forked from javadev/LeetCode-in-Kotlin
-
Notifications
You must be signed in to change notification settings - Fork 1
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
Showing
39 changed files
with
1,519 additions
and
0 deletions.
There are no files selected for viewing
10 changes: 10 additions & 0 deletions
10
src/main/kotlin/g2801_2900/s2806_account_balance_after_rounded_purchase/Solution.kt
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,10 @@ | ||
package g2801_2900.s2806_account_balance_after_rounded_purchase | ||
|
||
// #Easy #Math #2023_12_06_Time_108_ms_(100.00%)_Space_32.7_MB_(100.00%) | ||
|
||
class Solution { | ||
fun accountBalanceAfterPurchase(purchaseAmount: Int): Int { | ||
val x = ((purchaseAmount + 5) / 10.0).toInt() * 10 | ||
return 100 - x | ||
} | ||
} |
35 changes: 35 additions & 0 deletions
35
src/main/kotlin/g2801_2900/s2806_account_balance_after_rounded_purchase/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,35 @@ | ||
2806\. Account Balance After Rounded Purchase | ||
|
||
Easy | ||
|
||
Initially, you have a bank account balance of `100` dollars. | ||
|
||
You are given an integer `purchaseAmount` representing the amount you will spend on a purchase in dollars. | ||
|
||
At the store where you will make the purchase, the purchase amount is rounded to the **nearest multiple** of `10`. In other words, you pay a **non-negative** amount, `roundedAmount`, such that `roundedAmount` is a multiple of `10` and `abs(roundedAmount - purchaseAmount)` is **minimized**. | ||
|
||
If there is more than one nearest multiple of `10`, the **largest multiple** is chosen. | ||
|
||
Return _an integer denoting your account balance after making a purchase worth_ `purchaseAmount` _dollars from the store._ | ||
|
||
**Note:** `0` is considered to be a multiple of `10` in this problem. | ||
|
||
**Example 1:** | ||
|
||
**Input:** purchaseAmount = 9 | ||
|
||
**Output:** 90 | ||
|
||
**Explanation:** In this example, the nearest multiple of 10 to 9 is 10. Hence, your account balance becomes 100 - 10 = 90. | ||
|
||
**Example 2:** | ||
|
||
**Input:** purchaseAmount = 15 | ||
|
||
**Output:** 80 | ||
|
||
**Explanation:** In this example, there are two nearest multiples of 10 to 15: 10 and 20. So, the larger multiple, 20, is chosen. Hence, your account balance becomes 100 - 20 = 80. | ||
|
||
**Constraints:** | ||
|
||
* `0 <= purchaseAmount <= 100` |
36 changes: 36 additions & 0 deletions
36
src/main/kotlin/g2801_2900/s2807_insert_greatest_common_divisors_in_linked_list/Solution.kt
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,36 @@ | ||
package g2801_2900.s2807_insert_greatest_common_divisors_in_linked_list | ||
|
||
// #Medium #Array #Math #Linked_List #2023_12_06_Time_225_ms_(67.65%)_Space_37.6_MB_(97.06%) | ||
|
||
import com_github_leetcode.ListNode | ||
|
||
/* | ||
* Example: | ||
* var li = ListNode(5) | ||
* var v = li.`val` | ||
* Definition for singly-linked list. | ||
* class ListNode(var `val`: Int) { | ||
* var next: ListNode? = null | ||
* } | ||
*/ | ||
class Solution { | ||
fun insertGreatestCommonDivisors(head: ListNode?): ListNode? { | ||
var prevNode: ListNode? = null | ||
var currNode = head | ||
while (currNode != null) { | ||
if (prevNode != null) { | ||
val gcd = greatestCommonDivisor(prevNode.`val`, currNode.`val`) | ||
prevNode.next = ListNode(gcd, currNode) | ||
} | ||
prevNode = currNode | ||
currNode = currNode.next | ||
} | ||
return head | ||
} | ||
|
||
private fun greatestCommonDivisor(val1: Int, val2: Int): Int { | ||
return if (val2 == 0) { | ||
val1 | ||
} else greatestCommonDivisor(val2, val1 % val2) | ||
} | ||
} |
42 changes: 42 additions & 0 deletions
42
...otlin/g2801_2900/s2807_insert_greatest_common_divisors_in_linked_list/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,42 @@ | ||
2807\. Insert Greatest Common Divisors in Linked List | ||
|
||
Medium | ||
|
||
Given the head of a linked list `head`, in which each node contains an integer value. | ||
|
||
Between every pair of adjacent nodes, insert a new node with a value equal to the **greatest common divisor** of them. | ||
|
||
Return _the linked list after insertion_. | ||
|
||
The **greatest common divisor** of two numbers is the largest positive integer that evenly divides both numbers. | ||
|
||
**Example 1:** | ||
|
||
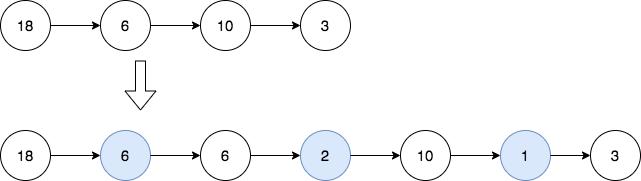 | ||
|
||
**Input:** head = [18,6,10,3] | ||
|
||
**Output:** [18,6,6,2,10,1,3] | ||
|
||
**Explanation:** The 1<sup>st</sup> diagram denotes the initial linked list and the 2<sup>nd</sup> diagram denotes the linked list after inserting the new nodes (nodes in blue are the inserted nodes). | ||
|
||
- We insert the greatest common divisor of 18 and 6 = 6 between the 1<sup>st</sup> and the 2<sup>nd</sup> nodes. | ||
- We insert the greatest common divisor of 6 and 10 = 2 between the 2<sup>nd</sup> and the 3<sup>rd</sup> nodes. | ||
- We insert the greatest common divisor of 10 and 3 = 1 between the 3<sup>rd</sup> and the 4<sup>th</sup> nodes. | ||
|
||
There are no more adjacent nodes, so we return the linked list. | ||
|
||
**Example 2:** | ||
|
||
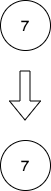 | ||
|
||
**Input:** head = [7] | ||
|
||
**Output:** [7] | ||
|
||
**Explanation:** The 1<sup>st</sup> diagram denotes the initial linked list and the 2<sup>nd</sup> diagram denotes the linked list after inserting the new nodes. There are no pairs of adjacent nodes, so we return the initial linked list. | ||
|
||
**Constraints:** | ||
|
||
* The number of nodes in the list is in the range `[1, 5000]`. | ||
* `1 <= Node.val <= 1000` |
28 changes: 28 additions & 0 deletions
28
src/main/kotlin/g2801_2900/s2808_minimum_seconds_to_equalize_a_circular_array/Solution.kt
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,28 @@ | ||
package g2801_2900.s2808_minimum_seconds_to_equalize_a_circular_array | ||
|
||
// #Medium #Array #Hash_Table #Greedy #2023_12_06_Time_847_ms_(50.00%)_Space_78.3_MB_(50.00%) | ||
|
||
import kotlin.math.max | ||
import kotlin.math.min | ||
|
||
class Solution { | ||
fun minimumSeconds(nums: List<Int>): Int { | ||
val n = nums.size | ||
var min = n / 2 | ||
val hm = HashMap<Int, MutableList<Int>>() | ||
for (i in 0 until n) { | ||
val v = nums[i] | ||
hm.computeIfAbsent(v) { k: Int? -> ArrayList() }.add(i) | ||
} | ||
for (list in hm.values) { | ||
if (list.size > 1) { | ||
var curr = (list[0] + n - list[list.size - 1]) / 2 | ||
for (i in 1 until list.size) { | ||
curr = max(curr.toDouble(), ((list[i] - list[i - 1]) / 2).toDouble()).toInt() | ||
} | ||
min = min(min.toDouble(), curr.toDouble()).toInt() | ||
} | ||
} | ||
return min | ||
} | ||
} |
47 changes: 47 additions & 0 deletions
47
.../kotlin/g2801_2900/s2808_minimum_seconds_to_equalize_a_circular_array/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,47 @@ | ||
2808\. Minimum Seconds to Equalize a Circular Array | ||
|
||
Medium | ||
|
||
You are given a **0-indexed** array `nums` containing `n` integers. | ||
|
||
At each second, you perform the following operation on the array: | ||
|
||
* For every index `i` in the range `[0, n - 1]`, replace `nums[i]` with either `nums[i]`, `nums[(i - 1 + n) % n]`, or `nums[(i + 1) % n]`. | ||
|
||
**Note** that all the elements get replaced simultaneously. | ||
|
||
Return _the **minimum** number of seconds needed to make all elements in the array_ `nums` _equal_. | ||
|
||
**Example 1:** | ||
|
||
**Input:** nums = [1,2,1,2] | ||
|
||
**Output:** 1 | ||
|
||
**Explanation:** We can equalize the array in 1 second in the following way: | ||
- At 1<sup>st</sup> second, replace values at each index with [nums[3],nums[1],nums[3],nums[3]]. After replacement, nums = [2,2,2,2]. It can be proven that 1 second is the minimum amount of seconds needed for equalizing the array. | ||
|
||
**Example 2:** | ||
|
||
**Input:** nums = [2,1,3,3,2] | ||
|
||
**Output:** 2 | ||
|
||
**Explanation:** We can equalize the array in 2 seconds in the following way: | ||
- At 1<sup>st</sup> second, replace values at each index with [nums[0],nums[2],nums[2],nums[2],nums[3]]. After replacement, nums = [2,3,3,3,3]. | ||
- At 2<sup>nd</sup> second, replace values at each index with [nums[1],nums[1],nums[2],nums[3],nums[4]]. After replacement, nums = [3,3,3,3,3]. | ||
|
||
It can be proven that 2 seconds is the minimum amount of seconds needed for equalizing the array. | ||
|
||
**Example 3:** | ||
|
||
**Input:** nums = [5,5,5,5] | ||
|
||
**Output:** 0 | ||
|
||
**Explanation:** We don't need to perform any operations as all elements in the initial array are the same. | ||
|
||
**Constraints:** | ||
|
||
* <code>1 <= n == nums.length <= 10<sup>5</sup></code> | ||
* <code>1 <= nums[i] <= 10<sup>9</sup></code> |
39 changes: 39 additions & 0 deletions
39
src/main/kotlin/g2801_2900/s2809_minimum_time_to_make_array_sum_at_most_x/Solution.kt
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,39 @@ | ||
package g2801_2900.s2809_minimum_time_to_make_array_sum_at_most_x | ||
|
||
// #Hard #Array #Dynamic_Programming #Sorting | ||
// #2023_12_06_Time_325_ms_(100.00%)_Space_42.6_MB_(100.00%) | ||
|
||
import kotlin.math.max | ||
|
||
class Solution { | ||
fun minimumTime(nums1: List<Int?>, nums2: List<Int?>, x: Int): Int { | ||
val n = nums1.size | ||
val nums = Array(n) { IntArray(2) } | ||
for (i in 0 until n) { | ||
nums[i] = intArrayOf(nums1[i]!!, nums2[i]!!) | ||
} | ||
nums.sortWith { a: IntArray, b: IntArray -> a[1] - b[1] } | ||
val dp = IntArray(n + 1) | ||
var sum1: Long = 0 | ||
var sum2: Long = 0 | ||
for (i in 0 until n) { | ||
sum1 += nums[i][0].toLong() | ||
sum2 += nums[i][1].toLong() | ||
} | ||
if (sum1 <= x) { | ||
return 0 | ||
} | ||
for (j in 0 until n) { | ||
for (i in j + 1 downTo 1) { | ||
dp[i] = max(dp[i].toDouble(), (nums[j][0] + nums[j][1] * i + dp[i - 1]).toDouble()) | ||
.toInt() | ||
} | ||
} | ||
for (i in 1..n) { | ||
if (sum1 + sum2 * i - dp[i] <= x) { | ||
return i | ||
} | ||
} | ||
return -1 | ||
} | ||
} |
40 changes: 40 additions & 0 deletions
40
...main/kotlin/g2801_2900/s2809_minimum_time_to_make_array_sum_at_most_x/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,40 @@ | ||
2809\. Minimum Time to Make Array Sum At Most x | ||
|
||
Hard | ||
|
||
You are given two **0-indexed** integer arrays `nums1` and `nums2` of equal length. Every second, for all indices `0 <= i < nums1.length`, value of `nums1[i]` is incremented by `nums2[i]`. **After** this is done, you can do the following operation: | ||
|
||
* Choose an index `0 <= i < nums1.length` and make `nums1[i] = 0`. | ||
|
||
You are also given an integer `x`. | ||
|
||
Return _the **minimum** time in which you can make the sum of all elements of_ `nums1` _to be **less than or equal** to_ `x`, _or_ `-1` _if this is not possible._ | ||
|
||
**Example 1:** | ||
|
||
**Input:** nums1 = [1,2,3], nums2 = [1,2,3], x = 4 | ||
|
||
**Output:** 3 | ||
|
||
**Explanation:** | ||
|
||
For the 1st second, we apply the operation on i = 0. Therefore nums1 = [0,2+2,3+3] = [0,4,6]. | ||
For the 2nd second, we apply the operation on i = 1. Therefore nums1 = [0+1,0,6+3] = [1,0,9]. | ||
For the 3rd second, we apply the operation on i = 2. Therefore nums1 = [1+1,0+2,0] = [2,2,0]. | ||
Now sum of nums1 = 4. It can be shown that these operations are optimal, so we return 3. | ||
|
||
**Example 2:** | ||
|
||
**Input:** nums1 = [1,2,3], nums2 = [3,3,3], x = 4 | ||
|
||
**Output:** -1 | ||
|
||
**Explanation:** It can be shown that the sum of nums1 will always be greater than x, no matter which operations are performed. | ||
|
||
**Constraints:** | ||
|
||
* <code>1 <= nums1.length <= 10<sup>3</sup></code> | ||
* <code>1 <= nums1[i] <= 10<sup>3</sup></code> | ||
* <code>0 <= nums2[i] <= 10<sup>3</sup></code> | ||
* `nums1.length == nums2.length` | ||
* <code>0 <= x <= 10<sup>6</sup></code> |
17 changes: 17 additions & 0 deletions
17
src/main/kotlin/g2801_2900/s2810_faulty_keyboard/Solution.kt
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,17 @@ | ||
package g2801_2900.s2810_faulty_keyboard | ||
|
||
// #Easy #String #Simulation #2023_12_06_Time_196_ms_(91.67%)_Space_36.9_MB_(91.67%) | ||
|
||
class Solution { | ||
fun finalString(s: String): String { | ||
val stringBuilder = StringBuilder() | ||
for (ch in s.toCharArray()) { | ||
if (ch == 'i') { | ||
stringBuilder.reverse() | ||
continue | ||
} | ||
stringBuilder.append(ch) | ||
} | ||
return stringBuilder.toString() | ||
} | ||
} |
59 changes: 59 additions & 0 deletions
59
src/main/kotlin/g2801_2900/s2810_faulty_keyboard/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,59 @@ | ||
2810\. Faulty Keyboard | ||
|
||
Easy | ||
|
||
Your laptop keyboard is faulty, and whenever you type a character `'i'` on it, it reverses the string that you have written. Typing other characters works as expected. | ||
|
||
You are given a **0-indexed** string `s`, and you type each character of `s` using your faulty keyboard. | ||
|
||
Return _the final string that will be present on your laptop screen._ | ||
|
||
**Example 1:** | ||
|
||
**Input:** s = "string" | ||
|
||
**Output:** "rtsng" | ||
|
||
**Explanation:** | ||
|
||
After typing first character, the text on the screen is "s". | ||
|
||
After the second character, the text is "st". | ||
|
||
After the third character, the text is "str". | ||
|
||
Since the fourth character is an 'i', the text gets reversed and becomes "rts". | ||
|
||
After the fifth character, the text is "rtsn". | ||
|
||
After the sixth character, the text is "rtsng". | ||
|
||
Therefore, we return "rtsng". | ||
|
||
**Example 2:** | ||
|
||
**Input:** s = "poiinter" | ||
|
||
**Output:** "ponter" | ||
|
||
**Explanation:** | ||
|
||
After the first character, the text on the screen is "p". | ||
|
||
After the second character, the text is "po". | ||
|
||
Since the third character you type is an 'i', the text gets reversed and becomes "op". | ||
|
||
Since the fourth character you type is an 'i', the text gets reversed and becomes "po". | ||
|
||
After the fifth character, the text is "pon". After the sixth character, the text is "pont". | ||
|
||
After the seventh character, the text is "ponte". After the eighth character, the text is "ponter". | ||
|
||
Therefore, we return "ponter". | ||
|
||
**Constraints:** | ||
|
||
* `1 <= s.length <= 100` | ||
* `s` consists of lowercase English letters. | ||
* `s[0] != 'i'` |
19 changes: 19 additions & 0 deletions
19
src/main/kotlin/g2801_2900/s2811_check_if_it_is_possible_to_split_array/Solution.kt
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,19 @@ | ||
package g2801_2900.s2811_check_if_it_is_possible_to_split_array | ||
|
||
// #Medium #Array #Dynamic_Programming #Greedy | ||
// #2023_12_06_Time_180_ms_(100.00%)_Space_36.1_MB_(100.00%) | ||
|
||
class Solution { | ||
fun canSplitArray(nums: List<Int>, m: Int): Boolean { | ||
if (nums.size < 3 && !nums.isEmpty()) { | ||
return true | ||
} | ||
var ans = false | ||
for (i in 0 until nums.size - 1) { | ||
if (nums[i] + nums[i + 1] >= m) { | ||
ans = true | ||
} | ||
} | ||
return ans | ||
} | ||
} |
Oops, something went wrong.