-
Notifications
You must be signed in to change notification settings - Fork 5
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
1 parent
f869edd
commit e184191
Showing
15 changed files
with
2,961 additions
and
0 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,138 @@ | ||
--- | ||
title: FR Bank Account Details OCR Python | ||
--- | ||
The Python OCR SDK supports the [Bank Account Details API](https://platform.mindee.com/mindee/bank_account_details). | ||
|
||
Using the [sample below](https://github.com/mindee/client-lib-test-data/blob/main/products/bank_account_details/default_sample.jpg), we are going to illustrate how to extract the data that we want using the OCR SDK. | ||
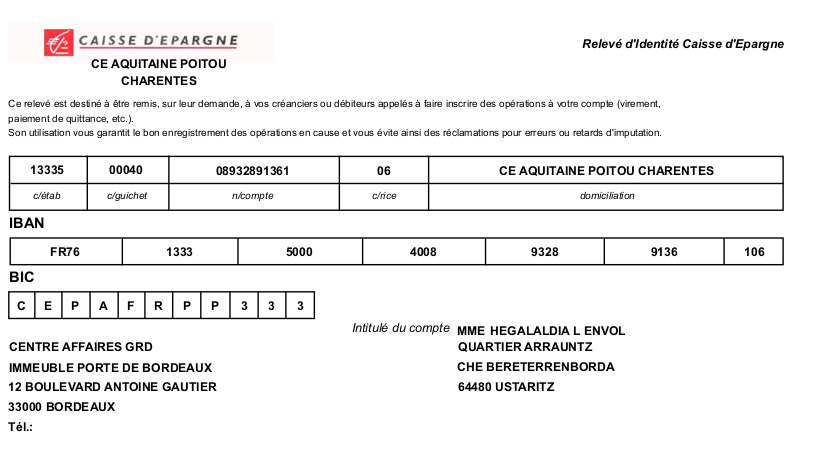 | ||
|
||
# Quick-Start | ||
```py | ||
from mindee import Client, PredictResponse, product | ||
|
||
# Init a new client | ||
mindee_client = Client(api_key="my-api-key") | ||
|
||
# Load a file from disk | ||
input_doc = mindee_client.source_from_path("/path/to/the/file.ext") | ||
|
||
# Load a file from disk and parse it. | ||
# The endpoint name must be specified since it cannot be determined from the class. | ||
result: PredictResponse = mindee_client.parse(product.fr.BankAccountDetailsV2, input_doc) | ||
|
||
# Print a brief summary of the parsed data | ||
print(result.document) | ||
|
||
# # Iterate over all the fields in the document | ||
# for field_name, field_values in result.document.inference.prediction.fields.items(): | ||
# print(field_name, "=", field_values) | ||
``` | ||
|
||
**Output (RST):** | ||
```rst | ||
######## | ||
Document | ||
######## | ||
:Mindee ID: bc8f7265-8dab-49fe-810c-d50049605578 | ||
:Filename: default_sample.jpg | ||
Inference | ||
######### | ||
:Product: mindee/bank_account_details v2.0 | ||
:Rotation applied: Yes | ||
Prediction | ||
========== | ||
:Account Holder's Names: MME HEGALALDIA L ENVOL | ||
:Basic Bank Account Number: | ||
:Bank Code: 13335 | ||
:Branch Code: 00040 | ||
:Key: 06 | ||
:Account Number: 08932891361 | ||
:IBAN: FR7613335000400893289136106 | ||
:SWIFT Code: CEPAFRPP333 | ||
Page Predictions | ||
================ | ||
Page 0 | ||
------ | ||
:Account Holder's Names: MME HEGALALDIA L ENVOL | ||
:Basic Bank Account Number: | ||
:Bank Code: 13335 | ||
:Branch Code: 00040 | ||
:Key: 06 | ||
:Account Number: 08932891361 | ||
:IBAN: FR7613335000400893289136106 | ||
:SWIFT Code: CEPAFRPP333 | ||
``` | ||
|
||
# Field Types | ||
## Standard Fields | ||
These fields are generic and used in several products. | ||
|
||
### Basic Field | ||
Each prediction object contains a set of fields that inherit from the generic `BaseField` class. | ||
A typical `BaseField` object will have the following attributes: | ||
|
||
* **value** (`Union[float, str]`): corresponds to the field value. Can be `None` if no value was extracted. | ||
* **confidence** (`float`): the confidence score of the field prediction. | ||
* **bounding_box** (`[Point, Point, Point, Point]`): contains exactly 4 relative vertices (points) coordinates of a right rectangle containing the field in the document. | ||
* **polygon** (`List[Point]`): contains the relative vertices coordinates (`Point`) of a polygon containing the field in the image. | ||
* **page_id** (`int`): the ID of the page, is `None` when at document-level. | ||
* **reconstructed** (`bool`): indicates whether or not an object was reconstructed (not extracted as the API gave it). | ||
|
||
> **Note:** A `Point` simply refers to a List of two numbers (`[float, float]`). | ||
|
||
Aside from the previous attributes, all basic fields have access to a custom `__str__` method that can be used to print their value as a string. | ||
|
||
### String Field | ||
The text field `StringField` only has one constraint: its **value** is an `Optional[str]`. | ||
|
||
## Specific Fields | ||
Fields which are specific to this product; they are not used in any other product. | ||
|
||
### Basic Bank Account Number Field | ||
Full extraction of BBAN, including: branch code, bank code, account and key. | ||
|
||
A `BankAccountDetailsV2Bban` implements the following attributes: | ||
|
||
* **bban_bank_code** (`str`): The BBAN bank code outputted as a string. | ||
* **bban_branch_code** (`str`): The BBAN branch code outputted as a string. | ||
* **bban_key** (`str`): The BBAN key outputted as a string. | ||
* **bban_number** (`str`): The BBAN Account number outputted as a string. | ||
|
||
# Attributes | ||
The following fields are extracted for Bank Account Details V2: | ||
|
||
## Account Holder's Names | ||
**account_holders_names** : Full extraction of the account holders names. | ||
|
||
```py | ||
print(result.document.inference.prediction.account_holders_names.value) | ||
``` | ||
|
||
## Basic Bank Account Number | ||
**bban** ([BankAccountDetailsV2Bban](#basic-bank-account-number-field)): Full extraction of BBAN, including: branch code, bank code, account and key. | ||
|
||
```py | ||
print(result.document.inference.prediction.bban.value) | ||
``` | ||
|
||
## IBAN | ||
**iban** : Full extraction of the IBAN number. | ||
|
||
```py | ||
print(result.document.inference.prediction.iban.value) | ||
``` | ||
|
||
## SWIFT Code | ||
**swift_code** : Full extraction of the SWIFT code. | ||
|
||
```py | ||
print(result.document.inference.prediction.swift_code.value) | ||
``` | ||
|
||
# Questions? | ||
[Join our Slack](https://join.slack.com/t/mindee-community/shared_invite/zt-1jv6nawjq-FDgFcF2T5CmMmRpl9LLptw) |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,176 @@ | ||
--- | ||
title: US Bank Check OCR Python | ||
--- | ||
The Python OCR SDK supports the [Bank Check API](https://platform.mindee.com/mindee/bank_check). | ||
|
||
Using the [sample below](https://github.com/mindee/client-lib-test-data/blob/main/products/bank_check/default_sample.jpg), we are going to illustrate how to extract the data that we want using the OCR SDK. | ||
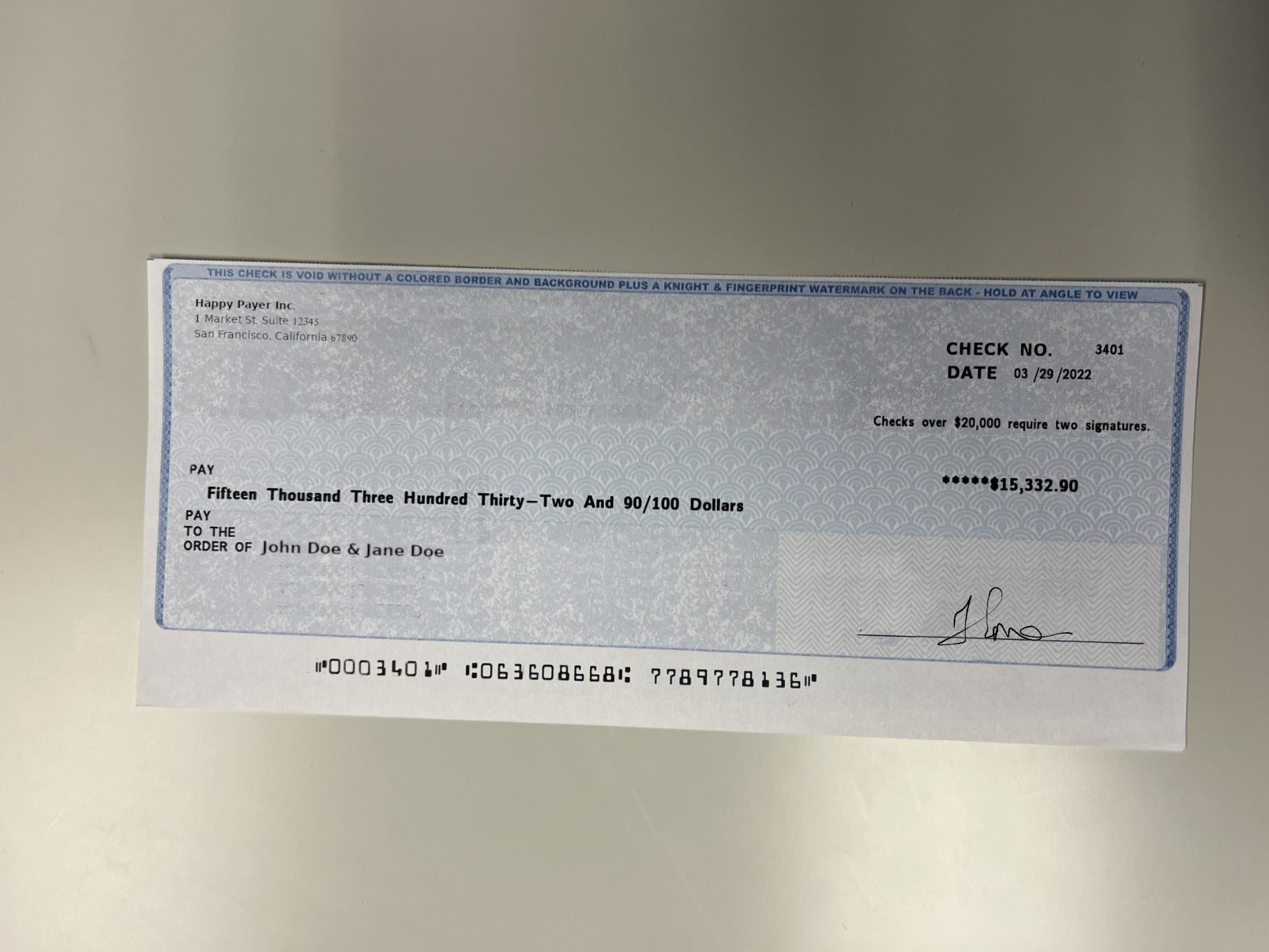 | ||
|
||
# Quick-Start | ||
```py | ||
from mindee import Client, PredictResponse, product | ||
|
||
# Init a new client | ||
mindee_client = Client(api_key="my-api-key") | ||
|
||
# Load a file from disk | ||
input_doc = mindee_client.source_from_path("/path/to/the/file.ext") | ||
|
||
# Load a file from disk and parse it. | ||
# The endpoint name must be specified since it cannot be determined from the class. | ||
result: PredictResponse = mindee_client.parse(product.us.BankCheckV1, input_doc) | ||
|
||
# Print a brief summary of the parsed data | ||
print(result.document) | ||
|
||
# # Iterate over all the fields in the document | ||
# for field_name, field_values in result.document.inference.prediction.fields.items(): | ||
# print(field_name, "=", field_values) | ||
``` | ||
|
||
**Output (RST):** | ||
```rst | ||
######## | ||
Document | ||
######## | ||
:Mindee ID: b9809586-57ae-4f84-a35d-a85b2be1f2a2 | ||
:Filename: default_sample.jpg | ||
Inference | ||
######### | ||
:Product: mindee/bank_check v1.0 | ||
:Rotation applied: Yes | ||
Prediction | ||
========== | ||
:Check Issue Date: 2022-03-29 | ||
:Amount: 15332.90 | ||
:Payees: JOHN DOE | ||
JANE DOE | ||
:Routing Number: | ||
:Account Number: 7789778136 | ||
:Check Number: 0003401 | ||
Page Predictions | ||
================ | ||
Page 0 | ||
------ | ||
:Check Position: Polygon with 21 points. | ||
:Signature Positions: Polygon with 6 points. | ||
:Check Issue Date: 2022-03-29 | ||
:Amount: 15332.90 | ||
:Payees: JOHN DOE | ||
JANE DOE | ||
:Routing Number: | ||
:Account Number: 7789778136 | ||
:Check Number: 0003401 | ||
``` | ||
|
||
# Field Types | ||
## Standard Fields | ||
These fields are generic and used in several products. | ||
|
||
### Basic Field | ||
Each prediction object contains a set of fields that inherit from the generic `BaseField` class. | ||
A typical `BaseField` object will have the following attributes: | ||
|
||
* **value** (`Union[float, str]`): corresponds to the field value. Can be `None` if no value was extracted. | ||
* **confidence** (`float`): the confidence score of the field prediction. | ||
* **bounding_box** (`[Point, Point, Point, Point]`): contains exactly 4 relative vertices (points) coordinates of a right rectangle containing the field in the document. | ||
* **polygon** (`List[Point]`): contains the relative vertices coordinates (`Point`) of a polygon containing the field in the image. | ||
* **page_id** (`int`): the ID of the page, is `None` when at document-level. | ||
* **reconstructed** (`bool`): indicates whether or not an object was reconstructed (not extracted as the API gave it). | ||
|
||
> **Note:** A `Point` simply refers to a List of two numbers (`[float, float]`). | ||
|
||
Aside from the previous attributes, all basic fields have access to a custom `__str__` method that can be used to print their value as a string. | ||
|
||
|
||
### Amount Field | ||
The amount field `AmountField` only has one constraint: its **value** is an `Optional[float]`. | ||
|
||
### Date Field | ||
Aside from the basic `BaseField` attributes, the date field `DateField` also implements the following: | ||
|
||
* **date_object** (`Date`): an accessible representation of the value as a python object. Can be `None`. | ||
|
||
|
||
### Position Field | ||
The position field `PositionField` does not implement all the basic `BaseField` attributes, only **bounding_box**, **polygon** and **page_id**. On top of these, it has access to: | ||
|
||
* **rectangle** (`[Point, Point, Point, Point]`): a Polygon with four points that may be oriented (even beyond canvas). | ||
* **quadrangle** (`[Point, Point, Point, Point]`): a free polygon made up of four points. | ||
|
||
### String Field | ||
The text field `StringField` only has one constraint: its **value** is an `Optional[str]`. | ||
|
||
## Page-Level Fields | ||
Some fields are constrained to the page level, and so will not be retrievable to through the document. | ||
|
||
# Attributes | ||
The following fields are extracted for Bank Check V1: | ||
|
||
## Account Number | ||
**account_number** : The check payer's account number. | ||
|
||
```py | ||
print(result.document.inference.prediction.account_number.value) | ||
``` | ||
|
||
## Amount | ||
**amount** : The amount of the check. | ||
|
||
```py | ||
print(result.document.inference.prediction.amount.value) | ||
``` | ||
|
||
## Check Number | ||
**check_number** : The issuer's check number. | ||
|
||
```py | ||
print(result.document.inference.prediction.check_number.value) | ||
``` | ||
|
||
## Check Position | ||
[📄](#page-level-fields "This field is only present on individual pages.")**check_position** : The position of the check on the document. | ||
|
||
```py | ||
for check_position_elem of result.document.check_position: | ||
print(check_position_elem.polygon) | ||
``` | ||
|
||
## Check Issue Date | ||
**date** : The date the check was issued. | ||
|
||
```py | ||
print(result.document.inference.prediction.date.value) | ||
``` | ||
|
||
## Payees | ||
**payees** : List of the check's payees (recipients). | ||
|
||
```py | ||
for payees_elem in result.document.inference.prediction.payees: | ||
print(payees_elem.value) | ||
``` | ||
|
||
## Routing Number | ||
**routing_number** : The check issuer's routing number. | ||
|
||
```py | ||
print(result.document.inference.prediction.routing_number.value) | ||
``` | ||
|
||
## Signature Positions | ||
[📄](#page-level-fields "This field is only present on individual pages.")**signatures_positions** : List of signature positions | ||
|
||
```py | ||
for page in result.document.inference.pages: | ||
for signatures_positions_elem of page.prediction.signatures_positions): | ||
print(signatures_positions_elem.polygon) | ||
``` | ||
|
||
# Questions? | ||
[Join our Slack](https://join.slack.com/t/mindee-community/shared_invite/zt-1jv6nawjq-FDgFcF2T5CmMmRpl9LLptw) |
Oops, something went wrong.