-
Notifications
You must be signed in to change notification settings - Fork 4
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Merge pull request #9 from datasparq-ai/release/v0.5.1
Release v0.5.1
- Loading branch information
Showing
13 changed files
with
406 additions
and
133 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,31 @@ | ||
name: goreleaser | ||
|
||
on: | ||
push: | ||
tags: | ||
- '*' | ||
|
||
permissions: | ||
contents: write | ||
|
||
jobs: | ||
goreleaser: | ||
runs-on: ubuntu-latest | ||
steps: | ||
- | ||
name: Checkout | ||
uses: actions/checkout@v4 | ||
with: | ||
fetch-depth: 0 | ||
- | ||
name: Set up Go | ||
uses: actions/setup-go@v4 | ||
- | ||
name: Run GoReleaser | ||
uses: goreleaser/goreleaser-action@v5 | ||
with: | ||
distribution: goreleaser | ||
version: latest | ||
args: release --clean | ||
env: | ||
GITHUB_TOKEN: ${{ secrets.GITHUB_TOKEN }} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,97 +1,55 @@ | ||
|
||
# Houston | ||
|
||
Houston is an open source, API based workflow orchestration tool. | ||
Open source, API based workflow orchestration tool. | ||
|
||
Documentation: [./docs](./docs/README.md) | ||
Homepage: [callhouston.io](https://callhouston.io) | ||
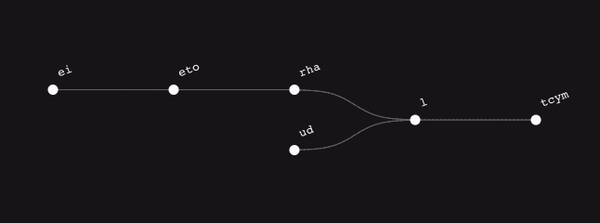 | ||
|
||
- Homepage: [callhouston.io](https://callhouston.io) | ||
- Quickstart guide: [houston-quickstart-python](https://github.com/datasparq-intelligent-products/houston-quickstart-python) | ||
- Docs: [./docs](./docs/README.md) | ||
|
||
This repo contains the API server, go client, and CLI. | ||
|
||
### Install | ||
|
||
If you have [go](https://golang.org/doc/install) installed you can install with: | ||
### Example Usage | ||
|
||
```bash | ||
go install github.com/datasparq-ai/houston | ||
``` | ||
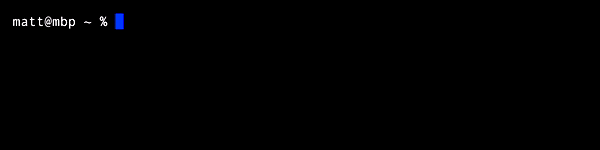 | ||
|
||
### Example Usage / Quickstart (1 minute) | ||
Start a local server with the default config: `houston api` | ||
|
||
Use `houston demo` to quickly run an end-to-end example workflow: | ||
Quickly run an end-to-end example workflow: `houston demo` | ||
|
||
```bash | ||
houston demo | ||
``` | ||
Or use the Docker container: `docker run -p 8000:8000 datasparq/houston-redis demo` | ||
|
||
Alternatively, start a local Houston server with the default config: | ||
See the quickstart for a guide on how to create microservices and complete Houston missions using them: | ||
[quickstart](https://github.com/datasparq-intelligent-products/houston-quickstart-python) | ||
|
||
```bash | ||
houston api | ||
``` | ||
|
||
The server is now running at `localhost:8000`. The Houston client will automatically look for Houston API servers | ||
running at this location. | ||
### Install | ||
|
||
(in a separate shell) Create a new Houston key with ID = 'quickstart': | ||
If you have [go](https://golang.org/doc/install) installed you can install with: | ||
|
||
```bash | ||
houston create-key --id quickstart --name "Houston Quickstart" | ||
go install github.com/datasparq-ai/houston | ||
``` | ||
|
||
Save this example plan to local file, e.g. 'example_plan.yaml': | ||
|
||
```yaml | ||
name: apollo | ||
stages: | ||
- name: engine-ignition | ||
- name: engine-thrust-ok | ||
upstream: | ||
- engine-ignition | ||
- name: release-holddown-arms | ||
- name: umbilical-disconnected | ||
- name: liftoff | ||
upstream: | ||
- engine-thrust-ok | ||
- release-holddown-arms | ||
- umbilical-disconnected | ||
``` | ||
|
||
Start a mission using this plan: | ||
### Why Houston? | ||
|
||
```bash | ||
export HOUSTON_KEY=quickstart | ||
houston start --plan example_plan.yaml | ||
``` | ||
Houston is a simpler, faster, and cheaper alternative to tools like Airflow. | ||
|
||
Then go to http://localhost:8000. Enter your Houston key 'quickstart'. | ||
API based orchestration comes with 5 key advantages: | ||
1. Code can run on serverless tools: lower cost, less maintenance, infinite scale | ||
2. The server isn't under heavy load, so can handle hundreds of concurrent missions | ||
3. Pub/Sub message delivery is guaranteed, improving reliability | ||
4. Multiple workflows can share the same task runners, aiding collaboration | ||
5. Task runners can run anywhere in any language, allowing for rapid development with no vendor lock-in | ||
|
||
You've created a plan and started a mission. You now need a microservice to complete each of the stages in this mission. | ||
See the quickstart for a guide on how to create microservices and complete Houston missions using them: | ||
[quickstart](https://github.com/datasparq-intelligent-products/houston-quickstart-python) | ||
|
||
### Contributing | ||
|
||
Please see the [contributing](./docs/contributing.md) guide. | ||
|
||
Development of Houston is supported by [Datasparq](https://datasparq.ai). | ||
|
||
### Run Unit Tests | ||
|
||
Test with development database: | ||
```bash | ||
go test ./... | ||
``` | ||
|
||
Test with Redis database: | ||
```bash | ||
# remove any existing redis database | ||
rm dump.rdb | ||
# prevent go from using cached test results | ||
go clean -testcache | ||
# create redis db | ||
redis-server & | ||
go test ./... | ||
# stop redis db | ||
kill $! | ||
``` |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,3 +1,68 @@ | ||
|
||
# Houston Go Client | ||
|
||
Example usage: | ||
|
||
```go | ||
package main | ||
|
||
import "github.com/datasparq-ai/houston/client" | ||
|
||
func main() { | ||
|
||
// provide a key and base URL to initialise the client | ||
houston := client.New("my-houston-key", "https://houston.example.com") | ||
err := houston.SavePlan("./my_plan.json") | ||
|
||
} | ||
``` | ||
|
||
The client will use environment variables by default: | ||
|
||
```go | ||
// this uses the 'HOUSTON_KEY' and 'HOUSTON_BASE_URL' environment variables for key and baseUrl respectively | ||
houston := client.New("", "") | ||
``` | ||
|
||
```go | ||
houston := client.New("", "") | ||
|
||
// we have previously saved a plan with this name | ||
// a mission ID is not provided, so one will be created for us | ||
res, err := houston.CreateMission("my-plan", "") | ||
|
||
// trigger first stage | ||
... | ||
``` | ||
|
||
The same can be achieved with the 'start' command. See [commands](../docs/commands.md): | ||
|
||
```go | ||
houston := client.New("", "") | ||
res, err := houston.Start("my-plan", "", nil, nil, nil) | ||
``` | ||
|
||
It may be easier to start missions with the command line tool. The equivalent would be: | ||
|
||
```bash | ||
houston start --plan my-plan | ||
``` | ||
|
||
Within services, the client is used to start and finish the stage: | ||
|
||
```go | ||
// get mission ID and stage name from trigger message | ||
... | ||
|
||
houston := client.New("", "") | ||
res, err = houston.StartStage(missionId, stageName, false) | ||
|
||
// complete task | ||
... | ||
|
||
res, err = houston.FinishStage(missionId, stageName, false) | ||
for _, nextStage := range res.Next { | ||
// trigger stage | ||
... | ||
} | ||
``` |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Oops, something went wrong.