-
Notifications
You must be signed in to change notification settings - Fork 0
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
1 parent
9ec577d
commit 8323fcb
Showing
9 changed files
with
1,103 additions
and
21 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,3 @@ | ||
# pydash-cheatsheet | ||
|
||
A simplified reference for pydash. |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,3 @@ | ||
# About | ||
|
||
This page is meant to be a starting point and quick reference for [pydash](https://pydash.readthedocs.io/en/latest/index.html), containing some of its most widely useful functions that aren't easily covered by modern Python. I also used this page as a project to get more familiarized with pydash and generating documentation programatically with [Material for MkDocs](https://squidfunk.github.io/mkdocs-material/). Any suggestions are welcome at [the repo](https://github.com/brunodantas/pydash-cheatsheet/issues). |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,26 @@ | ||
# pydash-cheatsheet | ||
|
||
A simplified reference for [pydash](https://pydash.readthedocs.io/en/latest/index.html). | ||
|
||
<div class="grid" markdown> | ||
|
||
{% for function in functions %} | ||
|
||
### {{ function[0] }} | ||
|
||
{{ function[1] }} | ||
|
||
=== "Example" | ||
|
||
```py | ||
>>> {{function[2] }} | ||
{{function[3]}} | ||
``` | ||
|
||
=== "Docstring" | ||
|
||
```{{ function[4] }}``` | ||
|
||
{% endfor %} | ||
|
||
</div> |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,3 +1 @@ | ||
# pydash-sheetcheat | ||
|
||
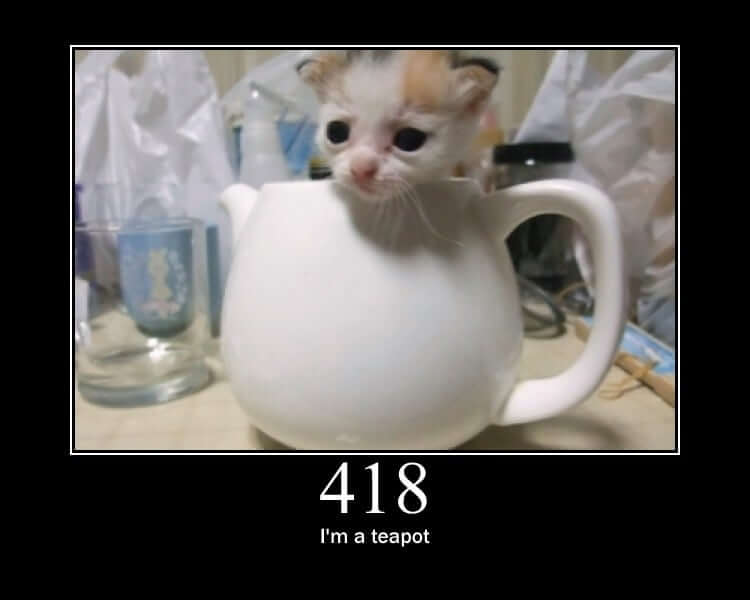 | ||
[English](en/index.md) |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,15 @@ | ||
from pydash import * | ||
import maps | ||
|
||
|
||
def define_env(env): | ||
function_map = maps.functions | ||
functions = [] | ||
for fun in function_map: | ||
title = fun.function.__name__ | ||
description = fun.description | ||
example = fun.test_case | ||
result = eval(example) | ||
docstring = fun.function.__doc__ | ||
functions.append((title, description, example, result, docstring)) | ||
env.variables.functions = functions |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,106 @@ | ||
from dataclasses import dataclass | ||
from typing import Callable | ||
from pydash import * | ||
|
||
|
||
@dataclass | ||
class Map: | ||
function: Callable | ||
description: str | ||
test_case: str | ||
|
||
|
||
functions = [ | ||
Map( | ||
get, | ||
"Easy access to properties at any level", | ||
"get({'a': {'b': 1}, 'c': 2}, 'a.b')", | ||
), | ||
Map( | ||
set_, | ||
"Set property at any level\n\nVariants: set_with", | ||
"set_({'a': 2}, 'b.c', 3)", | ||
), | ||
Map( | ||
has, | ||
"Check if property exists at any level", | ||
"has({'a': {'b': 1}, 'c': 2}, 'a.d')", | ||
), | ||
Map( | ||
find, | ||
"Find first element that passes a criterion function\n\nVariants: find_index, find_last, find_last_index", | ||
"find([1, 2, 3, 4], lambda n: n > 2)", | ||
), | ||
Map( | ||
index_of, | ||
"Find the index of an element\n\nVariants: last_index_of", | ||
"index_of([1, 2, 3, 2], 2)", | ||
), | ||
Map( | ||
pluck, | ||
"Retrieve property values from collections", | ||
"pluck([{'a': 1}, {'a': 2, 'b': 3}, {'a': 4}, {}], 'a')", | ||
), | ||
Map( | ||
pull, | ||
"Removes specific elements from array\n\nVariants: pull_all_by, pull_all_with, pull_at, remove, take, without", | ||
"pull([1, 2, 3, 4, 3, 2], 2, 3)", | ||
), | ||
Map( | ||
rename_keys, | ||
"New dict with renamed keys", | ||
"rename_keys({'a': 1, 'b': 2}, {'a': 'A', 'b': 'B'})", | ||
), | ||
Map( | ||
flatten, | ||
"Flatten an array by one level\n\nVariants: flatten_deep, flatten_depth", | ||
"flatten([1, [2, [3, [4]], 5]])", | ||
), | ||
Map(count_by, "Count occurences of values in sequence", "count_by('mississipi')"), | ||
Map( | ||
camel_case, | ||
"Text to camel case\n\nVariants: kebab_case, human_case, snake_case, slugify etc", | ||
"camel_case('Foo Bar')", | ||
), | ||
Map( | ||
sample_size, | ||
"Retrieve random sample of elements", | ||
"sample_size([1, 2, 3, 4], 2)", | ||
), | ||
Map( | ||
chunk, | ||
"Separate elements into chunks", | ||
"chunk([1, 2, 3, 4, 5, 6, 7, 8, 9, 10], 3)", | ||
), | ||
Map(compact, "Take falsey values out", "compact([0, 1, False, 2, '', 3])"), | ||
Map( | ||
difference, | ||
"Return elements that are in the first array but not in the others\n\nVariants: difference_by, difference_with", | ||
"difference([1, 2], [2, 3], [4, 5])", | ||
), | ||
Map( | ||
take_while, | ||
"Conditional slice\n\nVariants: drop_right_while, drop_while, drop_right_while", | ||
"take_while([1, 2, 3, 4], lambda n: n < 3)", | ||
), | ||
Map(duplicates, "Returns duplicate values", "duplicates([1, 2, 3, 4, 2, 3])"), | ||
Map( | ||
attempt, "Return function result or caught exception", "attempt(lambda: 1 / 0)" | ||
), | ||
Map(memoize, "Cache function results", "memoize(sum).cache"), | ||
Map( | ||
debounce, | ||
"Delay execution of a function\n\nVariants: delay", | ||
"debounce(lambda: print('Hello'), 1000)()", | ||
), | ||
Map( | ||
curry, | ||
"Partial application of functions", | ||
"curry(lambda a, b, c: a + b + c)(1)(2)(3)", | ||
), | ||
Map( | ||
xor, | ||
"Exclusive or of sequences\n\nVariants: xor_by, xor_with", | ||
"xor([1, 2], [2, 3], [3, 4])", | ||
), | ||
] |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Oops, something went wrong.