dev/null
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WePay/ProfitSharing.php
@@ -0,0 +1,145 @@
+callPostApi($url, $options, true);
+ }
+
+ /**
+ * 请求多次分账
+ * @param array $options
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function multiProfitSharing(array $options)
+ {
+ $url = 'https://api.mch.weixin.qq.com/secapi/pay/multiprofitsharing';
+ return $this->callPostApi($url, $options, true);
+ }
+
+ /**
+ * 查询分账结果
+ * @param array $options
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function profitSharingQuery(array $options)
+ {
+ $url = 'https://api.mch.weixin.qq.com/pay/profitsharingquery';
+ return $this->callPostApi($url, $options);
+ }
+
+ /**
+ * 添加分账接收方
+ * @param array $options
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function profitSharingAddReceiver(array $options)
+ {
+ $url = 'https://api.mch.weixin.qq.com/pay/profitsharingaddreceiver';
+ return $this->callPostApi($url, $options);
+ }
+
+ /**
+ * 删除分账接收方
+ * @param array $options
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function profitSharingRemoveReceiver(array $options)
+ {
+ $url = 'https://api.mch.weixin.qq.com/pay/profitsharingremovereceiver';
+ return $this->callPostApi($url, $options);
+ }
+
+ /**
+ * 完结分账
+ * @param array $options
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function profitSharingFinish(array $options)
+ {
+ $url = 'https://api.mch.weixin.qq.com/secapi/pay/profitsharingfinish';
+ return $this->callPostApi($url, $options, true);
+ }
+
+ /**
+ * 查询订单待分账金额
+ * @param array $options
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function profitSharingOrderAmountQuery(array $options)
+ {
+ $url = 'https://api.mch.weixin.qq.com/pay/profitsharingorderamountquery';
+ return $this->callPostApi($url, $options);
+ }
+
+ /**
+ * 分账回退
+ * @param array $options
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function profitSharingReturn(array $options)
+ {
+ $url = 'https://api.mch.weixin.qq.com/secapi/pay/profitsharingreturn';
+ return $this->callPostApi($url, $options, true);
+ }
+
+ /**
+ * 回退结果查询
+ * @param array $options
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function profitSharingReturnQuery(array $options)
+ {
+ $url = 'https://api.mch.weixin.qq.com/pay/profitsharingreturnquery';
+ return $this->callPostApi($url, $options);
+ }
+}
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WePay/Redpack.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WePay/Redpack.php
index bf97ad89f53..26fd1afba76 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WePay/Redpack.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WePay/Redpack.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
namespace WePay;
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WePay/Refund.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WePay/Refund.php
index 8e3c199fccb..14c61b051d5 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WePay/Refund.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WePay/Refund.php
@@ -3,19 +3,22 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
namespace WePay;
use WeChat\Contracts\BasicWePay;
use WeChat\Contracts\Tools;
+use WeChat\Exceptions\InvalidDecryptException;
use WeChat\Exceptions\InvalidResponseException;
/**
@@ -30,7 +33,7 @@ class Refund extends BasicWePay
* 创建退款订单
* @param array $options
* @return array
- * @throws InvalidResponseException
+ * @throws \WeChat\Exceptions\InvalidResponseException
* @throws \WeChat\Exceptions\LocalCacheException
*/
public function create(array $options)
@@ -43,7 +46,7 @@ public function create(array $options)
* 查询退款
* @param array $options
* @return array
- * @throws InvalidResponseException
+ * @throws \WeChat\Exceptions\InvalidResponseException
* @throws \WeChat\Exceptions\LocalCacheException
*/
public function query(array $options)
@@ -54,25 +57,25 @@ public function query(array $options)
/**
* 获取退款通知
+ * @param string $xml
* @return array
- * @throws InvalidResponseException
+ * @throws \WeChat\Exceptions\InvalidDecryptException
+ * @throws \WeChat\Exceptions\InvalidResponseException
*/
- public function getNotify()
+ public function getNotify($xml = '')
{
- $data = Tools::xml2arr(file_get_contents("php://input"));
+ $data = Tools::xml2arr(empty($xml) ? Tools::getRawInput() : $xml);
if (!isset($data['return_code']) || $data['return_code'] !== 'SUCCESS') {
throw new InvalidResponseException('获取退款通知XML失败!');
}
- if (!class_exists('Prpcrypt', false)) {
- include dirname(__DIR__) . '/WeChat/Contracts/Prpcrypt.php';
+ try {
+ $key = md5($this->config->get('mch_key'));
+ $decrypt = base64_decode($data['req_info']);
+ $response = openssl_decrypt($decrypt, 'aes-256-ecb', $key, OPENSSL_RAW_DATA);
+ $data['result'] = Tools::xml2arr($response);
+ return $data;
+ } catch (\Exception $exception) {
+ throw new InvalidDecryptException($exception->getMessage(), $exception->getCode());
}
- $pc = new \Prpcrypt(md5($this->config->get('mch_key')));
- $array = $pc->decrypt(base64_decode($data['req_info']));
- if (intval($array[0]) > 0) {
- throw new InvalidResponseException($array[1], $array[0]);
- }
- $data['decode'] = $array[1];
- return $data;
}
-
}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WePay/Transfers.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WePay/Transfers.php
index 89ff1d8c1a3..ce5af6bfb45 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WePay/Transfers.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WePay/Transfers.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
namespace WePay;
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WePay/TransfersBank.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WePay/TransfersBank.php
index 0509d010a2a..e187c01e165 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/WePay/TransfersBank.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WePay/TransfersBank.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
namespace WePay;
@@ -68,7 +70,7 @@ public function create(array $options)
* 商户企业付款到银行卡操作进行结果查询
* @param string $partnerTradeNo 商户订单号,需保持唯一
* @return array
- * @throws InvalidResponseException
+ * @throws \WeChat\Exceptions\InvalidResponseException
* @throws \WeChat\Exceptions\LocalCacheException
*/
public function query($partnerTradeNo)
@@ -121,5 +123,4 @@ private function getRsaContent()
Tools::setCache($cacheKey, $data['pub_key'], 600);
return $data['pub_key'];
}
-
}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WePayV3/Cert.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WePayV3/Cert.php
new file mode 100644
index 00000000000..7a4f4887731
--- /dev/null
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WePayV3/Cert.php
@@ -0,0 +1,63 @@
+config['mch_v3_key']);
+ $result = $this->doRequest('GET', '/v3/certificates');
+ $certs = [];
+ foreach ($result['data'] as $vo) {
+ $certs[$vo['serial_no']] = [
+ 'expire' => strtotime($vo['expire_time']),
+ 'content' => $aes->decryptToString(
+ $vo['encrypt_certificate']['associated_data'],
+ $vo['encrypt_certificate']['nonce'],
+ $vo['encrypt_certificate']['ciphertext']
+ )
+ ];
+ }
+ $this->tmpFile("{$this->config['mch_id']}_certs", $certs);
+ } catch (\Exception $exception) {
+ throw new InvalidResponseException($exception->getMessage(), $exception->getCode());
+ }
+ }
+}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WePayV3/Contracts/BasicWePay.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WePayV3/Contracts/BasicWePay.php
new file mode 100644
index 00000000000..c271c4c5e5f
--- /dev/null
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WePayV3/Contracts/BasicWePay.php
@@ -0,0 +1,352 @@
+ '', // 微信绑定APPID,需配置
+ 'mch_id' => '', // 微信商户编号,需要配置
+ 'mch_v3_key' => '', // 微信商户密钥,需要配置
+ 'cert_serial' => '', // 商户证书序号,无需配置
+ 'cert_public' => '', // 商户公钥内容,需要配置
+ 'cert_private' => '', // 商户密钥内容,需要配置
+ 'mp_cert_serial' => '', // 平台证书序号,无需配置
+ 'mp_cert_content' => '', // 平台证书内容,无需配置
+ ];
+
+ /**
+ * BasicWePayV3 constructor.
+ * @param array $options [mch_id, mch_v3_key, cert_public, cert_private]
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function __construct(array $options = [])
+ {
+ if (empty($options['mch_id'])) {
+ throw new InvalidArgumentException("Missing Config -- [mch_id]");
+ }
+ if (empty($options['mch_v3_key'])) {
+ throw new InvalidArgumentException("Missing Config -- [mch_v3_key]");
+ }
+ if (empty($options['cert_public'])) {
+ throw new InvalidArgumentException("Missing Config -- [cert_public]");
+ }
+ if (empty($options['cert_private'])) {
+ throw new InvalidArgumentException("Missing Config -- [cert_private]");
+ }
+
+ if (stripos($options['cert_public'], '-----BEGIN CERTIFICATE-----') === false) {
+ if (file_exists($options['cert_public'])) {
+ $options['cert_public'] = file_get_contents($options['cert_public']);
+ } else {
+ throw new InvalidArgumentException("File Non-Existent -- [cert_public]");
+ }
+ }
+
+ if (stripos($options['cert_private'], '-----BEGIN PRIVATE KEY-----') === false) {
+ if (file_exists($options['cert_private'])) {
+ $options['cert_private'] = file_get_contents($options['cert_private']);
+ } else {
+ throw new InvalidArgumentException("File Non-Existent -- [cert_private]");
+ }
+ }
+
+ $this->config['appid'] = isset($options['appid']) ? $options['appid'] : '';
+ $this->config['mch_id'] = $options['mch_id'];
+ $this->config['mch_v3_key'] = $options['mch_v3_key'];
+ $this->config['cert_public'] = $options['cert_public'];
+ $this->config['cert_private'] = $options['cert_private'];
+ if (empty($options['cert_serial'])) {
+ $this->config['cert_serial'] = openssl_x509_parse($this->config['cert_public'], true)['serialNumberHex'];
+ } else {
+ $this->config['cert_serial'] = $options['cert_serial'];
+ }
+ if (empty($this->config['cert_serial'])) {
+ throw new InvalidArgumentException('Failed to parse certificate public key');
+ }
+
+ if (!empty($options['cache_path'])) {
+ Tools::$cache_path = $options['cache_path'];
+ }
+
+ // 自动配置平台证书
+ if ($this->autoCert) {
+ $this->_autoCert();
+ }
+
+ // 服务商参数支持
+// if (!empty($options['sp_appid'])) {
+// $this->config['sp_appid'] = $options['sp_appid'];
+// }
+// if (!empty($options['sp_mchid'])) {
+// $this->config['sp_mchid'] = $options['sp_mchid'];
+// }
+// if (!empty($options['sub_appid'])) {
+// $this->config['sub_appid'] = $options['sub_appid'];
+// }
+// if (!empty($options['sub_mch_id'])) {
+// $this->config['sub_mch_id'] = $options['sub_mch_id'];
+// }
+ }
+
+ /**
+ * 静态创建对象
+ * @param array $config
+ * @return static
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public static function instance($config)
+ {
+ $key = md5(get_called_class() . serialize($config));
+ if (isset(self::$cache[$key])) return self::$cache[$key];
+ return self::$cache[$key] = new static($config);
+ }
+
+ /**
+ * 模拟发起请求
+ * @param string $method 请求访问
+ * @param string $pathinfo 请求路由
+ * @param string $jsondata 请求数据
+ * @param boolean $verify 是否验证
+ * @param boolean $isjson 返回JSON
+ * @return array|string
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ */
+ public function doRequest($method, $pathinfo, $jsondata = '', $verify = false, $isjson = true)
+ {
+ list($time, $nonce) = [time(), uniqid() . rand(1000, 9999)];
+ $signstr = join("\n", [$method, $pathinfo, $time, $nonce, $jsondata, '']);
+
+ // 生成数据签名TOKEN
+ $token = sprintf('mchid="%s",nonce_str="%s",timestamp="%d",serial_no="%s",signature="%s"',
+ $this->config['mch_id'], $nonce, $time, $this->config['cert_serial'], $this->signBuild($signstr)
+ );
+ $location = (preg_match('|^https?://|', $pathinfo) ? '' : $this->base) . $pathinfo;
+ list($header, $content) = $this->_doRequestCurl($method, $location, [
+ 'data' => $jsondata, 'header' => [
+ 'Accept: application/json',
+ 'Content-Type: application/json',
+ 'User-Agent: https://thinkadmin.top',
+ "Authorization: WECHATPAY2-SHA256-RSA2048 {$token}",
+ "Wechatpay-Serial: {$this->config['mp_cert_serial']}"
+ ],
+ ]);
+
+ if ($verify) {
+ $headers = [];
+ foreach (explode("\n", $header) as $line) {
+ if (stripos($line, 'Wechatpay') !== false) {
+ list($name, $value) = explode(':', $line);
+ list(, $keys) = explode('wechatpay-', strtolower($name));
+ $headers[$keys] = trim($value);
+ }
+ }
+ try {
+ if (empty($headers)) {
+ return $isjson ? json_decode($content, true) : $content;
+ }
+ $string = join("\n", [$headers['timestamp'], $headers['nonce'], $content, '']);
+ if (!$this->signVerify($string, $headers['signature'], $headers['serial'])) {
+ throw new InvalidResponseException('验证响应签名失败');
+ }
+ } catch (\Exception $exception) {
+ throw new InvalidResponseException($exception->getMessage(), $exception->getCode());
+ }
+ }
+
+ return $isjson ? json_decode($content, true) : $content;
+ }
+
+ /**
+ * 通过CURL模拟网络请求
+ * @param string $method 请求方法
+ * @param string $location 请求方法
+ * @param array $options 请求参数 [data, header]
+ * @return array [header,content]
+ */
+ private function _doRequestCurl($method, $location, $options = [])
+ {
+ $curl = curl_init();
+ // POST数据设置
+ if (strtolower($method) === 'post') {
+ curl_setopt($curl, CURLOPT_POST, true);
+ curl_setopt($curl, CURLOPT_POSTFIELDS, $options['data']);
+ }
+ // CURL头信息设置
+ if (!empty($options['header'])) {
+ curl_setopt($curl, CURLOPT_HTTPHEADER, $options['header']);
+ }
+ curl_setopt($curl, CURLOPT_URL, $location);
+ curl_setopt($curl, CURLOPT_HEADER, true);
+ curl_setopt($curl, CURLOPT_TIMEOUT, 60);
+ curl_setopt($curl, CURLOPT_RETURNTRANSFER, true);
+ curl_setopt($curl, CURLOPT_SSL_VERIFYPEER, false);
+ curl_setopt($curl, CURLOPT_SSL_VERIFYHOST, false);
+ $content = curl_exec($curl);
+ $headerSize = curl_getinfo($curl, CURLINFO_HEADER_SIZE);
+ curl_close($curl);
+ return [substr($content, 0, $headerSize), substr($content, $headerSize)];
+ }
+
+ /**
+ * 生成数据签名
+ * @param string $data 签名内容
+ * @return string
+ */
+ protected function signBuild($data)
+ {
+ $pkeyid = openssl_pkey_get_private($this->config['cert_private']);
+ openssl_sign($data, $signature, $pkeyid, 'sha256WithRSAEncryption');
+ return base64_encode($signature);
+ }
+
+ /**
+ * 验证内容签名
+ * @param string $data 签名内容
+ * @param string $sign 原签名值
+ * @param string $serial 证书序号
+ * @return int
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ protected function signVerify($data, $sign, $serial)
+ {
+ $cert = $this->_getCert($serial);
+ return @openssl_verify($data, base64_decode($sign), openssl_x509_read($cert), 'sha256WithRSAEncryption');
+ }
+
+ /**
+ * 获取平台证书
+ * @param string $serial
+ * @return string
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ protected function _getCert($serial = '')
+ {
+ $certs = $this->tmpFile("{$this->config['mch_id']}_certs");
+ if (empty($certs) || empty($certs[$serial]['content'])) {
+ Cert::instance($this->config)->download();
+ $certs = $this->tmpFile("{$this->config['mch_id']}_certs");
+ }
+ if (empty($certs[$serial]['content']) || $certs[$serial]['expire'] < time()) {
+ throw new InvalidResponseException("读取平台证书失败!");
+ } else {
+ return $certs[$serial]['content'];
+ }
+ }
+
+ /**
+ * 自动配置平台证书
+ * @return void
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ protected function _autoCert()
+ {
+ $certs = $this->tmpFile("{$this->config['mch_id']}_certs");
+ if (is_array($certs)) foreach ($certs as $k => $v) {
+ if ($v['expire'] < time()) unset($certs[$k]);
+ }
+ if (empty($certs)) {
+ Cert::instance($this->config)->download();
+ $certs = $this->tmpFile("{$this->config['mch_id']}_certs");
+ }
+ if (empty($certs) || !is_array($certs)) {
+ throw new InvalidResponseException("读取平台证书失败!");
+ }
+ foreach ($certs as $k => $v) if ($v['expire'] > time() + 10) {
+ $this->config['mp_cert_serial'] = $k;
+ $this->config['mp_cert_content'] = $v['content'];
+ break;
+ }
+ if (empty($this->config['mp_cert_serial']) || empty($this->config['mp_cert_content'])) {
+ throw new InvalidResponseException("自动配置平台证书失败!");
+ }
+ }
+
+ /**
+ * 写入或读取临时文件
+ * @param string $name
+ * @param null|array|string $content
+ * @param integer $expire
+ * @return array|string
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ protected function tmpFile($name, $content = null, $expire = 7200)
+ {
+ if (is_null($content)) {
+ $text = Tools::getCache($name);
+ if (empty($text)) return '';
+ $json = json_decode(Tools::getCache($name) ?: '', true);
+ return isset($json[0]) ? $json[0] : '';
+ } else {
+ return Tools::setCache($name, json_encode([$content], JSON_UNESCAPED_UNICODE), $expire);
+ }
+ }
+
+ /**
+ * RSA加密处理-平台证书
+ * @param string $string
+ * @return string
+ * @throws \WeChat\Exceptions\InvalidDecryptException
+ */
+ protected function rsaEncode($string)
+ {
+ $publicKey = $this->config['mp_cert_content'];
+ if (openssl_public_encrypt($string, $encrypted, $publicKey, OPENSSL_PKCS1_OAEP_PADDING)) {
+ return base64_encode($encrypted);
+ } else {
+ throw new InvalidDecryptException('Rsa Encrypt Error.');
+ }
+ }
+}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WePayV3/Contracts/DecryptAes.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WePayV3/Contracts/DecryptAes.php
new file mode 100644
index 00000000000..f5c232cd428
--- /dev/null
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WePayV3/Contracts/DecryptAes.php
@@ -0,0 +1,83 @@
+aesKey = $aesKey;
+ }
+
+ /**
+ * Decrypt AEAD_AES_256_GCM ciphertext
+ * @param string $associatedData AES GCM additional authentication data
+ * @param string $nonceStr AES GCM nonce
+ * @param string $ciphertext AES GCM cipher text
+ * @return string|bool Decrypted string on success or FALSE on failure
+ * @throws \WeChat\Exceptions\InvalidDecryptException
+ */
+ public function decryptToString($associatedData, $nonceStr, $ciphertext)
+ {
+ $ciphertext = \base64_decode($ciphertext);
+ if (strlen($ciphertext) <= self::AUTH_TAG_LENGTH_BYTE) {
+ return false;
+ }
+ try {
+ // ext-sodium (default installed on >= PHP 7.2)
+ if (function_exists('\sodium_crypto_aead_aes256gcm_is_available') && \sodium_crypto_aead_aes256gcm_is_available()) {
+ return \sodium_crypto_aead_aes256gcm_decrypt($ciphertext, $associatedData, $nonceStr, $this->aesKey);
+ }
+ // ext-libsodium (need install libsodium-php 1.x via pecl)
+ if (function_exists('\Sodium\crypto_aead_aes256gcm_is_available') && \Sodium\crypto_aead_aes256gcm_is_available()) {
+ return \Sodium\crypto_aead_aes256gcm_decrypt($ciphertext, $associatedData, $nonceStr, $this->aesKey);
+ }
+ // openssl (PHP >= 7.1 support AEAD)
+ if (PHP_VERSION_ID >= 70100 && in_array('aes-256-gcm', \openssl_get_cipher_methods())) {
+ $ctext = substr($ciphertext, 0, -self::AUTH_TAG_LENGTH_BYTE);
+ $authTag = substr($ciphertext, -self::AUTH_TAG_LENGTH_BYTE);
+ return \openssl_decrypt($ctext, 'aes-256-gcm', $this->aesKey, \OPENSSL_RAW_DATA, $nonceStr, $authTag, $associatedData);
+ }
+ } catch (\Exception $exception) {
+ throw new InvalidDecryptException($exception->getMessage(), $exception->getCode());
+ } catch (\SodiumException $exception) {
+ throw new InvalidDecryptException($exception->getMessage(), $exception->getCode());
+ }
+ throw new InvalidDecryptException('AEAD_AES_256_GCM 需要 PHP 7.1 以上或者安装 libsodium-php');
+ }
+}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WePayV3/Order.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WePayV3/Order.php
new file mode 100644
index 00000000000..f377aa04967
--- /dev/null
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WePayV3/Order.php
@@ -0,0 +1,202 @@
+ '/v3/pay/transactions/h5',
+ 'app' => '/v3/pay/transactions/app',
+ 'jsapi' => '/v3/pay/transactions/jsapi',
+ 'native' => '/v3/pay/transactions/native',
+ ];
+ if (empty($types[$type])) {
+ throw new InvalidArgumentException("Payment {$type} not defined.");
+ } else {
+ // 创建预支付码
+ $result = $this->doRequest('POST', $types[$type], json_encode($data, JSON_UNESCAPED_UNICODE), true);
+ if (empty($result['h5_url']) && empty($result['code_url']) && empty($result['prepay_id'])) {
+ $message = isset($result['code']) ? "[ {$result['code']} ] " : '';
+ $message .= isset($result['message']) ? $result['message'] : json_encode($result, JSON_UNESCAPED_UNICODE);
+ throw new InvalidResponseException($message);
+ }
+ // 支付参数签名
+ $time = strval(time());
+ $appid = $this->config['appid'];
+ $nonceStr = Tools::createNoncestr();
+ if ($type === 'app') {
+ $sign = $this->signBuild(join("\n", [$appid, $time, $nonceStr, $result['prepay_id'], '']));
+ return ['partnerId' => $this->config['mch_id'], 'prepayId' => $result['prepay_id'], 'package' => 'Sign=WXPay', 'nonceStr' => $nonceStr, 'timeStamp' => $time, 'sign' => $sign];
+ } elseif ($type === 'jsapi') {
+ $sign = $this->signBuild(join("\n", [$appid, $time, $nonceStr, "prepay_id={$result['prepay_id']}", '']));
+ return ['appId' => $appid, 'timestamp' => $time, 'timeStamp' => $time, 'nonceStr' => $nonceStr, 'package' => "prepay_id={$result['prepay_id']}", 'signType' => 'RSA', 'paySign' => $sign];
+ } else {
+ return $result;
+ }
+ }
+ }
+
+ /**
+ * 支付订单查询
+ * @param string $tradeNo 订单单号
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @document https://pay.weixin.qq.com/wiki/doc/apiv3/apis/chapter3_1_2.shtml
+ */
+ public function query($tradeNo)
+ {
+ $pathinfo = "/v3/pay/transactions/out-trade-no/{$tradeNo}";
+ return $this->doRequest('GET', "{$pathinfo}?mchid={$this->config['mch_id']}", '', true);
+ }
+
+ /**
+ * 关闭支付订单
+ * @param string $tradeNo 订单单号
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ */
+ public function close($tradeNo)
+ {
+ $data = ['mchid' => $this->config['mch_id']];
+ $path = "/v3/pay/transactions/out-trade-no/{$tradeNo}/close";
+ return $this->doRequest('POST', $path, json_encode($data, JSON_UNESCAPED_UNICODE), true);
+ }
+
+ /**
+ * 支付通知解析
+ * @param array $data
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidDecryptException
+ */
+ public function notify(array $data = [])
+ {
+ if (empty($data)) {
+ $data = json_decode(Tools::getRawInput(), true);
+ }
+ if (isset($data['resource'])) {
+ $aes = new DecryptAes($this->config['mch_v3_key']);
+ $data['result'] = $aes->decryptToString(
+ $data['resource']['associated_data'],
+ $data['resource']['nonce'],
+ $data['resource']['ciphertext']
+ );
+ }
+ return $data;
+ }
+
+ /**
+ * 创建退款订单
+ * @param array $data 退款参数
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @document https://pay.weixin.qq.com/wiki/doc/apiv3/apis/chapter3_1_9.shtml
+ */
+ public function createRefund($data)
+ {
+ $path = '/v3/refund/domestic/refunds';
+ return $this->doRequest('POST', $path, json_encode($data, JSON_UNESCAPED_UNICODE), true);
+ }
+
+ /**
+ * 退款订单查询
+ * @param string $refundNo 退款单号
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @document https://pay.weixin.qq.com/wiki/doc/apiv3/apis/chapter3_1_10.shtml
+ */
+ public function queryRefund($refundNo)
+ {
+ $path = "/v3/refund/domestic/refunds/{$refundNo}";
+ return $this->doRequest('GET', $path, '', true);
+ }
+
+ /**
+ * 获取退款通知
+ * @param mixed $data
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidDecryptException
+ * @deprecated 直接使用 Notify 方法
+ */
+ public function notifyRefund($data = [])
+ {
+ return $this->notify($data);
+ }
+
+ /**
+ * 申请交易账单
+ * @param array|string $params
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @document https://pay.weixin.qq.com/wiki/doc/apiv3/apis/chapter3_3_6.shtml
+ */
+ public function tradeBill($params)
+ {
+ $path = '/v3/bill/tradebill?' . is_array($params) ? http_build_query($params) : $params;
+ return $this->doRequest('GET', $path, '', true);
+ }
+
+ /**
+ * 申请资金账单
+ * @param array|string $params
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @document https://pay.weixin.qq.com/wiki/doc/apiv3/apis/chapter3_3_7.shtml
+ */
+ public function fundflowBill($params)
+ {
+ $path = '/v3/bill/fundflowbill?' . is_array($params) ? http_build_query($params) : $params;
+ return $this->doRequest('GET', $path, '', true);
+ }
+
+ /**
+ * 下载账单文件
+ * @param string $fileurl
+ * @return string 二进制 Excel 内容
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @document https://pay.weixin.qq.com/wiki/doc/apiv3_partner/apis/chapter7_6_1.shtml
+ */
+ public function downloadBill($fileurl)
+ {
+ return $this->doRequest('GET', $fileurl, '', false, false);
+ }
+}
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WePayV3/ProfitSharing.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WePayV3/ProfitSharing.php
new file mode 100644
index 00000000000..81b5cf5e3f7
--- /dev/null
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WePayV3/ProfitSharing.php
@@ -0,0 +1,100 @@
+config['appid'];
+ return $this->doRequest('POST', '/v3/profitsharing/orders', json_encode($options, JSON_UNESCAPED_UNICODE), true);
+ }
+
+
+ /**
+ * 查询分账结果
+ * @param string $outOrderNo 商户分账单号
+ * @param string $transactionId 微信订单号
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ */
+ public function query($outOrderNo, $transactionId)
+ {
+ $pathinfo = "/v3/profitsharing/orders/{$outOrderNo}?&transaction_id={$transactionId}";
+ return $this->doRequest('GET', $pathinfo, '', true);
+ }
+
+ /**
+ * 解冻剩余资金
+ * @param array $options
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ */
+ public function unfreeze($options)
+ {
+ return $this->doRequest('POST', '/v3/profitsharing/orders/unfreeze', json_encode($options, JSON_UNESCAPED_UNICODE), true);
+ }
+
+ /**
+ * 查询剩余待分金额
+ * @param string $transactionId 微信订单号
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ */
+ public function amounts($transactionId)
+ {
+ $pathinfo = "/v3/profitsharing/transactions/{$transactionId}/amounts";
+ return $this->doRequest('GET', $pathinfo, '', true);
+ }
+
+ /**
+ * 添加分账接收方
+ * @param array $options
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ */
+ public function addReceiver($options)
+ {
+ $options['appid'] = $this->config['appid'];
+ return $this->doRequest('POST', "/v3/profitsharing/receivers/add", json_encode($options, JSON_UNESCAPED_UNICODE), true);
+ }
+
+ /**
+ * 删除分账接收方
+ * @param array $options
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ */
+ public function deleteReceiver($options)
+ {
+ $options['appid'] = $this->config['appid'];
+ return $this->doRequest('POST', "/v3/profitsharing/receivers/delete", json_encode($options, JSON_UNESCAPED_UNICODE), true);
+ }
+}
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WePayV3/Refund.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WePayV3/Refund.php
new file mode 100644
index 00000000000..eaf06966156
--- /dev/null
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WePayV3/Refund.php
@@ -0,0 +1,82 @@
+config)->createRefund($data);
+ // return $this->doRequest('POST', '/v3/ecommerce/refunds/apply', json_encode($data, JSON_UNESCAPED_UNICODE), true);
+ }
+
+ /**
+ * 退款订单查询
+ * @param string $refundNo 退款单号
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function query($refundNo)
+ {
+ return Order::instance($this->config)->queryRefund($refundNo);
+ // $pathinfo = "/v3/ecommerce/refunds/out-refund-no/{$refundNo}";
+ // return $this->doRequest('GET', "{$pathinfo}?sub_mchid={$this->config['mch_id']}", '', true);
+ }
+
+ /**
+ * 获取退款通知
+ * @param mixed $xml
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidDecryptException
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ * @throws \WeChat\Exceptions\LocalCacheException
+ */
+ public function notify($xml = [])
+ {
+ return Order::instance($this->config)->notify($xml);
+// $data = Tools::xml2arr(empty($xml) ? Tools::getRawInput() : $xml);
+// if (!isset($data['return_code']) || $data['return_code'] !== 'SUCCESS') {
+// throw new InvalidResponseException('获取退款通知XML失败!');
+// }
+// try {
+// $key = md5($this->config['mch_v3_key']);
+// $decrypt = base64_decode($data['req_info']);
+// $response = openssl_decrypt($decrypt, 'aes-256-ecb', $key, OPENSSL_RAW_DATA);
+// $data['result'] = Tools::xml2arr($response);
+// return $data;
+// } catch (\Exception $exception) {
+// throw new InvalidDecryptException($exception->getMessage(), $exception->getCode());
+// }
+ }
+}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/WePayV3/Transfers.php b/upload/system/storage/vendor/zoujingli/wechat-developer/WePayV3/Transfers.php
new file mode 100644
index 00000000000..78ba5a683ba
--- /dev/null
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/WePayV3/Transfers.php
@@ -0,0 +1,104 @@
+config['appid'];
+ }
+ if (isset($body['transfer_detail_list']) && is_array($body['transfer_detail_list'])) {
+ foreach ($body['transfer_detail_list'] as &$item) if (isset($item['user_name'])) {
+ $item['user_name'] = $this->rsaEncode($item['user_name']);
+ }
+ }
+ if (empty($body['total_num'])) {
+ $body['total_num'] = count($body['transfer_detail_list']);
+ }
+ return $this->doRequest('POST', '/v3/transfer/batches', json_encode($body, JSON_UNESCAPED_UNICODE), true);
+ }
+
+ /**
+ * 通过微信批次单号查询批次单
+ * @param string $batchId 微信批次单号(二选一)
+ * @param string $outBatchNo 商家批次单号(二选一)
+ * @param boolean $needQueryDetail 查询指定状态
+ * @param integer $offset 请求资源的起始位置
+ * @param integer $limit 最大明细条数
+ * @param string $detailStatus 查询指定状态
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ */
+ public function query($batchId = '', $outBatchNo = '', $needQueryDetail = true, $offset = 0, $limit = 20, $detailStatus = 'ALL')
+ {
+ if (empty($batchId)) {
+ $pathinfo = "/v3/transfer/batches/out-batch-no/{$outBatchNo}";
+ } else {
+ $pathinfo = "/v3/transfer/batches/batch-id/{$batchId}";
+ }
+ $params = http_build_query([
+ 'limit' => $limit,
+ 'offset' => $offset,
+ 'detail_status' => $detailStatus,
+ 'need_query_detail' => $needQueryDetail ? 'true' : 'false',
+ ]);
+ return $this->doRequest('GET', "{$pathinfo}?{$params}", '', true);
+ }
+
+ /**
+ * 通过微信明细单号查询明细单
+ * @param string $batchId 微信批次单号
+ * @param string $detailId 微信支付系统内部区分转账批次单下不同转账明细单的唯一标识
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ */
+ public function detailBatchId($batchId, $detailId)
+ {
+ $pathinfo = "/v3/transfer/batches/batch-id/{$batchId}/details/detail-id/{$detailId}";
+ return $this->doRequest('GET', $pathinfo, '', true);
+ }
+
+ /**
+ * 通过商家明细单号查询明细单
+ * @param string $outBatchNo 商户系统内部的商家批次单号,在商户系统内部唯一
+ * @param string $outDetailNo 商户系统内部区分转账批次单下不同转账明细单的唯一标识
+ * @return array
+ * @throws \WeChat\Exceptions\InvalidResponseException
+ */
+ public function detailOutBatchNo($outBatchNo, $outDetailNo)
+ {
+ $pathinfo = "/v3/transfer/batches/out-batch-no/{$outBatchNo}/details/out-detail-no/{$outDetailNo}";
+ return $this->doRequest('GET', $pathinfo, '', true);
+ }
+}
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay-app.php b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay-app.php
index 0070eb31c48..82e7942e431 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay-app.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay-app.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
// 1. 手动加载入口文件
@@ -26,12 +28,17 @@
// 请参考(请求参数):https://docs.open.alipay.com/api_1/alipay.trade.app.pay
$result = $pay->apply([
- 'out_trade_no' => time(), // 商户订单号
+ 'out_trade_no' => strval(time()), // 商户订单号
'total_amount' => '1', // 支付金额
- 'subject' => 'test subject', // 支付订单描述
+ 'subject' => '支付宝订单标题', // 支付订单描述
]);
- echo '';
- var_export($result);
+ echo $result . PHP_EOL .'
'. PHP_EOL;
+
+ // 请求关闭订单
+ $result = $pay->close([
+ 'out_trade_no' => strval(time())
+ ]);
+ echo PHP_EOL . PHP_EOL . $result;
} catch (\Exception $e) {
echo $e->getMessage();
}
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay-bill.php b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay-bill.php
index af5c493cf6c..8f9a25bc328 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay-bill.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay-bill.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
// 1. 手动加载入口文件
@@ -24,10 +26,19 @@
// $pay = \We::AliPayBill($config);
$pay = \AliPay\Bill::instance($config);
+ /**
+ * 账单类型,商户通过接口或商户经开放平台授权后其所属服务商通过接口可以获取以下账单类型,支持:
+ * trade:商户基于支付宝交易收单的业务账单;
+ * signcustomer:基于商户支付宝余额收入及支出等资金变动的账务账单;
+ * merchant_act:营销活动账单,包含营销活动的发放,核销记录
+ * trade_zft_merchant:直付通二级商户查询交易的业务账单;
+ * zft_acc:直付通平台商查询二级商户流水使用,返回所有二级商户流水。
+ */
+
// 请参考(请求参数):https://docs.open.alipay.com/api_15/alipay.data.dataservice.bill.downloadurl.query
$result = $pay->apply([
- 'bill_date' => '2018-10-03', // 账单时间(日账单yyyy-MM-dd,月账单 yyyy-MM)
- 'bill_type' => 'signcustomer', // 账单类型(trade指商户基于支付宝交易收单的业务账单,signcustomer是指基于商户支付宝余额收入及支出等资金变动的帐务账单)
+ 'bill_date' => date('Y-m-d', strtotime('-1 month')), // 账单时间(日账单yyyy-MM-dd,月账单 yyyy-MM)
+ 'bill_type' => 'trade',
]);
echo '';
var_export($result);
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay-notify.php b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay-notify.php
index 0d5911682bb..c400b4e5b56 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay-notify.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay-notify.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
// 1. 手动加载入口文件
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay-pos.php b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay-pos.php
index e55c4e2e085..d5823e04b50 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay-pos.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay-pos.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
// 1. 手动加载入口文件
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay-refund.php b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay-refund.php
index 487ca7b27dc..ca5b4d470d0 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay-refund.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay-refund.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
// 1. 手动加载入口文件
@@ -31,7 +33,7 @@
// 参考链接:https://docs.open.alipay.com/api_1/alipay.trade.refund
$result = $pay->refund($out_trade_no, $refund_fee);
-
+
echo '';
var_export($result);
} catch (Exception $e) {
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay-scan.php b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay-scan.php
index 2bc880632c2..62cbd714ad0 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay-scan.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay-scan.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
// 1. 手动加载入口文件
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay-transfer-account.php b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay-transfer-account.php
index 1d2b289e416..a77f7c4a471 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay-transfer-account.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay-transfer-account.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
// 1. 手动加载入口文件
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay-transfer-create.php b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay-transfer-create.php
index 39d0fc42975..987c5464ca1 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay-transfer-create.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay-transfer-create.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
// 1. 手动加载入口文件
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay-transfer-query.php b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay-transfer-query.php
index 7e7d99feab2..c6942c2e1a3 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay-transfer-query.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay-transfer-query.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
// 1. 手动加载入口文件
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay-transfer.php b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay-transfer.php
index 4eaf6fb1f6f..11283191dbb 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay-transfer.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay-transfer.php
@@ -3,20 +3,22 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
// 1. 手动加载入口文件
include "../include.php";
// 2. 准备公众号配置参数
-$config = include "./alipay.php";
+$config = include "./alipay2.php";
try {
// 实例支付对象
@@ -28,10 +30,10 @@
$result = $pay->apply([
'out_biz_no' => time(), // 订单号
'payee_type' => 'ALIPAY_LOGONID', // 收款方账户类型(ALIPAY_LOGONID | ALIPAY_USERID)
- 'payee_account' => 'demo@sandbox.com', // 收款方账户
+ 'payee_account' => 'yvvfcr3065@sandbox.com', // 收款方账户
'amount' => '10', // 转账金额
'payer_show_name' => '未寒', // 付款方姓名
- 'payee_real_name' => '张三', // 收款方真实姓名
+ 'payee_real_name' => 'yvvfcr3065', // 收款方真实姓名
'remark' => '张三', // 转账备注
]);
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay-wap.php b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay-wap.php
index ac04021d2f0..8ddae77aa92 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay-wap.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay-wap.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
// 1. 手动加载入口文件
@@ -18,8 +20,8 @@
// 2. 准备公众号配置参数
$config = include "./alipay.php";
// 参考公共参数 https://docs.open.alipay.com/203/107090/
-$config['notify_url'] = 'http://pay.thinkadmin.top/test/alipay-notify.php';
-$config['return_url'] = 'http://pay.thinkadmin.top/test/alipay-success.php';
+$config['notify_url'] = 'https://pay.thinkadmin.top/test/alipay-notify.php';
+$config['return_url'] = 'https://pay.thinkadmin.top/test/alipay-success.php';
try {
// 实例支付对象
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay-web.php b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay-web.php
index 46f0efcf07b..c3a3497b9b6 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay-web.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay-web.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
// 1. 手动加载入口文件
@@ -19,8 +21,8 @@
$config = include "./alipay.php";
// 参考公共参数 https://docs.open.alipay.com/203/107090/
-$config['notify_url'] = 'http://pay.thinkadmin.top/test/alipay-notify.php';
-$config['return_url'] = 'http://pay.thinkadmin.top/test/alipay-success.php';
+$config['notify_url'] = 'https://pay.thinkadmin.top/test/alipay-notify.php';
+$config['return_url'] = 'https://pay.thinkadmin.top/test/alipay-success.php';
try {
// 实例支付对象
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay.php b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay.php
index 25a7379d35d..54bf89c3a62 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay.php
@@ -3,28 +3,56 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
+/**
+ * 【 名词解释 】
+ * 应用私钥:用来给应用消息进行签名,请务必要妥善保管,避免遗失或泄露。
+ * 应用公钥:需要提供给支付宝开放平台,平台会对应用发送的消息进行签名验证。
+ * 支付宝公钥:应用收到支付宝发送的同步、异步消息时,使用支付宝公钥验证签名信息。
+ * CSR 文件:CSR 即证书签名请求(Certificate Signing Request),CSR 文件(.csr)是申请证书时所需要的一个数据文件。
+ * 应用公钥证书:在开放平台上传 CSR 文件后可以获取 CA 机构颁发的应用证书文件(.crt),其中包含了组织/公司名称、应用公钥、证书有效期等内容,一般有效期为 5 年。
+ * 支付宝公钥证书:用来验证支付宝消息,包含了支付宝公钥、支付宝公司名称、证书有效期等内容,一般有效期为 5 年。
+ * 支付宝根证书:用来验证支付宝消息,包含了根 CA 名称、根 CA 的公钥、证书有效期等内容。
+ */
+
+/**
+ * 应用公钥证书SN(app_cert_sn)和支付宝根证书SN(alipay_root_cert_sn)的 sn 是指什么?
+ * 使用公钥证书签名方式下, 请求参数中需要携带应用公钥证书SN(app_cert_sn)、支付宝根证书SN(alipay_root_cert_sn),这里的SN是指基于开放平台提供的计算规则,动态计算出来的公钥证书序列号,与X.509证书中内置的序列号(serialNumber)不同。
+ * 具体的计算规则如下:解析X.509证书文件,获取证书签发机构名称(name)以及证书内置序列号(serialNumber)。 将name与serialNumber拼接成字符串,再对该字符串做MD5计算。
+ * 可以参考开放平台SDK源码中的 AlipaySignature.getCertSN 方法实现
+ *
+ * 不直接使用证书文件中内置的序列号原因:开放平台支持开发者上传自己找第三方权威CA签发的证书,而证书文件中内置序列号只能保证同一家签发机构签发的证书不重复
+ */
+
return [
// 沙箱模式
- 'debug' => true,
- // 签名类型(RSA|RSA2)
- 'sign_type' => "RSA2",
+ 'debug' => true,
+ // 签名类型 ( RSA|RSA2 )
+ 'sign_type' => 'RSA2',
// 应用ID
- 'appid' => '2016090900468879',
- // 支付宝公钥(1行填写,特别注意,这里是支付宝公钥,不是应用公钥,最好从开发者中心的网页上去复制)
- 'public_key' => 'MIIBIjANBgkqhkiG9w0BAQEFAAOCAQ8AMIIBCgKCAQEAtU71NY53UDGY7JNvLYAhsNa+taTF6KthIHJmGgdio9bkqeJGhHk6ttkTKkLqFgwIfgAkHpdKiOv1uZw6gVGZ7TCu5LfHTqKrCd6Uz+N7hxhY+4IwicLgprcV1flXQLmbkJYzFMZqkXGkSgOsR2yXh4LyQZczgk9N456uuzGtRy7MoB4zQy34PLUkkxR6W1B2ftNbLRGXv6tc7p/cmDcrY6K1bSxnGmfRxFSb8lRfhe0V0UM6pKq2SGGSeovrKHN0OLp+Nn5wcULVnFgATXGCENshRlp96piPEBFwneXs19n+sX1jx60FTR7/rME3sW3AHug0fhZ9mSqW4x401WjdnwIDAQAB',
- // 支付宝私钥(1行填写)
- 'private_key' => 'MIIEvQIBADANBgkqhkiG9w0BAQEFAASCBKcwggSjAgEAAoIBAQC3pbN7esinxgjE8uxXAsccgGNKIq+PR1LteNTFOy0fsete43ObQCrzd9DO0zaUeBUzpIOnxrKxez7QoZROZMYrinttFZ/V5rbObEM9E5AR5Tv/Fr4IBywoS8ZtN16Xb+fZmibfU91yq9O2RYSvscncU2qEYmmaTenM0QlUO80ZKqPsM5JkgCNdcYZTUeHclWeyER3dSImNtlSKiSBSSTHthb11fkudjzdiUXua0NKVWyYuAOoDMcpXbD6NJmYqEA/iZ/AxtQt08pv0Mow581GPB0Uop5+qA2hCV85DpagE94a067sKcRui0rtkJzHem9k7xVL+2RoFm1fv3RnUkMwhAgMBAAECggEAAetkddzxrfc+7jgPylUIGb8pyoOUTC4Vqs/BgZI9xYAJksNT2QKRsFvHPfItNt4Ocqy8h4tnIL3GCU43C564B4p6AcjhE85GiN/O0BudPOKlfuQQ9mqExqMMHuYeQfz0cmzPDTSGMwWiv9v4KBH2pyvkCCAzNF6uG+rvawb4/NNVuiI7C8Ku/wYsamtbgjMZVOFFdScYgIw1BgA99RUU/fWBLMnTQkoyowSRb9eSmEUHjt/WQt+/QgKAT2WmuX4RhaGy0qcQLbNaJNKXdJ+PVhQrSiasINNtqYMa8GsQuuKsk3X8TCg9K6/lowivt5ruhyWcP2sx93zY/LGzIHgHcQKBgQDoZlcs9RWxTdGDdtH8kk0J/r+QtMijNzWI0a+t+ZsWOyd3rw+uM/8O4JTNP4Y98TvvxhJXewITbfiuOIbW1mxh8bnO/fcz7+RXZKgPDeoTeNo717tZFZGBEyUdH9M9Inqvht7+hjVDIMCYBDomYebdk3Xqo4mDBjLRdVNGrhGmVQKBgQDKS/MgTMK8Ktfnu1KzwCbn/FfHTOrp1a1t1wWPv9AW0rJPYeaP6lOkgIoO/1odG9qDDhdB6njqM+mKY5Yr3N94PHamHbwJUCmbkqEunCWpGzgcQZ1Q254xk9D7UKq/XUqW2WDqDq80GQeNial+fBc46yelQzokwdA+JdIFKoyinQKBgQCBems9V/rTAtkk1nFdt6EGXZEbLS3PiXXhGXo4gqV+OEzf6H/i/YMwJb2hsK+5GQrcps0XQihA7PctEb9GOMa/tu5fva0ZmaDtc94SLR1p5d4okyQFGPgtIp594HpPSEN0Qb9BrUJFeRz0VP6U3dzDPGHo7V4yyqRLgIN6EIcy1QKBgAqdh6mHPaTAHspDMyjJiYEc5cJIj/8rPkmIQft0FkhMUB0IRyAALNlyAUyeK61hW8sKvz+vPR8VEEk5xpSQp41YpuU6pDZc5YILZLfca8F+8yfQbZ/jll6Foi694efezl4yE/rUQG9cbOAJfEJt4o4TEOaEK5XoMbRBKc8pl22lAoGARTq0qOr9SStihRAy9a+8wi2WEwL4QHcmOjH7iAuJxy5b5TRDSjlk6h+0dnTItiFlTXdfpO8KhWA8EoSJVBZ1kcACQDFgMIA+VM+yXydtzMotOn21W4stfZ4I6dHFiujMsnKpNYVpQh3oCrJf4SeXiQDdiSCodqb1HlKkEc6naHQ=',
+ 'appid' => '2021000122667306',
+ // 应用私钥内容 ( 需1行填写,特别注意:这里的应用私钥通常由支付宝密钥管理工具生成 )
+ 'private_key' => 'MIIEowIBAAKCAQEAndH26KVe3Iy+8GxVxDuG9ZolYrqGNm8Jpdi9GrQdM81ad4pPyul2NVO+9C2Kr6a6jK6Qw1gyzcwYxtkUC7xoLZUSPpmSH7sH3sD6r2B7Mf5FsrVSa29lcm1+3UkyFgZjYTkx45lfbLmAFHOzOl0WfGkMW0Sq3N/5OMr074E4EnYtALdE3jVQCDf8bzqN3j/Kwe7f10Aglvxili2BrFM564silqcbiJ8U1zDmTdZvmEkP7ia/YVkmt5w3rh7ZBoaubtcM/rVGYXL2hQPwr/pquNCTu7Eh1RcWfpcnbuw+gOnaNyXmNFmZkeNlegXIifcunt1GK6a1pX090R8eFN3LjQIDAQABAoIBACrLY4OETCvL8n6pMbyLU7ZHfTm/UGN0So5xLh4OlxiT56MgmzBvjAE72zzFGKU2tcEuGM0Pnn8Vh+ZruLbR+QHbOV5GMExwX9r0Q0XJCL7uryGdb2L4iu6zaEJC9dTpGIulgbSwwyJtTqC9Gu2Jjm5f4dzhyt8n0KGozzAevwCqI9RaJSD96gGWLbMlHCyWKGy1OdBP4V/+agPyHAGZ9gqpfKY7y4L0My8gUxhWzQWOwihtFACjV66ULhutUYT2bro3j1k9UekKlX7IiWrssPmmmw2vfUbrKiNugF6zkfyStPt7jGJ0CdzAHWe3pyF72TyO5NU2NGcX8eKgYlY2crUCgYEAzOcg5Zot9X+Ao+fYH/Oq/3eGZd6krzByiXfcjuRco7mGODwmUnzt3PpPT1fPry9TxarTajt+A9LuxqWawfQ9eWAfrTGAbtDJB0LYo6CynDqUoRqBukROuNaLQiUqEreOQqt08o6VVblgVLv8475ij8s4z/6C2NSSjgUJHf0PL38CgYEAxS0bcXGI+WtempZ4Q3QMTUmp/+B3zuw9JzSV1gvbVi7MleI9V62V2IXHPSXL5mRhYOuQWR0MnVOhbo69fkEA8HpdSd1q2JjaeS+OiZ0ditcJISQJbqWtvmF2+XtQcbVwfID69GWGxyBEHHTW8AtAzIPc6T7x2izyzBw0lXDHSvMCgYEAi+Y61ckhG/9EC6TeMWKjG+21u5P6CQshCK7nzkAo6DhhZb/bwnI9zaSxxdCEom3D2rA5zMx1y5KXKNYlBcwGtPpmZk/oCsFOoECJvZ6YlIaCuERq0oyU2yrQxgat5T2iSe7a2El1uKPrG6+GiNCSZu8wCQMSv4zTy1ew0+LWHW0CgYAvX7ESRpcEZjmqprBqdH1oLGS9566hdr0SqF2/ucWPJVteP6dBY6F3Dl1aYbRlvIRxBuf9oS8gtbE5oO4CYZfaL2wujRZYyBDlwPlcMvWgIB4/aish/IiMD1rIgkpHp7JJF6w0ABiryyLSO3hQ4ENHX/85wzfUlawYQkaYCSq45QKBgCdqrv58KD8tDYn4JnaHJNE+5TgKK5cNhYLZLAsYz7x1KfPdkiC7y/hnenn3TWkm4xw8Tw1rJ1ZIJ24iZgTCTO7EEsB7jZegvg4z/4zVbSK2Y4VI1lJ7jlyqmwg0ArimXTNZFoy66h9c9t2smG40YEZCmmmTLEqVlWgyR1MU5iM5',
+ // 公钥模式,支付宝公钥内容 ( 需1行填写,特别注意:这里不是应用公钥而是支付宝公钥,通常是上传应用公钥换取支付宝公钥,在网页可以复制 )
+ 'public_key' => '',
+ // 证书模式,应用公钥证书路径 ( 新版资金类接口转 app_cert_sn,如文件 appCertPublicKey.crt )
+ 'app_cert_path' => __DIR__ . '/alipay/appPublicCert.crt', // 'app_cert' => '证书内容',
+ // 证书模式,支付宝根证书路径 ( 新版资金类接口转 alipay_root_cert_sn,如文件 alipayRootCert.crt )
+ 'alipay_root_path' => __DIR__ . '/alipay/alipayRootCert.crt', // 'root_cert' => '证书内容',
+ // 证书模式,支付宝公钥证书路径 ( 未填写 public_key 时启用此参数,如文件 alipayPublicCert.crt )
+ 'alipay_cert_path' => __DIR__ . '/alipay/alipayPublicCert.crt', // 'public_key' => '证书内容'
// 支付成功通知地址
- 'notify_url' => '',
+ 'notify_url' => '',
// 网页支付回跳地址
- 'return_url' => '',
+ 'return_url' => '',
];
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay/alipayPublicCert.crt b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay/alipayPublicCert.crt
new file mode 100644
index 00000000000..0d7d61207b7
--- /dev/null
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay/alipayPublicCert.crt
@@ -0,0 +1,38 @@
+-----BEGIN CERTIFICATE-----
+MIIDszCCApugAwIBAgIQICMFBicVvB1uC0Lc3VFbITANBgkqhkiG9w0BAQsFADCBkTELMAkGA1UE
+BhMCQ04xGzAZBgNVBAoMEkFudCBGaW5hbmNpYWwgdGVzdDElMCMGA1UECwwcQ2VydGlmaWNhdGlv
+biBBdXRob3JpdHkgdGVzdDE+MDwGA1UEAww1QW50IEZpbmFuY2lhbCBDZXJ0aWZpY2F0aW9uIEF1
+dGhvcml0eSBDbGFzcyAyIFIxIHRlc3QwHhcNMjMwNTA2MDYwMTA3WhcNMjQwNTA1MDYwMTA3WjCB
+hDELMAkGA1UEBhMCQ04xHzAdBgNVBAoMFndtaWFrdTY3NDlAc2FuZGJveC5jb20xDzANBgNVBAsM
+BkFsaXBheTFDMEEGA1UEAww65pSv5LuY5a6dKOS4reWbvSnnvZHnu5zmioDmnK/mnInpmZDlhazl
+j7gtMjA4ODcyMTAwMjg0MzQxNDCCASIwDQYJKoZIhvcNAQEBBQADggEPADCCAQoCggEBALkO9F4+
+AB7CLI47hSthHsZFFQxnOiggc4R8pbeb24BkyRc6xJVekYtzuJ8cLSsy1spEX4zguTPc+b7eza5k
+nN1j2pdAfNkzbdEg1Tt4A0b5xbMAfaQtVhwU0aohhLF+i6TTgospMmBwJnN2++Eda6LccrTqS7ff
+x8I2bhkraLlEO4C6pxUcGCyorPLVvRWOTRC/RzxDURHEaGlBPMxxOpeIzYuYNg77OK+Sqp0zb5nk
+U3PO2cpSrOCT4UlrJDmqgSZgwHE0e+9MdBuveLSo1ubG5uGvz8Vjld3hBvVywOAnEuoMBxtSrEbv
+nkz7M0MJBDmQk/D8WSekm2lMGbFDuGUCAwEAAaMSMBAwDgYDVR0PAQH/BAQDAgTwMA0GCSqGSIb3
+DQEBCwUAA4IBAQC29uyA9W6uQivhMqc3YyGXPvi4LIOThU1ijeOSpovHiRUGVfaO/qIY4eAQ2ivF
+iKIrUEqcJnWdNN8LZwWdWd6UmInyngq2i+Pf4h3a2MLkV3ZufZNZkJP5GZJxbcjkKlnKuCTKFUd5
+wJ0zo369E+mPdNGlrPLvNXw4ziUpUb4KXmEn1yOVAkQMsBP43K6QB2QVIODrtp4O+rEs80KHgUQh
+cmla+PWGyX2nuwHURxUtEeIeJblra+ntyy+bTYfCVVG8jh4BN5bPExDprRa100aZGsqyExO1xtxk
+4pu0Jag5XRvGyZpmqH27SfQX2oKcsobuDyGCwff/yvYKOZk53JZD
+-----END CERTIFICATE-----
+-----BEGIN CERTIFICATE-----
+MIIDszCCApugAwIBAgIQIBkIGbgVxq210KxLJ+YA/TANBgkqhkiG9w0BAQsFADCBhDELMAkGA1UE
+BhMCQ04xFjAUBgNVBAoMDUFudCBGaW5hbmNpYWwxJTAjBgNVBAsMHENlcnRpZmljYXRpb24gQXV0
+aG9yaXR5IHRlc3QxNjA0BgNVBAMMLUFudCBGaW5hbmNpYWwgQ2VydGlmaWNhdGlvbiBBdXRob3Jp
+dHkgUjEgdGVzdDAeFw0xOTA4MTkxMTE2MDBaFw0yNDA4MDExMTE2MDBaMIGRMQswCQYDVQQGEwJD
+TjEbMBkGA1UECgwSQW50IEZpbmFuY2lhbCB0ZXN0MSUwIwYDVQQLDBxDZXJ0aWZpY2F0aW9uIEF1
+dGhvcml0eSB0ZXN0MT4wPAYDVQQDDDVBbnQgRmluYW5jaWFsIENlcnRpZmljYXRpb24gQXV0aG9y
+aXR5IENsYXNzIDIgUjEgdGVzdDCCASIwDQYJKoZIhvcNAQEBBQADggEPADCCAQoCggEBAMh4FKYO
+ZyRQHD6eFbPKZeSAnrfjfU7xmS9Yoozuu+iuqZlb6Z0SPLUqqTZAFZejOcmr07ln/pwZxluqplxC
+5+B48End4nclDMlT5HPrDr3W0frs6Xsa2ZNcyil/iKNB5MbGll8LRAxntsKvZZj6vUTMb705gYgm
+VUMILwi/ZxKTQqBtkT/kQQ5y6nOZsj7XI5rYdz6qqOROrpvS/d7iypdHOMIM9Iz9DlL1mrCykbBi
+t25y+gTeXmuisHUwqaRpwtCGK4BayCqxRGbNipe6W73EK9lBrrzNtTr9NaysesT/v+l25JHCL9tG
+wpNr1oWFzk4IHVOg0ORiQ6SUgxZUTYcCAwEAAaMSMBAwDgYDVR0PAQH/BAQDAgTwMA0GCSqGSIb3
+DQEBCwUAA4IBAQBWThEoIaQoBX2YeRY/I8gu6TYnFXtyuCljANnXnM38ft+ikhE5mMNgKmJYLHvT
+yWWWgwHoSAWEuml7EGbE/2AK2h3k0MdfiWLzdmpPCRG/RJHk6UB1pMHPilI+c0MVu16OPpKbg5Vf
+LTv7dsAB40AzKsvyYw88/Ezi1osTXo6QQwda7uefvudirtb8FcQM9R66cJxl3kt1FXbpYwheIm/p
+j1mq64swCoIYu4NrsUYtn6CV542DTQMI5QdXkn+PzUUly8F6kDp+KpMNd0avfWNL5+O++z+F5Szy
+1CPta1D7EQ/eYmMP+mOQ35oifWIoFCpN6qQVBS/Hob1J/UUyg7BW
+-----END CERTIFICATE-----
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay/alipayRootCert.crt b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay/alipayRootCert.crt
new file mode 100644
index 00000000000..76417c538f5
--- /dev/null
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay/alipayRootCert.crt
@@ -0,0 +1,88 @@
+-----BEGIN CERTIFICATE-----
+MIIBszCCAVegAwIBAgIIaeL+wBcKxnswDAYIKoEcz1UBg3UFADAuMQswCQYDVQQG
+EwJDTjEOMAwGA1UECgwFTlJDQUMxDzANBgNVBAMMBlJPT1RDQTAeFw0xMjA3MTQw
+MzExNTlaFw00MjA3MDcwMzExNTlaMC4xCzAJBgNVBAYTAkNOMQ4wDAYDVQQKDAVO
+UkNBQzEPMA0GA1UEAwwGUk9PVENBMFkwEwYHKoZIzj0CAQYIKoEcz1UBgi0DQgAE
+MPCca6pmgcchsTf2UnBeL9rtp4nw+itk1Kzrmbnqo05lUwkwlWK+4OIrtFdAqnRT
+V7Q9v1htkv42TsIutzd126NdMFswHwYDVR0jBBgwFoAUTDKxl9kzG8SmBcHG5Yti
+W/CXdlgwDAYDVR0TBAUwAwEB/zALBgNVHQ8EBAMCAQYwHQYDVR0OBBYEFEwysZfZ
+MxvEpgXBxuWLYlvwl3ZYMAwGCCqBHM9VAYN1BQADSAAwRQIgG1bSLeOXp3oB8H7b
+53W+CKOPl2PknmWEq/lMhtn25HkCIQDaHDgWxWFtnCrBjH16/W3Ezn7/U/Vjo5xI
+pDoiVhsLwg==
+-----END CERTIFICATE-----
+
+-----BEGIN CERTIFICATE-----
+MIIF0zCCA7ugAwIBAgIIH8+hjWpIDREwDQYJKoZIhvcNAQELBQAwejELMAkGA1UE
+BhMCQ04xFjAUBgNVBAoMDUFudCBGaW5hbmNpYWwxIDAeBgNVBAsMF0NlcnRpZmlj
+YXRpb24gQXV0aG9yaXR5MTEwLwYDVQQDDChBbnQgRmluYW5jaWFsIENlcnRpZmlj
+YXRpb24gQXV0aG9yaXR5IFIxMB4XDTE4MDMyMTEzNDg0MFoXDTM4MDIyODEzNDg0
+MFowejELMAkGA1UEBhMCQ04xFjAUBgNVBAoMDUFudCBGaW5hbmNpYWwxIDAeBgNV
+BAsMF0NlcnRpZmljYXRpb24gQXV0aG9yaXR5MTEwLwYDVQQDDChBbnQgRmluYW5j
+aWFsIENlcnRpZmljYXRpb24gQXV0aG9yaXR5IFIxMIICIjANBgkqhkiG9w0BAQEF
+AAOCAg8AMIICCgKCAgEAtytTRcBNuur5h8xuxnlKJetT65cHGemGi8oD+beHFPTk
+rUTlFt9Xn7fAVGo6QSsPb9uGLpUFGEdGmbsQ2q9cV4P89qkH04VzIPwT7AywJdt2
+xAvMs+MgHFJzOYfL1QkdOOVO7NwKxH8IvlQgFabWomWk2Ei9WfUyxFjVO1LVh0Bp
+dRBeWLMkdudx0tl3+21t1apnReFNQ5nfX29xeSxIhesaMHDZFViO/DXDNW2BcTs6
+vSWKyJ4YIIIzStumD8K1xMsoaZBMDxg4itjWFaKRgNuPiIn4kjDY3kC66Sl/6yTl
+YUz8AybbEsICZzssdZh7jcNb1VRfk79lgAprm/Ktl+mgrU1gaMGP1OE25JCbqli1
+Pbw/BpPynyP9+XulE+2mxFwTYhKAwpDIDKuYsFUXuo8t261pCovI1CXFzAQM2w7H
+DtA2nOXSW6q0jGDJ5+WauH+K8ZSvA6x4sFo4u0KNCx0ROTBpLif6GTngqo3sj+98
+SZiMNLFMQoQkjkdN5Q5g9N6CFZPVZ6QpO0JcIc7S1le/g9z5iBKnifrKxy0TQjtG
+PsDwc8ubPnRm/F82RReCoyNyx63indpgFfhN7+KxUIQ9cOwwTvemmor0A+ZQamRe
+9LMuiEfEaWUDK+6O0Gl8lO571uI5onYdN1VIgOmwFbe+D8TcuzVjIZ/zvHrAGUcC
+AwEAAaNdMFswCwYDVR0PBAQDAgEGMAwGA1UdEwQFMAMBAf8wHQYDVR0OBBYEFF90
+tATATwda6uWx2yKjh0GynOEBMB8GA1UdIwQYMBaAFF90tATATwda6uWx2yKjh0Gy
+nOEBMA0GCSqGSIb3DQEBCwUAA4ICAQCVYaOtqOLIpsrEikE5lb+UARNSFJg6tpkf
+tJ2U8QF/DejemEHx5IClQu6ajxjtu0Aie4/3UnIXop8nH/Q57l+Wyt9T7N2WPiNq
+JSlYKYbJpPF8LXbuKYG3BTFTdOVFIeRe2NUyYh/xs6bXGr4WKTXb3qBmzR02FSy3
+IODQw5Q6zpXj8prYqFHYsOvGCEc1CwJaSaYwRhTkFedJUxiyhyB5GQwoFfExCVHW
+05ZFCAVYFldCJvUzfzrWubN6wX0DD2dwultgmldOn/W/n8at52mpPNvIdbZb2F41
+T0YZeoWnCJrYXjq/32oc1cmifIHqySnyMnavi75DxPCdZsCOpSAT4j4lAQRGsfgI
+kkLPGQieMfNNkMCKh7qjwdXAVtdqhf0RVtFILH3OyEodlk1HYXqX5iE5wlaKzDop
+PKwf2Q3BErq1xChYGGVS+dEvyXc/2nIBlt7uLWKp4XFjqekKbaGaLJdjYP5b2s7N
+1dM0MXQ/f8XoXKBkJNzEiM3hfsU6DOREgMc1DIsFKxfuMwX3EkVQM1If8ghb6x5Y
+jXayv+NLbidOSzk4vl5QwngO/JYFMkoc6i9LNwEaEtR9PhnrdubxmrtM+RjfBm02
+77q3dSWFESFQ4QxYWew4pHE0DpWbWy/iMIKQ6UZ5RLvB8GEcgt8ON7BBJeMc+Dyi
+kT9qhqn+lw==
+-----END CERTIFICATE-----
+
+-----BEGIN CERTIFICATE-----
+MIICiDCCAgygAwIBAgIIQX76UsB/30owDAYIKoZIzj0EAwMFADB6MQswCQYDVQQG
+EwJDTjEWMBQGA1UECgwNQW50IEZpbmFuY2lhbDEgMB4GA1UECwwXQ2VydGlmaWNh
+dGlvbiBBdXRob3JpdHkxMTAvBgNVBAMMKEFudCBGaW5hbmNpYWwgQ2VydGlmaWNh
+dGlvbiBBdXRob3JpdHkgRTEwHhcNMTkwNDI4MTYyMDQ0WhcNNDkwNDIwMTYyMDQ0
+WjB6MQswCQYDVQQGEwJDTjEWMBQGA1UECgwNQW50IEZpbmFuY2lhbDEgMB4GA1UE
+CwwXQ2VydGlmaWNhdGlvbiBBdXRob3JpdHkxMTAvBgNVBAMMKEFudCBGaW5hbmNp
+YWwgQ2VydGlmaWNhdGlvbiBBdXRob3JpdHkgRTEwdjAQBgcqhkjOPQIBBgUrgQQA
+IgNiAASCCRa94QI0vR5Up9Yr9HEupz6hSoyjySYqo7v837KnmjveUIUNiuC9pWAU
+WP3jwLX3HkzeiNdeg22a0IZPoSUCpasufiLAnfXh6NInLiWBrjLJXDSGaY7vaokt
+rpZvAdmjXTBbMAsGA1UdDwQEAwIBBjAMBgNVHRMEBTADAQH/MB0GA1UdDgQWBBRZ
+4ZTgDpksHL2qcpkFkxD2zVd16TAfBgNVHSMEGDAWgBRZ4ZTgDpksHL2qcpkFkxD2
+zVd16TAMBggqhkjOPQQDAwUAA2gAMGUCMQD4IoqT2hTUn0jt7oXLdMJ8q4vLp6sg
+wHfPiOr9gxreb+e6Oidwd2LDnC4OUqCWiF8CMAzwKs4SnDJYcMLf2vpkbuVE4dTH
+Rglz+HGcTLWsFs4KxLsq7MuU+vJTBUeDJeDjdA==
+-----END CERTIFICATE-----
+
+-----BEGIN CERTIFICATE-----
+MIIDxTCCAq2gAwIBAgIUEMdk6dVgOEIS2cCP0Q43P90Ps5YwDQYJKoZIhvcNAQEF
+BQAwajELMAkGA1UEBhMCQ04xEzARBgNVBAoMCmlUcnVzQ2hpbmExHDAaBgNVBAsM
+E0NoaW5hIFRydXN0IE5ldHdvcmsxKDAmBgNVBAMMH2lUcnVzQ2hpbmEgQ2xhc3Mg
+MiBSb290IENBIC0gRzMwHhcNMTMwNDE4MDkzNjU2WhcNMzMwNDE4MDkzNjU2WjBq
+MQswCQYDVQQGEwJDTjETMBEGA1UECgwKaVRydXNDaGluYTEcMBoGA1UECwwTQ2hp
+bmEgVHJ1c3QgTmV0d29yazEoMCYGA1UEAwwfaVRydXNDaGluYSBDbGFzcyAyIFJv
+b3QgQ0EgLSBHMzCCASIwDQYJKoZIhvcNAQEBBQADggEPADCCAQoCggEBAOPPShpV
+nJbMqqCw6Bz1kehnoPst9pkr0V9idOwU2oyS47/HjJXk9Rd5a9xfwkPO88trUpz5
+4GmmwspDXjVFu9L0eFaRuH3KMha1Ak01citbF7cQLJlS7XI+tpkTGHEY5pt3EsQg
+wykfZl/A1jrnSkspMS997r2Gim54cwz+mTMgDRhZsKK/lbOeBPpWtcFizjXYCqhw
+WktvQfZBYi6o4sHCshnOswi4yV1p+LuFcQ2ciYdWvULh1eZhLxHbGXyznYHi0dGN
+z+I9H8aXxqAQfHVhbdHNzi77hCxFjOy+hHrGsyzjrd2swVQ2iUWP8BfEQqGLqM1g
+KgWKYfcTGdbPB1MCAwEAAaNjMGEwHQYDVR0OBBYEFG/oAMxTVe7y0+408CTAK8hA
+uTyRMB8GA1UdIwQYMBaAFG/oAMxTVe7y0+408CTAK8hAuTyRMA8GA1UdEwEB/wQF
+MAMBAf8wDgYDVR0PAQH/BAQDAgEGMA0GCSqGSIb3DQEBBQUAA4IBAQBLnUTfW7hp
+emMbuUGCk7RBswzOT83bDM6824EkUnf+X0iKS95SUNGeeSWK2o/3ALJo5hi7GZr3
+U8eLaWAcYizfO99UXMRBPw5PRR+gXGEronGUugLpxsjuynoLQu8GQAeysSXKbN1I
+UugDo9u8igJORYA+5ms0s5sCUySqbQ2R5z/GoceyI9LdxIVa1RjVX8pYOj8JFwtn
+DJN3ftSFvNMYwRuILKuqUYSHc2GPYiHVflDh5nDymCMOQFcFG3WsEuB+EYQPFgIU
+1DHmdZcz7Llx8UOZXX2JupWCYzK1XhJb+r4hK5ncf/w8qGtYlmyJpxk3hr1TfUJX
+Yf4Zr0fJsGuv
+-----END CERTIFICATE-----
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay/appPublicCert.crt b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay/appPublicCert.crt
new file mode 100644
index 00000000000..586f34184a8
--- /dev/null
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay/appPublicCert.crt
@@ -0,0 +1,19 @@
+-----BEGIN CERTIFICATE-----
+MIIDmTCCAoGgAwIBAgIQICMFBmpzKUKQGs/81YT4UDANBgkqhkiG9w0BAQsFADCBkTELMAkGA1UE
+BhMCQ04xGzAZBgNVBAoMEkFudCBGaW5hbmNpYWwgdGVzdDElMCMGA1UECwwcQ2VydGlmaWNhdGlv
+biBBdXRob3JpdHkgdGVzdDE+MDwGA1UEAww1QW50IEZpbmFuY2lhbCBDZXJ0aWZpY2F0aW9uIEF1
+dGhvcml0eSBDbGFzcyAyIFIxIHRlc3QwHhcNMjMwNTA2MDYwMTA3WhcNMjQwNTEwMDYwMTA3WjBr
+MQswCQYDVQQGEwJDTjEfMB0GA1UECgwWd21pYWt1Njc0OUBzYW5kYm94LmNvbTEPMA0GA1UECwwG
+QWxpcGF5MSowKAYDVQQDDCEyMDg4NzIxMDAyODQzNDE0LTIwMjEwMDAxMjI2NjczMDYwggEiMA0G
+CSqGSIb3DQEBAQUAA4IBDwAwggEKAoIBAQCd0fbopV7cjL7wbFXEO4b1miViuoY2bwml2L0atB0z
+zVp3ik/K6XY1U770LYqvprqMrpDDWDLNzBjG2RQLvGgtlRI+mZIfuwfewPqvYHsx/kWytVJrb2Vy
+bX7dSTIWBmNhOTHjmV9suYAUc7M6XRZ8aQxbRKrc3/k4yvTvgTgSdi0At0TeNVAIN/xvOo3eP8rB
+7t/XQCCW/GKWLYGsUznriyKWpxuInxTXMOZN1m+YSQ/uJr9hWSa3nDeuHtkGhq5u1wz+tUZhcvaF
+A/Cv+mq40JO7sSHVFxZ+lydu7D6A6do3JeY0WZmR42V6BciJ9y6e3UYrprWlfT3RHx4U3cuNAgMB
+AAGjEjAQMA4GA1UdDwEB/wQEAwIE8DANBgkqhkiG9w0BAQsFAAOCAQEAiLA1wk7or0uqxPSD9z31
+BLJRnamf51Uz2YOSDPjUivu9VJrkjf1PsCleK9RSgOcXcpy9QWZSFIIyakFqCCWylgBdjmhSvzAv
+po86ycJXEBrd7klOM/6VFh68BqpmK1CJl0g29JOJ1fNvIKYJ/WS4ue988NSGrpsVhXbNGALhgiBL
+Gqs7KkZQSsIAySx2HNCSknFM5G5xG6IR6wUJ619khba6rMaKOJ3D7mxuv0Vjyu7DrFBsPLOSdjP1
+Jzi5WmutetJGVrbOKayVjL4xDcU7lgOWTrhTs7S54Z6GnxqRLwy8gD7RmDbXtnVyGP/mUcAgnFtE
+BPFZBe7qjqsCCkf+Zw==
+-----END CERTIFICATE-----
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay2.php b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay2.php
new file mode 100644
index 00000000000..19635431223
--- /dev/null
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/alipay2.php
@@ -0,0 +1,58 @@
+ true,
+ // 签名类型 ( RSA|RSA2 )
+ 'sign_type' => 'RSA2',
+ // 应用ID
+ 'appid' => '2021000122667306',
+ // 应用私钥内容 ( 需1行填写,特别注意:这里的应用私钥通常由支付宝密钥管理工具生成 )
+ 'private_key' => 'MIIEowIBAAKCAQEAndH26KVe3Iy+8GxVxDuG9ZolYrqGNm8Jpdi9GrQdM81ad4pPyul2NVO+9C2Kr6a6jK6Qw1gyzcwYxtkUC7xoLZUSPpmSH7sH3sD6r2B7Mf5FsrVSa29lcm1+3UkyFgZjYTkx45lfbLmAFHOzOl0WfGkMW0Sq3N/5OMr074E4EnYtALdE3jVQCDf8bzqN3j/Kwe7f10Aglvxili2BrFM564silqcbiJ8U1zDmTdZvmEkP7ia/YVkmt5w3rh7ZBoaubtcM/rVGYXL2hQPwr/pquNCTu7Eh1RcWfpcnbuw+gOnaNyXmNFmZkeNlegXIifcunt1GK6a1pX090R8eFN3LjQIDAQABAoIBACrLY4OETCvL8n6pMbyLU7ZHfTm/UGN0So5xLh4OlxiT56MgmzBvjAE72zzFGKU2tcEuGM0Pnn8Vh+ZruLbR+QHbOV5GMExwX9r0Q0XJCL7uryGdb2L4iu6zaEJC9dTpGIulgbSwwyJtTqC9Gu2Jjm5f4dzhyt8n0KGozzAevwCqI9RaJSD96gGWLbMlHCyWKGy1OdBP4V/+agPyHAGZ9gqpfKY7y4L0My8gUxhWzQWOwihtFACjV66ULhutUYT2bro3j1k9UekKlX7IiWrssPmmmw2vfUbrKiNugF6zkfyStPt7jGJ0CdzAHWe3pyF72TyO5NU2NGcX8eKgYlY2crUCgYEAzOcg5Zot9X+Ao+fYH/Oq/3eGZd6krzByiXfcjuRco7mGODwmUnzt3PpPT1fPry9TxarTajt+A9LuxqWawfQ9eWAfrTGAbtDJB0LYo6CynDqUoRqBukROuNaLQiUqEreOQqt08o6VVblgVLv8475ij8s4z/6C2NSSjgUJHf0PL38CgYEAxS0bcXGI+WtempZ4Q3QMTUmp/+B3zuw9JzSV1gvbVi7MleI9V62V2IXHPSXL5mRhYOuQWR0MnVOhbo69fkEA8HpdSd1q2JjaeS+OiZ0ditcJISQJbqWtvmF2+XtQcbVwfID69GWGxyBEHHTW8AtAzIPc6T7x2izyzBw0lXDHSvMCgYEAi+Y61ckhG/9EC6TeMWKjG+21u5P6CQshCK7nzkAo6DhhZb/bwnI9zaSxxdCEom3D2rA5zMx1y5KXKNYlBcwGtPpmZk/oCsFOoECJvZ6YlIaCuERq0oyU2yrQxgat5T2iSe7a2El1uKPrG6+GiNCSZu8wCQMSv4zTy1ew0+LWHW0CgYAvX7ESRpcEZjmqprBqdH1oLGS9566hdr0SqF2/ucWPJVteP6dBY6F3Dl1aYbRlvIRxBuf9oS8gtbE5oO4CYZfaL2wujRZYyBDlwPlcMvWgIB4/aish/IiMD1rIgkpHp7JJF6w0ABiryyLSO3hQ4ENHX/85wzfUlawYQkaYCSq45QKBgCdqrv58KD8tDYn4JnaHJNE+5TgKK5cNhYLZLAsYz7x1KfPdkiC7y/hnenn3TWkm4xw8Tw1rJ1ZIJ24iZgTCTO7EEsB7jZegvg4z/4zVbSK2Y4VI1lJ7jlyqmwg0ArimXTNZFoy66h9c9t2smG40YEZCmmmTLEqVlWgyR1MU5iM5',
+ // 公钥模式,支付宝公钥内容 ( 需1行填写,特别注意:这里不是应用公钥而是支付宝公钥,通常是上传应用公钥换取支付宝公钥,在网页可以复制 )
+ 'public_key' => 'MIIBIjANBgkqhkiG9w0BAQEFAAOCAQ8AMIIBCgKCAQEAuQ70Xj4AHsIsjjuFK2EexkUVDGc6KCBzhHylt5vbgGTJFzrElV6Ri3O4nxwtKzLWykRfjOC5M9z5vt7NrmSc3WPal0B82TNt0SDVO3gDRvnFswB9pC1WHBTRqiGEsX6LpNOCiykyYHAmc3b74R1rotxytOpLt9/HwjZuGStouUQ7gLqnFRwYLKis8tW9FY5NEL9HPENREcRoaUE8zHE6l4jNi5g2Dvs4r5KqnTNvmeRTc87ZylKs4JPhSWskOaqBJmDAcTR770x0G694tKjW5sbm4a/PxWOV3eEG9XLA4CcS6gwHG1KsRu+eTPszQwkEOZCT8PxZJ6SbaUwZsUO4ZQIDAQAB',
+ // 证书模式,应用公钥证书路径 ( 新版资金类接口转 app_cert_sn,如文件 appCertPublicKey.crt )
+ 'app_cert_path' => __DIR__ . '/alipay/appPublicCert.crt', // 'app_cert' => '证书内容',
+ // 证书模式,支付宝根证书路径 ( 新版资金类接口转 alipay_root_cert_sn,如文件 alipayRootCert.crt )
+ 'alipay_root_path' => __DIR__ . '/alipay/alipayRootCert.crt', // 'root_cert' => '证书内容',
+ // 证书模式,支付宝公钥证书路径 ( 未填写 public_key 时启用此参数,如文件 alipayPublicCert.crt )
+ // 'alipay_cert_path' => __DIR__ . '/alipay/alipayPublicCert.crt', // 'public_key' => '证书内容'
+ // 支付成功通知地址
+ 'notify_url' => '',
+ // 网页支付回跳地址
+ 'return_url' => '',
+];
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/config.php b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/config.php
index e6f8075845a..677d67b0094 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/config.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/config.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
// 配置缓存处理函数
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/mini-login.php b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/mini-login.php
index c6f56bac087..b5e8e302268 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/mini-login.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/mini-login.php
@@ -1,5 +1,19 @@
time(),
'total_fee' => '1',
'openid' => 'o38gpszoJoC9oJYz3UHHf6bEp0Lo',
- 'trade_type' => 'JSAPI',
- 'notify_url' => 'http://a.com/text.html',
+ 'trade_type' => 'JSAPI', // JSAPI--JSAPI支付(服务号或小程序支付)、NATIVE--Native 支付、APP--APP支付,MWEB--H5支付
+ 'notify_url' => 'https://a.com/text.html',
'spbill_create_ip' => '127.0.0.1',
];
+
// 生成预支付码
$result = $wechat->createOrder($options);
+
+ echo '';
+ if ($options['trade_type'] === 'NATIVE') {
+ echo '二维码 NATIVE 支付,直接使用 code_url 生成二维码即可
';
+ var_export($result);
+ return;
+ }
+
// 创建JSAPI参数签名
$options = $wechat->createParamsForJsApi($result['prepay_id']);
- echo '';
- echo "\n--- 创建预支付码 ---\n";
+ echo "--- 创建 JSAPI 预支付码 ---
";
var_export($result);
+// array(
+// 'return_code' => 'SUCCESS',
+// 'return_msg' => 'OK',
+// 'result_code' => 'SUCCESS',
+// 'mch_id' => '1332187001',
+// 'appid' => 'wx60a43dd8161666d4',
+// 'nonce_str' => 'YIPDbGWT1jpLLM5R',
+// 'sign' => '7EBBA1B5F196CF122C920D10FA768D96',
+// 'prepay_id' => 'wx211858080224615a10c2fc9f6c824f0000',
+// 'trade_type' => 'JSAPI',
+// )
- echo "\n\n--- JSAPI 及 H5 参数 ---\n";
+ echo "--- 生成 JSAPI 及 H5 支付参数 ---
";
var_export($options);
+// array(
+// 'appId' => 'wx60a43dd8161666d4',
+// 'timeStamp' => '1669028299',
+// 'nonceStr' => '5s7h0dyp0nmzylbqytb462fpnb0tmrjg',
+// 'package' => 'prepay_id=wx21185819502283c23cca162e9d787f0000',
+// 'signType' => 'MD5',
+// 'paySign' => 'BBE0F426B8E1EEC9E45AC4459E8AE9D6',
+// 'timestamp' => '1669028299',
+// )
-} catch (Exception $e) {
+} catch (Exception $exception) {
// 出错啦,处理下吧
- echo $e->getMessage() . PHP_EOL;
+ echo $exception->getMessage() . PHP_EOL;
}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-order-notify.php b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-order-notify.php
index 7484aace977..32a84f5a03a 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-order-notify.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-order-notify.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
try {
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-order-query.php b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-order-query.php
index b7edf34a1b3..23e917865c2 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-order-query.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-order-query.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
try {
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-redpack-create.php b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-redpack-create.php
index c45dadeb428..f614f59da0f 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-redpack-create.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-redpack-create.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
try {
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-refund-create.php b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-refund-create.php
index 8e3df01ffc5..21e79d5043c 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-refund-create.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-refund-create.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
try {
@@ -28,7 +30,7 @@
// 4. 组装参数,可以参考官方商户文档
$options = [
'transaction_id' => '1008450740201411110005820873',
- 'out_refund_no' => '商户退款单号',
+ 'out_refund_no' => '3241251235123',
'total_fee' => '1',
'refund_fee' => '1',
];
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-refund-query.php b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-refund-query.php
index fa63acc9eb9..3af6db3aa65 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-refund-query.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-refund-query.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
try {
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-transfers-create.php b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-transfers-create.php
index 659d888e2d8..4053445f2d2 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-transfers-create.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-transfers-create.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
try {
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-transfersbank-create.php b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-transfersbank-create.php
index ca3979edfe8..885ab249e55 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-transfersbank-create.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-transfersbank-create.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
try {
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-v3-config-cert.php b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-v3-config-cert.php
new file mode 100644
index 00000000000..6b689af5f92
--- /dev/null
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-v3-config-cert.php
@@ -0,0 +1,31 @@
+download();
+
+} catch (\Exception $exception) {
+ // 出错啦,处理下吧
+ echo $exception->getMessage() . PHP_EOL;
+}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-v3-config.php b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-v3-config.php
new file mode 100644
index 00000000000..1723fd4b5c9
--- /dev/null
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-v3-config.php
@@ -0,0 +1,44 @@
+ '',
+ // 必填,微信商户编号ID
+ 'mch_id' => '',
+ // 必填,微信商户V3接口密钥
+ 'mch_v3_key' => '',
+ // 可选,微信商户证书序列号,可从公钥中提取
+ 'cert_serial' => '',
+ // 必填,微信商户证书公钥,支持证书内容或文件路径
+ 'cert_public' => $certPublic,
+ // 必填,微信商户证书私钥,支持证书内容或文件路径
+ 'cert_private' => $certPrivate,
+ // 可选,运行时的文件缓存路径
+ 'cache_path' => ''
+];
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-v3-order-app.php b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-v3-order-app.php
new file mode 100644
index 00000000000..aa932bacd3e
--- /dev/null
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-v3-order-app.php
@@ -0,0 +1,64 @@
+create('app', [
+ 'appid' => $config['appid'],
+ 'mchid' => $config['mch_id'],
+ 'description' => '商品描述',
+ 'out_trade_no' => $order,
+ 'notify_url' => 'https://thinkadmin.top',
+ 'amount' => ['total' => 2, 'currency' => 'CNY'],
+ ]);
+
+ echo '';
+ echo "\n--- 创建支付参数 ---\n";
+ var_export($result);
+
+ // 创建退款
+ $out_refund_no = strval(time());
+ $result = $payment->createRefund([
+ 'out_trade_no' => $order,
+ 'out_refund_no' => $out_refund_no,
+ 'amount' => [
+ 'refund' => 2,
+ 'total' => 2,
+ 'currency' => 'CNY'
+ ]
+ ]);
+ echo "\n--- 创建退款订单 ---\n";
+ var_export($result);
+
+ $result = $payment->queryRefund($out_refund_no);
+
+ echo "\n--- 查询退款订单 ---\n";
+ var_export($result);
+
+} catch (\Exception $exception) {
+ // 出错啦,处理下吧
+ echo $exception->getMessage() . PHP_EOL;
+}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-v3-order-h5.php b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-v3-order-h5.php
new file mode 100644
index 00000000000..784a2def4f0
--- /dev/null
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-v3-order-h5.php
@@ -0,0 +1,50 @@
+create('h5', [
+ 'appid' => $config['appid'],
+ 'mchid' => $config['mch_id'],
+ 'description' => '商品描述',
+ 'out_trade_no' => (string)time(),
+ 'notify_url' => 'https://thinkadmin.top',
+ 'amount' => ['total' => 2, 'currency' => 'CNY'],
+ 'scene_info' => [
+ 'h5_info' => [
+ 'type' => 'Wap',
+ ],
+ 'payer_client_ip' => '14.23.150.211',
+ ],
+ ]);
+
+ echo '';
+ echo "\n--- 创建支付参数 ---\n";
+ var_export($result);
+
+} catch (\Exception $exception) {
+ // 出错啦,处理下吧
+ echo $exception->getMessage() . PHP_EOL;
+}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-v3-order-jsapi.php b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-v3-order-jsapi.php
new file mode 100644
index 00000000000..3abe35730c6
--- /dev/null
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-v3-order-jsapi.php
@@ -0,0 +1,89 @@
+create('jsapi', [
+ 'appid' => $config['appid'],
+ 'mchid' => $config['mch_id'],
+ 'description' => '商品描述',
+ 'out_trade_no' => $order,
+ 'notify_url' => 'https://thinkadmin.top',
+ 'payer' => ['openid' => 'o38gps3vNdCqaggFfrBRCRikwlWY'],
+ 'amount' => ['total' => 2, 'currency' => 'CNY'],
+ ]);
+
+ echo '';
+ echo "\n--- 创建支付参数 ---\n";
+ var_export($result);
+
+// array(
+// 'appId' => 'wx60a43dd8161666d4',
+// 'timeStamp' => '1669027650',
+// 'nonceStr' => 'dfscg4lm02uqy448kjd1kjs2eo26joom',
+// 'package' => 'prepay_id=wx211847302881094d83b1917194ca880000',
+// 'signType' => 'RSA',
+// 'paySign' => '1wvvi4vmcJmP3GXB0H52mxp8lOhyqE4BtLmyi3Flg8DVKCES4fsb6+0z/L9sYkbp/TNinsnK0k7mUpTe2Yo86P1DLg18fR7zsIn5u1+3tI58boHk3VsAJl4Uhlti9ME3T7kRq1bEb4DGxp16+ixRynOqndkIkYXxrREhsrZIQlsGMfNCV0K1707s7jBTgqIm1vlkpIjNEg8nbcuG88Vzly4dR1a9K6Fux+sm0gu2rMroRwIo2R/0rgHGDANmnAZj6YEfLZlRrGTbr9r0V1+HHQPvV4BJLvTG8KXVJmJSJzBWSgq31PwrLWdOwdtpNKk7wJbY7yoScYUysYqqzM4DTQ==',
+// );
+
+ echo "\n\n--- 查询支付参数 ---\n";
+ $result = $payment->query($order);
+ var_export($result);
+
+// array(
+// 'amount' => array('payer_currency' => 'CNY', 'total' => 2),
+// 'appid' => 'wx60a43dd8161666d4',
+// 'mchid' => '1332187001',
+// 'out_trade_no' => '1669027802',
+// 'promotion_detail' => array(),
+// 'scene_info' => array('device_id' => ''),
+// 'trade_state' => 'NOTPAY',
+// 'trade_state_desc' => '订单未支付',
+// );
+
+ // 创建退款
+ $out_refund_no = strval(time());
+ $result = $payment->createRefund([
+ 'out_trade_no' => $order,
+ 'out_refund_no' => $out_refund_no,
+ 'amount' => [
+ 'refund' => 2,
+ 'total' => 2,
+ 'currency' => 'CNY'
+ ]
+ ]);
+ echo "\n--- 创建退款订单2 ---\n";
+ var_export($result);
+
+ $result = $payment->queryRefund($out_refund_no);
+
+ echo "\n--- 查询退款订单2 ---\n";
+ var_export($result);
+
+} catch (\Exception $exception) {
+ // 出错啦,处理下吧
+ echo $exception->getMessage() . PHP_EOL;
+}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-v3-order-native.php b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-v3-order-native.php
new file mode 100644
index 00000000000..9a6d5ee3312
--- /dev/null
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-v3-order-native.php
@@ -0,0 +1,82 @@
+create('native', [
+ 'appid' => $config['appid'],
+ 'mchid' => $config['mch_id'],
+ 'description' => '商品描述',
+ 'out_trade_no' => $order,
+ 'notify_url' => 'https://thinkadmin.top',
+ 'amount' => ['total' => 2, 'currency' => 'CNY'],
+ ]);
+
+ echo '';
+ echo "\n--- 创建支付参数 ---\n";
+ var_export($result);
+
+// array('code_url' => 'weixin://wxpay/bizpayurl?pr=cdJXOVDzz');
+
+
+ echo "\n\n--- 查询支付参数 ---\n";
+ $result = $payment->query($order);
+ var_export($result);
+
+// array(
+// 'amount' => array('payer_currency' => 'CNY', 'total' => 2),
+// 'appid' => 'wx60a43dd8161666d4',
+// 'mchid' => '1332187001',
+// 'out_trade_no' => '1669027871',
+// 'promotion_detail' => array(),
+// 'scene_info' => array('device_id' => ''),
+// 'trade_state' => 'NOTPAY',
+// 'trade_state_desc' => '订单未支付',
+// );
+
+ // 创建退款
+ $out_refund_no = strval(time());
+ $result = $payment->createRefund([
+ 'out_trade_no' => $order,
+ 'out_refund_no' => $out_refund_no,
+ 'amount' => [
+ 'refund' => 2,
+ 'total' => 2,
+ 'currency' => 'CNY'
+ ]
+ ]);
+ echo "\n--- 创建退款订单2 ---\n";
+ var_export($result);
+
+ $result = $payment->queryRefund($out_refund_no);
+
+ echo "\n--- 查询退款订单2 ---\n";
+ var_export($result);
+
+} catch (\Exception $exception) {
+ // 出错啦,处理下吧
+ echo $exception->getMessage() . PHP_EOL;
+}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-v3-transfer.php b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-v3-transfer.php
new file mode 100644
index 00000000000..bc018b06a7d
--- /dev/null
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/pay-v3-transfer.php
@@ -0,0 +1,48 @@
+batchs([
+ 'out_batch_no' => 'plfk2020042013',
+ 'batch_name' => '2019年1月深圳分部报销单',
+ 'batch_remark' => '2019年1月深圳分部报销单',
+ 'total_amount' => 100,
+ 'transfer_detail_list' => [
+ [
+ 'out_detail_no' => 'x23zy545Bd5436',
+ 'transfer_amount' => 100,
+ 'transfer_remark' => '2020年4月报销',
+ 'openid' => 'o-MYE42l80oelYMDE34nYD456Xoy',
+ 'user_name' => '小小邹'
+ ]
+ ]
+ ]);
+
+ echo "\n--- 批量打款 ---\n";
+ var_export($result);
+
+} catch (\Exception $exception) {
+ // 出错啦,处理下吧
+ echo $exception->getMessage() . PHP_EOL;
+}
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/wechat-jssdk-sign.php b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/wechat-jssdk-sign.php
index 6a3b6985c56..89d5ea11a56 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/wechat-jssdk-sign.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/wechat-jssdk-sign.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
try {
@@ -26,7 +28,7 @@
$wechat = \WeChat\Script::instance($config);
// 4. 获取JSSDK网址签名配置
- $result = $wechat->getJsSign('http://a.com/test.php');
+ $result = $wechat->getJsSign('https://a.com/test.php');
var_export($result);
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/wechat-menu-get.php b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/wechat-menu-get.php
index 5447aad17e3..1622fba8555 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/wechat-menu-get.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/wechat-menu-get.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
try {
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/wechat-qrcode-create.php b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/wechat-qrcode-create.php
index 256e30ad955..9470892aee4 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/wechat-qrcode-create.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/wechat-qrcode-create.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
try {
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/wechat-user-get.php b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/wechat-user-get.php
index d42cb5632cf..299c25268be 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/wechat-user-get.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/wechat-user-get.php
@@ -3,13 +3,15 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
try {
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/work-config.php b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/work-config.php
new file mode 100644
index 00000000000..799cdf42bc1
--- /dev/null
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/work-config.php
@@ -0,0 +1,20 @@
+ '', // 企业ID
+ 'appsecret' => '', // 应用的凭证密钥
+];
\ No newline at end of file
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/_test/work-department.php b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/work-department.php
new file mode 100644
index 00000000000..a894d72028c
--- /dev/null
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/_test/work-department.php
@@ -0,0 +1,32 @@
+callGetApi($url);
+ echo '';
+ print_r(BasicWeWork::instance($config)->config->get());
+ print_r($result);
+ echo '
';
+} catch (Exception $exception) {
+ echo $exception->getMessage() . PHP_EOL;
+}
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/composer.json b/upload/system/storage/vendor/zoujingli/wechat-developer/composer.json
index d8ee7c14c5f..ed28ef599b4 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/composer.json
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/composer.json
@@ -1,18 +1,17 @@
{
"type": "library",
"name": "zoujingli/wechat-developer",
- "homepage": "https://github.com/zoujingli/WeChatDeveloper",
- "description": "WeChat platform and WeChat payment development tools",
+ "homepage": "https://thinkadmin.top",
+ "description": "WeChat and Alipay Platform Development",
"license": "MIT",
"authors": [
{
"name": "Anyon",
"email": "zoujingli@qq.com",
- "homepage": "http://ctolog.com"
+ "homepage": "https://thinkadmin.top"
}
],
"keywords": [
- "WePay",
"AliPay",
"WeMini",
"WeChat",
@@ -21,8 +20,10 @@
],
"require": {
"php": ">=5.4",
+ "ext-xml": "*",
"ext-json": "*",
"ext-curl": "*",
+ "ext-bcmath": "*",
"ext-libxml": "*",
"ext-openssl": "*",
"ext-mbstring": "*",
@@ -34,9 +35,10 @@
],
"psr-4": {
"WePay\\": "WePay",
- "WeMini\\": "WeMini",
"WeChat\\": "WeChat",
- "AliPay\\": "AliPay"
+ "WeMini\\": "WeMini",
+ "AliPay\\": "AliPay",
+ "WePayV3\\": "WePayV3"
}
}
-}
\ No newline at end of file
+}
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/include.php b/upload/system/storage/vendor/zoujingli/wechat-developer/include.php
index e88c70ececa..b14e03d7df4 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/include.php
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/include.php
@@ -3,20 +3,22 @@
// +----------------------------------------------------------------------
// | WeChatDeveloper
// +----------------------------------------------------------------------
-// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
+// | 版权所有 2014~2024 ThinkAdmin [ thinkadmin.top ]
// +----------------------------------------------------------------------
-// | 官方网站: http://think.ctolog.com
+// | 官方网站: https://thinkadmin.top
// +----------------------------------------------------------------------
// | 开源协议 ( https://mit-license.org )
+// | 免责声明 ( https://thinkadmin.top/disclaimer )
// +----------------------------------------------------------------------
-// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
+// | gitee 代码仓库:https://gitee.com/zoujingli/WeChatDeveloper
+// | github 代码仓库:https://github.com/zoujingli/WeChatDeveloper
// +----------------------------------------------------------------------
spl_autoload_register(function ($classname) {
$pathname = __DIR__ . DIRECTORY_SEPARATOR;
$filename = str_replace('\\', DIRECTORY_SEPARATOR, $classname) . '.php';
if (file_exists($pathname . $filename)) {
- foreach (['WeChat', 'WeMini', 'AliPay', 'WePay', 'We'] as $prefix) {
+ foreach (['AliPay', 'WeChat', 'WeMini', 'WePay', 'We'] as $prefix) {
if (stripos($classname, $prefix) === 0) {
include $pathname . $filename;
return true;
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/MIT-LICENSE.txt b/upload/system/storage/vendor/zoujingli/wechat-developer/license
similarity index 95%
rename from upload/system/storage/vendor/zoujingli/wechat-developer/MIT-LICENSE.txt
rename to upload/system/storage/vendor/zoujingli/wechat-developer/license
index bceed538078..a3fafa8411a 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/MIT-LICENSE.txt
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/license
@@ -1,6 +1,6 @@
The MIT License
-Copyright (c) 2014-2018 Anyon
+Copyright (c) 2014-2023 Anyon
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
diff --git a/upload/system/storage/vendor/zoujingli/wechat-developer/readme.md b/upload/system/storage/vendor/zoujingli/wechat-developer/readme.md
index 529d1f4b89e..a39cddf9260 100644
--- a/upload/system/storage/vendor/zoujingli/wechat-developer/readme.md
+++ b/upload/system/storage/vendor/zoujingli/wechat-developer/readme.md
@@ -1,19 +1,23 @@
-[](https://packagist.org/packages/zoujingli/wechat-developer)
-[](https://packagist.org/packages/zoujingli/wechat-developer)
-[](https://packagist.org/packages/zoujingli/wechat-developer)
+# WeChatDeveloper for PHP
+
+[](https://packagist.org/packages/zoujingli/wechat-developer)
+[](https://packagist.org/packages/zoujingli/wechat-developer)
+[](https://packagist.org/packages/zoujingli/wechat-developer)
+[](https://packagist.org/packages/zoujingli/wechat-developer)
+[](https://packagist.org/packages/wechat-developer)
[](https://packagist.org/packages/zoujingli/wechat-developer)
-WeChatDeveloper for PHP
-----
* WeChatDeveloper 是基于 [wechat-php-sdk](https://github.com/zoujingli/wechat-php-sdk) 重构,优化并完善;
* 运行最底要求 PHP 版本 5.4 , 建议在 PHP7 上运行以获取最佳性能;
-* WeChatDeveloper 针对 access_token 失效增加了自动刷新机制;
-* 微信的部分接口需要缓存数据在本地,因此对目录需要有写权限;
+* 目前 WeChatDeveloper 针对 access_token 失效增加了自动刷新机制;
+* 微信的部分接口需要缓存数据在本地,因此配置目录并需要对目录有写权限;
* 我们鼓励大家使用 composer 来管理您的第三方库,方便后期更新操作;
* WeChatDeveloper 已历经数个线上项目考验,欢迎 fork 或 star 此项目。
+* 微信商户已经支持 v2 和 v3 接口,未加入的接口可以使用通用方式调用。
功能描述
----
+
* 微信小程序,服务端接口支持
* 微信认证服务号,服务端接口支持
* 微信支付(账单、卡券、红包、退款、转账、App支付、JSAPI支付、Web支付、扫码支付等)
@@ -23,68 +27,72 @@ WeChatDeveloper for PHP
----
PHP开发技术交流(QQ群 513350915)
-[](http://shang.qq.com/wpa/qunwpa?idkey=ae25cf789dafbef62e50a980ffc31242f150bc61a61164458216dd98c411832a)
+[](http://shang.qq.com/wpa/qunwpa?idkey=ae25cf789dafbef62e50a980ffc31242f150bc61a61164458216dd98c411832a)
WeChatDeveloper 是基于官方接口封装,在做微信开发前,必需先阅读微信官方文档。
+
* 微信官方文档:https://mp.weixin.qq.com/wiki
* 商户支付文档:https://pay.weixin.qq.com/wiki/doc/api/index.html
针对 WeChatDeveloper 也有一准备了帮助资料可供参考。
+
* ThinkAdmin:https://github.com/zoujingli/ThinkAdmin
* WeChatDeveloper:https://www.kancloud.cn/zoujingli/wechat-developer
-
代码仓库
----
WeChatDeveloper 为开源项目,允许把它用于任何地方,不受任何约束,欢迎 fork 项目。
+
* Gitee 托管地址:https://gitee.com/zoujingli/WeChatDeveloper
* GitHub 托管地址:https://github.com/zoujingli/WeChatDeveloper
-文件说明
+文件说明(后续会根据官方文档增加文件)
----
-|文件名|类名|描述|类型|加载 ①|
-|---|---|---|---|---|
-| App.php | AliPay\App | 支付宝App支付 | 支付宝支付 | \We::AliPayApp() |
-| Bill.php | AliPay\Bill | 支付宝账单下载 | 支付宝支付 | \We::AliPayBill() |
-| Pos.php | AliPay\Pos | 支付宝刷卡支付 | 支付宝支付 | \We::AliPayPos() |
-| Scan.php | AliPay\Scan | 支付宝扫码支付 | 支付宝支付 | \We::AliPayScan() |
-| Transfer.php | AliPay\Transfer | 支付宝转账 | 支付宝支付 | \We::AliPayTransfer() |
-| Wap.php | AliPay\Wap | 支付宝Wap支付 | 支付宝支付 | \We::AliPayWap() |
-| Web.php | AliPay\Web | 支付宝Web支付 | 支付宝支付 | \We::AliPayWeb() |
-| Card.php | WeChat\Card | 微信卡券接口支持 | 认证服务号 | \We::WeChatCard() |
-| Custom.php | WeChat\Custom | 微信客服消息接口支持 | 认证服务号 | \We::WeChatCustom() |
-| Media.php | WeChat\Media | 微信媒体素材接口支持 | 认证服务号 | \We::WeChatMedia() |
-| Oauth.php | WeChat\Oauth | 微信网页授权消息类接口 | 认证服务号 | \We::WeChatOauth() |
-| Pay.php | WeChat\Pay | 微信支付类接口 | 认证服务号 | \We::WeChatPay() |
-| Product.php | WeChat\Product | 微信商店类接口 | 认证服务号 | \We::WeChatProduct() |
-| Qrcode.php | WeChat\Qrcode | 微信二维码接口支持 | 认证服务号 | \We::WeChatQrcode() |
-| Receive.php | WeChat\Receive | 微信推送事件消息处理支持 | 认证服务号 | \We::WeChatReceive() |
-| Scan.php | WeChat\Scan | 微信扫一扫接口支持 | 认证服务号 | \We::WeChatScan() |
-| Script.php | WeChat\Script | 微信前端JSSDK支持 | 认证服务号 | \We::WeChatScript() |
-| Shake.php | WeChat\Shake | 微信蓝牙设备揺一揺接口 | 认证服务号 | \We::WeChatShake() |
-| Tags.php | WeChat\Tags | 微信粉丝标签接口支持 | 认证服务号 | \We::WeChatTags() |
-| Template.php | WeChat\Template | 微信模板消息接口支持 | 认证服务号 | \We::WeChatTemplate() |
-| User.php | WeChat\User | 微信粉丝管理接口支持 | 认证服务号 | \We::WeChatCard() |
-| Wifi.php | WeChat\Wifi | 微信门店WIFI管理支持 | 认证服务号 | \We::WeChatWifi() |
-| Bill.php | WePay\Bill | 微信商户账单及评论 | 微信支付 | \We::WePayBill() |
-| Coupon.php | WePay\Coupon | 微信商户代金券 | 微信支付 | \We::WePayCoupon() |
-| Order.php | WePay\Order | 微信商户订单 | 微信支付 | \We::WePayOrder() |
-| Redpack.php | WePay\Redpack | 微信红包支持 | 微信支付 | \We::WePayRedpack() |
-| Refund.php | WePay\Refund | 微信商户退款 | 微信支付 | \We::WePayRefund() |
-| Transfers.php | WePay\Transfers | 微信商户打款到零钱 | 微信支付 | \We::WePayTransfers() |
-| TransfersBank.php | WePay\TransfersBank | 微信商户打款到银行卡 | 微信支付 | \We::WePayTransfersBank() |
-| Crypt.php | WeMini\Crypt | 微信小程序数据加密处理 | 微信小程序 | \We::WeMiniCrypt() |
-| Plugs.php | WeMini\Plugs | 微信小程序插件管理 | 微信小程序 | \We::WeMiniPlugs() |
-| Poi.php | WeMini\Poi | 微信小程序地址管理 | 微信小程序 | \We::WeMiniPoi() |
-| Qrcode.php | WeMini\Qrcode | 微信小程序二维码管理 | 微信小程序 | \We::WeMiniCrypt() |
-| Template.php | WeMini\Template | 微信小程序模板消息支持 | 微信小程序 | \We::WeMiniTemplate() |
-| Total.php | WeMini\Total | 微信小程序数据接口 | 微信小程序 | \We::WeMiniTotal() |
-
+| 文件名 | 类名 | 描述 | 类型 | 加载 ① |
+|-------------------|---------------------|--------------|-------|---------------------------|
+| App.php | AliPay\App | 支付宝App支付 | 支付宝支付 | \We::AliPayApp() |
+| Bill.php | AliPay\Bill | 支付宝账单下载 | 支付宝支付 | \We::AliPayBill() |
+| Pos.php | AliPay\Pos | 支付宝刷卡支付 | 支付宝支付 | \We::AliPayPos() |
+| Scan.php | AliPay\Scan | 支付宝扫码支付 | 支付宝支付 | \We::AliPayScan() |
+| Transfer.php | AliPay\Transfer | 支付宝转账 | 支付宝支付 | \We::AliPayTransfer() |
+| Wap.php | AliPay\Wap | 支付宝Wap支付 | 支付宝支付 | \We::AliPayWap() |
+| Web.php | AliPay\Web | 支付宝Web支付 | 支付宝支付 | \We::AliPayWeb() |
+| Card.php | WeChat\Card | 微信卡券接口支持 | 认证服务号 | \We::WeChatCard() |
+| Custom.php | WeChat\Custom | 微信客服消息接口支持 | 认证服务号 | \We::WeChatCustom() |
+| Media.php | WeChat\Media | 微信媒体素材接口支持 | 认证服务号 | \We::WeChatMedia() |
+| Oauth.php | WeChat\Oauth | 微信网页授权消息类接口 | 认证服务号 | \We::WeChatOauth() |
+| Pay.php | WeChat\Pay | 微信支付类接口 | 认证服务号 | \We::WeChatPay() |
+| Product.php | WeChat\Product | 微信商店类接口 | 认证服务号 | \We::WeChatProduct() |
+| Qrcode.php | WeChat\Qrcode | 微信二维码接口支持 | 认证服务号 | \We::WeChatQrcode() |
+| Receive.php | WeChat\Receive | 微信推送事件消息处理支持 | 认证服务号 | \We::WeChatReceive() |
+| Scan.php | WeChat\Scan | 微信扫一扫接口支持 | 认证服务号 | \We::WeChatScan() |
+| Script.php | WeChat\Script | 微信前端JSSDK支持 | 认证服务号 | \We::WeChatScript() |
+| Shake.php | WeChat\Shake | 微信蓝牙设备揺一揺接口 | 认证服务号 | \We::WeChatShake() |
+| Tags.php | WeChat\Tags | 微信粉丝标签接口支持 | 认证服务号 | \We::WeChatTags() |
+| Template.php | WeChat\Template | 微信模板消息接口支持 | 认证服务号 | \We::WeChatTemplate() |
+| User.php | WeChat\User | 微信粉丝管理接口支持 | 认证服务号 | \We::WeChatCard() |
+| Wifi.php | WeChat\Wifi | 微信门店WIFI管理支持 | 认证服务号 | \We::WeChatWifi() |
+| Draft.php | WeChat\Draft | 微信草稿箱 | 认证服务号 | \We::WeChatDraft() |
+| Freepublish.php | WeChat\Freepublish | 微信发布能力 | 认证服务号 | \We::WeChatFreepublish() |
+| Bill.php | WePay\Bill | 微信商户账单及评论 | 微信支付 | \We::WePayBill() |
+| Coupon.php | WePay\Coupon | 微信商户代金券 | 微信支付 | \We::WePayCoupon() |
+| Order.php | WePay\Order | 微信商户订单 | 微信支付 | \We::WePayOrder() |
+| Redpack.php | WePay\Redpack | 微信红包支持 | 微信支付 | \We::WePayRedpack() |
+| Refund.php | WePay\Refund | 微信商户退款 | 微信支付 | \We::WePayRefund() |
+| Transfers.php | WePay\Transfers | 微信商户打款到零钱 | 微信支付 | \We::WePayTransfers() |
+| TransfersBank.php | WePay\TransfersBank | 微信商户打款到银行卡 | 微信支付 | \We::WePayTransfersBank() |
+| Crypt.php | WeMini\Crypt | 微信小程序数据加密处理 | 微信小程序 | \We::WeMiniCrypt() |
+| Plugs.php | WeMini\Plugs | 微信小程序插件管理 | 微信小程序 | \We::WeMiniPlugs() |
+| Poi.php | WeMini\Poi | 微信小程序地址管理 | 微信小程序 | \We::WeMiniPoi() |
+| Qrcode.php | WeMini\Qrcode | 微信小程序二维码管理 | 微信小程序 | \We::WeMiniCrypt() |
+| Template.php | WeMini\Template | 微信小程序模板消息支持 | 微信小程序 | \We::WeMiniTemplate() |
+| Total.php | WeMini\Total | 微信小程序数据接口 | 微信小程序 | \We::WeMiniTotal() |
安装使用
----
1.1 通过 Composer 来管理安装
+
```shell
# 首次安装 线上版本(稳定)
composer require zoujingli/wechat-developer
@@ -97,12 +105,14 @@ composer update zoujingli/wechat-developer
```
1.2 如果不使用 Composer, 可以下载 WeChatDeveloper 并解压到项目中
+
```php
# 在项目中加载初始化文件
include "您的目录/WeChatDeveloper/include.php";
```
2.1 接口实例所需参数
+
```php
$config = [
'token' => 'test',
@@ -121,6 +131,7 @@ $config = [
```
3.1 实例指定接口
+
```php
try {
@@ -144,6 +155,7 @@ try {
微信支付
---
+
```php
// 创建接口实例
$wechat = new \WeChat\Pay($config);
@@ -176,28 +188,41 @@ try {
}
```
+
* 更多功能请阅读测试代码或SDK封装源码
支付宝支付
----
+
* 支付参数配置(可用沙箱模式)
+
```php
$config = [
// 沙箱模式
- 'debug' => true,
+ 'debug' => true,
+ // 签名类型 ( RSA|RSA2 )
+ 'sign_type' => 'RSA2',
// 应用ID
- 'appid' => '2016090900468879',
- // 支付宝公钥(1行填写)
- 'public_key' => 'MIIBIjANBgkqhkiG9w0BAQEFAAOCAQ8AMIIBCgKCAQEAtU71NY53UDGY7JNvLYAhsNa+taTF6KthIHJmGgdio9bkqeJGhHk6ttkTKkLqFgwIfgAkHpdKiOv1uZw6gVGZ7TCu5LfHTqKrCd6Uz+N7hxhY+4IwicLgprcV1flXQLmbkJYzFMZqkXGkSgOsR2yXh4LyQZczgk9N456uuzGtRy7MoB4zQy34PLUkkxR6W1B2ftNbLRGXv6tc7p/cmDcrY6K1bSxnGmfRxFSb8lRfhe0V0UM6pKq2SGGSeovrKHN0OLp+Nn5wcULVnFgATXGCENshRlp96piPEBFwneXs19n+sX1jx60FTR7/rME3sW3AHug0fhZ9mSqW4x401WjdnwIDAQAB',
- // 支付宝私钥(1行填写)
- 'private_key' => 'MIIEvQIBADANBgkqhkiG9w0BAQEFAASCBKcwggSjAgEAAoIBAQC3pbN7esinxgjE8uxXAsccgGNKIq+PR1LteNTFOy0fsete43ObQCrzd9DO0zaUeBUzpIOnxrKxez7QoZROZMYrinttFZ/V5rbObEM9E5AR5Tv/Fr4IBywoS8ZtN16Xb+fZmibfU91yq9O2RYSvscncU2qEYmmaTenM0QlUO80ZKqPsM5JkgCNdcYZTUeHclWeyER3dSImNtlSKiSBSSTHthb11fkudjzdiUXua0NKVWyYuAOoDMcpXbD6NJmYqEA/iZ/AxtQt08pv0Mow581GPB0Uop5+qA2hCV85DpagE94a067sKcRui0rtkJzHem9k7xVL+2RoFm1fv3RnUkMwhAgMBAAECggEAAetkddzxrfc+7jgPylUIGb8pyoOUTC4Vqs/BgZI9xYAJksNT2QKRsFvHPfItNt4Ocqy8h4tnIL3GCU43C564B4p6AcjhE85GiN/O0BudPOKlfuQQ9mqExqMMHuYeQfz0cmzPDTSGMwWiv9v4KBH2pyvkCCAzNF6uG+rvawb4/NNVuiI7C8Ku/wYsamtbgjMZVOFFdScYgIw1BgA99RUU/fWBLMnTQkoyowSRb9eSmEUHjt/WQt+/QgKAT2WmuX4RhaGy0qcQLbNaJNKXdJ+PVhQrSiasINNtqYMa8GsQuuKsk3X8TCg9K6/lowivt5ruhyWcP2sx93zY/LGzIHgHcQKBgQDoZlcs9RWxTdGDdtH8kk0J/r+QtMijNzWI0a+t+ZsWOyd3rw+uM/8O4JTNP4Y98TvvxhJXewITbfiuOIbW1mxh8bnO/fcz7+RXZKgPDeoTeNo717tZFZGBEyUdH9M9Inqvht7+hjVDIMCYBDomYebdk3Xqo4mDBjLRdVNGrhGmVQKBgQDKS/MgTMK8Ktfnu1KzwCbn/FfHTOrp1a1t1wWPv9AW0rJPYeaP6lOkgIoO/1odG9qDDhdB6njqM+mKY5Yr3N94PHamHbwJUCmbkqEunCWpGzgcQZ1Q254xk9D7UKq/XUqW2WDqDq80GQeNial+fBc46yelQzokwdA+JdIFKoyinQKBgQCBems9V/rTAtkk1nFdt6EGXZEbLS3PiXXhGXo4gqV+OEzf6H/i/YMwJb2hsK+5GQrcps0XQihA7PctEb9GOMa/tu5fva0ZmaDtc94SLR1p5d4okyQFGPgtIp594HpPSEN0Qb9BrUJFeRz0VP6U3dzDPGHo7V4yyqRLgIN6EIcy1QKBgAqdh6mHPaTAHspDMyjJiYEc5cJIj/8rPkmIQft0FkhMUB0IRyAALNlyAUyeK61hW8sKvz+vPR8VEEk5xpSQp41YpuU6pDZc5YILZLfca8F+8yfQbZ/jll6Foi694efezl4yE/rUQG9cbOAJfEJt4o4TEOaEK5XoMbRBKc8pl22lAoGARTq0qOr9SStihRAy9a+8wi2WEwL4QHcmOjH7iAuJxy5b5TRDSjlk6h+0dnTItiFlTXdfpO8KhWA8EoSJVBZ1kcACQDFgMIA+VM+yXydtzMotOn21W4stfZ4I6dHFiujMsnKpNYVpQh3oCrJf4SeXiQDdiSCodqb1HlKkEc6naHQ=',
+ 'appid' => '2021000122667306',
+ // 应用私钥内容 ( 需1行填写,特别注意:这里的应用私钥通常由支付宝密钥管理工具生成 )
+ 'private_key' => 'MIIEowIBAAKCAQEAn...',
+ // 公钥模式,支付宝公钥内容 ( 需1行填写,特别注意:这里不是应用公钥而是支付宝公钥,通常是上传应用公钥换取支付宝公钥,在网页可以复制 )
+ 'public_key' => '',
+ // 证书模式,应用公钥证书路径 ( 新版资金类接口转 app_cert_sn,如文件 appCertPublicKey.crt )
+ 'app_cert_path' => __DIR__ . '/alipay/appPublicCert.crt', // 'app_cert' => '证书内容',
+ // 证书模式,支付宝根证书路径 ( 新版资金类接口转 alipay_root_cert_sn,如文件 alipayRootCert.crt )
+ 'alipay_root_path' => __DIR__ . '/alipay/alipayRootCert.crt', // 'root_cert' => '证书内容',
+ // 证书模式,支付宝公钥证书路径 ( 未填写 public_key 时启用此参数,如文件 alipayPublicCert.crt )
+ 'alipay_cert_path' => __DIR__ . '/alipay/alipayPublicCert.crt', // 'public_key' => '证书内容'
// 支付成功通知地址
- 'notify_url' => '', // 可以应用的时候配置哦
+ 'notify_url' => '',
// 网页支付回跳地址
- 'return_url' => '', // 可以应用的时候配置哦
+ 'return_url' => '',
];
```
+
* 支付宝发起PC网站支付
+
```php
// 参考公共参数 https://docs.open.alipay.com/203/107090/
$config['notify_url'] = 'http://pay.thinkadmin.top/test/alipay-notify.php';
@@ -225,7 +250,9 @@ try {
}
```
+
* 支付宝发起手机网站支付
+
```php
// 参考公共参数 https://docs.open.alipay.com/203/107090/
$config['notify_url'] = 'http://pay.thinkadmin.top/test/alipay-notify.php';
@@ -253,15 +280,17 @@ try {
}
```
+
* 更多功能请阅读测试代码或SDK封装源码
-开源协议
-----
-* WeChatDeveloper 基于`MIT`协议发布,任何人可以用在任何地方,不受约束
-* WeChatDeveloper 部分代码来自互联网,若有异议,可以联系作者进行删除
+## 版权说明
-赞助支持
-----
-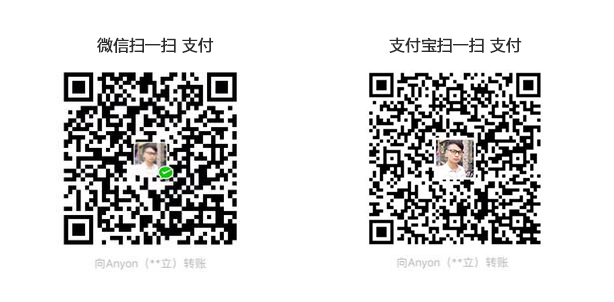
+**WeChatDeveloper** 遵循 **MIT** 开源协议发布,并免费提供使用。
+
+本项目包含的第三方源码和二进制文件的版权信息将另行标注,请在对应文件查看。
+
+版权所有 Copyright © 2014-2023 by ThinkAdmin (https://thinkadmin.top) All rights reserved。
+## 赞助打赏,请作者喝杯茶 ~
+