forked from LeetCode-in-Php/LeetCode-in-Php
-
Notifications
You must be signed in to change notification settings - Fork 0
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
Showing
30 changed files
with
1,025 additions
and
0 deletions.
There are no files selected for viewing
29 changes: 29 additions & 0 deletions
29
src/main/php/g0001_0100/s0011_container_with_most_water/Solution.php
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,29 @@ | ||
<?php | ||
|
||
namespace leetcode\g0001_0100\s0011_container_with_most_water; | ||
|
||
// #Medium #Top_100_Liked_Questions #Top_Interview_Questions #Array #Greedy #Two_Pointers | ||
// #Algorithm_II_Day_4_Two_Pointers #Big_O_Time_O(n)_Space_O(1) | ||
// #2023_12_07_Time_177_ms_(88.58%)_Space_31.7_MB_(66.67%) | ||
|
||
class Solution { | ||
/** | ||
* @param Integer[] $height | ||
* @return Integer | ||
*/ | ||
public function maxArea($height) { | ||
$maxArea = -1; | ||
$left = 0; | ||
$right = count($height) - 1; | ||
while ($left < $right) { | ||
if ($height[$left] < $height[$right]) { | ||
$maxArea = max($maxArea, $height[$left] * ($right - $left)); | ||
$left++; | ||
} else { | ||
$maxArea = max($maxArea, $height[$right] * ($right - $left)); | ||
$right--; | ||
} | ||
} | ||
return $maxArea; | ||
} | ||
} |
41 changes: 41 additions & 0 deletions
41
src/main/php/g0001_0100/s0011_container_with_most_water/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,41 @@ | ||
11\. Container With Most Water | ||
|
||
Medium | ||
|
||
Given `n` non-negative integers <code>a<sub>1</sub>, a<sub>2</sub>, ..., a<sub>n</sub></code> , where each represents a point at coordinate <code>(i, a<sub>i</sub>)</code>. `n` vertical lines are drawn such that the two endpoints of the line `i` is at <code>(i, a<sub>i</sub>)</code> and `(i, 0)`. Find two lines, which, together with the x-axis forms a container, such that the container contains the most water. | ||
|
||
**Notice** that you may not slant the container. | ||
|
||
**Example 1:** | ||
|
||
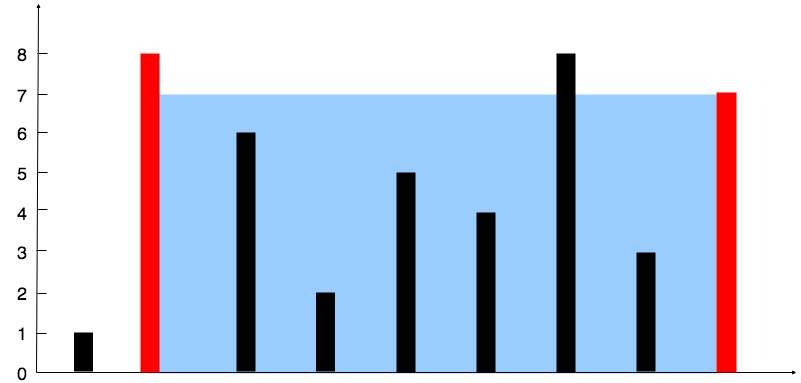 | ||
|
||
**Input:** height = [1,8,6,2,5,4,8,3,7] | ||
|
||
**Output:** 49 | ||
|
||
**Explanation:** The above vertical lines are represented by array [1,8,6,2,5,4,8,3,7]. In this case, the max area of water (blue section) the container can contain is 49. | ||
|
||
**Example 2:** | ||
|
||
**Input:** height = [1,1] | ||
|
||
**Output:** 1 | ||
|
||
**Example 3:** | ||
|
||
**Input:** height = [4,3,2,1,4] | ||
|
||
**Output:** 16 | ||
|
||
**Example 4:** | ||
|
||
**Input:** height = [1,2,1] | ||
|
||
**Output:** 2 | ||
|
||
**Constraints:** | ||
|
||
* `n == height.length` | ||
* <code>2 <= n <= 10<sup>5</sup></code> | ||
* <code>0 <= height[i] <= 10<sup>4</sup></code> |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,47 @@ | ||
<?php | ||
|
||
namespace leetcode\g0001_0100\s0015_3sum; | ||
|
||
// #Medium #Top_100_Liked_Questions #Top_Interview_Questions #Array #Sorting #Two_Pointers | ||
// #Data_Structure_II_Day_1_Array #Algorithm_II_Day_3_Two_Pointers #Udemy_Two_Pointers | ||
// #Big_O_Time_O(n^2)_Space_O(1) #Big_O_Time_O(n*log(n))_Space_O(n^2) | ||
// #2023_12_07_Time_252_ms_(77.94%)_Space_28.5_MB_(94.12%) | ||
|
||
class Solution { | ||
/** | ||
* @param Integer[] $nums | ||
* @return Integer[][] | ||
*/ | ||
public function threeSum($nums) { | ||
sort($nums); | ||
$len = count($nums); | ||
$result = []; | ||
for ($i = 0; $i < $len - 2; $i++) { | ||
$l = $i + 1; | ||
$r = $len - 1; | ||
while ($r > $l) { | ||
$sum = $nums[$i] + $nums[$l] + $nums[$r]; | ||
if ($sum < 0) { | ||
$l++; | ||
} elseif ($sum > 0) { | ||
$r--; | ||
} else { | ||
$list = [$nums[$i], $nums[$l], $nums[$r]]; | ||
$result[] = $list; | ||
while ($l < $r && $nums[$l + 1] == $nums[$l]) { | ||
$l++; | ||
} | ||
while ($r > $l && $nums[$r - 1] == $nums[$r]) { | ||
$r--; | ||
} | ||
$l++; | ||
$r--; | ||
} | ||
} | ||
while ($i < $len - 1 && $nums[$i + 1] == $nums[$i]) { | ||
$i++; | ||
} | ||
} | ||
return $result; | ||
} | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,30 @@ | ||
15\. 3Sum | ||
|
||
Medium | ||
|
||
Given an integer array nums, return all the triplets `[nums[i], nums[j], nums[k]]` such that `i != j`, `i != k`, and `j != k`, and `nums[i] + nums[j] + nums[k] == 0`. | ||
|
||
Notice that the solution set must not contain duplicate triplets. | ||
|
||
**Example 1:** | ||
|
||
**Input:** nums = [-1,0,1,2,-1,-4] | ||
|
||
**Output:** [[-1,-1,2],[-1,0,1]] | ||
|
||
**Example 2:** | ||
|
||
**Input:** nums = [] | ||
|
||
**Output:** [] | ||
|
||
**Example 3:** | ||
|
||
**Input:** nums = [0] | ||
|
||
**Output:** [] | ||
|
||
**Constraints:** | ||
|
||
* `0 <= nums.length <= 3000` | ||
* <code>-10<sup>5</sup> <= nums[i] <= 10<sup>5</sup></code> |
40 changes: 40 additions & 0 deletions
40
src/main/php/g0001_0100/s0017_letter_combinations_of_a_phone_number/Solution.php
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,40 @@ | ||
<?php | ||
|
||
namespace leetcode\g0001_0100\s0017_letter_combinations_of_a_phone_number; | ||
|
||
// #Medium #Top_100_Liked_Questions #Top_Interview_Questions #String #Hash_Table #Backtracking | ||
// #Algorithm_II_Day_11_Recursion_Backtracking #Udemy_Backtracking/Recursion | ||
// #Big_O_Time_O(4^n)_Space_O(n) #2023_12_07_Time_5_ms_(60.19%)_Space_19.1_MB_(59.26%) | ||
|
||
class Solution { | ||
/** | ||
* @param String $digits | ||
* @return String[] | ||
*/ | ||
public function letterCombinations($digits) { | ||
if (empty($digits)) { | ||
return []; | ||
} | ||
$letters = ["", "", "abc", "def", "ghi", "jkl", "mno", "pqrs", "tuv", "wxyz"]; | ||
$ans = []; | ||
$curr = ''; | ||
$this->findCombinations(0, $digits, $letters, $curr, $ans); | ||
return $ans; | ||
} | ||
|
||
private function findCombinations($start, $nums, $letters, &$curr, &$ans) { | ||
if (strlen($curr) == strlen($nums)) { | ||
$ans[] = $curr; | ||
return; | ||
} | ||
for ($i = $start; $i < strlen($nums); $i++) { | ||
$n = intval($nums[$i]); | ||
for ($j = 0; $j < strlen($letters[$n]); $j++) { | ||
$ch = $letters[$n][$j]; | ||
$curr .= $ch; | ||
$this->findCombinations($i + 1, $nums, $letters, $curr, $ans); | ||
$curr = substr($curr, 0, -1); | ||
} | ||
} | ||
} | ||
} |
32 changes: 32 additions & 0 deletions
32
src/main/php/g0001_0100/s0017_letter_combinations_of_a_phone_number/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,32 @@ | ||
17\. Letter Combinations of a Phone Number | ||
|
||
Medium | ||
|
||
Given a string containing digits from `2-9` inclusive, return all possible letter combinations that the number could represent. Return the answer in **any order**. | ||
|
||
A mapping of digit to letters (just like on the telephone buttons) is given below. Note that 1 does not map to any letters. | ||
|
||
 | ||
|
||
**Example 1:** | ||
|
||
**Input:** digits = "23" | ||
|
||
**Output:** ["ad","ae","af","bd","be","bf","cd","ce","cf"] | ||
|
||
**Example 2:** | ||
|
||
**Input:** digits = "" | ||
|
||
**Output:** [] | ||
|
||
**Example 3:** | ||
|
||
**Input:** digits = "2" | ||
|
||
**Output:** ["a","b","c"] | ||
|
||
**Constraints:** | ||
|
||
* `0 <= digits.length <= 4` | ||
* `digits[i]` is a digit in the range `['2', '9']`. |
43 changes: 43 additions & 0 deletions
43
src/main/php/g0001_0100/s0019_remove_nth_node_from_end_of_list/Solution.php
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,43 @@ | ||
<?php | ||
|
||
namespace leetcode\g0001_0100\s0019_remove_nth_node_from_end_of_list; | ||
|
||
// #Medium #Top_100_Liked_Questions #Top_Interview_Questions #Two_Pointers #Linked_List | ||
// #Algorithm_I_Day_5_Two_Pointers #Level_2_Day_3_Linked_List #Big_O_Time_O(L)_Space_O(L) | ||
// #2023_12_07_Time_4_ms_(81.93%)_Space_18.9_MB_(91.57%) | ||
|
||
use leetcode\com_github_leetcode\ListNode; | ||
|
||
/* | ||
* Definition for a singly-linked list. | ||
* class ListNode { | ||
* public $val = 0; | ||
* public $next = null; | ||
* function __construct($val = 0, $next = null) { | ||
* $this->val = $val; | ||
* $this->next = $next; | ||
* } | ||
* } | ||
*/ | ||
|
||
class Solution { | ||
private $n; | ||
|
||
public function removeNthFromEnd($head, $n) { | ||
$this->n = $n; | ||
$node = new ListNode(0, $head); | ||
$this->removeNth($node); | ||
return $node->next; | ||
} | ||
|
||
private function removeNth($node) { | ||
if ($node->next === null) { | ||
return; | ||
} | ||
$this->removeNth($node->next); | ||
$this->n--; | ||
if ($this->n == 0) { | ||
$node->next = $node->next->next; | ||
} | ||
} | ||
} |
34 changes: 34 additions & 0 deletions
34
src/main/php/g0001_0100/s0019_remove_nth_node_from_end_of_list/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,34 @@ | ||
19\. Remove Nth Node From End of List | ||
|
||
Medium | ||
|
||
Given the `head` of a linked list, remove the `nth` node from the end of the list and return its head. | ||
|
||
**Example 1:** | ||
|
||
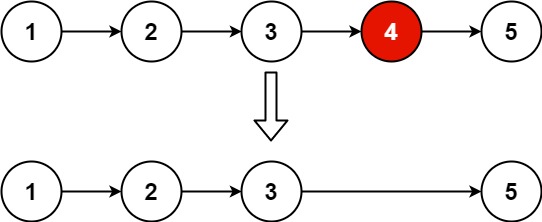 | ||
|
||
**Input:** head = [1,2,3,4,5], n = 2 | ||
|
||
**Output:** [1,2,3,5] | ||
|
||
**Example 2:** | ||
|
||
**Input:** head = [1], n = 1 | ||
|
||
**Output:** [] | ||
|
||
**Example 3:** | ||
|
||
**Input:** head = [1,2], n = 1 | ||
|
||
**Output:** [1] | ||
|
||
**Constraints:** | ||
|
||
* The number of nodes in the list is `sz`. | ||
* `1 <= sz <= 30` | ||
* `0 <= Node.val <= 100` | ||
* `1 <= n <= sz` | ||
|
||
**Follow up:** Could you do this in one pass? |
32 changes: 32 additions & 0 deletions
32
src/main/php/g0001_0100/s0020_valid_parentheses/Solution.php
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,32 @@ | ||
<?php | ||
|
||
namespace leetcode\g0001_0100\s0020_valid_parentheses; | ||
|
||
// #Easy #Top_100_Liked_Questions #Top_Interview_Questions #String #Stack | ||
// #Data_Structure_I_Day_9_Stack_Queue #Udemy_Strings #Big_O_Time_O(n)_Space_O(n) | ||
// #2023_12_07_Time_3_ms_(88.14%)_Space_19.1_MB_(76.99%) | ||
|
||
class Solution { | ||
/** | ||
* @param String $s | ||
* @return Boolean | ||
*/ | ||
public function isValid($s) { | ||
$stack = []; | ||
$length = strlen($s); | ||
for ($i = 0; $i < $length; $i++) { | ||
$c = $s[$i]; | ||
if ($c == '(' || $c == '[' || $c == '{') { | ||
array_push($stack, $c); | ||
} elseif (($c == ')' && !empty($stack) && end($stack) == '(') | ||
|| ($c == '}' && !empty($stack) && end($stack) == '{') | ||
|| ($c == ']' && !empty($stack) && end($stack) == '[') | ||
) { | ||
array_pop($stack); | ||
} else { | ||
return false; | ||
} | ||
} | ||
return empty($stack); | ||
} | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,45 @@ | ||
20\. Valid Parentheses | ||
|
||
Easy | ||
|
||
Given a string `s` containing just the characters `'('`, `')'`, `'{'`, `'}'`, `'['` and `']'`, determine if the input string is valid. | ||
|
||
An input string is valid if: | ||
|
||
1. Open brackets must be closed by the same type of brackets. | ||
2. Open brackets must be closed in the correct order. | ||
|
||
**Example 1:** | ||
|
||
**Input:** s = "()" | ||
|
||
**Output:** true | ||
|
||
**Example 2:** | ||
|
||
**Input:** s = "()[]{}" | ||
|
||
**Output:** true | ||
|
||
**Example 3:** | ||
|
||
**Input:** s = "(]" | ||
|
||
**Output:** false | ||
|
||
**Example 4:** | ||
|
||
**Input:** s = "([)]" | ||
|
||
**Output:** false | ||
|
||
**Example 5:** | ||
|
||
**Input:** s = "{[]}" | ||
|
||
**Output:** true | ||
|
||
**Constraints:** | ||
|
||
* <code>1 <= s.length <= 10<sup>4</sup></code> | ||
* `s` consists of parentheses only `'()[]{}'`. |
Oops, something went wrong.