Ever notice how repetitive it is to reserve a study room at UMass? You have to go on a different website for each building and fill out the same form over and over again. Is there a way we can automate it somehow? Yes there is! Here comes UMass Room Reserve, a platform where you only needs to fill out the form once and we will fill it out for you so that you can book the room with ease!
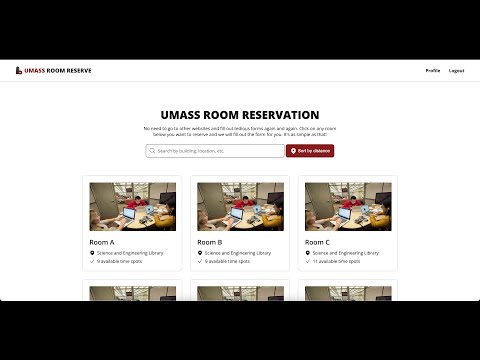
git clone https://github.com/weebao/umass-room-reserve.git
npm install
Below would be the optimal way to run the app. We decided to run the client and server on the same port so that we can redirect all routes to index.html
:
or
Since Milestone 2's criteria requires the website to be run on http-server
, you can run the command below to run the app. Make sure not to reload the page since this is a SPA which manipulates the URL so the page will not be able to load another page since they don't exist.
The backend is implemented using Node.js and Express.js. We have a simple server that authenticates user (need to have a UMass email account) and saves the user information inside the database.
Whenever the user books a room on our website, the backend will send a POST request to UMass's LibCal API, enter the user's information and book the room.
* Endpoints need to always start with /api
since the main path /
is serving the frontend.
GET
/api/user
(Retrieves user)
name |
type |
data type |
description |
email |
required |
string |
The user's email. |
http code |
content-type |
response |
200 |
application/json |
{"email":"<email>","name":"<name>","id":"<id>"} |
404 |
application/json |
{"message":"User not found"} |
500 |
application/json |
{"message":"Internal server error"} |
GET /api/user?email=richards@cs.umass.edu
PUT
/api/user
(Updates user)
name |
type |
data type |
description |
email |
required |
string |
The user's email. |
name |
type |
data type |
description |
firstName |
required |
string |
The user's first name. |
lastName |
required |
string |
The user's last name. |
role |
required |
string |
The user's role. ("Undergraduate", "Graduate", "Other") |
major |
required |
string |
The user's major. |
http code |
content-type |
response |
200 |
application/json |
{"email":"<email>","name":"<name>","id":"<id>"} |
404 |
application/json |
{"message":"User not found"} |
500 |
application/json |
{"message":"Internal server error"} |
PUT /api/user?email=richards@cs.umass.edu
Request Body:
{
"firstName": "Timothy D.",
"lastName": "Richards",
"role": "Other",
"major": "Computer Science"
}
DELETE
/api/user
(Deletes user)
name |
type |
data type |
description |
email |
required |
string |
The user's email. |
http code |
content-type |
response |
200 |
application/json |
{"message":"User deleted"} |
404 |
application/json |
{"message":"User not found"} |
500 |
application/json |
{"message":"Internal server error"} |
DELETE /api/user?email=richards@cs.umass.edu
POST
/api/user/login
(Logs in user)
name |
type |
data type |
description |
email |
required |
string |
The user's email. |
password |
required |
string |
The user's password. |
http code |
content-type |
response |
200 |
application/json |
{"email":"<email>","name":"<name>","id":"<id>"} |
400 |
application/json |
{"message":"Invalid credentials"} |
404 |
application/json |
{"message":"User not found"} |
500 |
application/json |
{"message":"Internal server error"} |
POST /api/user/login
Request Body:
{
"email": "richards@cs.umass.edu",
"password": "Iamthegoat"
}
POST
/api/user/register
(Registers user)
name |
type |
data type |
description |
email |
required |
string |
The user's email. |
password |
required |
string |
The user's password. |
firstName |
required |
string |
The user's first name. |
lastName |
required |
string |
The user's last name. |
role |
required |
string |
The user's role. ("Undergraduate", "Graduate", "Other") |
major |
required |
string |
The user's major. |
http code |
content-type |
response |
200 |
application/json |
{"email":"<email>","name":"<name>","id":"<id>"} |
400 |
application/json |
{"message":"Email already exists"} |
500 |
application/json |
{"message":"Internal server error"} |
POST /api/user/register
Request Body:
{
"email": "richards@cs.umass.edu",
"password": "Iamthegoat",
"firstName": "Tim",
"lastName": "Richards",
"role": "Other",
"major": "Computer Science"
}
GET
/api/room
(Retrieves rooms by ID)
name |
type |
data type |
description |
id |
required |
string |
The room's id. |
date |
optional |
string |
The date. (YYYY-MM-DD format) |
http code |
content-type |
response |
200 |
application/json |
{"id":"<id>","name":"<name>","buildingId":"<buildingId>","buildingName":"<buildingName>","availableTimes":["<availableTimes>"],"img":"<img>","checksums":["<checksums>"]} |
400 |
application/json |
{"message":"Room ID is required"} |
400 |
application/json |
{"message":"Invalid time range"} |
404 |
application/json |
{"message":"Room not found"} |
500 |
application/json |
{"message":"Internal server error"} |
GET
/api/room/all
(Retrieves all rooms within the time range or today if not specified)
name |
type |
data type |
description |
date |
optional |
string |
The date. (YYYY-MM-DD format) |
startTime |
optional |
string |
The start time. (HH:MM:SS format) |
endTime |
optional |
string |
The end time. (HH:MM:SS format) |
http code |
content-type |
response |
200 |
application/json |
{"rooms":[{"id":"<id>","name":"<name>","buildingId":"<buildingId>","buildingName":"<buildingName>","availableTimes":["<availableTimes>"],"img":"<img>","checksums":["<checksums>"]}]} |
400 |
application/json |
{"message":"Invalid time range"} |
GET /api/room/all?date=2021-04-21&startTime=08:00:00&endTime=10:00:00
POST
/api/room/book
(Books a room)
name |
type |
data type |
description |
id |
required |
string |
The room's id. |
date |
required |
string |
The date. (YYYY-MM-DD format) |
startTime |
required |
string |
The start time. (HH:MM:SS format) |
endTime |
required |
string |
The end time. (HH:MM:SS format) |
name |
type |
data type |
description |
firstName |
required |
string |
The user's first name. |
lastName |
required |
string |
The user's last name. |
email |
required |
string |
The user's email. |
studentRole |
required |
string |
The user's role. |
numPeople |
required |
("3-5" | "More than 5") |
The number of people. |
useComputer |
required |
("Yes" | "No" | "Maybe") |
Whether the user will use a computer. |
major |
required |
string |
The user's major. |
http code |
content-type |
response |
200 |
application/json |
{"message":"Room booked successfully"} |
400 |
application/json |
{"message":"Invalid time range"} |
400 |
application/json |
{"message":"Invalid form data"} |
404 |
application/json |
{"message":"Room not found"} |
500 |
application/json |
{"message":"Internal server error"} |
POST /api/room/book?id=1&date=2021-04-21&startTime=08:00:00&endTime=10:00:00
Request Body:
{
"firstName": "Tim",
"lastName": "Richards",
"email": "richards@cs.umass.edu",
"studentRole": "Other",
"numPeople": "3-5",
"useComputer": "Yes",
"major": "Computer Science"
}
-
Every commit/feature must be associated with an issue.
-
Contributors must create a new branch using the format <#issue>-<name>-<feature/description/etc>
.
-
Follow the code below:
git checkout -b "<branch-name>"
git add .
git commit -a -m "Message"
git push origin <branch-name>
-
Go to GitHub and create a pull request.