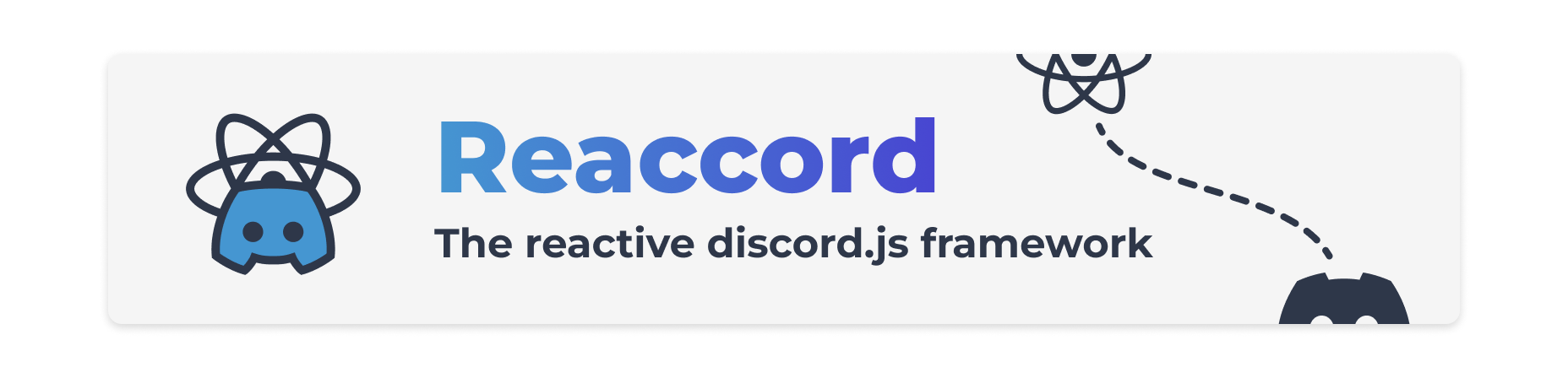
Reaccord is a framework for creating discord bots with react.
It is built on top of the discord.js library and is designed to be easy to use.
Turn your react code into fully featured discord bots now!
What we will be building:
You can view the complete source code here.
Define your App's behavior, as you would do in a regular React webapp.
const Counter = ({ start = 0 }) => {
const [count, setCount] = useState(start)
return (
<>
{count}
<Button onClick={() => setCount(count + 1)}>+</Button>
</>
)
}
Create end-user command.
const counterCommand = createSlashCommand("counter", "A simple counter")
.intParam("start", "Number to start counting from")
.render(({ start }) => <Counter start={start} />)
Instantiate the gateway client, and register the command.
const client = createClient({
token: "bot-token",
intents: [GatewayIntentBits.Guilds, GatewayIntentBits.GuildMessages],
devGuildId: "dev-guild-id",
clientId: "bot-client-id",
commands: [counterCommand],
})
Connect the client to discord's gateway
client.connect(() =>
console.log(`🚀 Client connected as ${client.user?.username}!`),
)