-
Notifications
You must be signed in to change notification settings - Fork 1
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
Showing
45 changed files
with
1,259 additions
and
10 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
4 changes: 2 additions & 2 deletions
4
src/main/racket/g0001_0100/s0002_add_two_numbers/Solution.rkt
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
4 changes: 2 additions & 2 deletions
4
src/main/racket/g0001_0100/s0003_longest_substring_without_repeating_characters/Solution.rkt
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
4 changes: 2 additions & 2 deletions
4
src/main/racket/g0001_0100/s0004_median_of_two_sorted_arrays/Solution.rkt
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
4 changes: 2 additions & 2 deletions
4
src/main/racket/g0001_0100/s0005_longest_palindromic_substring/Solution.rkt
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
53 changes: 53 additions & 0 deletions
53
src/main/racket/g0001_0100/s0064_minimum_path_sum/Solution.rkt
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,53 @@ | ||
; #Medium #Top_100_Liked_Questions #Array #Dynamic_Programming #Matrix | ||
; #Dynamic_Programming_I_Day_16 #Udemy_Dynamic_Programming #Top_Interview_150_Multidimensional_DP | ||
; #Big_O_Time_O(m*n)_Space_O(m*n) #2025_02_04_Time_92_(100.00%)_Space_133.91_(100.00%) | ||
|
||
; dynamic programming helper function | ||
(define (mpsAux grid curpos dpTable ub) | ||
(local | ||
[(define (get grid pos) (list-ref (list-ref grid (car pos)) (cdr pos)))] | ||
(cond | ||
; return start value | ||
[(equal? curpos (cons 0 0)) (car (car grid))] | ||
|
||
; handle out of bounds | ||
[(or (< (car curpos) 0) (< (cdr curpos) 0)) +inf.0] | ||
|
||
; position appeared before | ||
[(hash-ref dpTable curpos #f) (hash-ref dpTable curpos)] | ||
|
||
; inductive case | ||
[else | ||
(let* | ||
( | ||
; go up | ||
[u_val (mpsAux grid (cons (- (car curpos) 1) (cdr curpos)) dpTable ub)] | ||
|
||
; go left | ||
[l_val (mpsAux grid (cons (car curpos) (- (cdr curpos) 1)) dpTable ub)] | ||
|
||
; compute the current least cost | ||
[cur-least-cost (+ (get grid curpos) (min u_val l_val))] | ||
) | ||
(hash-set! dpTable curpos cur-least-cost) | ||
cur-least-cost | ||
) | ||
] | ||
) | ||
) | ||
) | ||
|
||
; the (x . y) position of the grid is the x'th row and y'th column of the grid | ||
(define/contract (min-path-sum grid) | ||
(-> (listof (listof exact-integer?)) exact-integer?) | ||
(local | ||
[(define dpTable (make-hash))] | ||
(let* | ||
( | ||
[curpos (cons (- (length grid) 1) (- (length (car grid)) 1))] | ||
[least-val (mpsAux grid curpos dpTable 200)] | ||
) | ||
(inexact->exact least-val) | ||
) | ||
) | ||
) |
30 changes: 30 additions & 0 deletions
30
src/main/racket/g0001_0100/s0064_minimum_path_sum/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,30 @@ | ||
64\. Minimum Path Sum | ||
|
||
Medium | ||
|
||
Given a `m x n` `grid` filled with non-negative numbers, find a path from top left to bottom right, which minimizes the sum of all numbers along its path. | ||
|
||
**Note:** You can only move either down or right at any point in time. | ||
|
||
**Example 1:** | ||
|
||
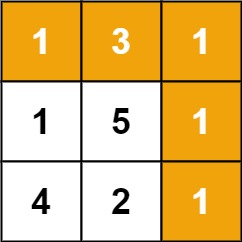 | ||
|
||
**Input:** grid = [[1,3,1],[1,5,1],[4,2,1]] | ||
|
||
**Output:** 7 | ||
|
||
**Explanation:** Because the path 1 → 3 → 1 → 1 → 1 minimizes the sum. | ||
|
||
**Example 2:** | ||
|
||
**Input:** grid = [[1,2,3],[4,5,6]] | ||
|
||
**Output:** 12 | ||
|
||
**Constraints:** | ||
|
||
* `m == grid.length` | ||
* `n == grid[i].length` | ||
* `1 <= m, n <= 200` | ||
* `0 <= grid[i][j] <= 100` |
40 changes: 40 additions & 0 deletions
40
src/main/racket/g0001_0100/s0070_climbing_stairs/Solution.rkt
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,40 @@ | ||
; #Easy #Top_100_Liked_Questions #Top_Interview_Questions #Dynamic_Programming #Math #Memoization | ||
; #Algorithm_I_Day_12_Dynamic_Programming #Dynamic_Programming_I_Day_2 | ||
; #Level_1_Day_10_Dynamic_Programming #Udemy_Dynamic_Programming #Top_Interview_150_1D_DP | ||
; #Big_O_Time_O(n)_Space_O(n) #2025_02_04_Time_0_(100.00%)_Space_101.51_(100.00%) | ||
|
||
(define (clmHelp n hTable) | ||
(cond | ||
; base cases | ||
((= 1 n) 1) | ||
((= 2 n) 2) | ||
((hash-ref hTable n #f) (hash-ref hTable n)) | ||
|
||
; inductive case | ||
(else | ||
(let* | ||
; the local variables | ||
( | ||
(a (clmHelp (- n 1) hTable)) | ||
(b (clmHelp (- n 2) hTable)) | ||
(numPos (+ a b)) | ||
) | ||
|
||
; the body | ||
(hash-set! hTable n numPos) | ||
numPos | ||
) | ||
) | ||
) | ||
) | ||
|
||
(define/contract (climb-stairs n) | ||
(-> exact-integer? exact-integer?) | ||
(local | ||
; local definitions | ||
((define hTable (make-hash))) | ||
|
||
; function body | ||
(clmHelp n hTable) | ||
) | ||
) |
37 changes: 37 additions & 0 deletions
37
src/main/racket/g0001_0100/s0070_climbing_stairs/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,37 @@ | ||
70\. Climbing Stairs | ||
|
||
Easy | ||
|
||
You are climbing a staircase. It takes `n` steps to reach the top. | ||
|
||
Each time you can either climb `1` or `2` steps. In how many distinct ways can you climb to the top? | ||
|
||
**Example 1:** | ||
|
||
**Input:** n = 2 | ||
|
||
**Output:** 2 | ||
|
||
**Explanation:** There are two ways to climb to the top. | ||
|
||
1. 1 step + 1 step | ||
|
||
2. 2 steps | ||
|
||
**Example 2:** | ||
|
||
**Input:** n = 3 | ||
|
||
**Output:** 3 | ||
|
||
**Explanation:** There are three ways to climb to the top. | ||
|
||
1. 1 step + 1 step + 1 step | ||
|
||
2. 1 step + 2 steps | ||
|
||
3. 2 steps + 1 step | ||
|
||
**Constraints:** | ||
|
||
* `1 <= n <= 45` |
25 changes: 25 additions & 0 deletions
25
src/main/racket/g0001_0100/s0072_edit_distance/Solution.rkt
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,25 @@ | ||
; #Medium #Top_100_Liked_Questions #String #Dynamic_Programming | ||
; #Algorithm_II_Day_18_Dynamic_Programming #Dynamic_Programming_I_Day_19 | ||
; #Udemy_Dynamic_Programming #Top_Interview_150_Multidimensional_DP #Big_O_Time_O(n^2)_Space_O(n2) | ||
; #2025_02_04_Time_4_(100.00%)_Space_102.31_(100.00%) | ||
|
||
(define/contract (min-distance word1 word2) | ||
(-> string? string? exact-integer?) | ||
(let* ((n1 (string-length word1)) | ||
(n2 (string-length word2))) | ||
(if (> n2 n1) | ||
(min-distance word2 word1) | ||
(let ((dp (make-vector (+ n2 1) 0))) | ||
(for ([j (in-range (+ n2 1))]) | ||
(vector-set! dp j j)) | ||
(for ([i (in-range 1 (+ n1 1))]) | ||
(let ((pre (vector-ref dp 0))) | ||
(vector-set! dp 0 i) | ||
(for ([j (in-range 1 (+ n2 1))]) | ||
(let* ((tmp (vector-ref dp j)) | ||
(cost (if (char=? (string-ref word1 (- i 1)) (string-ref word2 (- j 1))) | ||
pre | ||
(+ 1 (min pre (vector-ref dp j) (vector-ref dp (- j 1))))))) | ||
(vector-set! dp j cost) | ||
(set! pre tmp))))) | ||
(vector-ref dp n2))))) |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,48 @@ | ||
72\. Edit Distance | ||
|
||
Hard | ||
|
||
Given two strings `word1` and `word2`, return _the minimum number of operations required to convert `word1` to `word2`_. | ||
|
||
You have the following three operations permitted on a word: | ||
|
||
* Insert a character | ||
* Delete a character | ||
* Replace a character | ||
|
||
**Example 1:** | ||
|
||
**Input:** word1 = "horse", word2 = "ros" | ||
|
||
**Output:** 3 | ||
|
||
**Explanation:** | ||
|
||
horse -> rorse (replace 'h' with 'r') | ||
|
||
rorse -> rose (remove 'r') | ||
|
||
rose -> ros (remove 'e') | ||
|
||
**Example 2:** | ||
|
||
**Input:** word1 = "intention", word2 = "execution" | ||
|
||
**Output:** 5 | ||
|
||
**Explanation:** | ||
|
||
intention -> inention (remove 't') | ||
|
||
inention -> enention (replace 'i' with 'e') | ||
|
||
enention -> exention (replace 'n' with 'x') | ||
|
||
exention -> exection (replace 'n' with 'c') | ||
|
||
exection -> execution (insert 'u') | ||
|
||
**Constraints:** | ||
|
||
* `0 <= word1.length, word2.length <= 500` | ||
* `word1` and `word2` consist of lowercase English letters. |
8 changes: 8 additions & 0 deletions
8
src/main/racket/g0001_0100/s0074_search_a_2d_matrix/Solution.rkt
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,8 @@ | ||
; #Medium #Top_100_Liked_Questions #Array #Binary_Search #Matrix #Data_Structure_I_Day_5_Array | ||
; #Algorithm_II_Day_1_Binary_Search #Binary_Search_I_Day_8 #Level_2_Day_8_Binary_Search | ||
; #Udemy_2D_Arrays/Matrix #Top_Interview_150_Binary_Search #Big_O_Time_O(endRow+endCol)_Space_O(1) | ||
; #2025_02_04_Time_0_(100.00%)_Space_101.20_(100.00%) | ||
|
||
(define/contract (search-matrix matrix target) | ||
(-> (listof (listof exact-integer?)) exact-integer? boolean?) | ||
(not (equal? #f (member target (apply append matrix))))) |
31 changes: 31 additions & 0 deletions
31
src/main/racket/g0001_0100/s0074_search_a_2d_matrix/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,31 @@ | ||
74\. Search a 2D Matrix | ||
|
||
Medium | ||
|
||
Write an efficient algorithm that searches for a value `target` in an `m x n` integer matrix `matrix`. This matrix has the following properties: | ||
|
||
* Integers in each row are sorted from left to right. | ||
* The first integer of each row is greater than the last integer of the previous row. | ||
|
||
**Example 1:** | ||
|
||
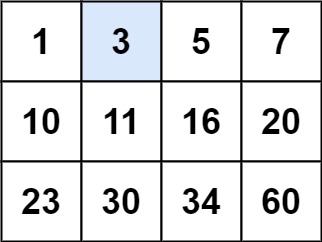 | ||
|
||
**Input:** matrix = [[1,3,5,7],[10,11,16,20],[23,30,34,60]], target = 3 | ||
|
||
**Output:** true | ||
|
||
**Example 2:** | ||
|
||
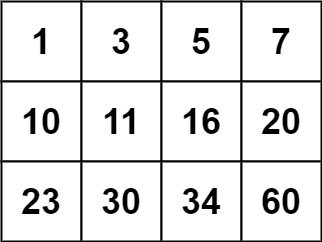 | ||
|
||
**Input:** matrix = [[1,3,5,7],[10,11,16,20],[23,30,34,60]], target = 13 | ||
|
||
**Output:** false | ||
|
||
**Constraints:** | ||
|
||
* `m == matrix.length` | ||
* `n == matrix[i].length` | ||
* `1 <= m, n <= 100` | ||
* <code>-10<sup>4</sup> <= matrix[i][j], target <= 10<sup>4</sup></code> |
66 changes: 66 additions & 0 deletions
66
src/main/racket/g0001_0100/s0076_minimum_window_substring/Solution.rkt
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,66 @@ | ||
; #Hard #Top_100_Liked_Questions #Top_Interview_Questions #String #Hash_Table #Sliding_Window | ||
; #Level_2_Day_14_Sliding_Window/Two_Pointer #Top_Interview_150_Sliding_Window | ||
; #Big_O_Time_O(s.length())_Space_O(1) #2025_02_04_Time_151_(100.00%)_Space_130.75_(100.00%) | ||
|
||
(define (zip lst1 lst2) | ||
(map cons lst1 lst2)) | ||
|
||
(define (make-zero-hash lst) | ||
(make-immutable-hash (zip lst (make-list (length lst) 0)))) | ||
|
||
(define (hash-inc k ht) | ||
(hash-update ht k add1 0)) | ||
|
||
(define (safe-hash-inc k ht) | ||
(if (hash-ref ht k #f) (hash-update ht k add1) ht)) | ||
|
||
(define (safe-hash-dec k ht) | ||
(if (hash-ref ht k #f) (hash-update ht k sub1) ht)) | ||
|
||
(define (frequencies lst) | ||
(foldl hash-inc (hash) lst)) | ||
|
||
(define (pair-min l1 r1 l2 r2) | ||
(if (< (- r1 l1) (- r2 l2)) (values l1 r1) (values l2 r2))) | ||
|
||
(define (sref s i) (string-ref s (min (sub1 (string-length s)) i))) | ||
|
||
(define (min-window s t) | ||
(define t-lst (string->list t)) | ||
|
||
(define target (frequencies t-lst)) | ||
|
||
(define (delta-dec delta seen c) | ||
(cond | ||
[(and (hash-ref target c #f) | ||
(< (hash-ref seen c) (hash-ref target c))) | ||
(sub1 delta)] | ||
[else delta])) | ||
|
||
(define (delta-inc delta seen c) | ||
(cond | ||
[(and (hash-ref target c #f) | ||
(<= (hash-ref seen c) (hash-ref target c))) | ||
(add1 delta)] | ||
[else delta])) | ||
|
||
(let loop ([l 0] [r 0] [seen (make-zero-hash t-lst)] | ||
[delta (length t-lst)] [sl 0] [sr 0]) | ||
(define-values (left-char right-char) (values (sref s l) (sref s r))) | ||
(define-values (l* r*) (pair-min l r sl sr)) | ||
(cond | ||
[(and (= 0 sr) | ||
(= delta 0)) | ||
(loop (add1 l) r (safe-hash-dec left-char seen) | ||
(delta-inc delta seen left-char) l r)] | ||
[(and (= r (string-length s)) (= delta 0)) | ||
(loop (add1 l) r (safe-hash-dec left-char seen) | ||
(delta-inc delta seen left-char) l* r*)] | ||
[(= r (string-length s)) | ||
(substring s sl sr)] | ||
[(= delta 0) | ||
(loop (add1 l) r (safe-hash-dec left-char seen) | ||
(delta-inc delta seen left-char) l* r*)] | ||
[else | ||
(loop l (add1 r) (safe-hash-inc right-char seen) | ||
(delta-dec delta seen right-char) sl sr)]))) |
Oops, something went wrong.