Hogyan működik?
Flask Backend:
from flask import Flask, render_template, request, redirect, url_for from datetime import datetime import os
app = Flask(name) notes_dir = os.path.join(os.getcwd(), 'notes')
if not os.path.exists(notes_dir): os.makedirs(notes_dir)
@app.route('/') def index(): notes = os.listdir(notes_dir) now = datetime.now() # Aktuális dátum és idő return render_template('index.html', notes=notes, now=now)
@app.route('/new_note', methods=['GET', 'POST']) def new_note(): if request.method == 'POST': note_name = request.form['note_name'] file_path = os.path.join(notes_dir, f"{note_name}.md") with open(file_path, 'w') as f: f.write(request.form['content']) return redirect(url_for('index')) return render_template('edit.html', note=None)
@app.route('/edit/<filename>', methods=['GET', 'POST']) def edit_note(filename): file_path = os.path.join(notes_dir, filename) if request.method == 'POST': with open(file_path, 'w') as f: f.write(request.form['content']) return redirect(url_for('index')) with open(file_path, 'r') as f: content = f.read() return render_template('edit.html', note=content, filename=filename)
@app.route('/view/<filename>') def view_note(filename): file_path = os.path.join(notes_dir, filename) with open(file_path, 'r') as f: content = f.read() return render_template('view.html', content=content)
@app.route('/delete/<filename>') def delete_note(filename): file_path = os.path.join(notes_dir, filename) if os.path.exists(file_path): os.remove(file_path) return redirect(url_for('index'))
if name == 'main': app.run(debug=True, host="0.0.0.0", port=5001)
És ez a base.htm erre épül fel a teljes oldal:
<!DOCTYPE html> <html lang="en"> <head> <link href="https://fonts.googleapis.com/css2?family=Roboto:wght@400;500;700&display=swap" rel="stylesheet"> <link rel="stylesheet" href="https://fonts.googleapis.com/css2?family=Material+Symbols+Outlined&display=swap"> <link href="https://fonts.googleapis.com/icon?family=Material+Icons" rel="stylesheet"> <link href="https://cdnjs.cloudflare.com/ajax/libs/angular-material/1.1.24/angular-material.min.css" rel="stylesheet"> <link href="https://fonts.googleapis.com/icon?family=Material+Icons" rel="stylesheet"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.3/dist/css/bootstrap.min.css" rel="stylesheet"> <meta charset="UTF-8">
<script src="https://cdn.tiny.cloud/1/8yauam9wk26s9zipy53adu6mzj02690i9jw1hpbd5opo66a6/tinymce/7/tinymce.min.js" referrerpolicy="origin"></script> <link href="https://fonts.googleapis.com/css2?family=Roboto:wght@400;500;700&display=swap" rel="stylesheet">
<script type="importmap"> { "imports": { "@material/web/": "https://esm.run/@material/web/" } } </script> <style> form { display: flex; flex-direction: column; align-items: flex-start; gap: 16px; } </style> </style> <script> import '@material/web/button/filled-button.js'; import '@material/web/button/outlined-button.js'; import '@material/web/checkbox/checkbox.js'; </script> <script type="module"> import * as d3 from 'https://esm.run/d3'; import '@material/web/all.js'; import {styles as typescaleStyles} from '@material/web/typography/md-typescale-styles.js';
document.adoptedStyleSheets.push(typescaleStyles.styleSheet);
</script> <script> tinymce.init({ selector: 'textarea', plugins: 'anchor autolink charmap codesample emoticons image link lists media searchreplace table visualblocks wordcount linkchecker', toolbar: 'undo redo | blocks fontfamily fontsize | bold italic underline strikethrough | link image media table | align lineheight | numlist bullist indent outdent | emoticons charmap | removeformat', }); </script> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="{{ url_for('static', filename='css/style.css') }}">
</head> <body> <div class="sidebar"> <div class="nav-item " > <md-icon slot="icon">home</md-icon> Főoldal </div> <div class="nav-item "> <md-icon slot="icon">grade</md-icon> Jegyeim </div> <div class="nav-item " > <md-icon slot="icon">calendar_month</md-icon> Órarend </div> <div class="nav-item " > <md-icon slot="icon">edit_document</md-icon> Dolgozatok </div> <div class="nav-item " > <md-icon slot="icon">logout</md-icon> Kilépés </div> <md-fab class="mode-switch" variant="primary" data-label="Switch Theme" size="medium"> <md-icon slot="icon" aria-hidden="true" id="theme-icon">dark_mode</md-icon> </md-fab> </div>
<div class="container"> {% block content %}{% endblock %} </div> <script> document.addEventListener("DOMContentLoaded", function () { const switchButton = document.querySelector('.mode-switch'); const themeIcon = document.getElementById('theme-icon'); // Function to toggle the dark and light modes const toggleTheme = () => { if (document.documentElement.classList.contains('dark-mode')) { document.documentElement.classList.remove('dark-mode'); document.documentElement.classList.add('light-mode'); themeIcon.textContent = 'light_mode'; // Change icon to light mode localStorage.setItem('theme', 'light'); // Save the theme in localStorage } else { document.documentElement.classList.remove('light-mode'); document.documentElement.classList.add('dark-mode'); themeIcon.textContent = 'dark_mode'; // Change icon to dark mode localStorage.setItem('theme', 'dark'); // Save the theme in localStorage } }; // Load the saved theme from localStorage const savedTheme = localStorage.getItem('theme'); if (savedTheme) { if (savedTheme === 'dark') { document.documentElement.classList.add('dark-mode'); themeIcon.textContent = 'dark_mode'; } else { document.documentElement.classList.add('light-mode'); themeIcon.textContent = 'light_mode'; } } else { // If no theme is saved, default to light mode document.documentElement.classList.add('light-mode'); themeIcon.textContent = 'light_mode'; } // Event listener for the switch button switchButton.addEventListener('click', toggleTheme); }); </script>
</body> </html>
A jegyzetek a .md (markdown) fájlokból kérődik le. Ezáltal teljesen kompatabilis az Obsidian nevü jegyzetkezelővel!
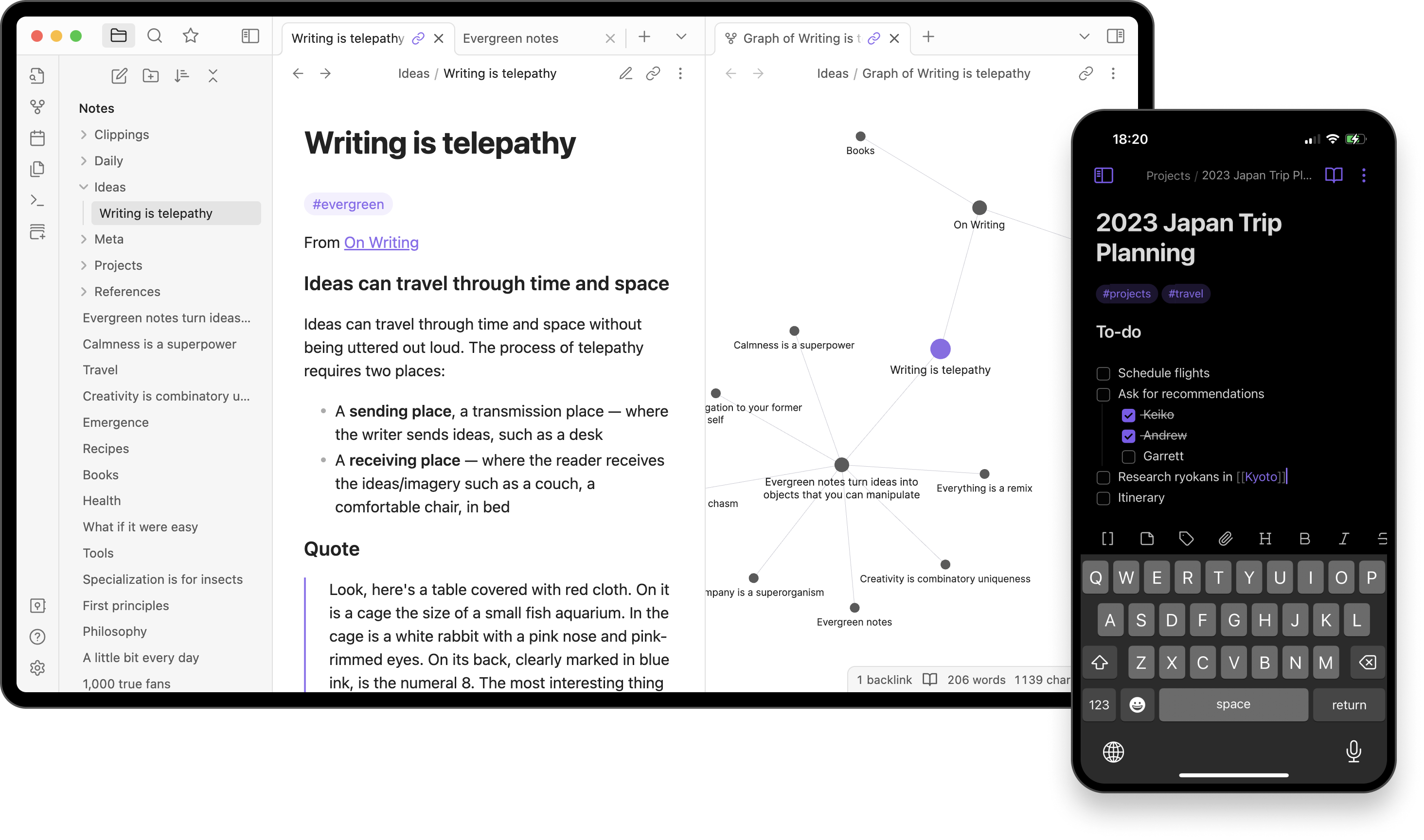
Ezen a linken letölthető az Obsidian!
Elönyök | Hátrányok | Vélemény |
Az Obsidian alapvetően ingyenes | Nincsen szinkronizálás az esközök között | |