-
Notifications
You must be signed in to change notification settings - Fork 24
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
Showing
27 changed files
with
1,309 additions
and
0 deletions.
There are no files selected for viewing
61 changes: 61 additions & 0 deletions
61
src/main/kotlin/g3401_3500/s3451_find_invalid_ip_addresses/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,61 @@ | ||
3451\. Find Invalid IP Addresses | ||
|
||
Hard | ||
|
||
Table: `logs` | ||
|
||
+-------------+---------+ | ||
| Column Name | Type | | ||
+-------------+---------+ | ||
| log_id | int | | ||
| ip | varchar | | ||
| status_code | int | | ||
+-------------+---------+ | ||
log_id is the unique key for this table. | ||
Each row contains server access log information including IP address and HTTP status code. | ||
|
||
Write a solution to find **invalid IP addresses**. An IPv4 address is invalid if it meets any of these conditions: | ||
|
||
* Contains numbers **greater than** `255` in any octet | ||
* Has **leading zeros** in any octet (like `01.02.03.04`) | ||
* Has **less or more** than `4` octets | ||
|
||
Return _the result table_ _ordered by_ `invalid_count`, `ip` _in **descending** order respectively_. | ||
|
||
The result format is in the following example. | ||
|
||
**Example:** | ||
|
||
**Input:** | ||
|
||
logs table: | ||
|
||
+--------+---------------+-------------+ | ||
| log_id | ip | status_code | | ||
+--------+---------------+-------------+ | ||
| 1 | 192.168.1.1 | 200 | | ||
| 2 | 256.1.2.3 | 404 | | ||
| 3 | 192.168.001.1 | 200 | | ||
| 4 | 192.168.1.1 | 200 | | ||
| 5 | 192.168.1 | 500 | | ||
| 6 | 256.1.2.3 | 404 | | ||
| 7 | 192.168.001.1 | 200 | | ||
+--------+---------------+-------------+ | ||
|
||
**Output:** | ||
|
||
+---------------+--------------+ | ||
| ip | invalid_count| | ||
+---------------+--------------+ | ||
| 256.1.2.3 | 2 | | ||
| 192.168.001.1 | 2 | | ||
| 192.168.1 | 1 | | ||
+---------------+--------------+ | ||
|
||
**Explanation:** | ||
|
||
* 256.1.2.3 is invalid because 256 > 255 | ||
* 192.168.001.1 is invalid because of leading zeros | ||
* 192.168.1 is invalid because it has only 3 octets | ||
|
||
The output table is ordered by invalid\_count, ip in descending order respectively. |
15 changes: 15 additions & 0 deletions
15
src/main/kotlin/g3401_3500/s3451_find_invalid_ip_addresses/script.sql
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,15 @@ | ||
# Write your MySQL query statement below | ||
# #Hard #Database #2025_02_18_Time_309_ms_(90.61%)_Space_0.0_MB_(100.00%) | ||
WITH cte_invalid_ip AS ( | ||
SELECT log_id, ip | ||
FROM logs | ||
WHERE NOT regexp_like(ip, '^(?:[1-9]|[1-9][0-9]|1[0-9][0-9]|2[0-4][0-9]|25[0-5])(?:[.](?:[1-9]|[1-9][0-9]|1[0-9][0-9]|2[0-4][0-9]|25[0-5])){3}$') | ||
), | ||
cte_invalid_ip_count AS ( | ||
SELECT ip, count(log_id) as invalid_count | ||
FROM cte_invalid_ip | ||
GROUP BY ip | ||
) | ||
SELECT ip, invalid_count | ||
FROM cte_invalid_ip_count | ||
ORDER BY invalid_count DESC, ip DESC; |
23 changes: 23 additions & 0 deletions
23
src/main/kotlin/g3401_3500/s3452_sum_of_good_numbers/Solution.kt
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,23 @@ | ||
package g3401_3500.s3452_sum_of_good_numbers | ||
|
||
// #Easy #Array #2025_02_18_Time_1_ms_(100.00%)_Space_38.08_MB_(84.85%) | ||
|
||
class Solution { | ||
fun sumOfGoodNumbers(nums: IntArray, k: Int): Int { | ||
var totalSum = 0 | ||
val n = nums.size | ||
for (i in 0..<n) { | ||
var isGood = true | ||
if (i - k >= 0 && nums[i] <= nums[i - k]) { | ||
isGood = false | ||
} | ||
if (i + k < n && nums[i] <= nums[i + k]) { | ||
isGood = false | ||
} | ||
if (isGood) { | ||
totalSum += nums[i] | ||
} | ||
} | ||
return totalSum | ||
} | ||
} |
33 changes: 33 additions & 0 deletions
33
src/main/kotlin/g3401_3500/s3452_sum_of_good_numbers/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,33 @@ | ||
3452\. Sum of Good Numbers | ||
|
||
Easy | ||
|
||
Given an array of integers `nums` and an integer `k`, an element `nums[i]` is considered **good** if it is **strictly** greater than the elements at indices `i - k` and `i + k` (if those indices exist). If neither of these indices _exists_, `nums[i]` is still considered **good**. | ||
|
||
Return the **sum** of all the **good** elements in the array. | ||
|
||
**Example 1:** | ||
|
||
**Input:** nums = [1,3,2,1,5,4], k = 2 | ||
|
||
**Output:** 12 | ||
|
||
**Explanation:** | ||
|
||
The good numbers are `nums[1] = 3`, `nums[4] = 5`, and `nums[5] = 4` because they are strictly greater than the numbers at indices `i - k` and `i + k`. | ||
|
||
**Example 2:** | ||
|
||
**Input:** nums = [2,1], k = 1 | ||
|
||
**Output:** 2 | ||
|
||
**Explanation:** | ||
|
||
The only good number is `nums[0] = 2` because it is strictly greater than `nums[1]`. | ||
|
||
**Constraints:** | ||
|
||
* `2 <= nums.length <= 100` | ||
* `1 <= nums[i] <= 1000` | ||
* `1 <= k <= floor(nums.length / 2)` |
58 changes: 58 additions & 0 deletions
58
src/main/kotlin/g3401_3500/s3453_separate_squares_i/Solution.kt
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,58 @@ | ||
package g3401_3500.s3453_separate_squares_i | ||
|
||
// #Medium #Array #Binary_Search #2025_02_18_Time_57_ms_(100.00%)_Space_102.84_MB_(84.85%) | ||
|
||
class Solution { | ||
fun separateSquares(squares: Array<IntArray>): Double { | ||
val n = squares.size | ||
val arr = Array(n) { LongArray(3) } | ||
var total = 0.0 | ||
var left = Long.MAX_VALUE | ||
var right = Long.MIN_VALUE | ||
for (i in 0..n - 1) { | ||
val y = squares[i][1].toLong() | ||
val z = squares[i][2].toLong() | ||
arr[i][0] = y | ||
arr[i][1] = y + z | ||
arr[i][2] = z | ||
total += (z * z).toDouble() | ||
left = minOf(left, arr[i][0]) | ||
right = maxOf(right, arr[i][1]) | ||
} | ||
while (left < right) { | ||
val mid = (left + right) / 2 | ||
var low = 0.0 | ||
for (a in arr) { | ||
if (a[0] >= mid) { | ||
continue | ||
} else if (a[1] <= mid) { | ||
low += a[2] * a[2] | ||
} else { | ||
low += a[2] * (mid - a[0]) | ||
} | ||
} | ||
if (low + low + 0.00001 >= total) { | ||
right = mid | ||
} else { | ||
left = mid + 1 | ||
} | ||
} | ||
left = right - 1 | ||
var a1 = 0.0 | ||
var a2 = 0.0 | ||
for (a in arr) { | ||
val x = a[0] | ||
val y = a[1] | ||
val z = a[2] | ||
if (left > x) { | ||
a1 += (minOf(y, left) - x) * z.toDouble() | ||
} | ||
if (right < y) { | ||
a2 += (y - maxOf(x, right)) * z.toDouble() | ||
} | ||
} | ||
val goal = (total - a1 - a1) / 2 | ||
val len = total - a1 - a2 | ||
return right - 1 + (goal / len) | ||
} | ||
} |
48 changes: 48 additions & 0 deletions
48
src/main/kotlin/g3401_3500/s3453_separate_squares_i/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,48 @@ | ||
3453\. Separate Squares I | ||
|
||
Medium | ||
|
||
You are given a 2D integer array `squares`. Each <code>squares[i] = [x<sub>i</sub>, y<sub>i</sub>, l<sub>i</sub>]</code> represents the coordinates of the bottom-left point and the side length of a square parallel to the x-axis. | ||
|
||
Find the **minimum** y-coordinate value of a horizontal line such that the total area of the squares above the line _equals_ the total area of the squares below the line. | ||
|
||
Answers within <code>10<sup>-5</sup></code> of the actual answer will be accepted. | ||
|
||
**Note**: Squares **may** overlap. Overlapping areas should be counted **multiple times**. | ||
|
||
**Example 1:** | ||
|
||
**Input:** squares = [[0,0,1],[2,2,1]] | ||
|
||
**Output:** 1.00000 | ||
|
||
**Explanation:** | ||
|
||
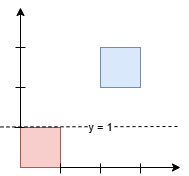 | ||
|
||
Any horizontal line between `y = 1` and `y = 2` will have 1 square unit above it and 1 square unit below it. The lowest option is 1. | ||
|
||
**Example 2:** | ||
|
||
**Input:** squares = [[0,0,2],[1,1,1]] | ||
|
||
**Output:** 1.16667 | ||
|
||
**Explanation:** | ||
|
||
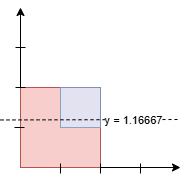 | ||
|
||
The areas are: | ||
|
||
* Below the line: `7/6 * 2 (Red) + 1/6 (Blue) = 15/6 = 2.5`. | ||
* Above the line: `5/6 * 2 (Red) + 5/6 (Blue) = 15/6 = 2.5`. | ||
|
||
Since the areas above and below the line are equal, the output is `7/6 = 1.16667`. | ||
|
||
**Constraints:** | ||
|
||
* <code>1 <= squares.length <= 5 * 10<sup>4</sup></code> | ||
* <code>squares[i] = [x<sub>i</sub>, y<sub>i</sub>, l<sub>i</sub>]</code> | ||
* `squares[i].length == 3` | ||
* <code>0 <= x<sub>i</sub>, y<sub>i</sub> <= 10<sup>9</sup></code> | ||
* <code>1 <= l<sub>i</sub> <= 10<sup>9</sup></code> |
Oops, something went wrong.