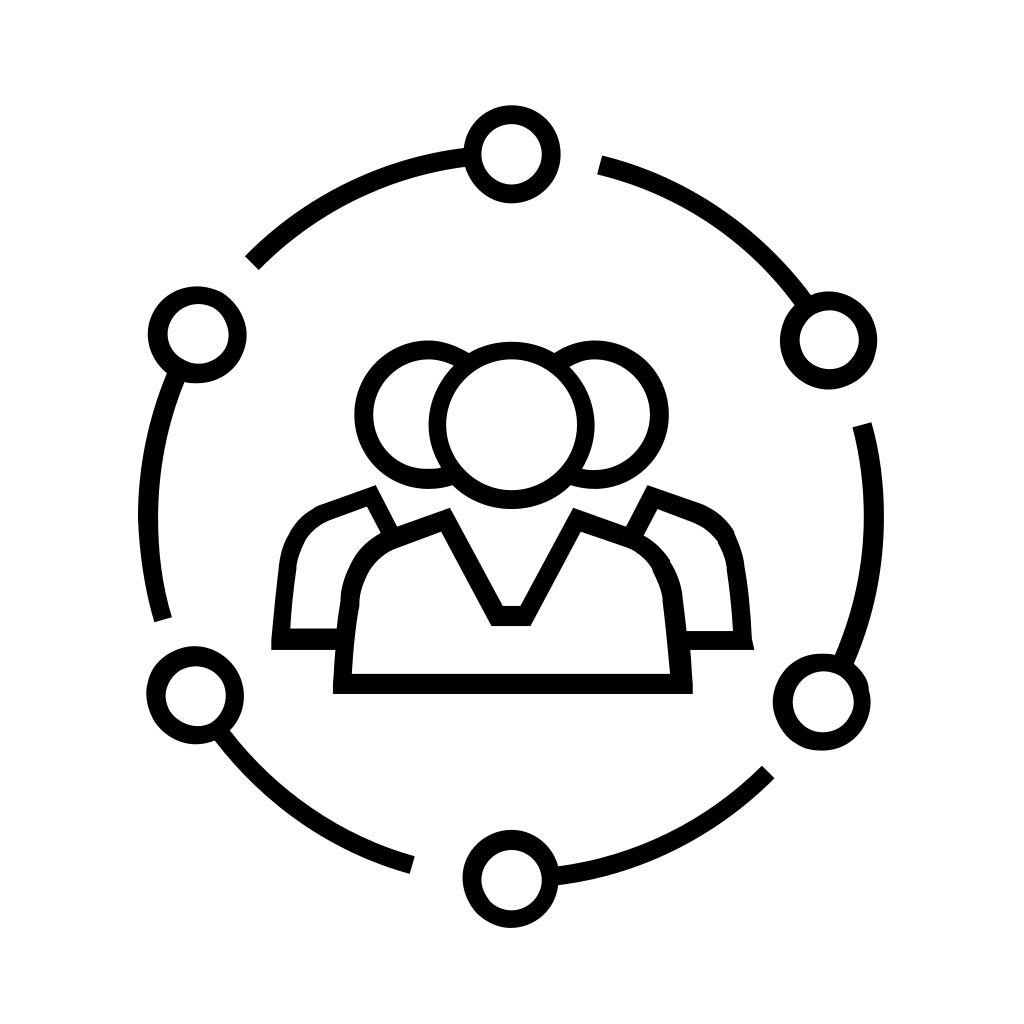
A web application project that was developed using Spring Boot framework, and supplies various RESTful API services to users, using various libraries such as Jackson, Spring HATEOAS, Swagger UI, Data REST.
Table of Contents
This project was developed fully in Java language, using Spring Boot framework.
In this project we implemented a system that manages users and offers a wide variety of functionalities. The user is offered not only the basic CRUD functionality, but rather get data about users using complex queries and conditions.
Throughout the whole project, RESTful representations were also implemented using HATEOAS library, Async programming was also used in this project by using CompletableFuture class, and data was fetched from 3 different API resources and mapped into POJOs using both RestTemplate and ObjectMapper.
In this project, one can create, get, edit and delete users, cell phone companies and/or perform manipulations with the data stored in the database.
Also, you may find several subjects such as:
- Java 11 Overview & Functional Programming.
- Multithreading and Java Streams.
- Concurrent Design Patterns and algorithms.
- Web Servers and Java Socket Programming.
- Spring Framework/Java EE overview: IoC and Dependency Injection.
- Introduction to Spring Boot and Web API development, Custom Exceptions and Handlers.
- Spring MVC: Beans, JPA, Hibernate, Lombok, openapi/Swagger and MVC Controllers.
- Spring Boot and RESTful representations using HATEOAS library Async programming using CompletableFuture<V>.
- RESTful API development and advanced HTTP response manipulation and Spring Data REST.
- Advanced Hibernate relations and RESTful representations assemblers.
- DAO/DTO using Jackson and JSON properties.
- Spring @Service and async tasks.
- Swagger UI – A library that allows us to visualize and interact with the APIs resources, expanding about each and every endpoint available.
- commons-io - A library of utilities to assist with developing IO functionality. We used this library in order to fetch a random user from external API.
- org.json – A library that contains JSON encoders/decoders implemented in Java, and allows us to convert JSON data to Java concrete data types, and much more..
- Lombok – A library that helps us to prevent boilerplate code in projects by using its annotations.
- jackson-databind – A library that offers the general-purpose data-binding functionality. In this project, we used Jackson ObjectMapper.
- H2 database - A library that allows us to use the H2 database functionality.
Follow these simple steps:
-
Clone the repo
git clone https://github.com/RamMichaeli17/Community.git
-
Run the program
press shift+F10 or press the "Run WebappApplication" button
-
Import requests to Postman using this link:
https://www.getpostman.com/collections/3ae56741681d12e2a393 -
You may enter to H2 DB UI using this link:
http://localhost:8080/h2 -
You may enter to Swagger-UI using this link:
http://localhost:8080/swagger-ui/index.html
** Increasing the speed of the video is recommended.
For more examples, please refer to the Documentation
Distributed under the MIT License. See LICENSE.txt
for more information.
We thank the following people who contributed to this project:
Ram Michaeli |
Yaniv Levi |
Tal Beno |
Ram Michaeli - ram153486@gmail.com
Project Link: https://github.com/RamMichaeli17/Community