-
Notifications
You must be signed in to change notification settings - Fork 0
3. CSS
Deepak Ramalingam edited this page Feb 18, 2023
·
7 revisions
We will use CSS to style our pages.
CSS, also known as Cascading Style Sheets is a style sheet language used for describing the presentation of a document.
Good resource to learn CSS: https://www.w3schools.com/css/
We will use CSS to style index.html
and rate-movie.html
In the header we will link the style sheet file and define classes for each relevant element
<!DOCTYPE html>
<html>
<head>
<!-- Title of Webpage (appears in tab name) -->
<title>Spicy Tomatoes</title>
<!-- Link CSS Stylesheet -->
<link rel="stylesheet" href="index.css">
<!-- Fix Scaling of Website on Mobile Devices -->
<meta id="Viewport" name="viewport" content="initial-scale=1, maximum-scale=1, minimum-scale=1, user-scalable=no">
</head>
<body>
<!-- Navbar -->
<div class="navbarContainer">
<img width="50px" height="50px" src="https://d35lsekltq0ycz.cloudfront.net/web-arcade/tomato-transparent.png"/>
<h1>Spicy Tomatoes</h1>
</div>
<!-- Write Review Button -->
<a href="rate-movie.html" class="linkBtn">Review Movie</a>
<!-- List of Ratings for Movies -->
<div class="reviewList">
<!-- Example Movie Rating -->
<div class="reviewContainer">
<p class="movieName">Movie Name 1</p>
<p class="reviewText">Review 1</p>
</div class="reviewContainer">
<!-- Example Movie Rating -->
<div class="reviewContainer">
<p class="movieName">Movie Name 2</p>
<p class="reviewText">Review 2</p>
</div>
<!-- Example Movie Rating -->
<div class="reviewContainer">
<p class="movieName">Movie Name 3</p>
<p class="reviewText">Review 3</p>
</div>
</div>
</body>
</html>
Then, we will define our styles in index.css
body {
margin: 0;
display: flex;
flex-direction: column;
row-gap: 20px;
overflow-y: scroll;
overflow-x: hidden;
}
/* Navbar */
.navbarContainer {
display: flex;
flex-direction: row;
align-items: center;
column-gap: 10px;
padding: 10px;
background-color: rgb(250, 50, 9);
}
.navbarContainer > img {
background-color: white;
border-radius: 100%;
padding: 8px;
}
.navbarContainer > h1 {
font-size: 2.4rem;
color: white;
margin: 0;
}
/* Review Movie and Back Links / Buttons */
.linkBtn {
text-align: center;
color: red;
font-size: 20px;
}
.linkBtn:link {
text-decoration: none;
}
.linkBtn:visited {
text-decoration: none;
}
.linkBtn:hover {
text-decoration: underline;
}
.linkBtn:active {
text-decoration: none;
}
/* Review List */
.reviewList {
display: flex;
flex-direction: column;
align-items: center;
row-gap: 30px;
}
/* Review Item */
.reviewContainer {
border-style: dashed;
border: 1px 1px 1px 1px black;
width: min(90%, 600px);
padding: 10px;
border-radius: 10px;
border-width: 4px;
}
.movieName {
font-weight: bold;
text-align: center;
font-size: 1.2rem;
}
.reviewText {
text-align: start;
font-size: 1rem;
}
In the header we will link the style sheet file and define classes for each relevant element
<!DOCTYPE html>
<html>
<head>
<!-- Title of Webpage (appears in tab name) -->
<title>Spicy Tomatoes</title>
<!-- Link CSS Stylesheet -->
<link rel="stylesheet" href="index.css">
<!-- Fix Scaling of Website on Mobile Devices -->
<meta id="Viewport" name="viewport" content="initial-scale=1, maximum-scale=1, minimum-scale=1, user-scalable=no">
</head>
<body>
<!-- Navbar -->
<div class="navbarContainer">
<img width="50px" height="50px" src="https://d35lsekltq0ycz.cloudfront.net/web-arcade/tomato-transparent.png"/>
<h1>Spicy Tomatoes</h1>
</div>
<!-- Form for Typing and Submitting Review -->
<div class="reviewFormContainer">
<input placeholder="movie name" type="text"/>
<input placeholder="review" type="text"/>
<button>Submit</button>
</div>
<!-- Continue to Ratings Page Button -->
<a href="index.html" class="linkBtn">Back</a>
</body>
</html>
Then, we will define our styles in index.css
body {
margin: 0;
display: flex;
flex-direction: column;
row-gap: 20px;
overflow-y: scroll;
overflow-x: hidden;
}
/* Navbar */
.navbarContainer {
display: flex;
flex-direction: row;
align-items: center;
column-gap: 10px;
padding: 10px;
background-color: rgb(250, 50, 9);
}
.navbarContainer > img {
background-color: white;
border-radius: 100%;
padding: 8px;
}
.navbarContainer > h1 {
font-size: 2.4rem;
color: white;
margin: 0;
}
/* Review Movie and Back Links / Buttons */
.linkBtn {
text-align: center;
color: red;
font-size: 20px;
}
.linkBtn:link {
text-decoration: none;
}
.linkBtn:visited {
text-decoration: none;
}
.linkBtn:hover {
text-decoration: underline;
}
.linkBtn:active {
text-decoration: none;
}
/* Review List */
.reviewList {
display: flex;
flex-direction: column;
align-items: center;
row-gap: 30px;
}
/* Review Item */
.reviewContainer {
border-style: dashed;
border: 1px 1px 1px 1px black;
width: min(90%, 600px);
padding: 10px;
border-radius: 10px;
border-width: 4px;
}
.movieName {
font-weight: bold;
text-align: center;
font-size: 1.2rem;
}
.reviewText {
text-align: start;
font-size: 1rem;
}
/* Review Form Container */
.reviewFormContainer {
display: flex;
flex-direction: column;
row-gap: 15px;
justify-content: center;
align-items: center;
width: 100%;
}
.reviewFormContainer > input {
width: min(90%, 400px);
padding: 12px 20px;
margin: 8px 0;
display: inline-block;
border: 1px solid #ccc;
border-radius: 4px;
box-sizing: border-box;
}
.reviewFormContainer > button {
font-size: 1.2rem;
padding: 10px;
color: white;
background-color: rgb(250, 50, 9);
border-radius: 5px;
border: none;
}
.reviewFormContainer > button:hover {
cursor: pointer;
background-color: rgb(219, 44, 9);
}
.reviewFormContainer > button:active {
cursor: pointer;
background-color: rgb(183, 38, 9);
}
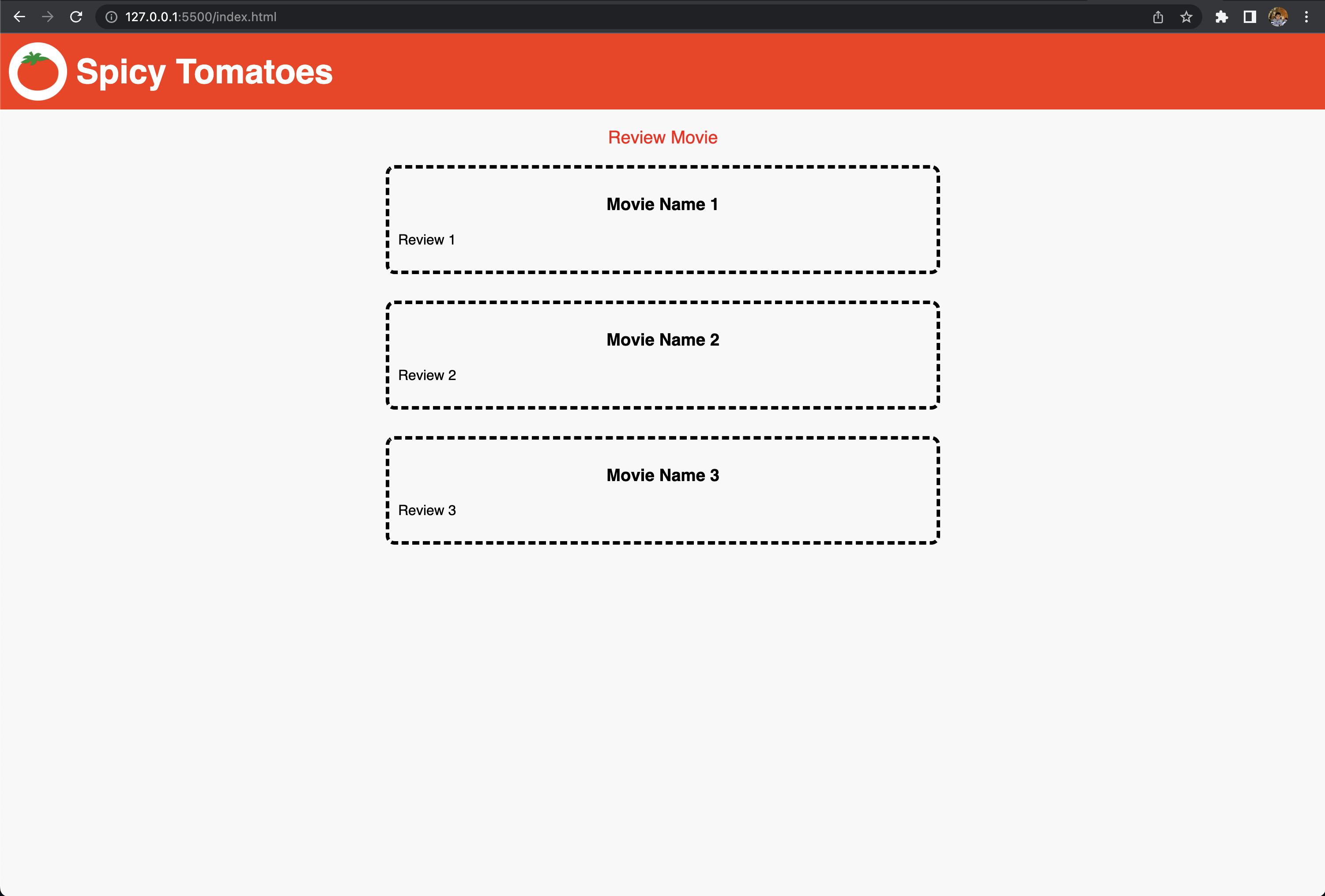
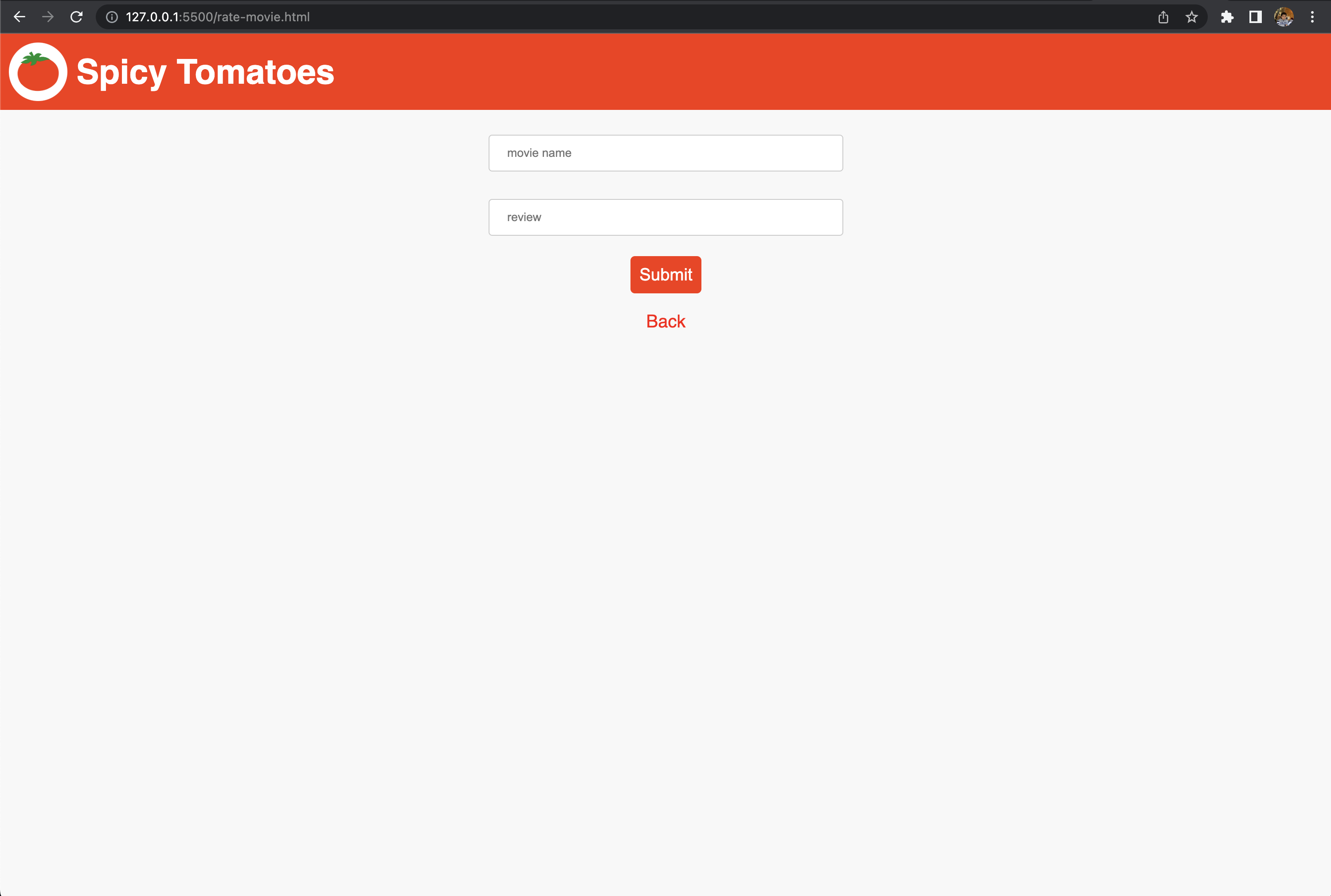