-
Notifications
You must be signed in to change notification settings - Fork 12
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Merge pull request #68 from KarY15K/ControlStatements
feat(CPP):Add C++ Control Statements
- Loading branch information
Showing
12 changed files
with
3,765 additions
and
0 deletions.
There are no files selected for viewing
187 changes: 187 additions & 0 deletions
187
...rce/programming/CPP/C++ControlStatements/c-c-if-else-if-ladder-with-examples.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,187 @@ | ||
# C++ if-else if 梯级语句 | ||
|
||
条件判断在 C++ 中帮助根据某些条件执行特定的代码块。 | ||
|
||
在 C++ 中,**if-else-if 梯级语句** 允许用户在多个选项中进行选择。C++ 中的 if 语句从上到下执行。一旦某个条件为真,便会执行与该 if 语句相关的代码,且剩余的 else-if 结构将被跳过。如果所有条件都不为真,则最终的 else 语句将会被执行。 | ||
|
||
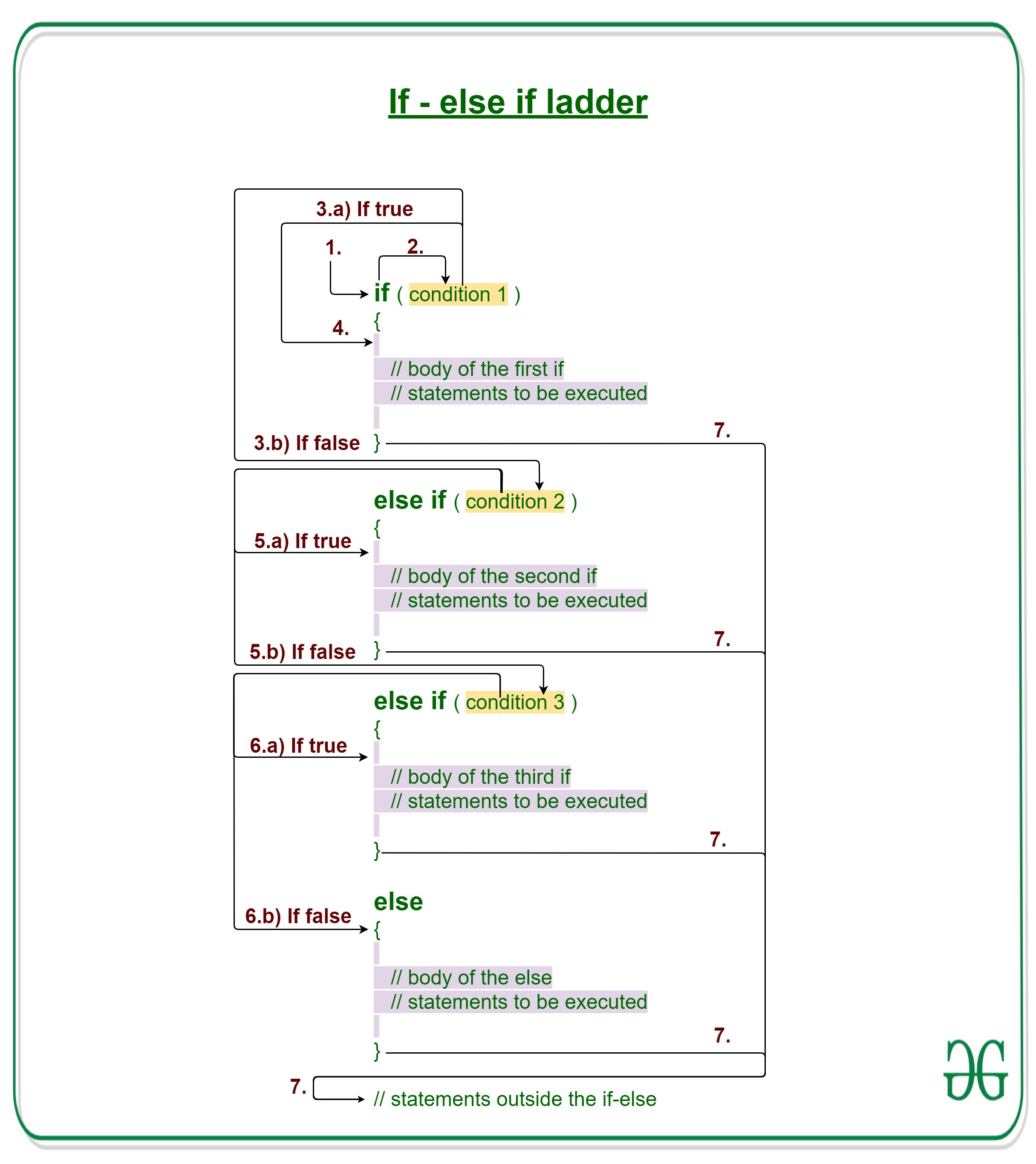 | ||
|
||
## 语法 | ||
|
||
``` | ||
if (condition) | ||
statement 1; | ||
else if (condition) | ||
statement 2; | ||
. | ||
. | ||
else | ||
statement; | ||
``` | ||
|
||
## 梯级 if-else-if 的工作原理 | ||
|
||
1. 控制权进入 if 块。 | ||
|
||
2. 流程跳转到条件 1。 | ||
|
||
3. 条件被测试。 | ||
|
||
如果条件为真,跳转到步骤 4。 | ||
|
||
如果条件为假,跳转到步骤 5。 | ||
|
||
4. 当前的代码块被执行,跳转到步骤 7。 | ||
|
||
5. 流程跳转到条件 2。 | ||
|
||
如果条件为真,跳转到步骤 4。 | ||
|
||
如果条件为假,跳转到步骤 6。 | ||
|
||
6. 流程跳转到条件 3。 | ||
|
||
如果条件为真,跳转到步骤 4。 | ||
|
||
如果条件为假,执行 else 代码块并跳转到步骤 7。 | ||
|
||
7. 退出 if-else-if 结构。 | ||
|
||
# C++ if else if Ladder | ||
|
||
条件判断在 C++ 中帮助根据某些条件执行特定的代码块。 | ||
|
||
在 C++ 中,**if-else-if ladder** 允许用户在多个选项中进行选择。C++ 中的 if 语句从上到下执行。一旦某个条件为真,便会执行与该 if 语句相关的代码,且剩余的 else-if ladder 将被跳过。如果所有条件都不为真,则最终的 else 语句将会被执行。 | ||
|
||
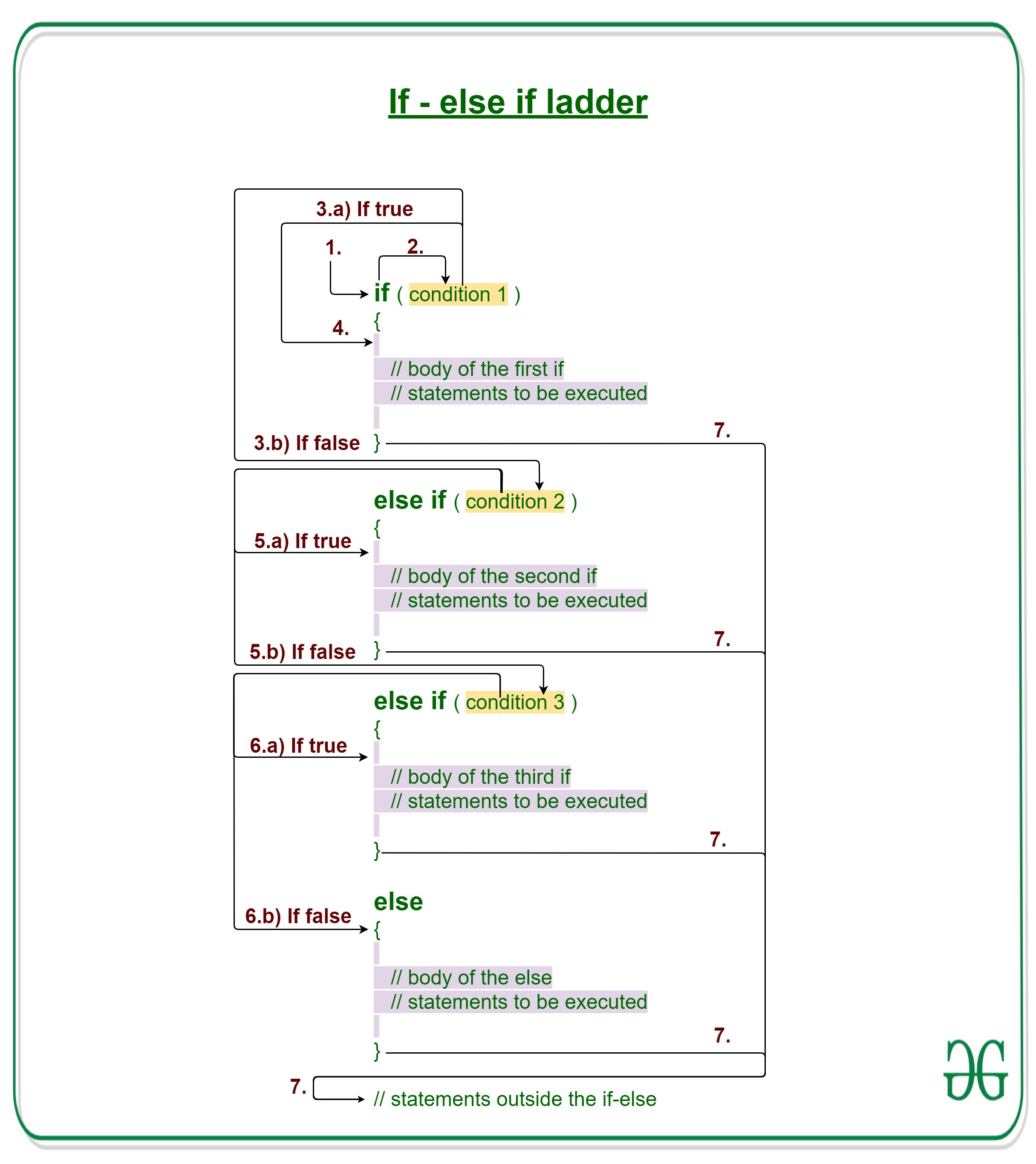 | ||
|
||
## **语法** | ||
|
||
```cpp | ||
if (condition) | ||
statement 1; | ||
else if (condition) | ||
statement 2; | ||
. | ||
. | ||
else | ||
statement; | ||
``` | ||
|
||
## **if-else-if ladder 的工作原理** | ||
|
||
1. 控制权进入 if 块。 | ||
2. 流程跳转到条件 1。 | ||
3. 条件被测试。 | ||
1. 如果条件为真,跳转到步骤 4。 | ||
2. 如果条件为假,跳转到步骤 5。 | ||
4. 当前的代码块被执行,跳转到步骤 7。 | ||
5. 流程跳转到条件 2。 | ||
1. 如果条件为真,跳转到步骤 4。 | ||
2. 如果条件为假,跳转到步骤 6。 | ||
6. 流程跳转到条件 3。 | ||
1. 如果条件为真,跳转到步骤 4。 | ||
2. 如果条件为假,执行 else 代码块并跳转到步骤 7。 | ||
7. 退出 if-else-if 结构。 | ||
|
||
## **if-else-if ladder 流程图** | ||
|
||
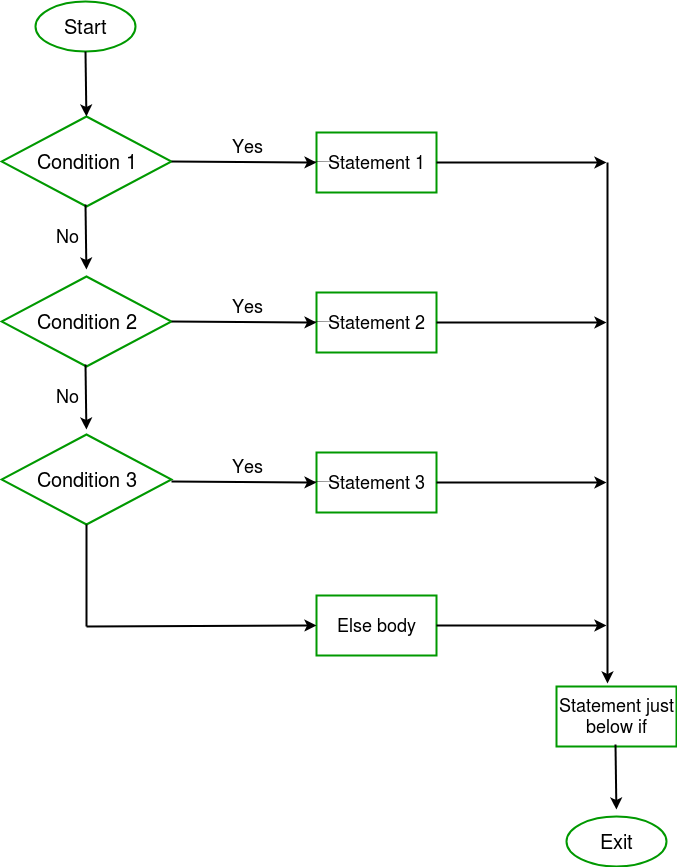 | ||
|
||
## 梯级 if-else-if 的示例 | ||
|
||
### 示例 1: | ||
|
||
以下程序演示了 C++ 中 if-else-if ladder 的使用。 | ||
|
||
- C++ | ||
|
||
```cpp | ||
// C++ program to illustrate if-else-if ladder | ||
#include <iostream> | ||
using namespace std; | ||
|
||
int main() | ||
{ | ||
int i = 20; | ||
|
||
// Check if i is 10 | ||
if (i == 10) | ||
cout << "i is 10"; | ||
|
||
// Since i is not 10 | ||
// Check if i is 15 | ||
else if (i == 15) | ||
cout << "i is 15"; | ||
|
||
// Since i is not 15 | ||
// Check if i is 20 | ||
else if (i == 20) | ||
cout << "i is 20"; | ||
|
||
// If none of the above conditions is true | ||
// Then execute the else statement | ||
else | ||
cout << "i is not present"; | ||
|
||
return 0; | ||
} | ||
``` | ||
|
||
**输出** | ||
|
||
``` | ||
i is 20 | ||
``` | ||
|
||
**解释:** | ||
|
||
- 程序开始。 | ||
- i 被初始化为 20。 | ||
- 检查条件 1:20 == 10,结果为假。 | ||
- 检查条件 2:20 == 15,结果为假。 | ||
- 检查条件 3:20 == 20,结果为真,因此输出 "i is 20"。 | ||
- 程序结束。 | ||
|
||
### 示例 2: | ||
|
||
另一个演示 C++ 中 if-else-if ladder 的示例。 | ||
|
||
- C++ | ||
|
||
```cpp | ||
// C++ program to illustrate if-else-if ladder | ||
|
||
#include <iostream> | ||
using namespace std; | ||
|
||
int main() | ||
{ | ||
int i = 25; | ||
|
||
// Check if i is between 0 and 10 | ||
if (i >= 0 && i <= 10) | ||
cout << "i is between 0 and 10" << endl; | ||
|
||
// Since i is not between 0 and 10 | ||
// Check if i is between 11 and 15 | ||
else if (i >= 11 && i <= 15) | ||
cout << "i is between 11 and 15" << endl; | ||
|
||
// Since i is not between 11 and 15 | ||
// Check if i is between 16 and 20 | ||
else if (i >= 16 && i <= 20) | ||
cout << "i is between 16 and 20" << endl; | ||
|
||
// Since i is not between 0 and 20 | ||
// It means i is greater than 20 | ||
else | ||
cout << "i is greater than 20" << endl; | ||
} | ||
``` | ||
|
||
**输出** | ||
|
||
``` | ||
i is greater than 20 | ||
``` |
202 changes: 202 additions & 0 deletions
202
...rce/programming/CPP/C++ControlStatements/c-c-if-else-statement-with-examples.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,202 @@ | ||
# C++ if else 语句 | ||
|
||
条件判断在 C++ 中有助于根据某些条件执行特定代码块。 | ||
|
||
*if* 语句本身用于检查条件是否为真,如果条件为真,则执行代码块;如果条件为假,则不执行。但是,如果条件为假时我们希望执行其他操作,则可以使用 C++ 中的 **else 语句**。我们可以将 else 语句与 if 语句配合使用,在条件为假时执行特定的代码块。 | ||
|
||
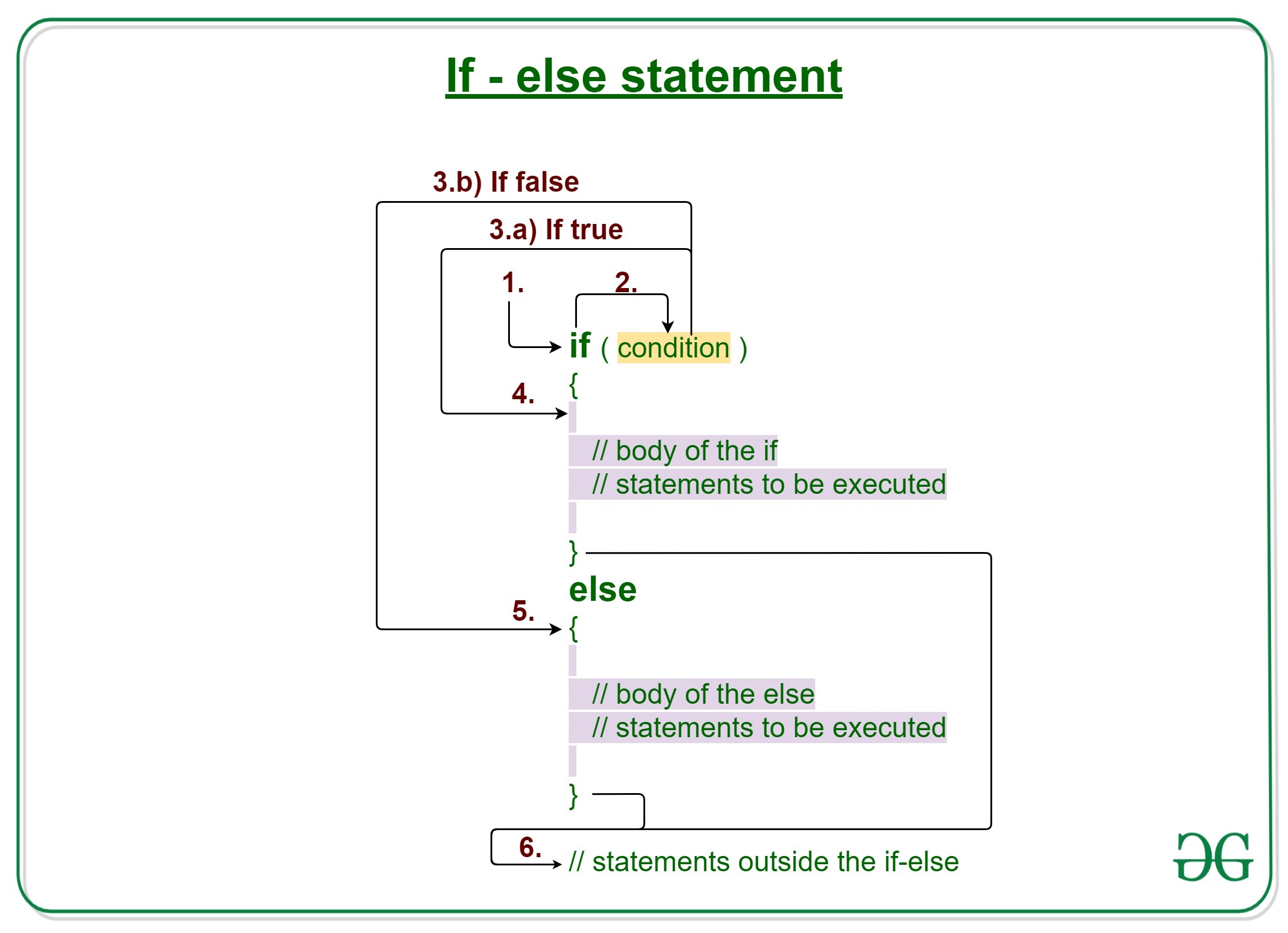 | ||
|
||
## 语法 | ||
|
||
``` | ||
if (condition) | ||
{ | ||
// Executes this block if | ||
// condition is true | ||
} | ||
else | ||
{ | ||
// Executes this block if | ||
// condition is false | ||
} | ||
``` | ||
|
||
## if-else 语句的工作原理 | ||
|
||
1. 控制权进入 if 块。 | ||
|
||
2. 流程跳转到条件。 | ||
|
||
3. 条件被测试。 | ||
|
||
如果条件为真,跳转到步骤 4。 | ||
|
||
如果条件为假,跳转到步骤 5。 | ||
|
||
4. 执行 if 块中的代码。 | ||
|
||
5. 执行 else 块中的代码。 | ||
|
||
6. 流程退出 if-else 块。 | ||
|
||
## if-else 流程图 | ||
|
||
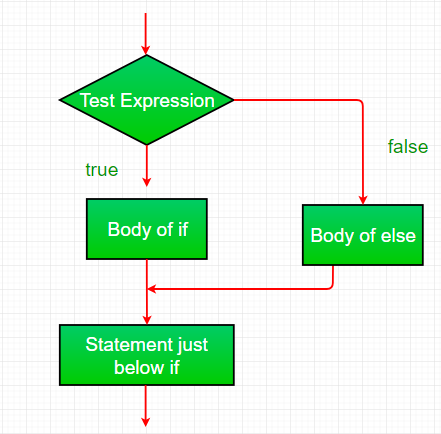 | ||
|
||
## C++ 中 if else 语句的示例 | ||
|
||
### 示例 1: | ||
|
||
下面的程序演示了 C++ 中 if else 语句的使用。 | ||
|
||
- C++ | ||
|
||
```cpp | ||
// C++ program to illustrate if-else statement | ||
|
||
#include <iostream> | ||
using namespace std; | ||
|
||
int main() | ||
{ | ||
int i = 20; | ||
|
||
// Check if i is 10 | ||
if (i == 10) | ||
cout << "i is 10"; | ||
|
||
// Since is not 10 | ||
// Then execute the else statement | ||
else | ||
cout << "i is 20\n"; | ||
|
||
cout << "Outside if-else block"; | ||
|
||
return 0; | ||
} | ||
``` | ||
|
||
**输出** | ||
|
||
``` | ||
i is 20 | ||
Outside if-else block | ||
``` | ||
|
||
**解释:** | ||
|
||
- 程序开始。 | ||
- i 被初始化为 20。 | ||
- 检查 if 条件。i == 10,结果为假。 | ||
- 流程进入 else 块。 | ||
- 输出 "i is 20"。 | ||
- 输出 "Outside if-else block"。 | ||
|
||
### 示例 2: | ||
|
||
# C++ if else 语句 | ||
|
||
条件判断在 C++ 中有助于根据某些条件执行特定代码块。 | ||
|
||
*if* 语句本身用于检查条件是否为真,如果条件为真,则执行代码块;如果条件为假,则不执行。但是,如果条件为假时我们希望执行其他操作,则可以使用 C++ 中的 **else 语句**。我们可以将 else 语句与 if 语句配合使用,在条件为假时执行特定的代码块。 | ||
|
||
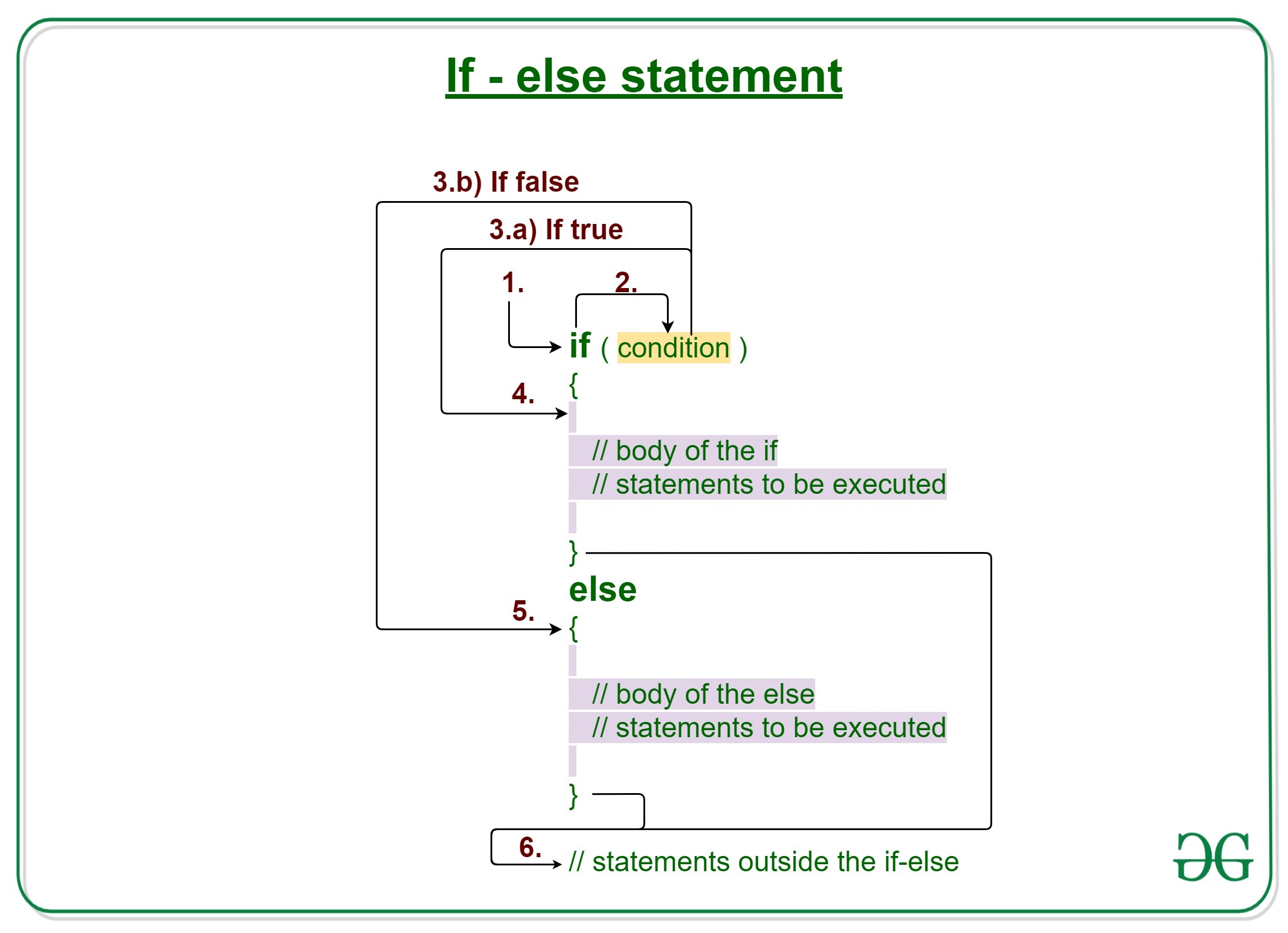 | ||
|
||
## **语法** | ||
|
||
``` | ||
arduino复制代码if (condition) | ||
{ | ||
// 当条件为真时执行该代码块 | ||
} | ||
else | ||
{ | ||
// 当条件为假时执行该代码块 | ||
} | ||
``` | ||
|
||
## **if-else 语句的工作原理** | ||
|
||
1. 控制权进入 if 块。 | ||
2. 流程跳转到条件。 | ||
3. 条件被测试。 | ||
1. 如果条件为真,跳转到步骤 4。 | ||
2. 如果条件为假,跳转到步骤 5。 | ||
4. 执行 if 块中的代码。 | ||
5. 执行 else 块中的代码。 | ||
6. 流程退出 if-else 块。 | ||
|
||
## **if-else 流程图** | ||
|
||
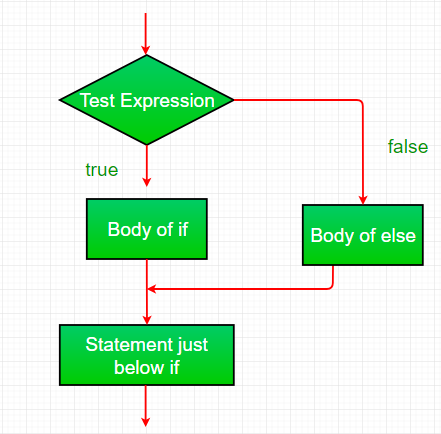 | ||
|
||
## C++ 中 if else 语句的示例 | ||
|
||
### **示例 1:** | ||
|
||
下面的程序演示了 C++ 中 if else 语句的使用。 | ||
|
||
``` | ||
cpp复制代码#include <iostream> | ||
using namespace std; | ||
int main() { | ||
int i = 20; | ||
// 检查 i 是否等于 10 | ||
if (i == 10) | ||
cout << "i is 10"; | ||
else | ||
cout << "i is 20\n"; | ||
cout << "Outside if-else block"; | ||
return 0; | ||
} | ||
``` | ||
|
||
**输出** | ||
|
||
``` | ||
csharp复制代码i is 20 | ||
Outside if-else block | ||
``` | ||
|
||
**解释:** | ||
|
||
- 程序开始。 | ||
- i 被初始化为 20。 | ||
- 检查 if 条件。i == 10,结果为假。 | ||
- 流程进入 else 块。 | ||
- 输出 "i is 20"。 | ||
- 输出 "Outside if-else block"。 | ||
|
||
### **示例 2:** | ||
|
||
另一个展示 C++ 中 if else 使用的程序。 | ||
|
||
- C++ | ||
|
||
```cpp | ||
// C++ program to illustrate if-else statement | ||
|
||
#include <iostream> | ||
using namespace std; | ||
|
||
int main() | ||
{ | ||
int i = 25; | ||
|
||
if (i > 15) | ||
cout << "i is greater than 15"; | ||
else | ||
cout << "i is smaller than 15"; | ||
|
||
return 0; | ||
} | ||
``` | ||
|
||
**输出** | ||
|
||
``` | ||
i is greater than 15 | ||
``` |
Oops, something went wrong.