forked from javadev/LeetCode-in-Kotlin
-
Notifications
You must be signed in to change notification settings - Fork 1
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
Showing
9 changed files
with
354 additions
and
0 deletions.
There are no files selected for viewing
18 changes: 18 additions & 0 deletions
18
src/main/kotlin/g2901_3000/s2942_find_words_containing_character/Solution.kt
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,18 @@ | ||
package g2901_3000.s2942_find_words_containing_character | ||
|
||
// #Easy #Array #String #2024_01_07_Time_216_ms_(98.97%)_Space_37.6_MB_(98.46%) | ||
|
||
class Solution { | ||
fun findWordsContaining(words: Array<String>, x: Char): List<Int> { | ||
val ans: MutableList<Int> = ArrayList() | ||
for (i in words.indices) { | ||
for (j in 0 until words[i].length) { | ||
if (words[i][j] == x) { | ||
ans.add(i) | ||
break | ||
} | ||
} | ||
} | ||
return ans | ||
} | ||
} |
40 changes: 40 additions & 0 deletions
40
src/main/kotlin/g2901_3000/s2942_find_words_containing_character/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,40 @@ | ||
2942\. Find Words Containing Character | ||
|
||
Easy | ||
|
||
You are given a **0-indexed** array of strings `words` and a character `x`. | ||
|
||
Return _an **array of indices** representing the words that contain the character_ `x`. | ||
|
||
**Note** that the returned array may be in **any** order. | ||
|
||
**Example 1:** | ||
|
||
**Input:** words = ["leet","code"], x = "e" | ||
|
||
**Output:** [0,1] | ||
|
||
**Explanation:** "e" occurs in both words: "l**<ins>ee</ins>**t", and "cod<ins>**e**</ins>". Hence, we return indices 0 and 1. | ||
|
||
**Example 2:** | ||
|
||
**Input:** words = ["abc","bcd","aaaa","cbc"], x = "a" | ||
|
||
**Output:** [0,2] | ||
|
||
**Explanation:** "a" occurs in "**<ins>a</ins>**bc", and "<ins>**aaaa**</ins>". Hence, we return indices 0 and 2. | ||
|
||
**Example 3:** | ||
|
||
**Input:** words = ["abc","bcd","aaaa","cbc"], x = "z" | ||
|
||
**Output:** [] | ||
|
||
**Explanation:** "z" does not occur in any of the words. Hence, we return an empty array. | ||
|
||
**Constraints:** | ||
|
||
* `1 <= words.length <= 50` | ||
* `1 <= words[i].length <= 50` | ||
* `x` is a lowercase English letter. | ||
* `words[i]` consists only of lowercase English letters. |
33 changes: 33 additions & 0 deletions
33
src/main/kotlin/g2901_3000/s2943_maximize_area_of_square_hole_in_grid/Solution.kt
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,33 @@ | ||
package g2901_3000.s2943_maximize_area_of_square_hole_in_grid | ||
|
||
// #Medium #Array #Sorting #2024_01_07_Time_180_ms_(86.67%)_Space_38.1_MB_(60.00%) | ||
|
||
import kotlin.math.max | ||
import kotlin.math.min | ||
|
||
@Suppress("UNUSED_PARAMETER") | ||
class Solution { | ||
fun maximizeSquareHoleArea(n: Int, m: Int, hBars: IntArray, vBars: IntArray): Int { | ||
val x = find(hBars) | ||
val y = find(vBars) | ||
val res = min(x, y) + 1 | ||
return res * res | ||
} | ||
|
||
private fun find(arr: IntArray): Int { | ||
arr.sort() | ||
var res = 1 | ||
var i = 0 | ||
val n = arr.size | ||
while (i < n) { | ||
var count = 1 | ||
while (i + 1 < n && arr[i] + 1 == arr[i + 1]) { | ||
i++ | ||
count++ | ||
} | ||
i++ | ||
res = max(res, count) | ||
} | ||
return res | ||
} | ||
} |
100 changes: 100 additions & 0 deletions
100
src/main/kotlin/g2901_3000/s2943_maximize_area_of_square_hole_in_grid/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,100 @@ | ||
2943\. Maximize Area of Square Hole in Grid | ||
|
||
Medium | ||
|
||
There is a grid with `n + 2` **horizontal** bars and `m + 2` **vertical** bars, and initially containing `1 x 1` unit cells. | ||
|
||
The bars are **1-indexed**. | ||
|
||
You are given the two integers, `n` and `m`. | ||
|
||
You are also given two integer arrays: `hBars` and `vBars`. | ||
|
||
* `hBars` contains **distinct** horizontal bars in the range `[2, n + 1]`. | ||
* `vBars` contains **distinct** vertical bars in the range `[2, m + 1]`. | ||
|
||
You are allowed to **remove** bars that satisfy any of the following conditions: | ||
|
||
* If it is a horizontal bar, it must correspond to a value in `hBars`. | ||
* If it is a vertical bar, it must correspond to a value in `vBars`. | ||
|
||
Return _an integer denoting the **maximum** area of a **square-shaped** hole in the grid after removing some bars (**possibly none**)._ | ||
|
||
**Example 1:** | ||
|
||
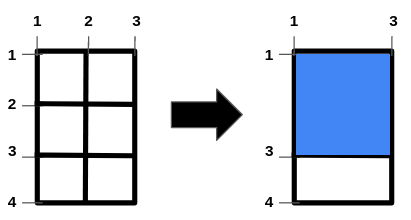 | ||
|
||
**Input:** n = 2, m = 1, hBars = [2,3], vBars = [2] | ||
|
||
**Output:** 4 | ||
|
||
**Explanation:** The left image shows the initial grid formed by the bars. | ||
|
||
The horizontal bars are in the range [1,4], and the vertical bars are in the range [1,3]. | ||
|
||
It is allowed to remove horizontal bars [2,3] and the vertical bar [2]. | ||
|
||
One way to get the maximum square-shaped hole is by removing horizontal bar 2 and vertical bar 2. | ||
|
||
The resulting grid is shown on the right. | ||
|
||
The hole has an area of 4. | ||
|
||
It can be shown that it is not possible to get a square hole with an area more than 4. | ||
|
||
Hence, the answer is 4. | ||
|
||
**Example 2:** | ||
|
||
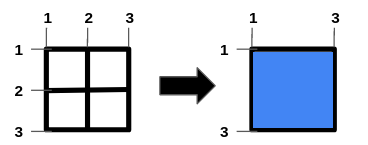 | ||
|
||
**Input:** n = 1, m = 1, hBars = [2], vBars = [2] | ||
|
||
**Output:** 4 | ||
|
||
**Explanation:** The left image shows the initial grid formed by the bars. | ||
|
||
The horizontal bars are in the range [1,3], and the vertical bars are in the range [1,3]. | ||
|
||
It is allowed to remove the horizontal bar [2] and the vertical bar [2]. | ||
|
||
To get the maximum square-shaped hole, we remove horizontal bar 2 and vertical bar 2. | ||
|
||
The resulting grid is shown on the right. | ||
|
||
The hole has an area of 4. | ||
|
||
Hence, the answer is 4, and it is the maximum possible. | ||
|
||
**Example 3:** | ||
|
||
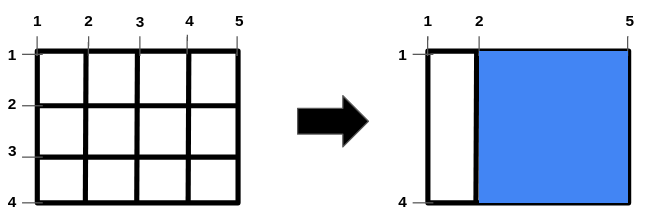 | ||
|
||
**Input:** n = 2, m = 3, hBars = [2,3], vBars = [2,3,4] | ||
|
||
**Output:** 9 | ||
|
||
**Explanation:** The left image shows the initial grid formed by the bars. | ||
|
||
The horizontal bars are in the range [1,4], and the vertical bars are in the range [1,5]. | ||
|
||
It is allowed to remove horizontal bars [2,3] and vertical bars [2,3,4]. | ||
|
||
One way to get the maximum square-shaped hole is by removing horizontal bars 2 and 3, and vertical bars 3 and 4. | ||
|
||
The resulting grid is shown on the right. | ||
|
||
The hole has an area of 9. | ||
|
||
It can be shown that it is not possible to get a square hole with an area more than 9. Hence, the answer is 9. | ||
|
||
**Constraints:** | ||
|
||
* <code>1 <= n <= 10<sup>9</sup></code> | ||
* <code>1 <= m <= 10<sup>9</sup></code> | ||
* `1 <= hBars.length <= 100` | ||
* `2 <= hBars[i] <= n + 1` | ||
* `1 <= vBars.length <= 100` | ||
* `2 <= vBars[i] <= m + 1` | ||
* All values in `hBars` are distinct. | ||
* All values in `vBars` are distinct. |
28 changes: 28 additions & 0 deletions
28
src/main/kotlin/g2901_3000/s2944_minimum_number_of_coins_for_fruits/Solution.kt
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,28 @@ | ||
package g2901_3000.s2944_minimum_number_of_coins_for_fruits | ||
|
||
// #Medium #Array #Dynamic_Programming #Heap_Priority_Queue #Queue #Monotonic_Queue | ||
// #2024_01_07_Time_194_ms_(84.62%)_Space_37.5_MB_(92.31%) | ||
|
||
import kotlin.math.min | ||
|
||
class Solution { | ||
fun minimumCoins(prices: IntArray): Int { | ||
val n = prices.size | ||
val dp = IntArray(n) | ||
dp[n - 1] = prices[n - 1] | ||
for (i in n - 2 downTo 0) { | ||
val pos = i + 1 | ||
val acquired = i + pos | ||
if (acquired + 1 < n) { | ||
var min = Int.MAX_VALUE | ||
for (j in acquired + 1 downTo i + 1) { | ||
min = min(min.toDouble(), dp[j].toDouble()).toInt() | ||
} | ||
dp[i] = prices[i] + min | ||
} else { | ||
dp[i] = prices[i] | ||
} | ||
} | ||
return dp[0] | ||
} | ||
} |
56 changes: 56 additions & 0 deletions
56
src/main/kotlin/g2901_3000/s2944_minimum_number_of_coins_for_fruits/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,56 @@ | ||
2944\. Minimum Number of Coins for Fruits | ||
|
||
Medium | ||
|
||
You are at a fruit market with different types of exotic fruits on display. | ||
|
||
You are given a **1-indexed** array `prices`, where `prices[i]` denotes the number of coins needed to purchase the <code>i<sup>th</sup></code> fruit. | ||
|
||
The fruit market has the following offer: | ||
|
||
* If you purchase the <code>i<sup>th</sup></code> fruit at `prices[i]` coins, you can get the next `i` fruits for free. | ||
|
||
**Note** that even if you **can** take fruit `j` for free, you can still purchase it for `prices[j]` coins to receive a new offer. | ||
|
||
Return _the **minimum** number of coins needed to acquire all the fruits_. | ||
|
||
**Example 1:** | ||
|
||
**Input:** prices = [3,1,2] | ||
|
||
**Output:** 4 | ||
|
||
**Explanation:** You can acquire the fruits as follows: | ||
|
||
- Purchase the 1<sup>st</sup> fruit with 3 coins, you are allowed to take the 2<sup>nd</sup> fruit for free. | ||
|
||
- Purchase the 2<sup>nd</sup> fruit with 1 coin, you are allowed to take the 3<sup>rd</sup> fruit for free. | ||
|
||
- Take the 3<sup>rd</sup> fruit for free. | ||
|
||
Note that even though you were allowed to take the 2<sup>nd</sup> fruit for free, you purchased it because it is more optimal. | ||
|
||
It can be proven that 4 is the minimum number of coins needed to acquire all the fruits. | ||
|
||
**Example 2:** | ||
|
||
**Input:** prices = [1,10,1,1] | ||
|
||
**Output:** 2 | ||
|
||
**Explanation:** You can acquire the fruits as follows: | ||
|
||
- Purchase the 1<sup>st</sup> fruit with 1 coin, you are allowed to take the 2<sup>nd</sup> fruit for free. | ||
|
||
- Take the 2<sup>nd</sup> fruit for free. | ||
|
||
- Purchase the 3<sup>rd</sup> fruit for 1 coin, you are allowed to take the 4<sup>th</sup> fruit for free. | ||
|
||
- Take the 4<sup>t</sup><sup>h</sup> fruit for free. | ||
|
||
It can be proven that 2 is the minimum number of coins needed to acquire all the fruits. | ||
|
||
**Constraints:** | ||
|
||
* `1 <= prices.length <= 1000` | ||
* <code>1 <= prices[i] <= 10<sup>5</sup></code> |
31 changes: 31 additions & 0 deletions
31
src/test/kotlin/g2901_3000/s2942_find_words_containing_character/SolutionTest.kt
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,31 @@ | ||
package g2901_3000.s2942_find_words_containing_character | ||
|
||
import org.hamcrest.CoreMatchers.equalTo | ||
import org.hamcrest.MatcherAssert.assertThat | ||
import org.junit.jupiter.api.Test | ||
|
||
internal class SolutionTest { | ||
@Test | ||
fun findWordsContaining() { | ||
assertThat( | ||
Solution().findWordsContaining(arrayOf("leet", "code"), 'e'), | ||
equalTo(mutableListOf(0, 1)) | ||
) | ||
} | ||
|
||
@Test | ||
fun findWordsContaining2() { | ||
assertThat( | ||
Solution().findWordsContaining(arrayOf("abc", "bcd", "aaaa", "cbc"), 'a'), | ||
equalTo(mutableListOf(0, 2)) | ||
) | ||
} | ||
|
||
@Test | ||
fun findWordsContaining3() { | ||
assertThat( | ||
Solution().findWordsContaining(arrayOf("abc", "bcd", "aaaa", "cbc"), 'z'), | ||
equalTo(mutableListOf<Any>()) | ||
) | ||
} | ||
} |
31 changes: 31 additions & 0 deletions
31
src/test/kotlin/g2901_3000/s2943_maximize_area_of_square_hole_in_grid/SolutionTest.kt
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,31 @@ | ||
package g2901_3000.s2943_maximize_area_of_square_hole_in_grid | ||
|
||
import org.hamcrest.CoreMatchers.equalTo | ||
import org.hamcrest.MatcherAssert.assertThat | ||
import org.junit.jupiter.api.Test | ||
|
||
internal class SolutionTest { | ||
@Test | ||
fun maximizeSquareHoleArea() { | ||
assertThat( | ||
Solution().maximizeSquareHoleArea(2, 1, intArrayOf(2, 3), intArrayOf(2)), | ||
equalTo(4) | ||
) | ||
} | ||
|
||
@Test | ||
fun maximizeSquareHoleArea2() { | ||
assertThat( | ||
Solution().maximizeSquareHoleArea(1, 1, intArrayOf(2), intArrayOf(2)), | ||
equalTo(4) | ||
) | ||
} | ||
|
||
@Test | ||
fun maximizeSquareHoleArea3() { | ||
assertThat( | ||
Solution().maximizeSquareHoleArea(2, 3, intArrayOf(2, 3), intArrayOf(2, 3, 4)), | ||
equalTo(9) | ||
) | ||
} | ||
} |
17 changes: 17 additions & 0 deletions
17
src/test/kotlin/g2901_3000/s2944_minimum_number_of_coins_for_fruits/SolutionTest.kt
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,17 @@ | ||
package g2901_3000.s2944_minimum_number_of_coins_for_fruits | ||
|
||
import org.hamcrest.CoreMatchers.equalTo | ||
import org.hamcrest.MatcherAssert.assertThat | ||
import org.junit.jupiter.api.Test | ||
|
||
internal class SolutionTest { | ||
@Test | ||
fun minimumCoins() { | ||
assertThat(Solution().minimumCoins(intArrayOf(3, 1, 2)), equalTo(4)) | ||
} | ||
|
||
@Test | ||
fun minimumCoins2() { | ||
assertThat(Solution().minimumCoins(intArrayOf(1, 10, 1, 1)), equalTo(2)) | ||
} | ||
} |