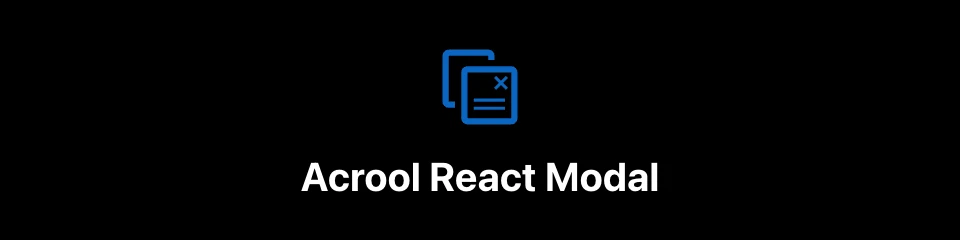
This is a toast message function for React development notifications
- Supports queue modal list
- Plug and unplug using
@acrool/react-portal
andframer-motion
- Quickly create light box effects and send them to the outside to avoid hierarchical problems
- Support @acrool/react-router-hash lightbox (using createControlledModal)
- Modal open auto add
acrool_model-open
body overflow style class - export BodyScroll utils (state control)
yarn add framer-motion @acrool/react-modal
in your packages. (Make the version of styled-component you use match the version of styled-component used in acrool-react-gird)
"resolutions": {
"framer-motion": "^11.x"
}
add in your index.tsx
import "@acrool/react-modal/dist/index.css";
add in your App.tsx
It should be noted that it must be within the scope of
Router Provider
. Another way is to place it inLayout
andOutlet
middle layer.
import {ModalPortal} from "@acrool/react-modal";
const App = () => {
return (
<div>
<BaseUsed/>
<ModalPortal/>
</div>
);
};
- Here are two ways to use it
Add the lightbox to the display column list by throwing the Show method
Defined Modal
import {animation, createModal, IModalOptions, useModal} from '@acrool/react-modal';
interface IProps {
myVar: string
}
const PromotionModal = (args: IProps) => {
const {hide} = useModal();
return <div>
<div>Test2 content</div>
<button type="button" onClick={hide}>X </button>
</div>;
}
export default createModal(
PromotionModal,
animation.generateFadeIn(),
);
Use Modal
then in your page
const ExamplePage = () => {
return <div>
<button type="button" onClick={() => PromotionModal.show({myVar: 'Imageine'})}>Show Modal</button>
</div>
}
The inside of the light box is controlled by its own state, which is displayed through rendering, such as using HashRouter.
Defined Modal
import {animation, createStateModal, IModalOptions, useModal} from '@acrool/react-modal';
import {useHashParams} from '@acrool/react-router-hash';
const PromotionHashModal = () => {
const {hide} = useModal();
const navigate = useNavigate();
const {id} = useHashParams<{id: string}>();
useEffect(() => {
return () => {
navigate({...location, hash: undefined});
};
}, []);
const handleOnHide = () => {
hide();
};
// const handleOnClose = () => {
// hide().then(() => {
// navigate({hash: undefined});
// })
// }
return <div>
<div>Test2 content</div>
<button type="button" onClick={handleOnClose}>Close Modal</button>
</div>;
}
export default createStateModal(
PromotionHashModal,
{
...animation.generateFadeIn(),
isEnableHideWithClickMask: true,
},
);
Defined Hash Route
It should be noted that it must be within the scope of
Router Provider
. Another way is to place it inLayout
andOutlet
middle layer.
import {HashRoute,HashRoutes} from '@acrool/react-router-hash';
import {createBrowserHistory} from 'history';
import {BrowserRouter as Router,Route, Routes} from 'react-router-dom';
const history = createBrowserHistory({window});
const RouterSetting = () => {
return <Router>
<Routes>
<Route path="/" element={<Example/>} />
</Routes>
<HashRoutes>
<HashRoute path="promotion/:id" element={<PromotionHashModal/>}/>
</HashRoutes>
{/* Add this */}
<ModalPortal/>
</Router>;
};
Use Modal
then in your page
import {useNavigate} from 'react-router-dom';
const ExamplePage = () => {
const navigate = useNavigate();
return <div>
<button type="button" onClick={() => navigate({hash: '/promotion/1'})}>Show Modal</button>
<button type="button" onClick={() => navigate({hash: '/promotion/2'})}>Show Modal</button>
</div>
}
- animation
- fadeInDown: (default), ex Base modal style
- zoomInDown
- slideInLeft: ex Drawer slider
- slideInRight: ex Drawer slider
- slideInUp: ex Dropdown
- custom (ref; https://www.framer.com/motion/animate-presence/#usage)
variants = { initial: {opacity: 0, y: -20, transition: {type:'spring'}}, animate: {opacity: 1, y: 0}, exit: {opacity: 0, y: -40} }
There is also a example that you can play with it: