diff --git a/Rakefile b/Rakefile
index 96c6f942345..e3c0a51d07a 100644
--- a/Rakefile
+++ b/Rakefile
@@ -84,6 +84,7 @@ commonOptions = {
/twitter.com\/[\.\w\-\/\?]+/,
/www.optimise-it.de\/[\.\w\-\/\?]+/,
/blackfire.io\/[\.\w\-\/\?]+/,
+ /www.cdata.com/virtuality\/[\.\w\-\/\?]+/,
/dixa.com\/[\.\w\-\/\?]+/,
/rxjs.dev\/[\.\w\-\/\?]+/,
/www.blackfire.io\/[\.\w\-\/\?]+/,
@@ -96,10 +97,12 @@ commonOptions = {
/code.visualstudio.com\/[\.\w\-\/\?]+/,
/www.jetbrains.com\/[\.\w\-\/\?]+/,
/docs.spring.io\/[\.\w\-\/\?]+/,
- "http://redisdesktop.com/",
- "https://developer.computop.com/display/EN/Test+Cards",
- "https://www.centralbank.cy/",
- "https://www.facebook.com/Spryker/"
+ /redisdesktop.com\/[\.\w\-\/\?]+/,
+ /developer.computop.com/display/EN/Test+Cards\/[\.\w\-\/\?]+/,
+ /www.centralbank.cy\/[\.\w\-\/\?]+/,
+ /dashboard.algolia.com/\/[\.\w\-\/\?]+/,
+ /www.facebook.com/Spryker\/[\.\w\-\/\?]+/
+
],
:ignore_files => [],
:typhoeus => {
diff --git a/_scripts/heading_level_checker/heading_level_checker.js b/_scripts/heading_level_checker/heading_level_checker.js
new file mode 100644
index 00000000000..a226e763858
--- /dev/null
+++ b/_scripts/heading_level_checker/heading_level_checker.js
@@ -0,0 +1,136 @@
+#!/usr/bin/env node
+
+const fs = require('fs');
+const path = require('path');
+
+function fixHeadings(content) {
+ // Parse frontmatter
+ const fmMatch = content.match(/^---\n([\s\S]*?)\n---/);
+ if (!fmMatch) return { content, changes: 0 };
+
+ const frontmatter = fmMatch[1];
+ const title = frontmatter.match(/title:\s*(.*)/)?.[1];
+ if (!title) return { content: content, changes: 0 };
+
+ // Get the content after frontmatter
+ const body = content.slice(fmMatch[0].length);
+
+ // Find all heading levels in the content (excluding code blocks)
+ const lines = body.split('\n');
+ let inCodeBlock = false;
+ const headingLevels = [];
+
+ lines.forEach(line => {
+ if (line.trim().startsWith('```')) {
+ inCodeBlock = !inCodeBlock;
+ return;
+ }
+ if (inCodeBlock) return;
+
+ const headingMatch = line.match(/^(#{1,6}) /);
+ if (headingMatch) {
+ headingLevels.push(headingMatch[1].length);
+ }
+ });
+
+ if (headingLevels.length === 0) return { content: content, changes: 0 };
+
+ // Find the highest level (minimum number of #)
+ const highestLevel = Math.min(...headingLevels);
+
+ // Calculate how many levels to shift to make highest level H2
+ const levelShift = 2 - highestLevel;
+
+ // If no shift needed (highest level is already H2), return unchanged
+ if (levelShift === 0) return { content: content, changes: 0 };
+
+ // Apply the shift to all headings while preserving code blocks
+ let changes = 0;
+ inCodeBlock = false;
+ const fixedLines = lines.map(line => {
+ if (line.trim().startsWith('```')) {
+ inCodeBlock = !inCodeBlock;
+ return line;
+ }
+ if (inCodeBlock) return line;
+
+ const headingMatch = line.match(/^(#{1,6}) (.*)/);
+ if (headingMatch) {
+ const newLevel = Math.max(1, Math.min(6, headingMatch[1].length + levelShift));
+ changes++;
+ return `${'#'.repeat(newLevel)} ${headingMatch[2]}`;
+ }
+ return line;
+ });
+
+ return {
+ content: `---\n${frontmatter}\n---${fixedLines.join('\n')}`,
+ changes: changes
+ };
+}
+
+function findMarkdownFiles(dir) {
+ let results = [];
+ const files = fs.readdirSync(dir);
+
+ for (const file of files) {
+ const filePath = path.join(dir, file);
+ const stat = fs.statSync(filePath);
+
+ if (stat.isDirectory()) {
+ results = results.concat(findMarkdownFiles(filePath));
+ } else if (file.endsWith('.md')) {
+ results.push(filePath);
+ }
+ }
+
+ return results;
+}
+
+function processFile(filePath, stats) {
+ try {
+ const content = fs.readFileSync(filePath, 'utf8');
+ const result = fixHeadings(content);
+ stats.totalFiles++;
+
+ if (result.changes > 0) {
+ fs.writeFileSync(filePath, result.content);
+ console.log(`Processed ${filePath} - ${result.changes} heading(s) adjusted`);
+ stats.filesChanged++;
+ stats.totalHeadingsAdjusted += result.changes;
+ } else {
+ console.log(`Processed ${filePath} - no changes needed (headings already correct)`);
+ }
+ } catch (err) {
+ console.error(`Error processing ${filePath}:`, err);
+ }
+}
+
+// Get command line arguments
+const args = process.argv.slice(2);
+
+// If no arguments provided, process all .md files in /docs/
+let filesToProcess;
+
+if (args.length === 0) {
+ console.log('No files specified, processing all .md files in /docs/...\n');
+ filesToProcess = findMarkdownFiles('docs');
+} else {
+ filesToProcess = args;
+}
+
+// Initialize statistics
+const stats = {
+ totalFiles: 0,
+ filesChanged: 0,
+ totalHeadingsAdjusted: 0
+};
+
+// Process all files
+filesToProcess.forEach(file => processFile(file, stats));
+
+// Print detailed summary
+console.log('\nSummary:');
+console.log(`Total files checked: ${stats.totalFiles}`);
+console.log(`Files that needed changes: ${stats.filesChanged}`);
+console.log(`Total headings adjusted: ${stats.totalHeadingsAdjusted}`);
diff --git a/_scripts/heading_level_checker/run_heading_level_checker.md b/_scripts/heading_level_checker/run_heading_level_checker.md
new file mode 100644
index 00000000000..b507e34ce04
--- /dev/null
+++ b/_scripts/heading_level_checker/run_heading_level_checker.md
@@ -0,0 +1,19 @@
+The heading level checker checks and corrects headings according to the standard convention. The standard hierarchy of headings in a document body is h2>h3>h4. h1 is a document's title, which is part of the front matter. The script checks and shifts the highest-level heading to be h2. Then it shifts the other headings to follow the hierarchy.
+
+Run the checker in the `docs` folder:
+
+```bash
+node _scripts/heading_level_checker/heading_level_checker.js
+```
+
+Run the checker for a particular file or folder:
+
+```bash
+node _scripts/heading_level_checker/heading_level_checker.js {PATH_TO_FILE}
+```
+
+Example of a targeted run:
+
+```bash
+node _scripts/heading_level_checker/heading_level_checker.js docs/dg/dev/guidelines/coding-guidelines
+```
diff --git a/docs/ca/dev/configure-deployment-pipelines/customize-deployment-pipelines.md b/docs/ca/dev/configure-deployment-pipelines/customize-deployment-pipelines.md
index 09fbac20649..78c1a88970b 100644
--- a/docs/ca/dev/configure-deployment-pipelines/customize-deployment-pipelines.md
+++ b/docs/ca/dev/configure-deployment-pipelines/customize-deployment-pipelines.md
@@ -26,7 +26,7 @@ This document describes how to customize deployment pipelines.
{% endinfo_block %}
-### Adding a single command to a deployment pipeline
+## Adding a single command to a deployment pipeline
To customize the `pre-deploy` stage of a pipeline:
@@ -46,7 +46,7 @@ image:
During the next deployment, the command will be executed in the `pre-deploy` stage.
-### Adding multiple commands to a deployment pipeline via a shell script
+## Adding multiple commands to a deployment pipeline via a shell script
To add multiple commands to the `pre-deploy` stage:
@@ -70,7 +70,7 @@ Do not include the `.yml` extension of the file name in `{path_to_script}`. For
During the next deployment, the commands in the script will be executed in the `pre-deploy` stage.
-### Adding different commands for different environments and pipeline types
+## Adding different commands for different environments and pipeline types
By default, in `pre-deploy` and `post-deploy` stages, there is no possibility to run different commands for combinations of different environments and pipeline types. To do that, you can set up a custom shell script with _if statements_.
@@ -94,7 +94,7 @@ if [ "${SPRYKER_PIPELINE_TYPE}" == "destructive" ]; then
fi
```
-### Adding commands to the install stage of deployment pipelines
+## Adding commands to the install stage of deployment pipelines
To add one or more commands to the `install` stage of a deployment pipeline:
diff --git a/docs/ca/dev/performance-testing-in-staging-enivronments.md b/docs/ca/dev/performance-testing-in-staging-enivronments.md
index 6867a411678..e8ec8fa6aa2 100644
--- a/docs/ca/dev/performance-testing-in-staging-enivronments.md
+++ b/docs/ca/dev/performance-testing-in-staging-enivronments.md
@@ -18,24 +18,24 @@ If you are unable to use real data for your load tests, you can use the [test da
Based on our experience, the [Load testing tool](https://github.com/spryker-sdk/load-testing) can greatly assist you in conducting more effective load tests.
-# Load testing tool for Spryker
+## Load testing tool for Spryker
To assist in performance testing, we have a [load testing tool](https://github.com/spryker-sdk/load-testing). The tool contains predefined test scenarios that are specific to Spryker. Test runs based on Gatling.io, an open-source tool. Web UI helps to manage runs and multiple target projects are supported simultaneously.
The tool can be used as a package integrated into the Spryker project or as a standalone package.
-## What is Gatling?
+### What is Gatling?
Gatling is a powerful performance testing tool that supports HTTP, WebSocket, Server-Sent-Events, and JMS. Gatling is built on top of Akka that enables thousands of virtual users on a single machine. Akka has a message-driven architecture, and this overrides the JVM limitation of handling many threads. Virtual users are not threads but messages.
Gatling is capable of creating an immense amount of traffic from a single node, which helps obtain the most precise information during the load testing.
-## Prerequisites
+### Prerequisites
- Java 8+
- Node 10.10+
-## Including the load testing tool into an environment
+### Including the load testing tool into an environment
The purpose of this guide is to show you how to integrate Spryker's load testing tool into your environment. While the instructions here will focus on setting this up with using one of Spryker’s many available demo shops, it can also be implemented into an on-going project.
@@ -57,7 +57,7 @@ git clone https://github.com/spryker-shop/b2c-demo-shop.git ./
git clone git@github.com:spryker/docker-sdk.git docker
```
-### Integrating Gatling
+#### Integrating Gatling
With the B2C Demo Shop and Docker SDK cloned, you will need to make a few changes to integrate Gatling into your project. These changes include requiring the load testing tool with composer as well as updating the [Router module](/docs/dg/dev/upgrade-and-migrate/silex-replacement/router/router-yves.html) inside of Yves.
@@ -122,7 +122,7 @@ extensions:
...
```
-### Setting up the environment
+#### Setting up the environment
{% info_block infoBox %}
@@ -160,13 +160,13 @@ docker/sdk up --build --assets --data
You've set up your Spryker B2C Demo Shop and can now access your applications.
-### Data preparation
+#### Data preparation
With the integrations done and the environment set up, you will need to create and load the data fixtures. This is done by first generating the necessary fixtures before triggering a *publish* of all events and then running the *queue worker*. As this will be running tests for this data preparation step, this will need to be done in the [testing mode for the Docker SDK](/docs/dg/dev/sdks/the-docker-sdk/running-tests-with-the-docker-sdk.html).
These steps assume you are working from a local environment. If you are attempting to implement these changes to a production or staging environment, you will need to take separate steps to generate parity data between the load-testing tool and your cloud-based environment.
-#### Steps for using a cloud-hosted environment.
+##### Steps for using a cloud-hosted environment.
The Gatling test tool uses pre-seeded data which is used locally for both testing and generating the fixtures in the project's database. If you wish to test a production or a staging environment, there are several factors which need to be addressed.
@@ -178,7 +178,7 @@ The Gatling test tool uses pre-seeded data which is used locally for both testin
Data used for Gatling's load testing can be found in **/load-test-tool-dir/tests/_data**. Any data that you generate from your cloud-hosted environment will need to be stored here.
-##### Setting up for basic authentication.
+###### Setting up for basic authentication.
If your environment is set for `BASIC AUTH` authentication and requires a user name and password before the site can be loaded, Gatling needs additional configuration. Found within **/load-test-tool-dir/resources/scenarios/spryker/**, two files control the HTTP protocol which is used by each test within the same directory. `GlueProtocol.scala` and `YvesProtocol.scala` each have a value (`httpProtocol`) which needs an additional argument to account for this authentication mode.
@@ -195,7 +195,7 @@ val httpProtocol = http
**usernamehere** and **passwordhere** should match the username and password used for your environment's basic authentication, and not an account created within Spryker. This username and password are typically set up within your deploy file.
-##### Generating product data
+###### Generating product data
{% info_block errorBox %}
@@ -216,7 +216,7 @@ FROM `us-docker`.`spy_product_concrete_storage`;
This command parses through the JSON entry and extracts what we need. Once this information has been generated, it should be saved as `product_concrete.csv` and saved in the **/load-test-tool-dir/tests/_data** directory.
-##### Generating customer data
+###### Generating customer data
{% info_block errorBox %}
@@ -259,7 +259,7 @@ For each of these, the username is typically the email of the user that was crea
Once users have been created and access tokens generated, this information should be stored and formatted in `customer.csv` and saved in the **/load-test-tool-dir/tests/_data** directory. Make sure to put the correct information under the appropriate column name.
-#### Steps for using a local environment
+##### Steps for using a local environment
To start, entering testing mode with the following command:
@@ -289,7 +289,7 @@ console queue:worker:start -s
You should have the fixtures loaded into the databases and can now exit the CLI to install Gatling into the project.
-#### Alternative method to generate local fixtures.
+##### Alternative method to generate local fixtures.
Jenkins is the default scheduler which ships with Spryker. It is an automation service which helps to automate tasks within Spryker. If you would like an alternative way to generate fixtures for your local environment, Jenkins can be used to schedule the necessary tasks you need for the data preparation step.
@@ -340,7 +340,7 @@ While it's possible to change the Jenkins cronjobs found at **/config/Zed/cronjo
You are now done and can move on to [Installing Gatling](#installing-gatling)!
-### Installing Gatling
+#### Installing Gatling
{% info_block infoBox %}
@@ -370,7 +370,7 @@ cd vendor/spryker-sdk/load-testing
After this step has been finished, you will be able to run the Web UI and tool to perform load testing for your project on the local level.
-#### Installing Gatling as a standalone package
+##### Installing Gatling as a standalone package
It is possible for you to run Gatling as a standalone package. Fixtures and data are still needed to be generated on the project level to determine what loads to send with each test. However, as the Spryker load testing tool utilizes NPM to run a localized server for the Web UI, you can do the following to install it:
@@ -389,7 +389,7 @@ cd load-testing
This should install Gatling with Spryker's available Web UI, making it ready for load testing.
-### Running Gatling
+#### Running Gatling
To get the Web UI of the Gatling tool, run:
@@ -432,7 +432,7 @@ Tests like **CartApi** and **GuestCartApi** use an older method of the `cart` en
{% endinfo_block %}
-## Using Gatling
+### Using Gatling
In the testing tool Web UI, you can do the following:
- Create, edit, and delete instances.
@@ -444,11 +444,11 @@ You can perform all these actions from the main page:

-### Managing instances
+#### Managing instances
You can create new instances and edit or delete the existing ones.
-#### Creating an instance
+##### Creating an instance
To create an instance:
@@ -462,7 +462,7 @@ To create an instance:
Now, the new instance should appear in the navigation bar in *INSTANCES* section.
-#### Editing an instance
+##### Editing an instance
For the already available instances, you can edit Yves URL and Glue URL. Instance names cannot be edited.
To edit an instance:
@@ -474,7 +474,7 @@ To edit an instance:
Now, the instance data is updated.
-#### Deleting an instance
+##### Deleting an instance
To delete an instance:
1. In the navigation bar, click **New instance**. The *Instance* page opens.
2. Click the X sign next to the instance you want to delete:
@@ -483,7 +483,7 @@ To delete an instance:
Your instance is now deleted.
-### Running tests
+#### Running tests
To run a new load test:
@@ -502,7 +502,7 @@ To run a new load test:
That's it - your test should run now. While it runs, you see a page where logs are generated. Once the time you specified in the Duration field from step 6 elapses, the test stops, and you can view the detailed test report.
-### Viewing the test reports
+#### Viewing the test reports
On the main page, you can check what tests are currently being run as well as view the detailed log for the completed tests.
@@ -521,7 +521,7 @@ To view the reports of the completed tests, on the main page, in the *Done* sect
-## Example test: Measuring the capacity
+### Example test: Measuring the capacity
Let's consider the example of measuring the capacity with the `AddToCustomerCart` or `AddToGuestCart` test.
@@ -541,7 +541,7 @@ For the *Steady probe* test type, the following is done:
- Checking that the response time is in acceptable boundaries. [< 400ms for 90% of requests]

-## Gatling Reports
+### Gatling Reports
Gatling reports are a valuable source of information to read the performance data by providing some details about requests and response timing.
@@ -556,18 +556,18 @@ There are the following report types in Gatling:
- Response time against Global RPS
-### Indicators
+#### Indicators
This chart shows how response times are distributed among the standard ranges.
The right panel shows the number of OK/KO requests.

-### Statistics
+#### Statistics
This table shows some standard statistics such as min, max, average, standard deviation, and percentiles globally and per request.

-### Active users among time
+#### Active users among time
This chart displays the active users during the simulation: total and per scenario.
@@ -581,27 +581,27 @@ It’s computed as:
```

-### Response time distribution
+#### Response time distribution
This chart displays the distribution of response times.

-### Response time percentiles over time (OK)
+#### Response time percentiles over time (OK)
This chart displays a variety of response time percentiles over time, but only for successful requests. As failed requests can end prematurely or be caused by timeouts, they would have a drastic effect on the computation for percentiles.

-### Number of requests per second
+#### Number of requests per second
This chart displays the number of requests sent per second overtime.

-### Number of responses per second
+#### Number of responses per second
This chart displays the number of responses received per second overtime: total, successes, and failures.

-### Response time against Global RPS
+#### Response time against Global RPS
This chart shows how the response time for the given request is distributed, depending on the overall number of requests at the same time.

diff --git a/docs/dg/dev/backend-development/data-manipulation/data-publishing/publish-and-synchronization.md b/docs/dg/dev/backend-development/data-manipulation/data-publishing/publish-and-synchronization.md
index a7697c00242..0c46e5ce706 100644
--- a/docs/dg/dev/backend-development/data-manipulation/data-publishing/publish-and-synchronization.md
+++ b/docs/dg/dev/backend-development/data-manipulation/data-publishing/publish-and-synchronization.md
@@ -53,11 +53,11 @@ Both Publish and Synchronize implement the queue pattern. See [Spryker Queue
The process relies heavily on Propel behaviors, which are used to trigger actions automatically when updating the database. Thus, you don't need to trigger any step of the process in the code manually. See [Boostrapping a Behavior](http://propelorm.org/documentation/cookbook/writing-behavior.html) to learn more.
-### Triggering the Publish process
+## Triggering the Publish process
There are 2 ways to start the Publish process: automated and manual.
-#### Automated event emitting
+### Automated event emitting
Any changes done to an entity implementing the _event_ Propel behavior triggers a publish event immediately. CUD (create, update, delete) operations are covered by this Propel behavior. So you can expect these three types of events on creation, update, and deletion of DB entities managed by Propel ORM.
@@ -71,7 +71,7 @@ $productAbstractEntity->save();
Implementing event behaviors is recommended for your project features to keep the Shop App data up-to-date. For example, behaviors are widely used in the `Availability` module to inform customers whether a certain product is available for purchase.
-#### Manual event emitting
+### Manual event emitting
You can trigger the publish event manually using the [Event Facade](/docs/dg/dev/backend-development/data-manipulation/event/add-events.html):
@@ -81,12 +81,12 @@ $this->eventFacade->trigger(CmsStorageConfig::CMS_KEY_PUBLISH_WRITE, (new EventE
Manual even emitting is best suited when an entity passes several stages before becoming available to a customer. A typical use case for this method is content management. In most cases, a page does not become available once you create it. Usually, it exists as a draft to be published later. For example, when a new product is released to the market.
-### How Publish and Synchronize works
+## How Publish and Synchronize works
Publish and Synchronize Process schema:
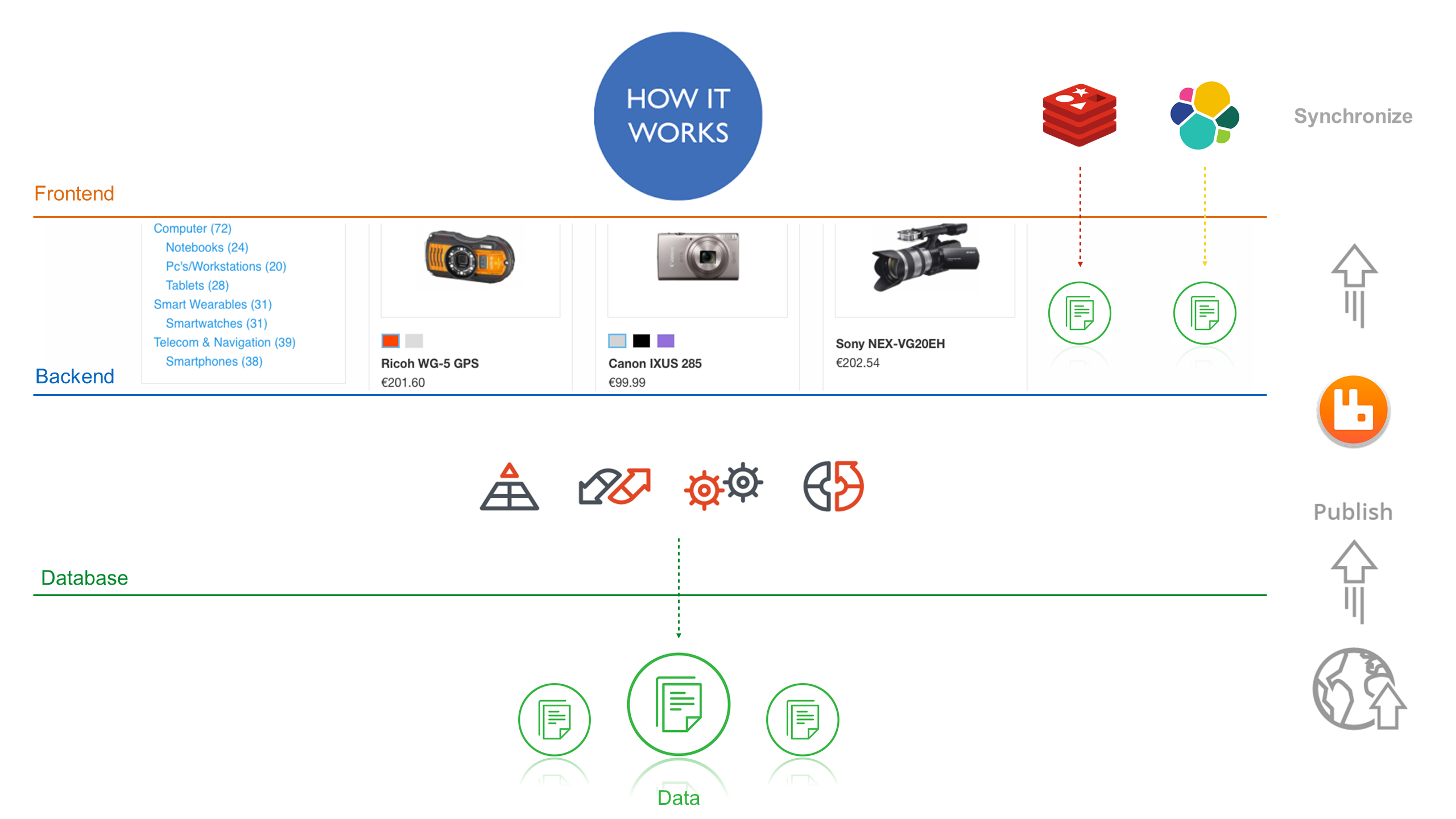
-### Publish
+## Publish
When the publish process is triggered, an event or events are posted to a queue. Each event message posted to the queue contains the following information on the event that triggered it:
* Event name
@@ -128,7 +128,7 @@ To consume an event, the queue adapter calls the publisher plugin specified in t
The transformed data is stored in a dedicated database table. It serves as a _mirror table_ for the respective Redis or Elasticsearch storage. The `data` column of the table contains the data to be synced to the front end, defining [the storage and the key](/docs/dg/dev/backend-development/data-manipulation/data-publishing/handle-data-with-publish-and-synchronization.html). It is stored in JSON for easy and fast synchronization. The table also contains the foreign keys used to backtrack data and the timestamp of the last change for each row. The timestamp is used to track changes rapidly.
-### Synchronize
+## Synchronize
When a change happens in the mirror table, its *synchronization behavior* sends the updated rows as messages to one of the Sync Queues. After consuming a message, the data is pushed to Redis or Elastisearch.
@@ -178,7 +178,7 @@ When a change happens in the mirror table, its *synchronization behavior* sends
}
```
-#### Direct synchronize
+### Direct synchronize
To optimize performance and flexibility, you can enable direct synchronization on the project level. This approach uses in-memory storage to retain all synchronization events instead of sending them to the queue. With this setup, you can control if entities are synchronized directly or through the traditional queue-based method.
@@ -241,7 +241,7 @@ class SynchronizationBehaviorConfig extends SprykerSynchronizationBehaviorConfig
```
-#### Environment limitations related to DMS
+### Environment limitations related to DMS
When Dynamic Multi-Store (DMS) is disabled, the Direct Sync feature has the following limitations:
- Single-store configuration: The feature is only supported for configurations with a single store.
@@ -272,7 +272,7 @@ stores:
When DMS is enabled, there're no environment limitations for the Direct Sync feature.
-### Data Architecture
+## Data Architecture
P&S plays a major role in data denormalization and distribution to Spryker storefronts and API. Denormalization procedure aims for preparing data in the form it will be consumed by data clients. Distribution procedure aims to distribute the data closer to the end users, so that they feel like accessing a local storage.
@@ -290,7 +290,7 @@ When designing a solution using P&S we need to consider the following concerns i
- horizontal scalability of publish process (native) and sync process (requires development)
- data object limitations
-#### Data Object Limitations
+### Data Object Limitations
In order to build a healthy commerce system, we need to make sure that P&S process is healthy at all times. And first we start with healthy NFRs for P&S.
- storage sync message size should not be over 256Kb - this prevents us from problems in data processing, but even more important in data comsumption, when an API consumer might experience failure when reviceing an aggregated object of a high size.
diff --git a/docs/dg/dev/backend-development/zed/persistence-layer/query-container/using-a-query-container.md b/docs/dg/dev/backend-development/zed/persistence-layer/query-container/using-a-query-container.md
index 0cb4db03069..661d9cd8d40 100644
--- a/docs/dg/dev/backend-development/zed/persistence-layer/query-container/using-a-query-container.md
+++ b/docs/dg/dev/backend-development/zed/persistence-layer/query-container/using-a-query-container.md
@@ -25,7 +25,7 @@ The query container of the current unterminated query is available via `$this->g
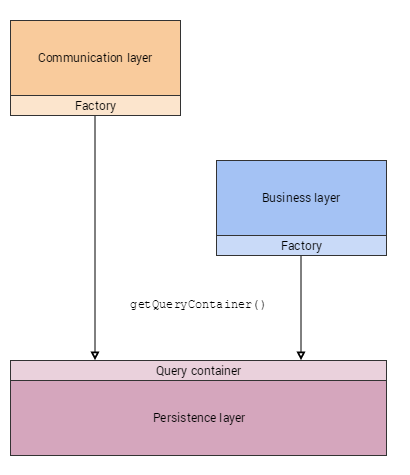
-### Executing the query
+## Executing the query
You can adjust the query itself, but avoid adding more filters or joins because this is the responsibility of the query container only.
diff --git a/docs/dg/dev/glue-api/202311.0/document-glue-api-endpoints.md b/docs/dg/dev/glue-api/202311.0/document-glue-api-endpoints.md
index 0d532a49b7a..4fcb06b782c 100644
--- a/docs/dg/dev/glue-api/202311.0/document-glue-api-endpoints.md
+++ b/docs/dg/dev/glue-api/202311.0/document-glue-api-endpoints.md
@@ -145,7 +145,7 @@ The following table lists descriptions of the properties you can use in the anno
| `isEmptyResponse` | The flag used to mark an endpoint that returns empty responses. |
| `responses` | A list of possible responses of the endpoint. The object must contain key-value pairs with HTTP codes as key, and a description as the value. |
-### Extending the behavior
+## Extending the behavior
The following interfaces can be used to add more data to the generated documentation.
diff --git a/docs/dg/dev/glue-api/202404.0/document-glue-api-endpoints.md b/docs/dg/dev/glue-api/202404.0/document-glue-api-endpoints.md
index 4862d85b370..f64ebb83c68 100644
--- a/docs/dg/dev/glue-api/202404.0/document-glue-api-endpoints.md
+++ b/docs/dg/dev/glue-api/202404.0/document-glue-api-endpoints.md
@@ -145,7 +145,7 @@ The following table lists descriptions of the properties you can use in the anno
| `isEmptyResponse` | The flag used to mark an endpoint that returns empty responses. |
| `responses` | A list of possible responses of the endpoint. The object must contain key-value pairs with HTTP codes as key, and a description as the value. |
-### Extending the behavior
+## Extending the behavior
The following interfaces can be used to add more data to the generated documentation.
diff --git a/docs/dg/dev/glue-api/202410.0/document-glue-api-endpoints.md b/docs/dg/dev/glue-api/202410.0/document-glue-api-endpoints.md
index 4862d85b370..f64ebb83c68 100644
--- a/docs/dg/dev/glue-api/202410.0/document-glue-api-endpoints.md
+++ b/docs/dg/dev/glue-api/202410.0/document-glue-api-endpoints.md
@@ -145,7 +145,7 @@ The following table lists descriptions of the properties you can use in the anno
| `isEmptyResponse` | The flag used to mark an endpoint that returns empty responses. |
| `responses` | A list of possible responses of the endpoint. The object must contain key-value pairs with HTTP codes as key, and a description as the value. |
-### Extending the behavior
+## Extending the behavior
The following interfaces can be used to add more data to the generated documentation.
diff --git a/docs/dg/dev/guidelines/testing-guidelines/test-helpers/test-helpers-best-practices.md b/docs/dg/dev/guidelines/testing-guidelines/test-helpers/test-helpers-best-practices.md
index 545954b874a..e90495f3319 100644
--- a/docs/dg/dev/guidelines/testing-guidelines/test-helpers/test-helpers-best-practices.md
+++ b/docs/dg/dev/guidelines/testing-guidelines/test-helpers/test-helpers-best-practices.md
@@ -14,11 +14,11 @@ You should organize helpers according to their specific roles and intended use c
During the "Act" stage, we often only invoke a specific method that requires testing. In certain situations, it might make sense to create a helper method for executing bigger processes, such as `Helper::checkout()`. This method could include actions like adding a product to the cart, initiating the checkout procedure, completing address forms, selecting payment options, and more. This helper method could then also be reused by other tests.
-### Root helper
+## Root helper
Each module should have a helper named after the module. For example, in the `spryker/customer` module, there is `CustomerHelper`. This particular helper facilitates interactions with customer-related functionalities, such as creating `CustomerTransfer` or registering a customer.
You can also use these helpers to configure the system in a manner where, for example, an in-memory message broker is used instead a remote or a locally running one. This switch simplifies testing procedures and enhances overall performance.
-### Assert helper
+## Assert helper
To prevent overburdening the root helper, specific assertions should reside in separate helpers. In the context of the Customer module example, you could establish a CustomerAssertHelper. This dedicated helper should exclusively encompass assertion methods that can be used in any other module as well.
diff --git a/docs/dg/dev/integrate-and-configure/Integrate-profiler-module.md b/docs/dg/dev/integrate-and-configure/Integrate-profiler-module.md
index 0745bd4a5af..fa59cb1ab22 100644
--- a/docs/dg/dev/integrate-and-configure/Integrate-profiler-module.md
+++ b/docs/dg/dev/integrate-and-configure/Integrate-profiler-module.md
@@ -9,7 +9,7 @@ redirect_from:
This document describes how to integrate profiler module into a Spryker project.
-## Prerequisites
+### Prerequisites
To start the integration, install the necessary features:
@@ -19,7 +19,7 @@ To start the integration, install the necessary features:
| Web Profiler for Zed | {{page.version}} | [Web Profiler feature integration](/docs/dg/dev/integrate-and-configure/integrate-development-tools/integrate-web-profiler-for-zed.html) |
| Web Profiler for Yves | {{page.version}} | [Web Profiler feature integration](/docs/dg/dev/integrate-and-configure/integrate-development-tools/integrate-web-profiler-widget-for-yves.html) |
-## 1) Enable extension
+### 1) Enable extension
To collect execution traces, enable the `xhprof` extension.
@@ -37,13 +37,13 @@ image:
- xhprof
```
-## 2) Bootstrap the Docker setup
+### 2) Bootstrap the Docker setup
```shell
docker/sdk boot deploy.dev.yml
```
-## 3) Install the required modules using Composer
+### 3) Install the required modules using Composer
{% info_block warningBox "Verification" %}
@@ -60,7 +60,7 @@ Ensure that the following modules have been updated:
composer require --dev spryker/profiler --ignore-platform-reqs
```
-### Set up behavior
+#### Set up behavior
1. For `Yves` application, register the following plugins:
@@ -191,12 +191,12 @@ class WebProfilerDependencyProvider extends SprykerWebProfilerDependencyProvider
}
```
-# Generate transfers
+## Generate transfers
Run the `console transfer:generate` command to generate all the necessary transfer objects.
-# Enable the configuration
+## Enable the configuration
Module Profile works as a part of Web Profiler feature. By default, Web Profiler is disabled.
To enable Web Profiler, please update `config/Shared/config_default-docker.dev.php` configuration file.
diff --git a/docs/dg/dev/integrate-and-configure/integrate-development-tools/integrate-scss-linter.md b/docs/dg/dev/integrate-and-configure/integrate-development-tools/integrate-scss-linter.md
index 85bfb87aa3a..6dff49db92a 100644
--- a/docs/dg/dev/integrate-and-configure/integrate-development-tools/integrate-scss-linter.md
+++ b/docs/dg/dev/integrate-and-configure/integrate-development-tools/integrate-scss-linter.md
@@ -19,7 +19,7 @@ related:
link: docs/scos/dev/technical-enhancement-integration-guides/integrating-development-tools/integrating-web-profiler-widget-for-yves.html
---
-Follow the steps below to integrate the [SCSS linter ](/docs/scos/dev/sdk/development-tools/scss-linter.html)into your project.
+Follow the steps below to integrate the [SCSS linter ](/docs/dg/dev/sdks/sdk/development-tools/scss-linter.html)into your project.
## 1. Install the dependencies
diff --git a/docs/dg/dev/integrate-and-configure/integrate-development-tools/integrate-ts-linter.md b/docs/dg/dev/integrate-and-configure/integrate-development-tools/integrate-ts-linter.md
index b3bf73bebd5..a09125e90ac 100644
--- a/docs/dg/dev/integrate-and-configure/integrate-development-tools/integrate-ts-linter.md
+++ b/docs/dg/dev/integrate-and-configure/integrate-development-tools/integrate-ts-linter.md
@@ -19,7 +19,7 @@ related:
link: docs/scos/dev/technical-enhancement-integration-guides/integrating-development-tools/integrating-web-profiler-widget-for-yves.html
---
-Follow the steps below to integrate [TS linter](/docs/scos/dev/sdk/development-tools/ts-linter.html) into your project.
+Follow the steps below to integrate [TS linter](/docs/dg/dev/sdks/sdk/development-tools/ts-linter.html) into your project.
## 1. Install the dependencies
diff --git a/docs/dg/dev/integrate-and-configure/integrate-separate-endpoint-bootstraps.md b/docs/dg/dev/integrate-and-configure/integrate-separate-endpoint-bootstraps.md
index 6b38eadac7d..74581e0aa52 100644
--- a/docs/dg/dev/integrate-and-configure/integrate-separate-endpoint-bootstraps.md
+++ b/docs/dg/dev/integrate-and-configure/integrate-separate-endpoint-bootstraps.md
@@ -13,7 +13,7 @@ Gateway and ZedRestApi requests require a different stack of plugins to be proce
To separate application bootstrapping into individual endpoints, take the following steps:
-### 1) Update modules using Composer
+## 1) Update modules using Composer
Update the required modules:
@@ -34,7 +34,7 @@ Update the required modules:
composer update spryker/twig spryker/session spryker/router spryker/monitoring spryker/event-dispatcher spryker/application
```
-### 2) Update modules using npm
+## 2) Update modules using npm
Update the required module:
@@ -64,7 +64,7 @@ npm install
npm run zed
```
-### 3) Add application entry points
+## 3) Add application entry points
1. Add the following application entry points:
@@ -299,7 +299,7 @@ The maintenance page is not yet compatible with Spryker Cloud.
}
```
-### 4) Separate application plugin stacks
+## 4) Separate application plugin stacks
1. Replace `ApplicationDependencyProvider::getApplicationPlugins();` with separate plugin stacks per endpoint:
@@ -378,7 +378,7 @@ class ApplicationDependencyProvider extends SprykerApplicationDependencyProvider
```
-### 5) Separate event dispatcher plugin stacks
+## 5) Separate event dispatcher plugin stacks
Update `src/Pyz/Zed/EventDispatcher/EventDispatcherDependencyProvider.php` with the following changes:
@@ -422,7 +422,7 @@ class EventDispatcherDependencyProvider extends SprykerEventDispatcherDependency
}
```
-### 6) Separate router plugin stacks
+## 6) Separate router plugin stacks
Replace `RouterDependencyProvider::getRouterPlugins();` with two new methods:
@@ -455,7 +455,7 @@ class RouterDependencyProvider extends SprykerRouterDependencyProvider
}
```
-### 7) Add console commands
+## 7) Add console commands
1. Configure the following console commands with a router cache warmup per endpoint:
@@ -514,7 +514,7 @@ sections:
...
```
-### 8) Configure the application
+## 8) Configure the application
1. Configure the Back Office error page, default port, and the ACL rule for the rest endpoint:
@@ -589,7 +589,7 @@ class SecurityGuiConfig extends SprykerSecurityGuiConfig
Make sure to do this at least 5 working days prior to the planned change.
-### 9) Update the Docker SDK
+## 9) Update the Docker SDK
1. Update the Docker SDK to version `1.36.1` or higher.
2. In the needed deploy files, replace the `zed` application with `backoffice`, `backend-gateway` and `backend-api` as follows.
diff --git a/docs/dg/dev/sdks/sdk/build-flavored-spryker-sdks.md b/docs/dg/dev/sdks/sdk/build-flavored-spryker-sdks.md
index 1149d7d499c..477484ea626 100644
--- a/docs/dg/dev/sdks/sdk/build-flavored-spryker-sdks.md
+++ b/docs/dg/dev/sdks/sdk/build-flavored-spryker-sdks.md
@@ -1,6 +1,6 @@
---
title: Build flavored Spryker SDKs
-description: Find out how you can build flavored Spryker SDKs
+description: Find out how you can build flavored Spryker SDKs with extension or deep integration of the SDK within your Spryker projects.
template: howto-guide-template
last_updated: Nov 22, 2022
redirect_from:
diff --git a/docs/dg/dev/sdks/sdk/developing-with-spryker-sdk.md b/docs/dg/dev/sdks/sdk/developing-with-spryker-sdk.md
index 5056899df2f..f53df70550d 100644
--- a/docs/dg/dev/sdks/sdk/developing-with-spryker-sdk.md
+++ b/docs/dg/dev/sdks/sdk/developing-with-spryker-sdk.md
@@ -1,6 +1,6 @@
---
title: Developing with Spryker SDK
-description: You can install and use Spryker SDK for your development needs
+description: Learn how you can install and use Spryker SDK for your development needs with the dev enironment.
template: howto-guide-template
last_updated: Nov 22, 2022
related:
diff --git a/docs/dg/dev/sdks/sdk/development-tools/architecture-sniffer.md b/docs/dg/dev/sdks/sdk/development-tools/architecture-sniffer.md
index 706f6d09213..9a474c1595d 100644
--- a/docs/dg/dev/sdks/sdk/development-tools/architecture-sniffer.md
+++ b/docs/dg/dev/sdks/sdk/development-tools/architecture-sniffer.md
@@ -18,23 +18,23 @@ redirect_from:
- /docs/scos/dev/sdk/development-tools/architecture-sniffer.html
related:
- title: Code sniffer
- link: docs/scos/dev/sdk/development-tools/code-sniffer.html
+ link: docs/dg/dev/sdks/sdk/development-tools/code-sniffer.html
- title: Formatter
- link: docs/scos/dev/sdk/development-tools/formatter.html
+ link: docs/dg/dev/sdks/sdk/development-tools/formatter.html
- title: Performance audit tool- Benchmark
- link: docs/scos/dev/sdk/development-tools/performance-audit-tool-benchmark.html
+ link: docs/dg/dev/sdks/sdk/development-tools/benchmark-performance-audit-tool.html
- title: PHPStan
link: docs/dg/dev/sdks/sdk/development-tools/phpstan.html
- title: SCSS linter
- link: docs/scos/dev/sdk/development-tools/scss-linter.html
+ link: docs/dg/dev/sdks/sdk/development-tools/scss-linter.html
- title: TS linter
- link: docs/scos/dev/sdk/development-tools/ts-linter.html
+ link: docs/dg/dev/sdks/sdk/development-tools/ts-linter.html
- title: Spryk code generator
- link: docs/scos/dev/sdk/development-tools/spryk-code-generator.html
+ link: docs/dg/dev/sdks/sdk/spryks/spryks.html
- title: Static Security Checker
- link: docs/scos/dev/sdk/development-tools/static-security-checker.html
+ link: docs/dg/dev/sdks/sdk/development-tools/static-security-checker.html
- title: Tooling config file
- link: docs/scos/dev/sdk/development-tools/tooling-config-file.html
+ link: docs/dg/dev/sdks/sdk/development-tools/tooling-configuration-file.html
---
We use our [Architecture Sniffer Tool](https://github.com/spryker/architecture-sniffer) to ensure the quality of Spryker architecture for both core and project.
diff --git a/docs/dg/dev/sdks/sdk/development-tools/benchmark-performance-audit-tool.md b/docs/dg/dev/sdks/sdk/development-tools/benchmark-performance-audit-tool.md
index 8092730d514..a18b5a06fea 100644
--- a/docs/dg/dev/sdks/sdk/development-tools/benchmark-performance-audit-tool.md
+++ b/docs/dg/dev/sdks/sdk/development-tools/benchmark-performance-audit-tool.md
@@ -1,6 +1,6 @@
---
title: "Benchmark: Performance audit tool"
-description: The Benchmark tool allows you to assess an application's performance by seeing how long it takes to load a page and how much memory the application consumes during requests.
+description: The Benchmark tool allows you to assess an application's performance by how long it takes to load a page and how much memory the it consumes during requests.
last_updated: Jun 16, 2021
template: concept-topic-template
originalLink: https://documentation.spryker.com/2021080/docs/performance-audit-tool-benchmark
diff --git a/docs/dg/dev/sdks/sdk/development-tools/code-sniffer.md b/docs/dg/dev/sdks/sdk/development-tools/code-sniffer.md
index 660edbdc68d..005dc13c849 100644
--- a/docs/dg/dev/sdks/sdk/development-tools/code-sniffer.md
+++ b/docs/dg/dev/sdks/sdk/development-tools/code-sniffer.md
@@ -17,23 +17,23 @@ redirect_from:
- /docs/scos/dev/sdk/development-tools/code-sniffer.html
related:
- title: Architecture sniffer
- link: docs/scos/dev/sdk/development-tools/architecture-sniffer.html
+ link: docs/dg/dev/sdks/sdk/development-tools/architecture-sniffer.html
- title: Formatter
- link: docs/scos/dev/sdk/development-tools/formatter.html
+ link: docs/dg/dev/sdks/sdk/development-tools/formatter.html
- title: Performance audit tool- Benchmark
- link: docs/scos/dev/sdk/development-tools/performance-audit-tool-benchmark.html
+ link: docs/dg/dev/sdks/sdk/development-tools/benchmark-performance-audit-tool.html
- title: PHPStan
link: docs/dg/dev/sdks/sdk/development-tools/phpstan.html
- title: SCSS linter
- link: docs/scos/dev/sdk/development-tools/scss-linter.html
+ link: docs/dg/dev/sdks/sdk/development-tools/scss-linter.html
- title: TS linter
- link: docs/scos/dev/sdk/development-tools/ts-linter.html
+ link: docs/dg/dev/sdks/sdk/development-tools/ts-linter.html
- title: Spryk code generator
- link: docs/scos/dev/sdk/development-tools/spryk-code-generator.html
+ link: docs/dg/dev/sdks/sdk/spryks/spryks.html
- title: Static Security Checker
- link: docs/scos/dev/sdk/development-tools/static-security-checker.html
+ link: docs/dg/dev/sdks/sdk/development-tools/static-security-checker.html
- title: Tooling config file
- link: docs/scos/dev/sdk/development-tools/tooling-config-file.html
+ link: docs/dg/dev/sdks/sdk/development-tools/tooling-configuration-file.html
---
To correspond to [PSR-2](http://www.php-fig.org/psr/psr-2/) and additional standards, we integrated the well known [PHPCodeSniffer](https://github.com/squizlabs/PHP_CodeSniffer).
diff --git a/docs/dg/dev/sdks/sdk/development-tools/development-tools.md b/docs/dg/dev/sdks/sdk/development-tools/development-tools.md
index 74375689af5..86c682fe700 100644
--- a/docs/dg/dev/sdks/sdk/development-tools/development-tools.md
+++ b/docs/dg/dev/sdks/sdk/development-tools/development-tools.md
@@ -1,6 +1,6 @@
---
title: Development tools
-description: How to use the tools associated with developing using the Spryker SDK
+description: Learn how you can use the tools associated with developing with Spryker using the Spryker SDK
last_updated: Jan 12, 2023
template: concept-topic-template
redirect_from:
diff --git a/docs/dg/dev/sdks/sdk/development-tools/formatter.md b/docs/dg/dev/sdks/sdk/development-tools/formatter.md
index cb54da3d9b7..48829511935 100644
--- a/docs/dg/dev/sdks/sdk/development-tools/formatter.md
+++ b/docs/dg/dev/sdks/sdk/development-tools/formatter.md
@@ -16,24 +16,24 @@ redirect_from:
- /docs/scos/dev/sdk/202108.0/development-tools/formatter.html
- /docs/scos/dev/sdk/development-tools/formatter.html
related:
- - title: Architecture sniffer
- link: docs/scos/dev/sdk/development-tools/architecture-sniffer.html
- title: Code sniffer
- link: docs/scos/dev/sdk/development-tools/code-sniffer.html
+ link: docs/dg/dev/sdks/sdk/development-tools/code-sniffer.html
+ - title: Architecture sniffer
+ link: docs/dg/dev/sdks/sdk/development-tools/architecture-sniffer.html
- title: Performance audit tool- Benchmark
- link: docs/scos/dev/sdk/development-tools/performance-audit-tool-benchmark.html
+ link: docs/dg/dev/sdks/sdk/development-tools/benchmark-performance-audit-tool.html
- title: PHPStan
link: docs/dg/dev/sdks/sdk/development-tools/phpstan.html
- title: SCSS linter
- link: docs/scos/dev/sdk/development-tools/scss-linter.html
+ link: docs/dg/dev/sdks/sdk/development-tools/scss-linter.html
- title: TS linter
- link: docs/scos/dev/sdk/development-tools/ts-linter.html
+ link: docs/dg/dev/sdks/sdk/development-tools/ts-linter.html
- title: Spryk code generator
- link: docs/scos/dev/sdk/development-tools/spryk-code-generator.html
+ link: docs/dg/dev/sdks/sdk/spryks/spryks.html
- title: Static Security Checker
- link: docs/scos/dev/sdk/development-tools/static-security-checker.html
+ link: docs/dg/dev/sdks/sdk/development-tools/static-security-checker.html
- title: Tooling config file
- link: docs/scos/dev/sdk/development-tools/tooling-config-file.html
+ link: docs/dg/dev/sdks/sdk/development-tools/tooling-configuration-file.html
---
*Formatter* allows you to find and fix code style mistakes and keep your code more readable.
diff --git a/docs/dg/dev/sdks/sdk/development-tools/phpstan.md b/docs/dg/dev/sdks/sdk/development-tools/phpstan.md
index d65475e777f..15b317c9bce 100644
--- a/docs/dg/dev/sdks/sdk/development-tools/phpstan.md
+++ b/docs/dg/dev/sdks/sdk/development-tools/phpstan.md
@@ -1,6 +1,6 @@
---
title: PHPStan
-description: Learn how to install and use PHPStan, a static code analyzer
+description: Learn how to install and use PHPStan, a static code analyzer within your Spryker SDK projects.
last_updated: Jun 16, 2021
template: concept-topic-template
originalLink: https://documentation.spryker.com/2021080/docs/phpstan
@@ -17,23 +17,21 @@ redirect_from:
- /docs/scos/dev/sdk/development-tools/phpstan.html
related:
- title: Architecture sniffer
- link: docs/scos/dev/sdk/development-tools/architecture-sniffer.html
- - title: Code sniffer
- link: docs/scos/dev/sdk/development-tools/code-sniffer.html
+ link: docs/dg/dev/sdks/sdk/development-tools/architecture-sniffer.html
- title: Formatter
- link: docs/scos/dev/sdk/development-tools/formatter.html
+ link: docs/dg/dev/sdks/sdk/development-tools/formatter.html
- title: Performance audit tool- Benchmark
- link: docs/scos/dev/sdk/development-tools/performance-audit-tool-benchmark.html
+ link: docs/dg/dev/sdks/sdk/development-tools/benchmark-performance-audit-tool.html
- title: SCSS linter
- link: docs/scos/dev/sdk/development-tools/scss-linter.html
+ link: docs/dg/dev/sdks/sdk/development-tools/scss-linter.html
- title: TS linter
- link: docs/scos/dev/sdk/development-tools/ts-linter.html
+ link: docs/dg/dev/sdks/sdk/development-tools/ts-linter.html
- title: Spryk code generator
- link: docs/scos/dev/sdk/development-tools/spryk-code-generator.html
+ link: docs/dg/dev/sdks/sdk/spryks/spryks.html
- title: Static Security Checker
- link: docs/scos/dev/sdk/development-tools/static-security-checker.html
+ link: docs/dg/dev/sdks/sdk/development-tools/static-security-checker.html
- title: Tooling config file
- link: docs/scos/dev/sdk/development-tools/tooling-config-file.html
+ link: docs/dg/dev/sdks/sdk/development-tools/tooling-configuration-file.html
---
[PHPStan](https://github.com/phpstan/phpstan) is a static code analyzer that introspects the code without running it and catches various classes of bugs prior to unit testing.
diff --git a/docs/dg/dev/sdks/sdk/development-tools/scss-linter.md b/docs/dg/dev/sdks/sdk/development-tools/scss-linter.md
index 32261780f7c..000d2ade0c0 100644
--- a/docs/dg/dev/sdks/sdk/development-tools/scss-linter.md
+++ b/docs/dg/dev/sdks/sdk/development-tools/scss-linter.md
@@ -16,24 +16,24 @@ redirect_from:
- /docs/scos/dev/sdk/202108.0/development-tools/scss-linter.html
- /docs/scos/dev/sdk/development-tools/scss-linter.html
related:
- - title: Architecture sniffer
- link: docs/scos/dev/sdk/development-tools/architecture-sniffer.html
- title: Code sniffer
- link: docs/scos/dev/sdk/development-tools/code-sniffer.html
+ link: docs/dg/dev/sdks/sdk/development-tools/code-sniffer.html
- title: Formatter
- link: docs/scos/dev/sdk/development-tools/formatter.html
+ link: docs/dg/dev/sdks/sdk/development-tools/formatter.html
+ - title: Architecture sniffer
+ link: docs/dg/dev/sdks/sdk/development-tools/architecture-sniffer.html
- title: Performance audit tool- Benchmark
- link: docs/scos/dev/sdk/development-tools/performance-audit-tool-benchmark.html
+ link: docs/dg/dev/sdks/sdk/development-tools/benchmark-performance-audit-tool.html
- title: PHPStan
link: docs/dg/dev/sdks/sdk/development-tools/phpstan.html
- title: TS linter
- link: docs/scos/dev/sdk/development-tools/ts-linter.html
+ link: docs/dg/dev/sdks/sdk/development-tools/ts-linter.html
- title: Spryk code generator
- link: docs/scos/dev/sdk/development-tools/spryk-code-generator.html
+ link: docs/dg/dev/sdks/sdk/spryks/spryks.html
- title: Static Security Checker
- link: docs/scos/dev/sdk/development-tools/static-security-checker.html
+ link: docs/dg/dev/sdks/sdk/development-tools/static-security-checker.html
- title: Tooling config file
- link: docs/scos/dev/sdk/development-tools/tooling-config-file.html
+ link: docs/dg/dev/sdks/sdk/development-tools/tooling-configuration-file.html
---
*SCSS linter* allows you to find and fix code style mistakes. It helps a team follow the same standards and make code more readable.
diff --git a/docs/dg/dev/sdks/sdk/development-tools/static-security-checker.md b/docs/dg/dev/sdks/sdk/development-tools/static-security-checker.md
index ce1705dd665..515b2fe7560 100644
--- a/docs/dg/dev/sdks/sdk/development-tools/static-security-checker.md
+++ b/docs/dg/dev/sdks/sdk/development-tools/static-security-checker.md
@@ -16,24 +16,24 @@ redirect_from:
- /docs/scos/dev/sdk/202108.0/development-tools/static-security-checker.html
- /docs/scos/dev/sdk/development-tools/static-security-checker.html
related:
- - title: Architecture sniffer
- link: docs/scos/dev/sdk/development-tools/architecture-sniffer.html
- title: Code sniffer
- link: docs/scos/dev/sdk/development-tools/code-sniffer.html
+ link: docs/dg/dev/sdks/sdk/development-tools/code-sniffer.html
- title: Formatter
- link: docs/scos/dev/sdk/development-tools/formatter.html
+ link: docs/dg/dev/sdks/sdk/development-tools/formatter.html
+ - title: Architecture sniffer
+ link: docs/dg/dev/sdks/sdk/development-tools/architecture-sniffer.html
- title: Performance audit tool- Benchmark
- link: docs/scos/dev/sdk/development-tools/performance-audit-tool-benchmark.html
+ link: docs/dg/dev/sdks/sdk/development-tools/benchmark-performance-audit-tool.html
- title: PHPStan
link: docs/dg/dev/sdks/sdk/development-tools/phpstan.html
- title: SCSS linter
- link: docs/scos/dev/sdk/development-tools/scss-linter.html
+ link: docs/dg/dev/sdks/sdk/development-tools/scss-linter.html
- title: TS linter
- link: docs/scos/dev/sdk/development-tools/ts-linter.html
+ link: docs/dg/dev/sdks/sdk/development-tools/ts-linter.html
- title: Spryk code generator
- link: docs/scos/dev/sdk/development-tools/spryk-code-generator.html
+ link: docs/dg/dev/sdks/sdk/spryks/spryks.html
- title: Tooling config file
- link: docs/scos/dev/sdk/development-tools/tooling-config-file.html
+ link: docs/dg/dev/sdks/sdk/development-tools/tooling-configuration-file.html
---
The Spryker static Security Checker allows you to detect packages with security vulnerabilities. It is based on the [Local PHP Security Checker](https://github.com/fabpot/local-php-security-checker).
diff --git a/docs/dg/dev/sdks/sdk/development-tools/tooling-configuration-file.md b/docs/dg/dev/sdks/sdk/development-tools/tooling-configuration-file.md
index d59b1f9fdb6..6e0b027aba0 100644
--- a/docs/dg/dev/sdks/sdk/development-tools/tooling-configuration-file.md
+++ b/docs/dg/dev/sdks/sdk/development-tools/tooling-configuration-file.md
@@ -16,24 +16,24 @@ redirect_from:
- /docs/scos/dev/sdk/202108.0/development-tools/tooling-config-file.html
- /docs/scos/dev/sdk/development-tools/tooling-config-file.html
related:
- - title: Architecture sniffer
- link: docs/scos/dev/sdk/development-tools/architecture-sniffer.html
- title: Code sniffer
- link: docs/scos/dev/sdk/development-tools/code-sniffer.html
+ link: docs/dg/dev/sdks/sdk/development-tools/code-sniffer.html
- title: Formatter
- link: docs/scos/dev/sdk/development-tools/formatter.html
+ link: docs/dg/dev/sdks/sdk/development-tools/formatter.html
+ - title: Architecture sniffer
+ link: docs/dg/dev/sdks/sdk/development-tools/architecture-sniffer.html
- title: Performance audit tool- Benchmark
- link: docs/scos/dev/sdk/development-tools/performance-audit-tool-benchmark.html
+ link: docs/dg/dev/sdks/sdk/development-tools/benchmark-performance-audit-tool.html
- title: PHPStan
link: docs/dg/dev/sdks/sdk/development-tools/phpstan.html
- title: SCSS linter
- link: docs/scos/dev/sdk/development-tools/scss-linter.html
+ link: docs/dg/dev/sdks/sdk/development-tools/scss-linter.html
- title: TS linter
- link: docs/scos/dev/sdk/development-tools/ts-linter.html
+ link: docs/dg/dev/sdks/sdk/development-tools/ts-linter.html
- title: Spryk code generator
- link: docs/scos/dev/sdk/development-tools/spryk-code-generator.html
+ link: docs/dg/dev/sdks/sdk/spryks/spryks.html
- title: Static Security Checker
- link: docs/scos/dev/sdk/development-tools/static-security-checker.html
+ link: docs/dg/dev/sdks/sdk/development-tools/static-security-checker.html
---
In order to make the tool configuring more convenient, we introduced the `.tooling.yml` file. It contains a variety of settings for different tools in one place, helping you to keep the number of files on the root level as small as possible. The `.tooling.yml` file should also be in `.gitattributes` to be ignored for tagging:
diff --git a/docs/dg/dev/sdks/sdk/development-tools/ts-linter.md b/docs/dg/dev/sdks/sdk/development-tools/ts-linter.md
index 45333ac1a56..9d2ce5070b9 100644
--- a/docs/dg/dev/sdks/sdk/development-tools/ts-linter.md
+++ b/docs/dg/dev/sdks/sdk/development-tools/ts-linter.md
@@ -23,24 +23,24 @@ redirect_from:
- /docs/sdk/dev/development-tools/ts-linter.html
related:
- - title: Architecture sniffer
- link: docs/scos/dev/sdk/development-tools/architecture-sniffer.html
- title: Code sniffer
- link: docs/scos/dev/sdk/development-tools/code-sniffer.html
+ link: docs/dg/dev/sdks/sdk/development-tools/code-sniffer.html
- title: Formatter
- link: docs/scos/dev/sdk/development-tools/formatter.html
+ link: docs/dg/dev/sdks/sdk/development-tools/formatter.html
+ - title: Architecture sniffer
+ link: docs/dg/dev/sdks/sdk/development-tools/architecture-sniffer.html
- title: Performance audit tool- Benchmark
- link: docs/scos/dev/sdk/development-tools/performance-audit-tool-benchmark.html
+ link: docs/dg/dev/sdks/sdk/development-tools/benchmark-performance-audit-tool.html
- title: PHPStan
link: docs/dg/dev/sdks/sdk/development-tools/phpstan.html
- title: SCSS linter
- link: docs/scos/dev/sdk/development-tools/scss-linter.html
+ link: docs/dg/dev/sdks/sdk/development-tools/scss-linter.html
- title: Spryk code generator
- link: docs/scos/dev/sdk/development-tools/spryk-code-generator.html
+ link: docs/dg/dev/sdks/sdk/spryks/spryks.html
- title: Static Security Checker
- link: docs/scos/dev/sdk/development-tools/static-security-checker.html
+ link: docs/dg/dev/sdks/sdk/development-tools/static-security-checker.html
- title: Tooling config file
- link: docs/scos/dev/sdk/development-tools/tooling-config-file.html
+ link: docs/dg/dev/sdks/sdk/development-tools/tooling-configuration-file.html
---
{% info_block warningBox "No longer supported" %}
diff --git a/docs/dg/dev/sdks/sdk/extending-spryker-sdk.md b/docs/dg/dev/sdks/sdk/extending-spryker-sdk.md
index 5cc32e67f23..e91b6d917e2 100644
--- a/docs/dg/dev/sdks/sdk/extending-spryker-sdk.md
+++ b/docs/dg/dev/sdks/sdk/extending-spryker-sdk.md
@@ -1,6 +1,6 @@
---
title: Extending Spryker SDK
-description: Find out how you can extend the Spryker SDK
+description: Find out how you can extend the Spryker SDK allowing third parties to contribute to the SDK without modifying it.
template: howto-guide-template
last_updated: Jan 13, 2023
redirect_from:
diff --git a/docs/dg/dev/sdks/sdk/initialize-and-run-workflows.md b/docs/dg/dev/sdks/sdk/initialize-and-run-workflows.md
index 0541a2f7110..a2b18548a6e 100644
--- a/docs/dg/dev/sdks/sdk/initialize-and-run-workflows.md
+++ b/docs/dg/dev/sdks/sdk/initialize-and-run-workflows.md
@@ -1,6 +1,6 @@
---
title: Initialize and run workflows
-description: Learn about the Spryker SDK telemetry configuration.
+description: Learn about the Spryker SDK telemetry configuration and how you can Initialise and run workflows within your Spryker SDK project.
template: howto-guide-template
last_updated: Dec 16, 2022
redirect_from:
diff --git a/docs/dg/dev/sdks/sdk/integrate-spryker-sdk-commands-into-php-storm-command-line-tools.md b/docs/dg/dev/sdks/sdk/integrate-spryker-sdk-commands-into-php-storm-command-line-tools.md
index 151a4c17703..518131781c9 100644
--- a/docs/dg/dev/sdks/sdk/integrate-spryker-sdk-commands-into-php-storm-command-line-tools.md
+++ b/docs/dg/dev/sdks/sdk/integrate-spryker-sdk-commands-into-php-storm-command-line-tools.md
@@ -1,6 +1,6 @@
---
title: Integrate Spryker SDK commands into PhpStorm command line tools
-description: You can make the Spryker SDK available for the PhpStorm.
+description: Learn how to integrate Spryker SDK commands in to PHPStorm command line tools for your Spryker based projects.
template: task-topic-template
last_updated: Nov 22, 2022
redirect_from:
diff --git a/docs/dg/dev/sdks/sdk/manifest-validation.md b/docs/dg/dev/sdks/sdk/manifest-validation.md
index 4dc603147e6..776d312f053 100644
--- a/docs/dg/dev/sdks/sdk/manifest-validation.md
+++ b/docs/dg/dev/sdks/sdk/manifest-validation.md
@@ -1,6 +1,6 @@
---
title: Manifest validation
-description: The manifest validation validates the YAML structure for a task.
+description: Learn about the Manifest validation feature where it validates the YAML structure for a task within your Spryker projects.
template: howto-guide-template
redirect_from:
- /docs/sdk/dev/manifest-validation.html
diff --git a/docs/dg/dev/sdks/sdk/spryker-sdk.md b/docs/dg/dev/sdks/sdk/spryker-sdk.md
index 494d0f53cf2..af9110e58c8 100644
--- a/docs/dg/dev/sdks/sdk/spryker-sdk.md
+++ b/docs/dg/dev/sdks/sdk/spryker-sdk.md
@@ -1,6 +1,6 @@
---
title: Spryker SDK
-description: Learn about the Spryker SDK and how you can use it in your project.
+description: Learn all about the Spryker SDK and how you can use it to enhance your Spryker projects.
template: concept-topic-template
last_updated: Aug 31, 2023
redirect_from:
diff --git a/docs/dg/dev/sdks/sdk/spryks/adding-spryks.md b/docs/dg/dev/sdks/sdk/spryks/adding-spryks.md
index 1f8b33ffb95..7eb5e60453b 100644
--- a/docs/dg/dev/sdks/sdk/spryks/adding-spryks.md
+++ b/docs/dg/dev/sdks/sdk/spryks/adding-spryks.md
@@ -1,6 +1,6 @@
---
title: Adding Spryks
-description: Find out how you can add a new Spryk
+description: Find out how you can add a new Spryk in to your Spryker project with Spryker SDKs
template: howto-guide-template
redirect_from:
- /docs/sdk/dev/spryks/adding-a-new-spryk.html
@@ -8,7 +8,7 @@ redirect_from:
last_updated: Nov 10, 2022
related:
- title: Spryk configuration reference
- link: docs/sdk/dev/spryks/spryk-configuration-reference.html
+ link: docs/dg/dev/sdks/sdk/spryks/spryk-configuration-reference.html
---
To add a new Spryk, you need to add a YAML configuration file to the `config/spryk/spryks/` directory.
diff --git a/docs/dg/dev/sdks/sdk/spryks/checking-and-debugging-spryks.md b/docs/dg/dev/sdks/sdk/spryks/checking-and-debugging-spryks.md
index 033231f1a59..eae66659748 100644
--- a/docs/dg/dev/sdks/sdk/spryks/checking-and-debugging-spryks.md
+++ b/docs/dg/dev/sdks/sdk/spryks/checking-and-debugging-spryks.md
@@ -1,6 +1,6 @@
---
title: Checking and debugging Spryks
-description: Find out how you can check and debug Spryks
+description: Learn how you can check, troubleshoot and debug Spryks nad how to avoid dependencies within your Spryker project.
template: howto-guide-template
redirect_from:
- /docs/sdk/dev/spryks/checking-and-debugging-spryks.html
diff --git a/docs/dg/dev/sdks/sdk/spryks/enabling-and-disabling-the-code-sniffer-for-spryks.md b/docs/dg/dev/sdks/sdk/spryks/enabling-and-disabling-the-code-sniffer-for-spryks.md
index 32294dc3057..469c2c3a8ce 100644
--- a/docs/dg/dev/sdks/sdk/spryks/enabling-and-disabling-the-code-sniffer-for-spryks.md
+++ b/docs/dg/dev/sdks/sdk/spryks/enabling-and-disabling-the-code-sniffer-for-spryks.md
@@ -1,6 +1,6 @@
---
title: Enabling and disabling the Code Sniffer for Spryks
-description: Find out how you can enable or disable the Code Sniffer when running Spryks
+description: Find out how you can enable or disable the Code Sniffer when running Spryks within your spryker projects.
template: howto-guide-template
redirect_from:
- /docs/sdk/dev/spryks/enabling-and-disabling-the-code-sniffer-for-spryks.html
diff --git a/docs/dg/dev/sdks/sdk/spryks/fileresolver.md b/docs/dg/dev/sdks/sdk/spryks/fileresolver.md
index 4c5d9320076..f1ac4c0ad49 100644
--- a/docs/dg/dev/sdks/sdk/spryks/fileresolver.md
+++ b/docs/dg/dev/sdks/sdk/spryks/fileresolver.md
@@ -1,6 +1,6 @@
---
title: FileResolver
-description: Find out how you can enable or disable the Code Sniffer when running Spryks
+description: Learn all about the FileResolver, a core part to file management within your Spryker Projects.
template: howto-guide-template
redirect_from:
- /docs/sdk/dev/spryks/fileresolver.html
diff --git a/docs/dg/dev/sdks/sdk/spryks/spryk-configuration-reference.md b/docs/dg/dev/sdks/sdk/spryks/spryk-configuration-reference.md
index a30a9a6b93f..a2f2e015be4 100644
--- a/docs/dg/dev/sdks/sdk/spryks/spryk-configuration-reference.md
+++ b/docs/dg/dev/sdks/sdk/spryks/spryk-configuration-reference.md
@@ -1,6 +1,6 @@
---
title: Spryk configuration reference
-description: Learn about the Spryk file structure and its elements
+description: Learn about the Spryk file structure and its elements with this Spryks configuration reference for your projects.
template: howto-guide-template
redirect_from:
- /docs/sdk/dev/spryks/spryk-configuration-reference.html
diff --git a/docs/dg/dev/sdks/sdk/spryks/spryks-qa-automation.md b/docs/dg/dev/sdks/sdk/spryks/spryks-qa-automation.md
index 722c25d0a4f..42220fd430b 100644
--- a/docs/dg/dev/sdks/sdk/spryks/spryks-qa-automation.md
+++ b/docs/dg/dev/sdks/sdk/spryks/spryks-qa-automation.md
@@ -1,6 +1,6 @@
---
title: Spryks QA automation
-description: QA automation lets you run QA tools and unit tests required by Spryker
+description: Learn all about QA automation lets you run QA tools and unit tests required by Spryker
template: howto-guide-template
redirect_from:
- /docs/sdk/dev/spryks/qa-automation.html
diff --git a/docs/dg/dev/sdks/sdk/task-set.md b/docs/dg/dev/sdks/sdk/task-set.md
index 8874d41a65a..298734e1cb6 100644
--- a/docs/dg/dev/sdks/sdk/task-set.md
+++ b/docs/dg/dev/sdks/sdk/task-set.md
@@ -1,6 +1,6 @@
---
title: Task set
-description: Task set is a group of related tasks that you can run with one command.
+description: Learn about task sets and how a Task set is a group of related tasks that you can run with one command.
template: concept-topic-template
redirect_from:
- /docs/sdk/dev/task-set.html
diff --git a/docs/dg/dev/sdks/sdk/task.md b/docs/dg/dev/sdks/sdk/task.md
index 8815b425043..71c16876c1f 100644
--- a/docs/dg/dev/sdks/sdk/task.md
+++ b/docs/dg/dev/sdks/sdk/task.md
@@ -1,6 +1,6 @@
---
title: Task
-description: Task is the smallest unit for running commands in the Spryker SDK which serves as a command wrapper.
+description: Learn about Tasks and how a Task is the smallest unit for running commands in the Spryker SDK which serves as a command wrapper.
template: concept-topic-template
redirect_from:
- /docs/sdk/dev/task.html
diff --git a/docs/dg/dev/sdks/sdk/telemetry.md b/docs/dg/dev/sdks/sdk/telemetry.md
index e57a737943b..b5b6e7fc8a0 100644
--- a/docs/dg/dev/sdks/sdk/telemetry.md
+++ b/docs/dg/dev/sdks/sdk/telemetry.md
@@ -1,6 +1,6 @@
---
title: Telemetry
-description: Learn about the Spryker SDK telemetry configuration.
+description: Learn about the Spryker SDK telemetry configuration and how to implement custom events within your Spryker project.
template: howto-guide-template
redirect_from:
- /docs/sdk/dev/telemetry.html
diff --git a/docs/dg/dev/sdks/sdk/troubleshooting/spryker-sdk-command-not-found.md b/docs/dg/dev/sdks/sdk/troubleshooting/spryker-sdk-command-not-found.md
index ea8a43d23fd..86886931913 100644
--- a/docs/dg/dev/sdks/sdk/troubleshooting/spryker-sdk-command-not-found.md
+++ b/docs/dg/dev/sdks/sdk/troubleshooting/spryker-sdk-command-not-found.md
@@ -1,27 +1,27 @@
---
title: spryker-sdk command not found
-description: Troubleshooting for the Spryker SDK document.
+description: Learn how to Troubleshooting and resolve for the Spryker SDK command not found within your spryker projects.
template: concept-topic-template
redirect_from:
- /docs/sdk/dev/troubleshooting/spryker-sdk-command-not-found.html
last_updated: Jan 18, 2023
---
-# Troubleshooting
+## Troubleshooting
-## `spryker-sdk` command not found.
+### `spryker-sdk` command not found.
The `spryker-sdk` command cannot be found.
-## Description
+### Description
The `spryker-sdk` command cannot be found.
-## Cause
+### Cause
The command might not exist in your file.
-## Solution
+### Solution
1. Add the command manually to your `shell rc` file. Depending on your shell it can be `~/.bashrc`, `~/.zshrc`, `~/.profile`, and other names.
diff --git a/docs/dg/dev/sdks/sdk/value-resolvers.md b/docs/dg/dev/sdks/sdk/value-resolvers.md
index cbe759204ce..7c451b6ce2e 100644
--- a/docs/dg/dev/sdks/sdk/value-resolvers.md
+++ b/docs/dg/dev/sdks/sdk/value-resolvers.md
@@ -1,6 +1,6 @@
---
title: Value resolvers
-description: Spryker SDK has several value resolvers described in this document.
+description: Learn about Spryker SDK and its several value resolvers described in this document for your spryker projects.
template: concept-topic-template
redirect_from:
- /docs/sdk/dev/value-resolvers.html
diff --git a/docs/dg/dev/sdks/sdk/vcs-connector.md b/docs/dg/dev/sdks/sdk/vcs-connector.md
index 938de0884b6..2cf388c879a 100644
--- a/docs/dg/dev/sdks/sdk/vcs-connector.md
+++ b/docs/dg/dev/sdks/sdk/vcs-connector.md
@@ -1,6 +1,6 @@
---
title: VCS connector
-description: The VCS connector feature for the Spryker SDK.
+description: A quick guide to learn everyting you need to know about the VCS connector feature for the Spryker SDK.
template: concept-topic-template
redirect_from:
- /docs/sdk/dev/vcs.html
diff --git a/docs/dg/dev/sdks/the-docker-sdk/choosing-a-mount-mode.md b/docs/dg/dev/sdks/the-docker-sdk/choosing-a-mount-mode.md
index ac692374c4a..4c9881c0ba6 100644
--- a/docs/dg/dev/sdks/the-docker-sdk/choosing-a-mount-mode.md
+++ b/docs/dg/dev/sdks/the-docker-sdk/choosing-a-mount-mode.md
@@ -1,6 +1,6 @@
---
title: Choosing a mount mode
-description: Learn about supported mount modes and how to choose one.
+description: Learn about supported mount modes and how to choose one depending of your operating system for your Spryker Project.
last_updated: Jun 16, 2021
template: howto-guide-template
originalLink: https://documentation.spryker.com/2021080/docs/choosing-a-mount-mode
diff --git a/docs/dg/dev/sdks/the-docker-sdk/configure-a-mount-mode.md b/docs/dg/dev/sdks/the-docker-sdk/configure-a-mount-mode.md
index ab7ffa6e48c..cf52d4f1550 100644
--- a/docs/dg/dev/sdks/the-docker-sdk/configure-a-mount-mode.md
+++ b/docs/dg/dev/sdks/the-docker-sdk/configure-a-mount-mode.md
@@ -1,6 +1,6 @@
---
title: Configure a mount mode
-description: Learn how to configure a mount mode.
+description: Learn about supported mount modes and how to configure one depending of your operating system for your Spryker Project.
last_updated: Jun 16, 2021
template: howto-guide-template
originalLink: https://documentation.spryker.com/2021080/docs/configuring-a-mount-mode
diff --git a/docs/dg/dev/sdks/the-docker-sdk/configure-access-to-private-repositories.md b/docs/dg/dev/sdks/the-docker-sdk/configure-access-to-private-repositories.md
index 5247e2276b0..766d19cee9b 100644
--- a/docs/dg/dev/sdks/the-docker-sdk/configure-access-to-private-repositories.md
+++ b/docs/dg/dev/sdks/the-docker-sdk/configure-access-to-private-repositories.md
@@ -1,6 +1,6 @@
---
title: Configure access to private repositories
-description: Configure your local environment to access private repositories.
+description: Learn how to configure your local environment to access private repositories for your Spryker project.
last_updated: Jun 16, 2021
template: howto-guide-template
originalLink: https://documentation.spryker.com/2021080/docs/configuring-access-to-private-repositories
diff --git a/docs/dg/dev/sdks/the-docker-sdk/deploy-file/deploy-file-inheritance-common-use-cases.md b/docs/dg/dev/sdks/the-docker-sdk/deploy-file/deploy-file-inheritance-common-use-cases.md
index c46f86716a3..7a716ac8c87 100644
--- a/docs/dg/dev/sdks/the-docker-sdk/deploy-file/deploy-file-inheritance-common-use-cases.md
+++ b/docs/dg/dev/sdks/the-docker-sdk/deploy-file/deploy-file-inheritance-common-use-cases.md
@@ -1,6 +1,6 @@
---
title: "Deploy file inheritance: common use cases"
-description: Examples of using deploy file inheritance
+description: Learn about common use cases for deploy file inheritance and how you can enhance your Spryker projects.
template: concept-topic-template
last_updated: Nov 21, 2023
related:
diff --git a/docs/dg/dev/sdks/the-docker-sdk/deploy-file/deploy-file.md b/docs/dg/dev/sdks/the-docker-sdk/deploy-file/deploy-file.md
index a9c6316bdbf..e541b1247f2 100644
--- a/docs/dg/dev/sdks/the-docker-sdk/deploy-file/deploy-file.md
+++ b/docs/dg/dev/sdks/the-docker-sdk/deploy-file/deploy-file.md
@@ -1,6 +1,6 @@
---
title: Deploy file
-description: Overview of the deploy file
+description: Learn about the Spryker Deploy yaml file and how it works to deploy your Spryker environment.
template: concept-topic-template
last_updated: Nov 21, 2023
related:
diff --git a/docs/dg/dev/sdks/the-docker-sdk/docker-environment-infrastructure.md b/docs/dg/dev/sdks/the-docker-sdk/docker-environment-infrastructure.md
index 5bf14361a59..6e44a77f270 100644
--- a/docs/dg/dev/sdks/the-docker-sdk/docker-environment-infrastructure.md
+++ b/docs/dg/dev/sdks/the-docker-sdk/docker-environment-infrastructure.md
@@ -1,5 +1,6 @@
---
title: Docker environment infrastructure
+description: Learn all about the infrastructure of Spryker in a Docker environment for your Spryker baed projects.
last_updated: Jun 16, 2021
template: howto-guide-template
originalLink: https://documentation.spryker.com/2021080/docs/docker-environment-infrastructure
diff --git a/docs/dg/dev/sdks/the-docker-sdk/docker-sdk-configuration-reference.md b/docs/dg/dev/sdks/the-docker-sdk/docker-sdk-configuration-reference.md
index d9916e12a35..af94582a188 100644
--- a/docs/dg/dev/sdks/the-docker-sdk/docker-sdk-configuration-reference.md
+++ b/docs/dg/dev/sdks/the-docker-sdk/docker-sdk-configuration-reference.md
@@ -1,6 +1,6 @@
---
title: Docker SDK configuration reference
-description: Instructions for the most common configuration cases of the Docker SDK.
+description: A guide with instructions for the most common configuration cases of the Docker SDK for Spryker based projects.
last_updated: Jun 16, 2021
template: howto-guide-template
originalLink: https://documentation.spryker.com/2021080/docs/docker-sdk-configuration-reference
diff --git a/docs/dg/dev/sdks/the-docker-sdk/docker-sdk-quick-start-guide.md b/docs/dg/dev/sdks/the-docker-sdk/docker-sdk-quick-start-guide.md
index 67e1e9883c3..cbe5f8dbbfa 100644
--- a/docs/dg/dev/sdks/the-docker-sdk/docker-sdk-quick-start-guide.md
+++ b/docs/dg/dev/sdks/the-docker-sdk/docker-sdk-quick-start-guide.md
@@ -1,6 +1,6 @@
---
title: Docker SDK quick start guide
-description: Get started with Spryker Docker SDK.
+description: Get up and running quickly with this Quick start guide for Docker SDK for your Spryker projects.
last_updated: Jun 16, 2021
template: howto-guide-template
originalLink: https://documentation.spryker.com/2021080/docs/docker-sdk-quick-start-guide
diff --git a/docs/dg/dev/sdks/the-docker-sdk/installation-recipes-of-deployment-pipelines.md b/docs/dg/dev/sdks/the-docker-sdk/installation-recipes-of-deployment-pipelines.md
index 8234476ea51..840f314fc9d 100644
--- a/docs/dg/dev/sdks/the-docker-sdk/installation-recipes-of-deployment-pipelines.md
+++ b/docs/dg/dev/sdks/the-docker-sdk/installation-recipes-of-deployment-pipelines.md
@@ -1,6 +1,6 @@
---
title: Installation recipes of deployment pipelines
-description: Installation recipe commands and file structure.
+description: Learn all about the Spryker Installation recipes of your deployment pipelines for your spryker based projects.
last_updated: Nov 29, 2022
template: howto-guide-template
redirect_from:
diff --git a/docs/dg/dev/sdks/the-docker-sdk/reset-docker-environments.md b/docs/dg/dev/sdks/the-docker-sdk/reset-docker-environments.md
index cfa8cfa03e2..4645af7983d 100644
--- a/docs/dg/dev/sdks/the-docker-sdk/reset-docker-environments.md
+++ b/docs/dg/dev/sdks/the-docker-sdk/reset-docker-environments.md
@@ -1,6 +1,6 @@
---
title: Reset Docker environments
-description: Learn how to restart your Spryker in Docker from scratch.
+description: Learn how to restart and reset your docker environments to start from scratch for your Spryker projects.
last_updated: Jun 18, 2021
template: howto-guide-template
originalLink: https://documentation.spryker.com/2021080/docs/howto-reset-a-docker-environment
diff --git a/docs/dg/dev/sdks/the-docker-sdk/running-commands-with-the-docker-sdk.md b/docs/dg/dev/sdks/the-docker-sdk/running-commands-with-the-docker-sdk.md
index 94a5fab4bc8..7ca9efbd34f 100644
--- a/docs/dg/dev/sdks/the-docker-sdk/running-commands-with-the-docker-sdk.md
+++ b/docs/dg/dev/sdks/the-docker-sdk/running-commands-with-the-docker-sdk.md
@@ -1,6 +1,6 @@
---
title: Running commands with the Docker SDK
-description: How to use Docker SDK command line interface.
+description: Learn how to use Docker SDK command line interface to run commands for your Spryker based project.
last_updated: Jan 16, 2024
template: howto-guide-template
---
diff --git a/docs/dg/dev/sdks/the-docker-sdk/running-tests-with-the-docker-sdk.md b/docs/dg/dev/sdks/the-docker-sdk/running-tests-with-the-docker-sdk.md
index d4092b24502..9774e3942c5 100644
--- a/docs/dg/dev/sdks/the-docker-sdk/running-tests-with-the-docker-sdk.md
+++ b/docs/dg/dev/sdks/the-docker-sdk/running-tests-with-the-docker-sdk.md
@@ -1,6 +1,6 @@
---
title: Running tests with the Docker SDK
-description: Learn how to run tests with the Docker SDK.
+description: Learn how you can run tests in different ways with the Docker SDK for your Spryker based projects.
last_updated: Jun 16, 2021
template: howto-guide-template
originalLink: https://documentation.spryker.com/2021080/docs/running-tests-with-the-docker-sdk
diff --git a/docs/dg/dev/sdks/the-docker-sdk/update-the-docker-sdk.md b/docs/dg/dev/sdks/the-docker-sdk/update-the-docker-sdk.md
index 708dc07eff6..5c3f9f45b4a 100644
--- a/docs/dg/dev/sdks/the-docker-sdk/update-the-docker-sdk.md
+++ b/docs/dg/dev/sdks/the-docker-sdk/update-the-docker-sdk.md
@@ -1,5 +1,6 @@
---
title: Update the Docker SDK
+description: Learn how you can update the Docker SDK to a newer version depending on the installation of your Spryker instance.
last_updated: Jun 1, 2024
template: howto-guide-template
diff --git a/docs/pbc/all/cart-and-checkout/202311.0/base-shop/extend-and-customize/checkout-process-review-and-implementation.md b/docs/pbc/all/cart-and-checkout/202311.0/base-shop/extend-and-customize/checkout-process-review-and-implementation.md
index b389e6be211..8f349b7a7cd 100644
--- a/docs/pbc/all/cart-and-checkout/202311.0/base-shop/extend-and-customize/checkout-process-review-and-implementation.md
+++ b/docs/pbc/all/cart-and-checkout/202311.0/base-shop/extend-and-customize/checkout-process-review-and-implementation.md
@@ -27,7 +27,7 @@ related:
link: docs/scos/dev/back-end-development/data-manipulation/datapayload-conversion/checkout/checkout-steps.html
---
-### Checkout process
+## Checkout process
To use the checkout in Yves, you need to configure it correctly and provide dependencies. Each step can have a form, a controller action, the implementation of the step logic, and a Twig template to render the HTML.
@@ -54,13 +54,13 @@ Using a data provider is the only way to pass any external data to the form and
Each data provider is passed when `FormCollection` is created. When the handle method is called, the `FormCollection` handler creates all form types and passes the data from data providers to them.
-### Checkout quote transfer lifetime
+## Checkout quote transfer lifetime
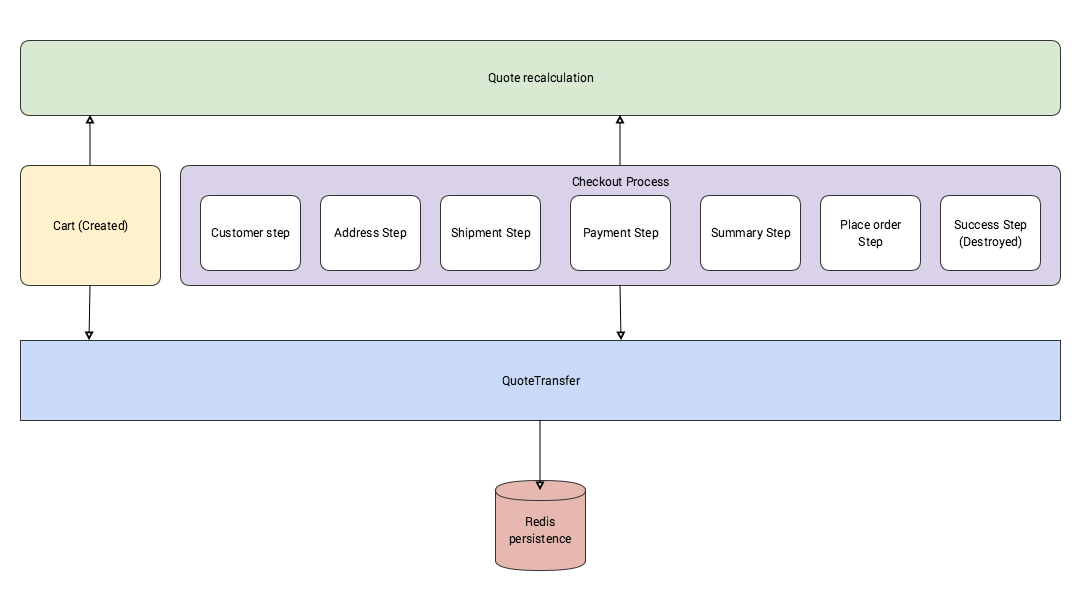
When a process or `postCondition()` is called on `StepProcess`, it tries to get the current valid step by walking through the stack of steps and calling `postCondition()` for each, starting from the first in the stack. If `postCondition()` fails, then this step is used for later processing. After that, the view variables with `QuoteTransfer` and form are passed to Twig, and the HTML is rendered.
-#### Postcondition
+### Postcondition
_Postcondition_ is an essential part of the Processing step. It indicates if a step has all the data that it needs and if its requirements are satisfied. You can't access the next step from the stack if the previous step's postconditions are not met, but you can navigate to any step where postconditions are satisfied (`return true`).
@@ -68,17 +68,17 @@ Postconditions are called twice per step processing:
* To find the current step or if we can access the current step.
* After `execute()`, to make sure the step was completed and that we can continue to the next step.
-#### Postcondition error route
+### Postcondition error route
Inside your step, you can set a postcondition error route if you need to redirect to another error route than the one specified during the step construction.
-### How the quote transfer is mapped inside forms
+## How the quote transfer is mapped inside forms
Symfony forms provide a mechanism to store data into objects without needing manual mapping. It's called [Data transformers](https://symfony.com/doc/current/form/data_transformers.html). There are a few important conditions required to make this work. Because you are passing the entire `QuoteTransfer`, the form handler does not know which fields you are trying to use. Symfony provides a few ways to handle this situation:
* Using [property_path](https://symfony.com/doc/current/reference/forms/types/form.html#property-path) configuration directive. It uses the full path to object property you are about to map form into—for example, `payment.paypal` maps your form to `QuoteTransfer:payment:paypal`; this works when the property is not on the same level and when you are using subforms.
* Using the main form that includes subforms. Each subform has to be configured with the `data_class` option, which is the FQCN of the transfer object you are about to use. This works when the property is on the top level.
-### Checkout form submission
+## Checkout form submission
On form submission, the same processing starts with the difference that if form submit's detected, then the validation is called:
* If the form is invalid, then the view is rendered with validation errors.
@@ -88,17 +88,17 @@ For example, add the address to `QuoteTransfer` or get payment details from Zed,
You decide what to do in each `execute()` method. It's essential that after `execute()` runs, the updated returned `QuoteTransfer` must satisfy `postCondition()` so that `StepProcess` can take another step from the stack.
-#### Required input
+### Required input
Normally each step requires input from the customer. However, there are cases when there is no need to render a form or a view, but some processing is still required, that is, `PlaceOrderStep` and `EntryStep`. Each step provides the implementation of the `requireInput()` method. `StepProcess` calls this method and reacts accordingly. If `requireInput()` is false, after running `execute()`, `postConditions` must be satisfied.
-#### Precondition and escape route
+### Precondition and escape route
Preconditions are called before each step; this is a check to indicate that the step can't be processed in a usual way.
For example, the cart is empty. If `preCondition()` returns false, the customer is redirected to `escapeRoute` provided when configuring the step.
-#### External redirect URL
+### External redirect URL
Sometimes it's required to redirect the customer to an external URL (outside application). The step must implement `StepWithExternalRedirectInterface::getExternalRedirectUrl()`, which returns the URL to redirect customer after `execute()` is ran.
@@ -110,11 +110,11 @@ Each step must implement `StepInterface`.
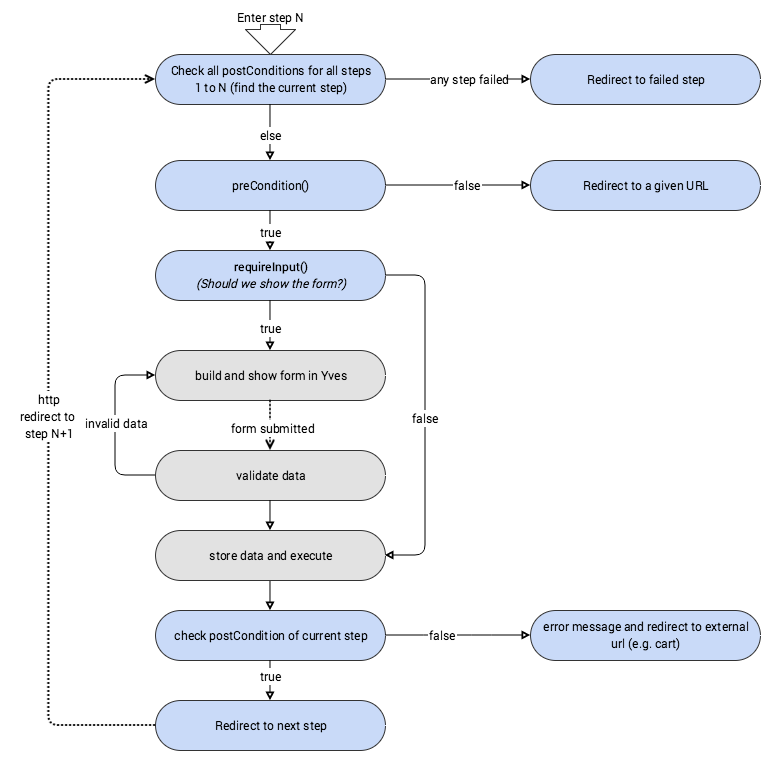
-### Placing the order
+## Placing the order
After the customer clicks the submit button during `SummaryStep`, `PlaceOrderStep` is started. This step takes `QuoteTransfer` and starts the checkout workflow to store the order in the system. Zed's Checkout module contains some plugins where you can add additional behavior, check preconditions, and save or execute postcondition checks.
-#### Plugins
+### Plugins
Zed's Checkout module contains four types of plugins to extend the behavior on placing an order. Any plugin has access to `QuoteTransfer` that is supposed to be read-only and `CheckoutResponseTransfer` data object that can be modified.
@@ -123,7 +123,7 @@ Zed's Checkout module contains four types of plugins to extend the behavior on p
* `CheckPostConditions`—is for checking conditions after saving, the last time to react if something did not happen according to plan. It's called after the state machine execution.
* `PostSaveHook`—is called after order placement, and sets the success flag to false, if redirect must be headed to an error page afterward.
-#### Checkout response transfer
+### Checkout response transfer
* `isSuccess` (boolean)—indicates if the checkout process was successful.
* errors (`CheckoutErrorTransfer`)—list of errors that occurred during the execution of the plugins.
@@ -131,19 +131,19 @@ Zed's Checkout module contains four types of plugins to extend the behavior on p
* `redirectUrl` (string)—URL to redirect customer after the order was placed successfully.
* `saveOrder` (`SaveOrderTransfer`)—stores ids of the items that OrderSaver plugins have saved.
-#### Checkout error transfer
+### Checkout error transfer
* `errorCode` (int)—numeric error code. The checkout error codes are listed in the following section [Checkout error codes](#checkout-error-codes).
* `message` (string)—error message.
-#### Checkout error codes
+### Checkout error codes
* `4001`—customer email already used.
* `4002`—product unavailable.
* `4003`—cart amount does not match.
* `5000`—unknown error.
-#### Save order transfer
+### Save order transfer
* `idSalesOrder` (int)—The unique ID of the current saved order.
* `orderReference` (string)—An auto-generated unique ID of the order.
@@ -151,12 +151,12 @@ Zed's Checkout module contains four types of plugins to extend the behavior on p
There are already some plugins implemented with each of those types:
-#### Precondition plugins
+### Precondition plugins
* `CustomerPreConditionCheckerPlugin`—checks if the email of the customer is already used.
* `ProductsAvailableCheckoutPreConditionPlugin`—check if the items contained in the cart are in stock.
-#### Postcondition plugins
+### Postcondition plugins
* `OrderCustomerSavePlugin`—saves or creates a customer in the database if the customer is new or the ID is not set (guest customers are ignored).
* `SalesOrderSaverPlugin`—saves order information and creates `sales_order` and `sales_order_item` tables.
@@ -165,11 +165,11 @@ There are already some plugins implemented with each of those types:
* `OrderShipmentSavePlugin`—saves order shipment information to the `sales_expense` table.
* `SalesPaymentCheckoutDoSaveOrderPlugin`—saves order payments to the `spy_sales_payment` table.
-#### Pre-save condition plugin
+### Pre-save condition plugin
`SalesOrderExpanderPlugin`—expands items by quantity and recalculate quote.
-#### State machine
+### State machine
A state machine is triggered in the `CheckoutWorkflow` class from the Checkout module; the execution starts after precondition and order saver plugins store no errors into `CheckoutResponseTransfer`.
diff --git a/docs/pbc/all/cart-and-checkout/202404.0/base-shop/extend-and-customize/checkout-process-review-and-implementation.md b/docs/pbc/all/cart-and-checkout/202404.0/base-shop/extend-and-customize/checkout-process-review-and-implementation.md
index b389e6be211..8f349b7a7cd 100644
--- a/docs/pbc/all/cart-and-checkout/202404.0/base-shop/extend-and-customize/checkout-process-review-and-implementation.md
+++ b/docs/pbc/all/cart-and-checkout/202404.0/base-shop/extend-and-customize/checkout-process-review-and-implementation.md
@@ -27,7 +27,7 @@ related:
link: docs/scos/dev/back-end-development/data-manipulation/datapayload-conversion/checkout/checkout-steps.html
---
-### Checkout process
+## Checkout process
To use the checkout in Yves, you need to configure it correctly and provide dependencies. Each step can have a form, a controller action, the implementation of the step logic, and a Twig template to render the HTML.
@@ -54,13 +54,13 @@ Using a data provider is the only way to pass any external data to the form and
Each data provider is passed when `FormCollection` is created. When the handle method is called, the `FormCollection` handler creates all form types and passes the data from data providers to them.
-### Checkout quote transfer lifetime
+## Checkout quote transfer lifetime
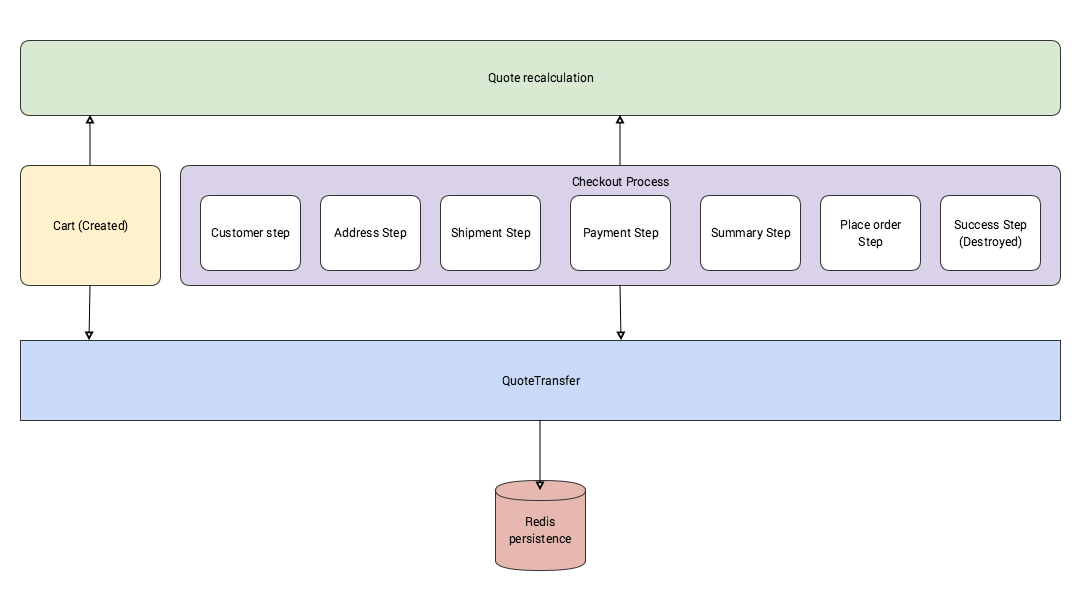
When a process or `postCondition()` is called on `StepProcess`, it tries to get the current valid step by walking through the stack of steps and calling `postCondition()` for each, starting from the first in the stack. If `postCondition()` fails, then this step is used for later processing. After that, the view variables with `QuoteTransfer` and form are passed to Twig, and the HTML is rendered.
-#### Postcondition
+### Postcondition
_Postcondition_ is an essential part of the Processing step. It indicates if a step has all the data that it needs and if its requirements are satisfied. You can't access the next step from the stack if the previous step's postconditions are not met, but you can navigate to any step where postconditions are satisfied (`return true`).
@@ -68,17 +68,17 @@ Postconditions are called twice per step processing:
* To find the current step or if we can access the current step.
* After `execute()`, to make sure the step was completed and that we can continue to the next step.
-#### Postcondition error route
+### Postcondition error route
Inside your step, you can set a postcondition error route if you need to redirect to another error route than the one specified during the step construction.
-### How the quote transfer is mapped inside forms
+## How the quote transfer is mapped inside forms
Symfony forms provide a mechanism to store data into objects without needing manual mapping. It's called [Data transformers](https://symfony.com/doc/current/form/data_transformers.html). There are a few important conditions required to make this work. Because you are passing the entire `QuoteTransfer`, the form handler does not know which fields you are trying to use. Symfony provides a few ways to handle this situation:
* Using [property_path](https://symfony.com/doc/current/reference/forms/types/form.html#property-path) configuration directive. It uses the full path to object property you are about to map form into—for example, `payment.paypal` maps your form to `QuoteTransfer:payment:paypal`; this works when the property is not on the same level and when you are using subforms.
* Using the main form that includes subforms. Each subform has to be configured with the `data_class` option, which is the FQCN of the transfer object you are about to use. This works when the property is on the top level.
-### Checkout form submission
+## Checkout form submission
On form submission, the same processing starts with the difference that if form submit's detected, then the validation is called:
* If the form is invalid, then the view is rendered with validation errors.
@@ -88,17 +88,17 @@ For example, add the address to `QuoteTransfer` or get payment details from Zed,
You decide what to do in each `execute()` method. It's essential that after `execute()` runs, the updated returned `QuoteTransfer` must satisfy `postCondition()` so that `StepProcess` can take another step from the stack.
-#### Required input
+### Required input
Normally each step requires input from the customer. However, there are cases when there is no need to render a form or a view, but some processing is still required, that is, `PlaceOrderStep` and `EntryStep`. Each step provides the implementation of the `requireInput()` method. `StepProcess` calls this method and reacts accordingly. If `requireInput()` is false, after running `execute()`, `postConditions` must be satisfied.
-#### Precondition and escape route
+### Precondition and escape route
Preconditions are called before each step; this is a check to indicate that the step can't be processed in a usual way.
For example, the cart is empty. If `preCondition()` returns false, the customer is redirected to `escapeRoute` provided when configuring the step.
-#### External redirect URL
+### External redirect URL
Sometimes it's required to redirect the customer to an external URL (outside application). The step must implement `StepWithExternalRedirectInterface::getExternalRedirectUrl()`, which returns the URL to redirect customer after `execute()` is ran.
@@ -110,11 +110,11 @@ Each step must implement `StepInterface`.
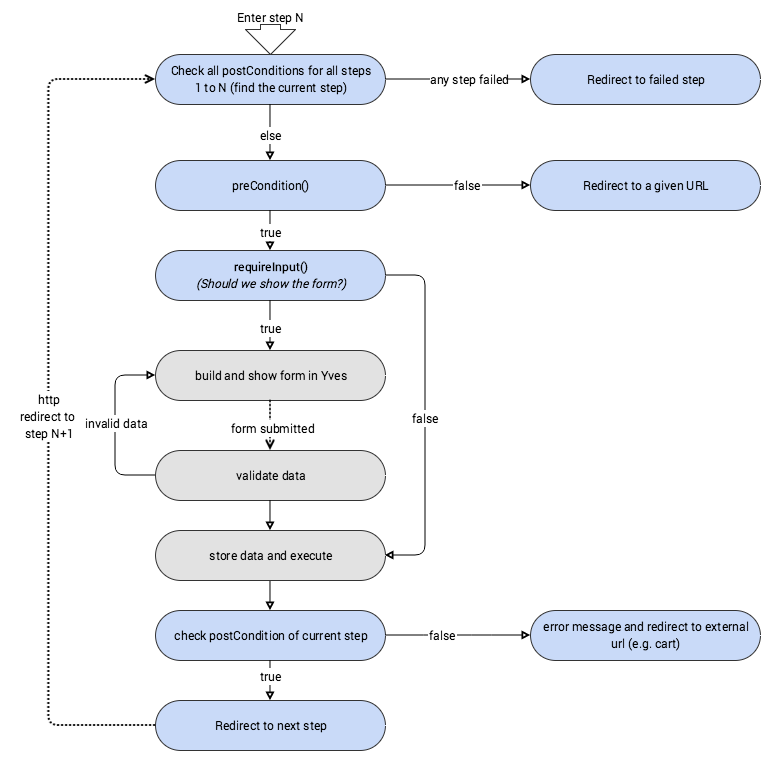
-### Placing the order
+## Placing the order
After the customer clicks the submit button during `SummaryStep`, `PlaceOrderStep` is started. This step takes `QuoteTransfer` and starts the checkout workflow to store the order in the system. Zed's Checkout module contains some plugins where you can add additional behavior, check preconditions, and save or execute postcondition checks.
-#### Plugins
+### Plugins
Zed's Checkout module contains four types of plugins to extend the behavior on placing an order. Any plugin has access to `QuoteTransfer` that is supposed to be read-only and `CheckoutResponseTransfer` data object that can be modified.
@@ -123,7 +123,7 @@ Zed's Checkout module contains four types of plugins to extend the behavior on p
* `CheckPostConditions`—is for checking conditions after saving, the last time to react if something did not happen according to plan. It's called after the state machine execution.
* `PostSaveHook`—is called after order placement, and sets the success flag to false, if redirect must be headed to an error page afterward.
-#### Checkout response transfer
+### Checkout response transfer
* `isSuccess` (boolean)—indicates if the checkout process was successful.
* errors (`CheckoutErrorTransfer`)—list of errors that occurred during the execution of the plugins.
@@ -131,19 +131,19 @@ Zed's Checkout module contains four types of plugins to extend the behavior on p
* `redirectUrl` (string)—URL to redirect customer after the order was placed successfully.
* `saveOrder` (`SaveOrderTransfer`)—stores ids of the items that OrderSaver plugins have saved.
-#### Checkout error transfer
+### Checkout error transfer
* `errorCode` (int)—numeric error code. The checkout error codes are listed in the following section [Checkout error codes](#checkout-error-codes).
* `message` (string)—error message.
-#### Checkout error codes
+### Checkout error codes
* `4001`—customer email already used.
* `4002`—product unavailable.
* `4003`—cart amount does not match.
* `5000`—unknown error.
-#### Save order transfer
+### Save order transfer
* `idSalesOrder` (int)—The unique ID of the current saved order.
* `orderReference` (string)—An auto-generated unique ID of the order.
@@ -151,12 +151,12 @@ Zed's Checkout module contains four types of plugins to extend the behavior on p
There are already some plugins implemented with each of those types:
-#### Precondition plugins
+### Precondition plugins
* `CustomerPreConditionCheckerPlugin`—checks if the email of the customer is already used.
* `ProductsAvailableCheckoutPreConditionPlugin`—check if the items contained in the cart are in stock.
-#### Postcondition plugins
+### Postcondition plugins
* `OrderCustomerSavePlugin`—saves or creates a customer in the database if the customer is new or the ID is not set (guest customers are ignored).
* `SalesOrderSaverPlugin`—saves order information and creates `sales_order` and `sales_order_item` tables.
@@ -165,11 +165,11 @@ There are already some plugins implemented with each of those types:
* `OrderShipmentSavePlugin`—saves order shipment information to the `sales_expense` table.
* `SalesPaymentCheckoutDoSaveOrderPlugin`—saves order payments to the `spy_sales_payment` table.
-#### Pre-save condition plugin
+### Pre-save condition plugin
`SalesOrderExpanderPlugin`—expands items by quantity and recalculate quote.
-#### State machine
+### State machine
A state machine is triggered in the `CheckoutWorkflow` class from the Checkout module; the execution starts after precondition and order saver plugins store no errors into `CheckoutResponseTransfer`.
diff --git a/docs/pbc/all/cart-and-checkout/202410.0/base-shop/extend-and-customize/checkout-process-review-and-implementation.md b/docs/pbc/all/cart-and-checkout/202410.0/base-shop/extend-and-customize/checkout-process-review-and-implementation.md
index b389e6be211..8f349b7a7cd 100644
--- a/docs/pbc/all/cart-and-checkout/202410.0/base-shop/extend-and-customize/checkout-process-review-and-implementation.md
+++ b/docs/pbc/all/cart-and-checkout/202410.0/base-shop/extend-and-customize/checkout-process-review-and-implementation.md
@@ -27,7 +27,7 @@ related:
link: docs/scos/dev/back-end-development/data-manipulation/datapayload-conversion/checkout/checkout-steps.html
---
-### Checkout process
+## Checkout process
To use the checkout in Yves, you need to configure it correctly and provide dependencies. Each step can have a form, a controller action, the implementation of the step logic, and a Twig template to render the HTML.
@@ -54,13 +54,13 @@ Using a data provider is the only way to pass any external data to the form and
Each data provider is passed when `FormCollection` is created. When the handle method is called, the `FormCollection` handler creates all form types and passes the data from data providers to them.
-### Checkout quote transfer lifetime
+## Checkout quote transfer lifetime
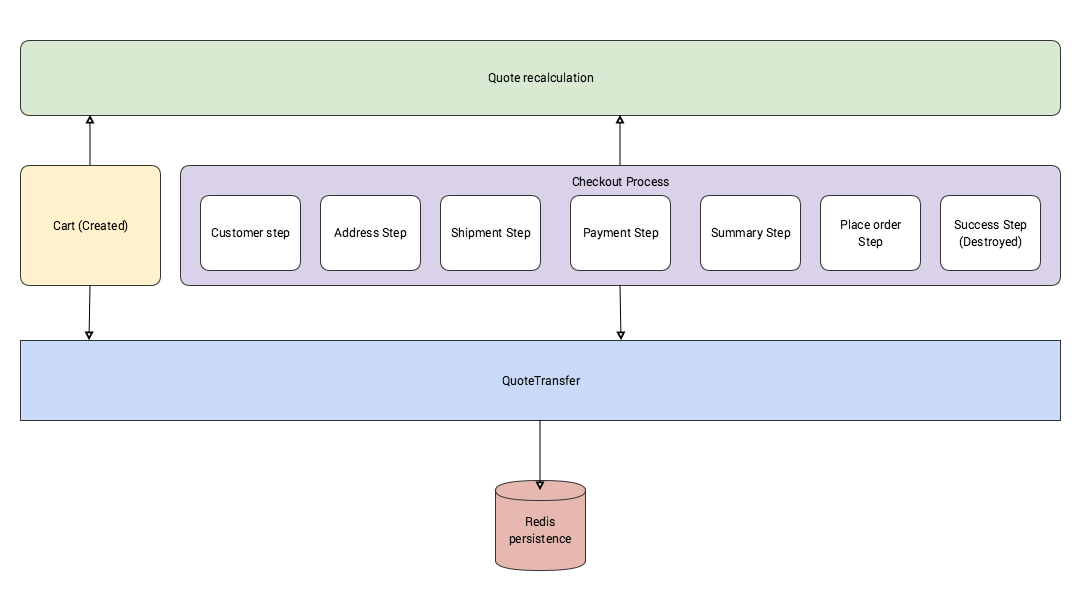
When a process or `postCondition()` is called on `StepProcess`, it tries to get the current valid step by walking through the stack of steps and calling `postCondition()` for each, starting from the first in the stack. If `postCondition()` fails, then this step is used for later processing. After that, the view variables with `QuoteTransfer` and form are passed to Twig, and the HTML is rendered.
-#### Postcondition
+### Postcondition
_Postcondition_ is an essential part of the Processing step. It indicates if a step has all the data that it needs and if its requirements are satisfied. You can't access the next step from the stack if the previous step's postconditions are not met, but you can navigate to any step where postconditions are satisfied (`return true`).
@@ -68,17 +68,17 @@ Postconditions are called twice per step processing:
* To find the current step or if we can access the current step.
* After `execute()`, to make sure the step was completed and that we can continue to the next step.
-#### Postcondition error route
+### Postcondition error route
Inside your step, you can set a postcondition error route if you need to redirect to another error route than the one specified during the step construction.
-### How the quote transfer is mapped inside forms
+## How the quote transfer is mapped inside forms
Symfony forms provide a mechanism to store data into objects without needing manual mapping. It's called [Data transformers](https://symfony.com/doc/current/form/data_transformers.html). There are a few important conditions required to make this work. Because you are passing the entire `QuoteTransfer`, the form handler does not know which fields you are trying to use. Symfony provides a few ways to handle this situation:
* Using [property_path](https://symfony.com/doc/current/reference/forms/types/form.html#property-path) configuration directive. It uses the full path to object property you are about to map form into—for example, `payment.paypal` maps your form to `QuoteTransfer:payment:paypal`; this works when the property is not on the same level and when you are using subforms.
* Using the main form that includes subforms. Each subform has to be configured with the `data_class` option, which is the FQCN of the transfer object you are about to use. This works when the property is on the top level.
-### Checkout form submission
+## Checkout form submission
On form submission, the same processing starts with the difference that if form submit's detected, then the validation is called:
* If the form is invalid, then the view is rendered with validation errors.
@@ -88,17 +88,17 @@ For example, add the address to `QuoteTransfer` or get payment details from Zed,
You decide what to do in each `execute()` method. It's essential that after `execute()` runs, the updated returned `QuoteTransfer` must satisfy `postCondition()` so that `StepProcess` can take another step from the stack.
-#### Required input
+### Required input
Normally each step requires input from the customer. However, there are cases when there is no need to render a form or a view, but some processing is still required, that is, `PlaceOrderStep` and `EntryStep`. Each step provides the implementation of the `requireInput()` method. `StepProcess` calls this method and reacts accordingly. If `requireInput()` is false, after running `execute()`, `postConditions` must be satisfied.
-#### Precondition and escape route
+### Precondition and escape route
Preconditions are called before each step; this is a check to indicate that the step can't be processed in a usual way.
For example, the cart is empty. If `preCondition()` returns false, the customer is redirected to `escapeRoute` provided when configuring the step.
-#### External redirect URL
+### External redirect URL
Sometimes it's required to redirect the customer to an external URL (outside application). The step must implement `StepWithExternalRedirectInterface::getExternalRedirectUrl()`, which returns the URL to redirect customer after `execute()` is ran.
@@ -110,11 +110,11 @@ Each step must implement `StepInterface`.
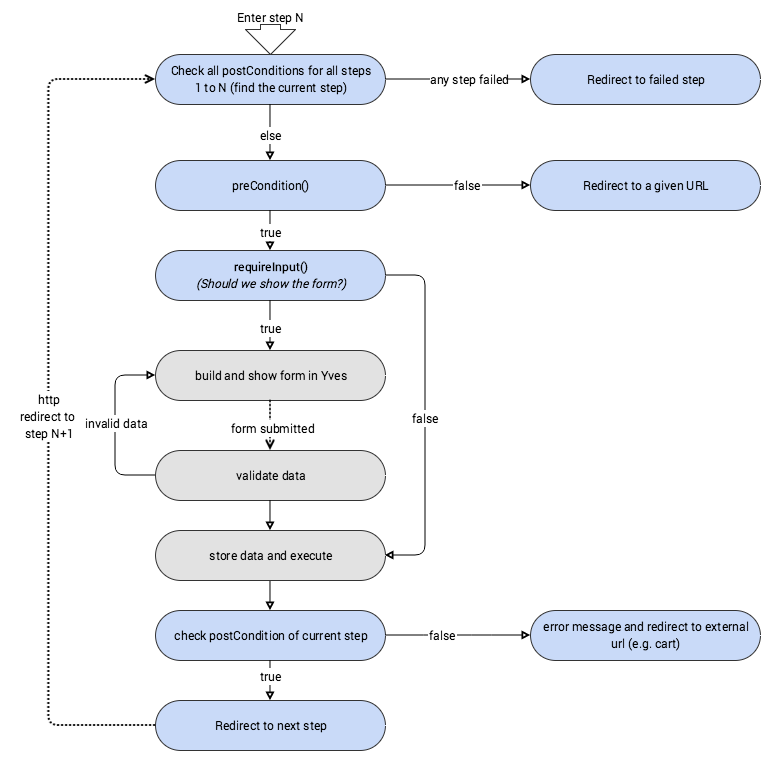
-### Placing the order
+## Placing the order
After the customer clicks the submit button during `SummaryStep`, `PlaceOrderStep` is started. This step takes `QuoteTransfer` and starts the checkout workflow to store the order in the system. Zed's Checkout module contains some plugins where you can add additional behavior, check preconditions, and save or execute postcondition checks.
-#### Plugins
+### Plugins
Zed's Checkout module contains four types of plugins to extend the behavior on placing an order. Any plugin has access to `QuoteTransfer` that is supposed to be read-only and `CheckoutResponseTransfer` data object that can be modified.
@@ -123,7 +123,7 @@ Zed's Checkout module contains four types of plugins to extend the behavior on p
* `CheckPostConditions`—is for checking conditions after saving, the last time to react if something did not happen according to plan. It's called after the state machine execution.
* `PostSaveHook`—is called after order placement, and sets the success flag to false, if redirect must be headed to an error page afterward.
-#### Checkout response transfer
+### Checkout response transfer
* `isSuccess` (boolean)—indicates if the checkout process was successful.
* errors (`CheckoutErrorTransfer`)—list of errors that occurred during the execution of the plugins.
@@ -131,19 +131,19 @@ Zed's Checkout module contains four types of plugins to extend the behavior on p
* `redirectUrl` (string)—URL to redirect customer after the order was placed successfully.
* `saveOrder` (`SaveOrderTransfer`)—stores ids of the items that OrderSaver plugins have saved.
-#### Checkout error transfer
+### Checkout error transfer
* `errorCode` (int)—numeric error code. The checkout error codes are listed in the following section [Checkout error codes](#checkout-error-codes).
* `message` (string)—error message.
-#### Checkout error codes
+### Checkout error codes
* `4001`—customer email already used.
* `4002`—product unavailable.
* `4003`—cart amount does not match.
* `5000`—unknown error.
-#### Save order transfer
+### Save order transfer
* `idSalesOrder` (int)—The unique ID of the current saved order.
* `orderReference` (string)—An auto-generated unique ID of the order.
@@ -151,12 +151,12 @@ Zed's Checkout module contains four types of plugins to extend the behavior on p
There are already some plugins implemented with each of those types:
-#### Precondition plugins
+### Precondition plugins
* `CustomerPreConditionCheckerPlugin`—checks if the email of the customer is already used.
* `ProductsAvailableCheckoutPreConditionPlugin`—check if the items contained in the cart are in stock.
-#### Postcondition plugins
+### Postcondition plugins
* `OrderCustomerSavePlugin`—saves or creates a customer in the database if the customer is new or the ID is not set (guest customers are ignored).
* `SalesOrderSaverPlugin`—saves order information and creates `sales_order` and `sales_order_item` tables.
@@ -165,11 +165,11 @@ There are already some plugins implemented with each of those types:
* `OrderShipmentSavePlugin`—saves order shipment information to the `sales_expense` table.
* `SalesPaymentCheckoutDoSaveOrderPlugin`—saves order payments to the `spy_sales_payment` table.
-#### Pre-save condition plugin
+### Pre-save condition plugin
`SalesOrderExpanderPlugin`—expands items by quantity and recalculate quote.
-#### State machine
+### State machine
A state machine is triggered in the `CheckoutWorkflow` class from the Checkout module; the execution starts after precondition and order saver plugins store no errors into `CheckoutResponseTransfer`.
diff --git a/docs/pbc/all/emails/202311.0/third-party-integrations/integrate-inxmail.md b/docs/pbc/all/emails/202311.0/third-party-integrations/integrate-inxmail.md
index 5dc114886ee..c1b9eb52d79 100644
--- a/docs/pbc/all/emails/202311.0/third-party-integrations/integrate-inxmail.md
+++ b/docs/pbc/all/emails/202311.0/third-party-integrations/integrate-inxmail.md
@@ -10,7 +10,7 @@ redirect_from:
This document desribes how to integrate Inxmail.
-### New customer registration event
+## New customer registration event
Inxmail module has `\SprykerEco\Zed\Inxmail\Communication\Plugin\Customer\InxmailPostCustomerRegistrationPlugin`. This plugin implements `PostCustomerRegistrationPluginInterface` and can be used in `\Pyz\Zed\Customer\CustomerDependencyProvider::getPostCustomerRegistrationPlugins.`
@@ -32,7 +32,7 @@ Inxmail module has `\SprykerEco\Zed\Inxmail\Communication\Plugin\Customer\Inxmai
}
```
-### The customer asked to reset password event
+## The customer asked to reset password event
Inxmail module has `\SprykerEco\Zed\Inxmail\Communication\Plugin\Customer\InxmailCustomerRestorePasswordMailTypePlugin`. This plugin implements `MailTypePluginInterface` and can be used in `\Pyz\Zed\Mail\MailDependencyProvider::provideBusinessLayerDependencies`
diff --git a/docs/pbc/all/emails/202404.0/third-party-integrations/integrate-inxmail.md b/docs/pbc/all/emails/202404.0/third-party-integrations/integrate-inxmail.md
index 5dc114886ee..c1b9eb52d79 100644
--- a/docs/pbc/all/emails/202404.0/third-party-integrations/integrate-inxmail.md
+++ b/docs/pbc/all/emails/202404.0/third-party-integrations/integrate-inxmail.md
@@ -10,7 +10,7 @@ redirect_from:
This document desribes how to integrate Inxmail.
-### New customer registration event
+## New customer registration event
Inxmail module has `\SprykerEco\Zed\Inxmail\Communication\Plugin\Customer\InxmailPostCustomerRegistrationPlugin`. This plugin implements `PostCustomerRegistrationPluginInterface` and can be used in `\Pyz\Zed\Customer\CustomerDependencyProvider::getPostCustomerRegistrationPlugins.`
@@ -32,7 +32,7 @@ Inxmail module has `\SprykerEco\Zed\Inxmail\Communication\Plugin\Customer\Inxmai
}
```
-### The customer asked to reset password event
+## The customer asked to reset password event
Inxmail module has `\SprykerEco\Zed\Inxmail\Communication\Plugin\Customer\InxmailCustomerRestorePasswordMailTypePlugin`. This plugin implements `MailTypePluginInterface` and can be used in `\Pyz\Zed\Mail\MailDependencyProvider::provideBusinessLayerDependencies`
diff --git a/docs/pbc/all/emails/202410.0/third-party-integrations/integrate-inxmail.md b/docs/pbc/all/emails/202410.0/third-party-integrations/integrate-inxmail.md
index 7b1a15e2267..a30ae01258e 100644
--- a/docs/pbc/all/emails/202410.0/third-party-integrations/integrate-inxmail.md
+++ b/docs/pbc/all/emails/202410.0/third-party-integrations/integrate-inxmail.md
@@ -10,7 +10,7 @@ redirect_from:
This document desribes how to integrate Inxmail.
-### New customer registration event
+## New customer registration event
Inxmail module has `\SprykerEco\Zed\Inxmail\Communication\Plugin\Customer\InxmailPostCustomerRegistrationPlugin`. This plugin implements `PostCustomerRegistrationPluginInterface` and can be used in `\Pyz\Zed\Customer\CustomerDependencyProvider::getPostCustomerRegistrationPlugins.`
@@ -32,7 +32,7 @@ Inxmail module has `\SprykerEco\Zed\Inxmail\Communication\Plugin\Customer\Inxmai
}
```
-### The customer asked to reset password event
+## The customer asked to reset password event
Inxmail module has `\SprykerEco\Zed\Inxmail\Communication\Plugin\Customer\InxmailCustomerRestorePasswordMailTypePlugin`. This plugin implements `MailTypePluginInterface` and can be used in `\Pyz\Zed\Mail\MailDependencyProvider::provideBusinessLayerDependencies`
diff --git a/docs/pbc/all/payment-service-provider/202311.0/base-shop/third-party-integrations/heidelpay/integrate-payment-methods-for-heidelpay/integrate-the-ideal-payment-method-for-heidelpay.md b/docs/pbc/all/payment-service-provider/202311.0/base-shop/third-party-integrations/heidelpay/integrate-payment-methods-for-heidelpay/integrate-the-ideal-payment-method-for-heidelpay.md
index 3983f6f3c1c..5c991ee2454 100644
--- a/docs/pbc/all/payment-service-provider/202311.0/base-shop/third-party-integrations/heidelpay/integrate-payment-methods-for-heidelpay/integrate-the-ideal-payment-method-for-heidelpay.md
+++ b/docs/pbc/all/payment-service-provider/202311.0/base-shop/third-party-integrations/heidelpay/integrate-payment-methods-for-heidelpay/integrate-the-ideal-payment-method-for-heidelpay.md
@@ -35,11 +35,11 @@ related:
link: docs/pbc/all/payment-service-provider/page.version/base-shop/third-party-integrations/heidelpay/install-heidelpay.html
---
-### Setup
+## Setup
The following configuration should be made after Heidelpay has been [installed](/docs/pbc/all/payment-service-provider/{{page.version}}/base-shop/third-party-integrations/heidelpay/install-heidelpay.html) and [integrated](/docs/pbc/all/payment-service-provider/{{page.version}}/base-shop/third-party-integrations/heidelpay/integrate-heidelpay.html).
-#### Configuration
+### Configuration
Example (for testing only):
@@ -48,15 +48,15 @@ $config[HeidelpayConstants::CONFIG_HEIDELPAY_TRANSACTION_CHANNEL_IDEAL] = '31HA0
```
This value should be taken from HEIDELPAY.
-#### Checkout Payment Step Display
+### Checkout Payment Step Display
Displays payment method name with a radio button. No extra input fields are required.
-#### Payment Step Submitting
+### Payment Step Submitting
No extra actions needed, quote being filled with payment method selection as default.
-#### Summary Review and Order
+### Summary Review and Order
Submitting
diff --git a/docs/pbc/all/payment-service-provider/202311.0/base-shop/third-party-integrations/heidelpay/integrate-payment-methods-for-heidelpay/integrate-the-paypal-authorize-payment-method-for-heidelpay.md b/docs/pbc/all/payment-service-provider/202311.0/base-shop/third-party-integrations/heidelpay/integrate-payment-methods-for-heidelpay/integrate-the-paypal-authorize-payment-method-for-heidelpay.md
index 5eb276aed00..9bc2385216c 100644
--- a/docs/pbc/all/payment-service-provider/202311.0/base-shop/third-party-integrations/heidelpay/integrate-payment-methods-for-heidelpay/integrate-the-paypal-authorize-payment-method-for-heidelpay.md
+++ b/docs/pbc/all/payment-service-provider/202311.0/base-shop/third-party-integrations/heidelpay/integrate-payment-methods-for-heidelpay/integrate-the-paypal-authorize-payment-method-for-heidelpay.md
@@ -35,11 +35,11 @@ related:
link: docs/pbc/all/payment-service-provider/page.version/base-shop/third-party-integrations/heidelpay/integrate-payment-methods-for-heidelpay/integrate-the-split-payment-marketplace-payment-method-for-heidelpay.html
---
-### Setup
+## Setup
The following configuration should be made after Heidelpay has been [installed](/docs/pbc/all/payment-service-provider/{{page.version}}/base-shop/third-party-integrations/heidelpay/install-heidelpay.html) and [integrated](/docs/pbc/all/payment-service-provider/{{page.version}}/base-shop/third-party-integrations/heidelpay/configure-heidelpay.html).
-#### Configuration
+### Configuration
Example (for testing only):
@@ -49,15 +49,15 @@ $config[HeidelpayConstants::CONFIG_HEIDELPAY_TRANSACTION_CHANNEL_PAYPAL] = '31HA
This value should be taken from HEIDELPAY
-#### Checkout Payment Step Display
+### Checkout Payment Step Display
Displays payment method name with radio button. No extra input fields are required.
-#### Payment Step Submitting
+### Payment Step Submitting
No extra actions needed, quote is filled with payment method selection by default.
-### Workflow: Summary Review and Order Submitting
+## Workflow: Summary Review and Order Submitting
**On "save order" event** save Heidelpay payment per order and items, as usual.
diff --git a/docs/pbc/all/payment-service-provider/202311.0/base-shop/third-party-integrations/heidelpay/integrate-payment-methods-for-heidelpay/integrate-the-paypal-debit-payment-method-for-heidelpay.md b/docs/pbc/all/payment-service-provider/202311.0/base-shop/third-party-integrations/heidelpay/integrate-payment-methods-for-heidelpay/integrate-the-paypal-debit-payment-method-for-heidelpay.md
index 3f7a46307d0..2017f00830b 100644
--- a/docs/pbc/all/payment-service-provider/202311.0/base-shop/third-party-integrations/heidelpay/integrate-payment-methods-for-heidelpay/integrate-the-paypal-debit-payment-method-for-heidelpay.md
+++ b/docs/pbc/all/payment-service-provider/202311.0/base-shop/third-party-integrations/heidelpay/integrate-payment-methods-for-heidelpay/integrate-the-paypal-debit-payment-method-for-heidelpay.md
@@ -35,11 +35,11 @@ related:
link: docs/pbc/all/payment-service-provider/page.version/base-shop/third-party-integrations/heidelpay/heidelpay-workflow-for-errors.html
---
-### Setup
+## Setup
The following configuration should be made after Heidelpay has been [installed](/docs/pbc/all/payment-service-provider/{{page.version}}/base-shop/third-party-integrations/heidelpay/install-heidelpay.html) and [integrated](/docs/pbc/all/payment-service-provider/{{page.version}}/base-shop/third-party-integrations/heidelpay/integrate-heidelpay.html).
-#### Configuration
+### Configuration
Example (for testing only):
@@ -48,17 +48,17 @@ $config[HeidelpayConstants::CONFIG_HEIDELPAY_TRANSACTION_CHANNEL_PAYPAL] = '31HA
```
This value should be taken from HEIDELPAY
-#### Checkout Payment Step Display
+### Checkout Payment Step Display
Displays payment method name with radio button. No extra input fields are required.
-#### Payment step submit
+### Payment step submit
No extra actions needed, quote being filled with payment method selection as default.
-### Workflow
+## Workflow
-#### Summary Review and Order Submitting
+### Summary Review and Order Submitting
**On "save order" event** - save Heidelpay payment per order and items, as usual.
diff --git a/docs/pbc/all/payment-service-provider/202311.0/base-shop/third-party-integrations/unzer/install-unzer/integrate-unzer.md b/docs/pbc/all/payment-service-provider/202311.0/base-shop/third-party-integrations/unzer/install-unzer/integrate-unzer.md
index bb91fd36b9e..84190a6bd64 100644
--- a/docs/pbc/all/payment-service-provider/202311.0/base-shop/third-party-integrations/unzer/install-unzer/integrate-unzer.md
+++ b/docs/pbc/all/payment-service-provider/202311.0/base-shop/third-party-integrations/unzer/install-unzer/integrate-unzer.md
@@ -11,15 +11,15 @@ related:
link: docs/pbc/all/payment-service-provider/page.version/base-shop/third-party-integrations/unzer/install-unzer/install-and-configure-unzer.html
---
-# Unzer feature integration
+## Unzer feature integration
This document describes how to integrate [Unzer](/docs/pbc/all/payment-service-provider/{{page.version}}/base-shop/third-party-integrations/unzer/unzer.html) into your project.
-## Install feature core
+### Install feature core
To integrate the Unzer, follow these steps.
-### Prerequisites
+#### Prerequisites
[Install and configure Unzer](/docs/pbc/all/payment-service-provider/{{page.version}}/base-shop/third-party-integrations/unzer/install-unzer/install-and-configure-unzer.html).
@@ -29,7 +29,7 @@ The following state machines are examples of the payment service provider flow.
{% endinfo_block %}
-### 1) Set up the configuration
+#### 1) Set up the configuration
1. Add the Unzer OMS processes to the project on the project level or provide your own:
@@ -80,7 +80,7 @@ $config[SalesConstants::PAYMENT_METHOD_STATEMACHINE_MAPPING] = [
---
-### 2) Set up database schema and transfer objects
+#### 2) Set up database schema and transfer objects
Apply database changes and generate entity and transfer changes:
@@ -233,7 +233,7 @@ Make sure that the following changes have been triggered in transfer objects:
---
-### 3) Add translations
+#### 3) Add translations
Append glossary according to your configuration:
@@ -278,7 +278,7 @@ Make sure that, in the database, the configured data are added to the `spy_gloss
---
-### 4) Add Zed translations
+#### 4) Add Zed translations
Generate a new translation cache for Zed:
@@ -294,7 +294,7 @@ Make sure that all labels and help tooltips in the Unzer forms have English and
---
-### 5) Set up behavior
+#### 5) Set up behavior
Set up the following behaviors:
@@ -685,11 +685,11 @@ class CheckoutPageDependencyProvider extends SprykerShopCheckoutPageDependencyPr
---
-## Install feature frontend
+### Install feature frontend
Follow these steps to install the Unzer feature front end.
-### 1) Set up behavior
+#### 1) Set up behavior
Set up the following behaviors:
@@ -732,7 +732,7 @@ class RouterDependencyProvider extends SprykerRouterDependencyProvider
-### 2) Set up template
+#### 2) Set up template
src/Pyz/Yves/CheckoutPage/Theme/default/views/payment/payment.twig
@@ -753,7 +753,7 @@ class RouterDependencyProvider extends SprykerRouterDependencyProvider
-### 3) Enable Javascript and CSS changes
+#### 3) Enable Javascript and CSS changes
```bash
console frontend:yves:build
diff --git a/docs/pbc/all/payment-service-provider/202404.0/base-shop/third-party-integrations/heidelpay/integrate-payment-methods-for-heidelpay/integrate-the-ideal-payment-method-for-heidelpay.md b/docs/pbc/all/payment-service-provider/202404.0/base-shop/third-party-integrations/heidelpay/integrate-payment-methods-for-heidelpay/integrate-the-ideal-payment-method-for-heidelpay.md
index 3983f6f3c1c..5c991ee2454 100644
--- a/docs/pbc/all/payment-service-provider/202404.0/base-shop/third-party-integrations/heidelpay/integrate-payment-methods-for-heidelpay/integrate-the-ideal-payment-method-for-heidelpay.md
+++ b/docs/pbc/all/payment-service-provider/202404.0/base-shop/third-party-integrations/heidelpay/integrate-payment-methods-for-heidelpay/integrate-the-ideal-payment-method-for-heidelpay.md
@@ -35,11 +35,11 @@ related:
link: docs/pbc/all/payment-service-provider/page.version/base-shop/third-party-integrations/heidelpay/install-heidelpay.html
---
-### Setup
+## Setup
The following configuration should be made after Heidelpay has been [installed](/docs/pbc/all/payment-service-provider/{{page.version}}/base-shop/third-party-integrations/heidelpay/install-heidelpay.html) and [integrated](/docs/pbc/all/payment-service-provider/{{page.version}}/base-shop/third-party-integrations/heidelpay/integrate-heidelpay.html).
-#### Configuration
+### Configuration
Example (for testing only):
@@ -48,15 +48,15 @@ $config[HeidelpayConstants::CONFIG_HEIDELPAY_TRANSACTION_CHANNEL_IDEAL] = '31HA0
```
This value should be taken from HEIDELPAY.
-#### Checkout Payment Step Display
+### Checkout Payment Step Display
Displays payment method name with a radio button. No extra input fields are required.
-#### Payment Step Submitting
+### Payment Step Submitting
No extra actions needed, quote being filled with payment method selection as default.
-#### Summary Review and Order
+### Summary Review and Order
Submitting
diff --git a/docs/pbc/all/payment-service-provider/202404.0/base-shop/third-party-integrations/heidelpay/integrate-payment-methods-for-heidelpay/integrate-the-paypal-authorize-payment-method-for-heidelpay.md b/docs/pbc/all/payment-service-provider/202404.0/base-shop/third-party-integrations/heidelpay/integrate-payment-methods-for-heidelpay/integrate-the-paypal-authorize-payment-method-for-heidelpay.md
index 5eb276aed00..9bc2385216c 100644
--- a/docs/pbc/all/payment-service-provider/202404.0/base-shop/third-party-integrations/heidelpay/integrate-payment-methods-for-heidelpay/integrate-the-paypal-authorize-payment-method-for-heidelpay.md
+++ b/docs/pbc/all/payment-service-provider/202404.0/base-shop/third-party-integrations/heidelpay/integrate-payment-methods-for-heidelpay/integrate-the-paypal-authorize-payment-method-for-heidelpay.md
@@ -35,11 +35,11 @@ related:
link: docs/pbc/all/payment-service-provider/page.version/base-shop/third-party-integrations/heidelpay/integrate-payment-methods-for-heidelpay/integrate-the-split-payment-marketplace-payment-method-for-heidelpay.html
---
-### Setup
+## Setup
The following configuration should be made after Heidelpay has been [installed](/docs/pbc/all/payment-service-provider/{{page.version}}/base-shop/third-party-integrations/heidelpay/install-heidelpay.html) and [integrated](/docs/pbc/all/payment-service-provider/{{page.version}}/base-shop/third-party-integrations/heidelpay/configure-heidelpay.html).
-#### Configuration
+### Configuration
Example (for testing only):
@@ -49,15 +49,15 @@ $config[HeidelpayConstants::CONFIG_HEIDELPAY_TRANSACTION_CHANNEL_PAYPAL] = '31HA
This value should be taken from HEIDELPAY
-#### Checkout Payment Step Display
+### Checkout Payment Step Display
Displays payment method name with radio button. No extra input fields are required.
-#### Payment Step Submitting
+### Payment Step Submitting
No extra actions needed, quote is filled with payment method selection by default.
-### Workflow: Summary Review and Order Submitting
+## Workflow: Summary Review and Order Submitting
**On "save order" event** save Heidelpay payment per order and items, as usual.
diff --git a/docs/pbc/all/payment-service-provider/202404.0/base-shop/third-party-integrations/heidelpay/integrate-payment-methods-for-heidelpay/integrate-the-paypal-debit-payment-method-for-heidelpay.md b/docs/pbc/all/payment-service-provider/202404.0/base-shop/third-party-integrations/heidelpay/integrate-payment-methods-for-heidelpay/integrate-the-paypal-debit-payment-method-for-heidelpay.md
index 3f7a46307d0..2017f00830b 100644
--- a/docs/pbc/all/payment-service-provider/202404.0/base-shop/third-party-integrations/heidelpay/integrate-payment-methods-for-heidelpay/integrate-the-paypal-debit-payment-method-for-heidelpay.md
+++ b/docs/pbc/all/payment-service-provider/202404.0/base-shop/third-party-integrations/heidelpay/integrate-payment-methods-for-heidelpay/integrate-the-paypal-debit-payment-method-for-heidelpay.md
@@ -35,11 +35,11 @@ related:
link: docs/pbc/all/payment-service-provider/page.version/base-shop/third-party-integrations/heidelpay/heidelpay-workflow-for-errors.html
---
-### Setup
+## Setup
The following configuration should be made after Heidelpay has been [installed](/docs/pbc/all/payment-service-provider/{{page.version}}/base-shop/third-party-integrations/heidelpay/install-heidelpay.html) and [integrated](/docs/pbc/all/payment-service-provider/{{page.version}}/base-shop/third-party-integrations/heidelpay/integrate-heidelpay.html).
-#### Configuration
+### Configuration
Example (for testing only):
@@ -48,17 +48,17 @@ $config[HeidelpayConstants::CONFIG_HEIDELPAY_TRANSACTION_CHANNEL_PAYPAL] = '31HA
```
This value should be taken from HEIDELPAY
-#### Checkout Payment Step Display
+### Checkout Payment Step Display
Displays payment method name with radio button. No extra input fields are required.
-#### Payment step submit
+### Payment step submit
No extra actions needed, quote being filled with payment method selection as default.
-### Workflow
+## Workflow
-#### Summary Review and Order Submitting
+### Summary Review and Order Submitting
**On "save order" event** - save Heidelpay payment per order and items, as usual.
diff --git a/docs/pbc/all/payment-service-provider/202404.0/base-shop/third-party-integrations/unzer/install-unzer/integrate-unzer.md b/docs/pbc/all/payment-service-provider/202404.0/base-shop/third-party-integrations/unzer/install-unzer/integrate-unzer.md
index bb91fd36b9e..84190a6bd64 100644
--- a/docs/pbc/all/payment-service-provider/202404.0/base-shop/third-party-integrations/unzer/install-unzer/integrate-unzer.md
+++ b/docs/pbc/all/payment-service-provider/202404.0/base-shop/third-party-integrations/unzer/install-unzer/integrate-unzer.md
@@ -11,15 +11,15 @@ related:
link: docs/pbc/all/payment-service-provider/page.version/base-shop/third-party-integrations/unzer/install-unzer/install-and-configure-unzer.html
---
-# Unzer feature integration
+## Unzer feature integration
This document describes how to integrate [Unzer](/docs/pbc/all/payment-service-provider/{{page.version}}/base-shop/third-party-integrations/unzer/unzer.html) into your project.
-## Install feature core
+### Install feature core
To integrate the Unzer, follow these steps.
-### Prerequisites
+#### Prerequisites
[Install and configure Unzer](/docs/pbc/all/payment-service-provider/{{page.version}}/base-shop/third-party-integrations/unzer/install-unzer/install-and-configure-unzer.html).
@@ -29,7 +29,7 @@ The following state machines are examples of the payment service provider flow.
{% endinfo_block %}
-### 1) Set up the configuration
+#### 1) Set up the configuration
1. Add the Unzer OMS processes to the project on the project level or provide your own:
@@ -80,7 +80,7 @@ $config[SalesConstants::PAYMENT_METHOD_STATEMACHINE_MAPPING] = [
---
-### 2) Set up database schema and transfer objects
+#### 2) Set up database schema and transfer objects
Apply database changes and generate entity and transfer changes:
@@ -233,7 +233,7 @@ Make sure that the following changes have been triggered in transfer objects:
---
-### 3) Add translations
+#### 3) Add translations
Append glossary according to your configuration:
@@ -278,7 +278,7 @@ Make sure that, in the database, the configured data are added to the `spy_gloss
---
-### 4) Add Zed translations
+#### 4) Add Zed translations
Generate a new translation cache for Zed:
@@ -294,7 +294,7 @@ Make sure that all labels and help tooltips in the Unzer forms have English and
---
-### 5) Set up behavior
+#### 5) Set up behavior
Set up the following behaviors:
@@ -685,11 +685,11 @@ class CheckoutPageDependencyProvider extends SprykerShopCheckoutPageDependencyPr
---
-## Install feature frontend
+### Install feature frontend
Follow these steps to install the Unzer feature front end.
-### 1) Set up behavior
+#### 1) Set up behavior
Set up the following behaviors:
@@ -732,7 +732,7 @@ class RouterDependencyProvider extends SprykerRouterDependencyProvider
-### 2) Set up template
+#### 2) Set up template
src/Pyz/Yves/CheckoutPage/Theme/default/views/payment/payment.twig
@@ -753,7 +753,7 @@ class RouterDependencyProvider extends SprykerRouterDependencyProvider
-### 3) Enable Javascript and CSS changes
+#### 3) Enable Javascript and CSS changes
```bash
console frontend:yves:build
diff --git a/docs/pbc/all/payment-service-provider/202410.0/base-shop/third-party-integrations/heidelpay/integrate-payment-methods-for-heidelpay/integrate-the-ideal-payment-method-for-heidelpay.md b/docs/pbc/all/payment-service-provider/202410.0/base-shop/third-party-integrations/heidelpay/integrate-payment-methods-for-heidelpay/integrate-the-ideal-payment-method-for-heidelpay.md
index f59415c1ddf..a1951db1d9c 100644
--- a/docs/pbc/all/payment-service-provider/202410.0/base-shop/third-party-integrations/heidelpay/integrate-payment-methods-for-heidelpay/integrate-the-ideal-payment-method-for-heidelpay.md
+++ b/docs/pbc/all/payment-service-provider/202410.0/base-shop/third-party-integrations/heidelpay/integrate-payment-methods-for-heidelpay/integrate-the-ideal-payment-method-for-heidelpay.md
@@ -35,11 +35,11 @@ related:
link: docs/pbc/all/payment-service-provider/page.version/base-shop/third-party-integrations/heidelpay/install-heidelpay.html
---
-### Setup
+## Setup
The following configuration should be made after Heidelpay has been [installed](/docs/pbc/all/payment-service-provider/{{page.version}}/base-shop/third-party-integrations/heidelpay/install-heidelpay.html) and [integrated](/docs/pbc/all/payment-service-provider/{{page.version}}/base-shop/third-party-integrations/heidelpay/integrate-heidelpay.html).
-#### Configuration
+### Configuration
Example (for testing only):
@@ -48,15 +48,15 @@ $config[HeidelpayConstants::CONFIG_HEIDELPAY_TRANSACTION_CHANNEL_IDEAL] = '31HA0
```
This value should be taken from HEIDELPAY.
-#### Checkout Payment Step Display
+### Checkout Payment Step Display
Displays payment method name with a radio button. No extra input fields are required.
-#### Payment Step Submitting
+### Payment Step Submitting
No extra actions needed, quote being filled with payment method selection as default.
-#### Summary Review and Order
+### Summary Review and Order
Submitting
diff --git a/docs/pbc/all/payment-service-provider/202410.0/base-shop/third-party-integrations/heidelpay/integrate-payment-methods-for-heidelpay/integrate-the-paypal-authorize-payment-method-for-heidelpay.md b/docs/pbc/all/payment-service-provider/202410.0/base-shop/third-party-integrations/heidelpay/integrate-payment-methods-for-heidelpay/integrate-the-paypal-authorize-payment-method-for-heidelpay.md
index 5eb276aed00..9bc2385216c 100644
--- a/docs/pbc/all/payment-service-provider/202410.0/base-shop/third-party-integrations/heidelpay/integrate-payment-methods-for-heidelpay/integrate-the-paypal-authorize-payment-method-for-heidelpay.md
+++ b/docs/pbc/all/payment-service-provider/202410.0/base-shop/third-party-integrations/heidelpay/integrate-payment-methods-for-heidelpay/integrate-the-paypal-authorize-payment-method-for-heidelpay.md
@@ -35,11 +35,11 @@ related:
link: docs/pbc/all/payment-service-provider/page.version/base-shop/third-party-integrations/heidelpay/integrate-payment-methods-for-heidelpay/integrate-the-split-payment-marketplace-payment-method-for-heidelpay.html
---
-### Setup
+## Setup
The following configuration should be made after Heidelpay has been [installed](/docs/pbc/all/payment-service-provider/{{page.version}}/base-shop/third-party-integrations/heidelpay/install-heidelpay.html) and [integrated](/docs/pbc/all/payment-service-provider/{{page.version}}/base-shop/third-party-integrations/heidelpay/configure-heidelpay.html).
-#### Configuration
+### Configuration
Example (for testing only):
@@ -49,15 +49,15 @@ $config[HeidelpayConstants::CONFIG_HEIDELPAY_TRANSACTION_CHANNEL_PAYPAL] = '31HA
This value should be taken from HEIDELPAY
-#### Checkout Payment Step Display
+### Checkout Payment Step Display
Displays payment method name with radio button. No extra input fields are required.
-#### Payment Step Submitting
+### Payment Step Submitting
No extra actions needed, quote is filled with payment method selection by default.
-### Workflow: Summary Review and Order Submitting
+## Workflow: Summary Review and Order Submitting
**On "save order" event** save Heidelpay payment per order and items, as usual.
diff --git a/docs/pbc/all/payment-service-provider/202410.0/base-shop/third-party-integrations/heidelpay/integrate-payment-methods-for-heidelpay/integrate-the-paypal-debit-payment-method-for-heidelpay.md b/docs/pbc/all/payment-service-provider/202410.0/base-shop/third-party-integrations/heidelpay/integrate-payment-methods-for-heidelpay/integrate-the-paypal-debit-payment-method-for-heidelpay.md
index 3f7a46307d0..2017f00830b 100644
--- a/docs/pbc/all/payment-service-provider/202410.0/base-shop/third-party-integrations/heidelpay/integrate-payment-methods-for-heidelpay/integrate-the-paypal-debit-payment-method-for-heidelpay.md
+++ b/docs/pbc/all/payment-service-provider/202410.0/base-shop/third-party-integrations/heidelpay/integrate-payment-methods-for-heidelpay/integrate-the-paypal-debit-payment-method-for-heidelpay.md
@@ -35,11 +35,11 @@ related:
link: docs/pbc/all/payment-service-provider/page.version/base-shop/third-party-integrations/heidelpay/heidelpay-workflow-for-errors.html
---
-### Setup
+## Setup
The following configuration should be made after Heidelpay has been [installed](/docs/pbc/all/payment-service-provider/{{page.version}}/base-shop/third-party-integrations/heidelpay/install-heidelpay.html) and [integrated](/docs/pbc/all/payment-service-provider/{{page.version}}/base-shop/third-party-integrations/heidelpay/integrate-heidelpay.html).
-#### Configuration
+### Configuration
Example (for testing only):
@@ -48,17 +48,17 @@ $config[HeidelpayConstants::CONFIG_HEIDELPAY_TRANSACTION_CHANNEL_PAYPAL] = '31HA
```
This value should be taken from HEIDELPAY
-#### Checkout Payment Step Display
+### Checkout Payment Step Display
Displays payment method name with radio button. No extra input fields are required.
-#### Payment step submit
+### Payment step submit
No extra actions needed, quote being filled with payment method selection as default.
-### Workflow
+## Workflow
-#### Summary Review and Order Submitting
+### Summary Review and Order Submitting
**On "save order" event** - save Heidelpay payment per order and items, as usual.
diff --git a/docs/pbc/all/payment-service-provider/202410.0/base-shop/third-party-integrations/unzer/install-unzer/integrate-unzer.md b/docs/pbc/all/payment-service-provider/202410.0/base-shop/third-party-integrations/unzer/install-unzer/integrate-unzer.md
index 7bf4099f4f0..754f766c89c 100644
--- a/docs/pbc/all/payment-service-provider/202410.0/base-shop/third-party-integrations/unzer/install-unzer/integrate-unzer.md
+++ b/docs/pbc/all/payment-service-provider/202410.0/base-shop/third-party-integrations/unzer/install-unzer/integrate-unzer.md
@@ -11,15 +11,15 @@ related:
link: docs/pbc/all/payment-service-provider/page.version/base-shop/third-party-integrations/unzer/install-unzer/install-and-configure-unzer.html
---
-# Unzer feature integration
+## Unzer feature integration
This document describes how to integrate [Unzer](/docs/pbc/all/payment-service-provider/{{page.version}}/base-shop/third-party-integrations/unzer/unzer.html) into your project.
-## Install feature core
+### Install feature core
To integrate the Unzer, follow these steps.
-### Prerequisites
+#### Prerequisites
[Install and configure Unzer](/docs/pbc/all/payment-service-provider/{{page.version}}/base-shop/third-party-integrations/unzer/install-unzer/install-and-configure-unzer.html).
@@ -29,7 +29,7 @@ The following state machines are examples of the payment service provider flow.
{% endinfo_block %}
-### 1) Set up the configuration
+#### 1) Set up the configuration
1. Add the Unzer OMS processes to the project on the project level or provide your own:
@@ -80,7 +80,7 @@ $config[SalesConstants::PAYMENT_METHOD_STATEMACHINE_MAPPING] = [
---
-### 2) Set up database schema and transfer objects
+#### 2) Set up database schema and transfer objects
Apply database changes and generate entity and transfer changes:
@@ -233,7 +233,7 @@ Make sure that the following changes have been triggered in transfer objects:
---
-### 3) Add translations
+#### 3) Add translations
Append glossary according to your configuration:
@@ -278,7 +278,7 @@ Make sure that, in the database, the configured data are added to the `spy_gloss
---
-### 4) Add Zed translations
+#### 4) Add Zed translations
Generate a new translation cache for Zed:
@@ -294,7 +294,7 @@ Make sure that all labels and help tooltips in the Unzer forms have English and
---
-### 5) Set up behavior
+#### 5) Set up behavior
Set up the following behaviors:
@@ -685,11 +685,11 @@ class CheckoutPageDependencyProvider extends SprykerShopCheckoutPageDependencyPr
---
-## Install feature frontend
+### Install feature frontend
Follow these steps to install the Unzer feature front end.
-### 1) Set up behavior
+#### 1) Set up behavior
Set up the following behaviors:
@@ -732,7 +732,7 @@ class RouterDependencyProvider extends SprykerRouterDependencyProvider
-### 2) Set up template
+#### 2) Set up template
src/Pyz/Yves/CheckoutPage/Theme/default/views/payment/payment.twig
@@ -753,7 +753,7 @@ class RouterDependencyProvider extends SprykerRouterDependencyProvider
-### 3) Enable Javascript and CSS changes
+#### 3) Enable Javascript and CSS changes
```bash
console frontend:yves:build
diff --git a/docs/pbc/all/search/202311.0/base-shop/best-practices/data-driven-ranking.md b/docs/pbc/all/search/202311.0/base-shop/best-practices/data-driven-ranking.md
index 3547e0d31a4..2ff67a56c19 100644
--- a/docs/pbc/all/search/202311.0/base-shop/best-practices/data-driven-ranking.md
+++ b/docs/pbc/all/search/202311.0/base-shop/best-practices/data-driven-ranking.md
@@ -51,7 +51,7 @@ When a query returns hundreds or thousands of results, the most relevant to the
A common solution is to manually assign ranks to products (sometimes even within categories). However, this approach is not practical for large catalogs and might result in a bad search experience—for example, when products that are out of stock are listed at the top because of their manually assigned rank.
-### Sorting by formulas based on scores
+## Sorting by formulas based on scores
We recommend an approach where a list of normalized scores per product at import time is precomputed and included in the documents that are sent to Elasticsearch. These are the scores from our previous hammer example (the interesting scores are left out because of the sensitivity of this information):
@@ -138,7 +138,7 @@ In queries, search results are sorted using an algebraic expression that combine
Very different kinds of scoring functions are conceivable, and the advantages of combining scores at query time are twofold: Your stakeholders (category and product managers) can help you in finding good formulas by testing the effect of different expressions on the sorting of actual queries at run-time. Second, you can use different ranking strategies on different parts of the website.
-### Computing normalized scores
+## Computing normalized scores
To combine scores in such expressions, we normalize them between 0 and 1 and try to make sure that they are more or less equally distributed across all documents. If, for example, the ranking formula is *0.3 * score_1 + 0.7 * score_2* and the scores are in the same range, then you could say that score_1 has a 30% influence on the outcome of the sorting and score_2 an influence of 70%. The equal distribution is important because if, for example, most documents have a very high score_2, then having a high score_2 becomes much more important for appearing at the top of the ranking than having a high score_1 (an effect that can be consciously used).
diff --git a/docs/pbc/all/search/202311.0/base-shop/best-practices/generic-faceted-search.md b/docs/pbc/all/search/202311.0/base-shop/best-practices/generic-faceted-search.md
index eefe86552cc..e969f8ec4b7 100644
--- a/docs/pbc/all/search/202311.0/base-shop/best-practices/generic-faceted-search.md
+++ b/docs/pbc/all/search/202311.0/base-shop/best-practices/generic-faceted-search.md
@@ -55,7 +55,7 @@ The main idea behind faceted search is to present the attributes of the document
To support faceted search, Elasticsearch offers a simple but powerful concept of aggregations. One of the nice features of aggregations is that they can be nested. In other words, you can define top-level aggregations that create "buckets" of documents and other aggregations that are executed inside those buckets on a subset of documents. The concept of aggregations is in general similar to the SQL `GROUP_BY` command (but much more powerful). Nested aggregations are analogous to SQL grouping but with multiple column names in the GROUP BY part of the query.
-### Indexing facet values
+## Indexing facet values
Before building aggregations, document attributes that can serve as facets need to be indexed in Elasticsearch. One way to index them is to list all attributes and their values under the same field like in the following example:
@@ -140,7 +140,7 @@ This requires special treatment in the mapping because otherwise, Elasticsearch
}
```
-### Facet queries
+## Facet queries
Filtering and aggregating a structure like this requires nested filters and nested aggregations in queries.
diff --git a/docs/pbc/all/search/202404.0/base-shop/best-practices/data-driven-ranking.md b/docs/pbc/all/search/202404.0/base-shop/best-practices/data-driven-ranking.md
index 3547e0d31a4..2ff67a56c19 100644
--- a/docs/pbc/all/search/202404.0/base-shop/best-practices/data-driven-ranking.md
+++ b/docs/pbc/all/search/202404.0/base-shop/best-practices/data-driven-ranking.md
@@ -51,7 +51,7 @@ When a query returns hundreds or thousands of results, the most relevant to the
A common solution is to manually assign ranks to products (sometimes even within categories). However, this approach is not practical for large catalogs and might result in a bad search experience—for example, when products that are out of stock are listed at the top because of their manually assigned rank.
-### Sorting by formulas based on scores
+## Sorting by formulas based on scores
We recommend an approach where a list of normalized scores per product at import time is precomputed and included in the documents that are sent to Elasticsearch. These are the scores from our previous hammer example (the interesting scores are left out because of the sensitivity of this information):
@@ -138,7 +138,7 @@ In queries, search results are sorted using an algebraic expression that combine
Very different kinds of scoring functions are conceivable, and the advantages of combining scores at query time are twofold: Your stakeholders (category and product managers) can help you in finding good formulas by testing the effect of different expressions on the sorting of actual queries at run-time. Second, you can use different ranking strategies on different parts of the website.
-### Computing normalized scores
+## Computing normalized scores
To combine scores in such expressions, we normalize them between 0 and 1 and try to make sure that they are more or less equally distributed across all documents. If, for example, the ranking formula is *0.3 * score_1 + 0.7 * score_2* and the scores are in the same range, then you could say that score_1 has a 30% influence on the outcome of the sorting and score_2 an influence of 70%. The equal distribution is important because if, for example, most documents have a very high score_2, then having a high score_2 becomes much more important for appearing at the top of the ranking than having a high score_1 (an effect that can be consciously used).
diff --git a/docs/pbc/all/search/202404.0/base-shop/best-practices/generic-faceted-search.md b/docs/pbc/all/search/202404.0/base-shop/best-practices/generic-faceted-search.md
index eefe86552cc..e969f8ec4b7 100644
--- a/docs/pbc/all/search/202404.0/base-shop/best-practices/generic-faceted-search.md
+++ b/docs/pbc/all/search/202404.0/base-shop/best-practices/generic-faceted-search.md
@@ -55,7 +55,7 @@ The main idea behind faceted search is to present the attributes of the document
To support faceted search, Elasticsearch offers a simple but powerful concept of aggregations. One of the nice features of aggregations is that they can be nested. In other words, you can define top-level aggregations that create "buckets" of documents and other aggregations that are executed inside those buckets on a subset of documents. The concept of aggregations is in general similar to the SQL `GROUP_BY` command (but much more powerful). Nested aggregations are analogous to SQL grouping but with multiple column names in the GROUP BY part of the query.
-### Indexing facet values
+## Indexing facet values
Before building aggregations, document attributes that can serve as facets need to be indexed in Elasticsearch. One way to index them is to list all attributes and their values under the same field like in the following example:
@@ -140,7 +140,7 @@ This requires special treatment in the mapping because otherwise, Elasticsearch
}
```
-### Facet queries
+## Facet queries
Filtering and aggregating a structure like this requires nested filters and nested aggregations in queries.
diff --git a/docs/pbc/all/search/202410.0/base-shop/best-practices/data-driven-ranking.md b/docs/pbc/all/search/202410.0/base-shop/best-practices/data-driven-ranking.md
index 3547e0d31a4..2ff67a56c19 100644
--- a/docs/pbc/all/search/202410.0/base-shop/best-practices/data-driven-ranking.md
+++ b/docs/pbc/all/search/202410.0/base-shop/best-practices/data-driven-ranking.md
@@ -51,7 +51,7 @@ When a query returns hundreds or thousands of results, the most relevant to the
A common solution is to manually assign ranks to products (sometimes even within categories). However, this approach is not practical for large catalogs and might result in a bad search experience—for example, when products that are out of stock are listed at the top because of their manually assigned rank.
-### Sorting by formulas based on scores
+## Sorting by formulas based on scores
We recommend an approach where a list of normalized scores per product at import time is precomputed and included in the documents that are sent to Elasticsearch. These are the scores from our previous hammer example (the interesting scores are left out because of the sensitivity of this information):
@@ -138,7 +138,7 @@ In queries, search results are sorted using an algebraic expression that combine
Very different kinds of scoring functions are conceivable, and the advantages of combining scores at query time are twofold: Your stakeholders (category and product managers) can help you in finding good formulas by testing the effect of different expressions on the sorting of actual queries at run-time. Second, you can use different ranking strategies on different parts of the website.
-### Computing normalized scores
+## Computing normalized scores
To combine scores in such expressions, we normalize them between 0 and 1 and try to make sure that they are more or less equally distributed across all documents. If, for example, the ranking formula is *0.3 * score_1 + 0.7 * score_2* and the scores are in the same range, then you could say that score_1 has a 30% influence on the outcome of the sorting and score_2 an influence of 70%. The equal distribution is important because if, for example, most documents have a very high score_2, then having a high score_2 becomes much more important for appearing at the top of the ranking than having a high score_1 (an effect that can be consciously used).
diff --git a/docs/pbc/all/search/202410.0/base-shop/best-practices/generic-faceted-search.md b/docs/pbc/all/search/202410.0/base-shop/best-practices/generic-faceted-search.md
index eefe86552cc..e969f8ec4b7 100644
--- a/docs/pbc/all/search/202410.0/base-shop/best-practices/generic-faceted-search.md
+++ b/docs/pbc/all/search/202410.0/base-shop/best-practices/generic-faceted-search.md
@@ -55,7 +55,7 @@ The main idea behind faceted search is to present the attributes of the document
To support faceted search, Elasticsearch offers a simple but powerful concept of aggregations. One of the nice features of aggregations is that they can be nested. In other words, you can define top-level aggregations that create "buckets" of documents and other aggregations that are executed inside those buckets on a subset of documents. The concept of aggregations is in general similar to the SQL `GROUP_BY` command (but much more powerful). Nested aggregations are analogous to SQL grouping but with multiple column names in the GROUP BY part of the query.
-### Indexing facet values
+## Indexing facet values
Before building aggregations, document attributes that can serve as facets need to be indexed in Elasticsearch. One way to index them is to list all attributes and their values under the same field like in the following example:
@@ -140,7 +140,7 @@ This requires special treatment in the mapping because otherwise, Elasticsearch
}
```
-### Facet queries
+## Facet queries
Filtering and aggregating a structure like this requires nested filters and nested aggregations in queries.