Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Fix part of #632: Simplify MarginBindingAdapters and fix some RTL cas…
…es. Fix #2116: UI misalignment due to not resetting the layout. (#4965) ## Explanation Fix #2116: Fix part of #632: Simplify MarginBindingAdapters. The code is mostly the same as in PR #4874, which was reverted. This PR fixes the bug that caused the rollback. The rest of the reverted changes in PR #4874 will be included in a separate PR. The fix consists of resetting the layout params after changing the top and bottom margins, by calling View.setLayoutParams(), as explained in the [layout params documentation](https://developer.android.com/reference/android/view/ViewGroup.MarginLayoutParams#bottomMargin). The same call is not needed when calling a method instead of setting a property. It also fixes an RTL issue: if the view is not attached yet, there is no layout direction information available, making the old implementation render in LTR always. Some screenshots: |new|current| |-|-| |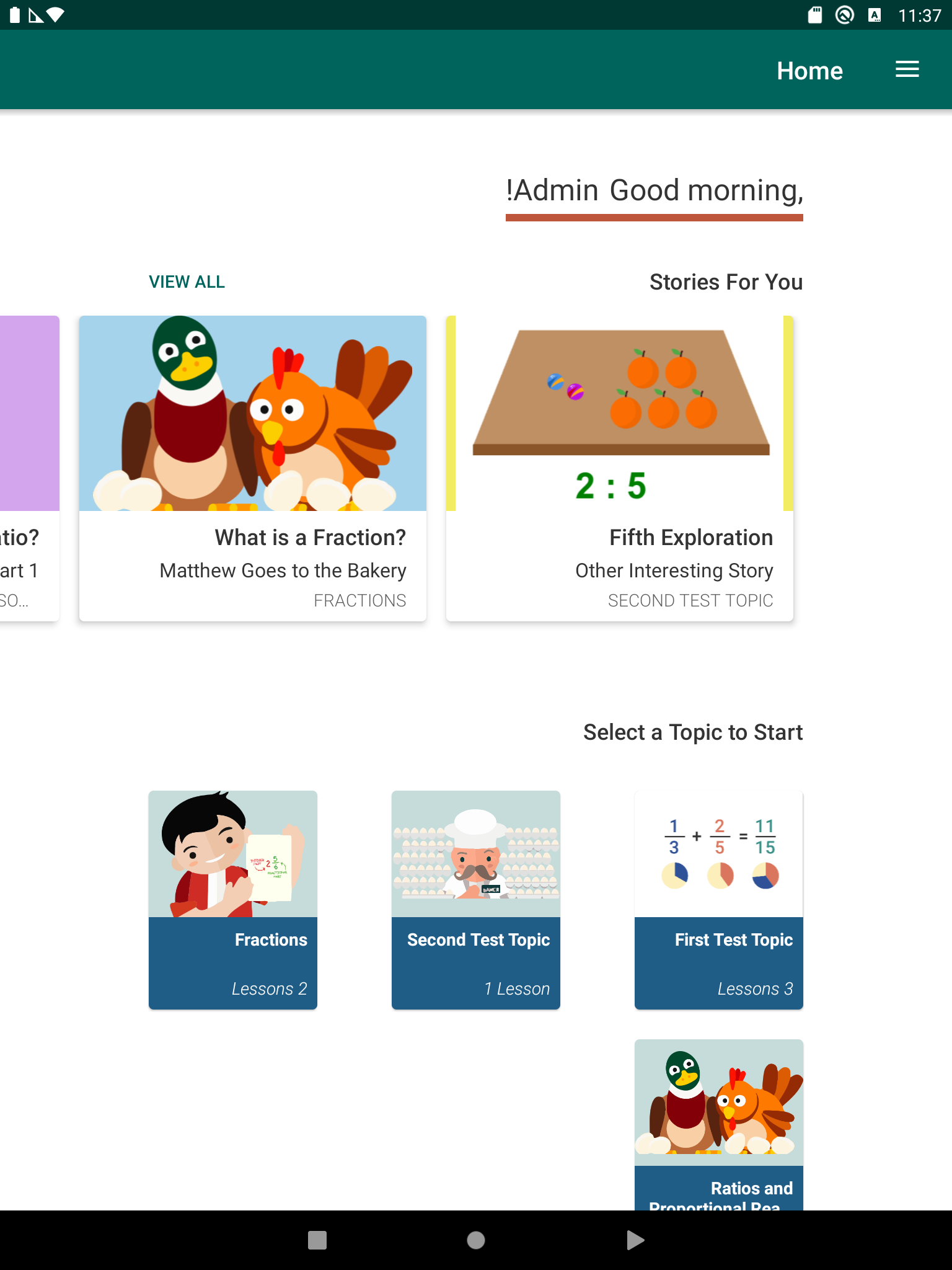 | 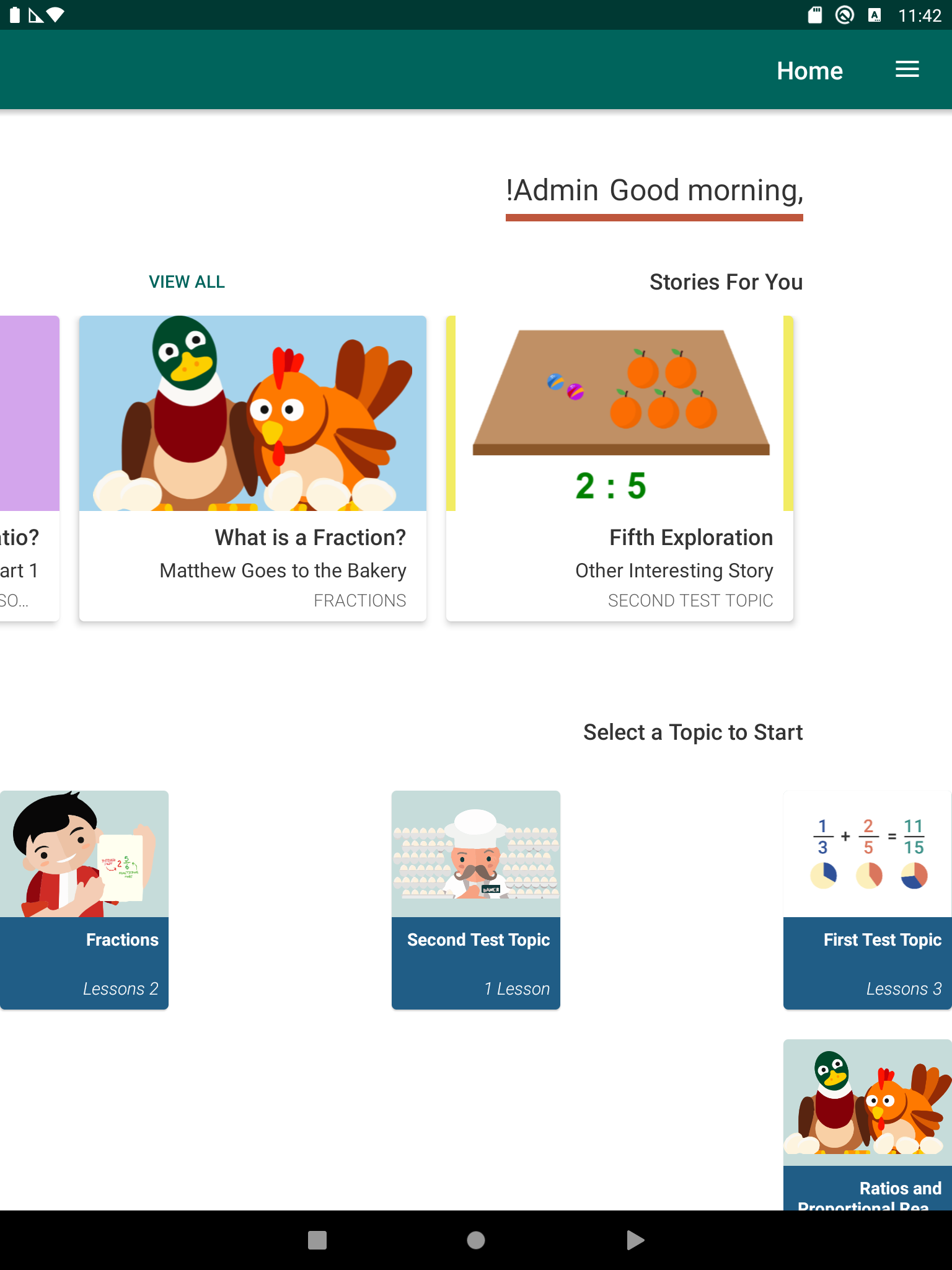| |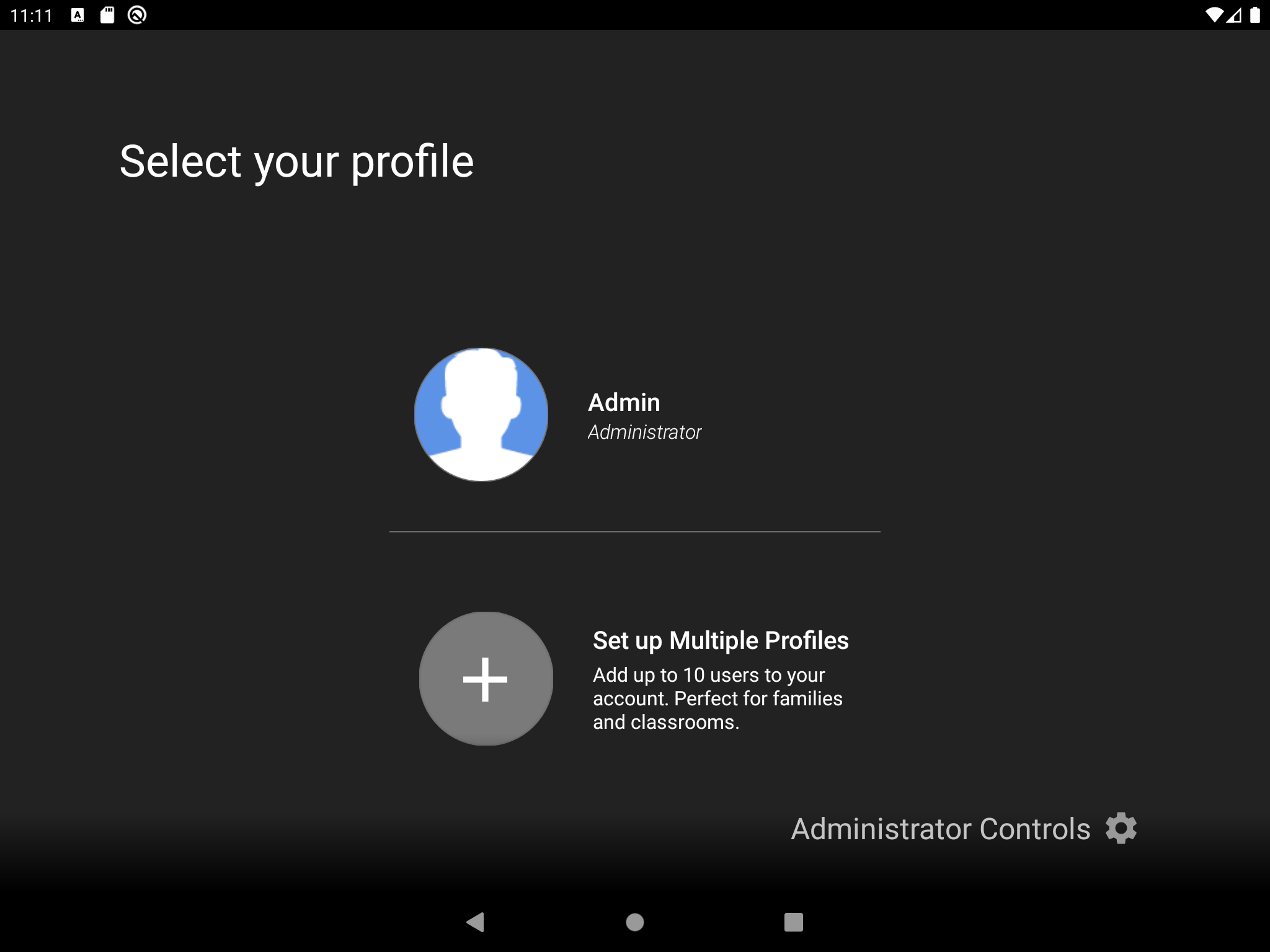|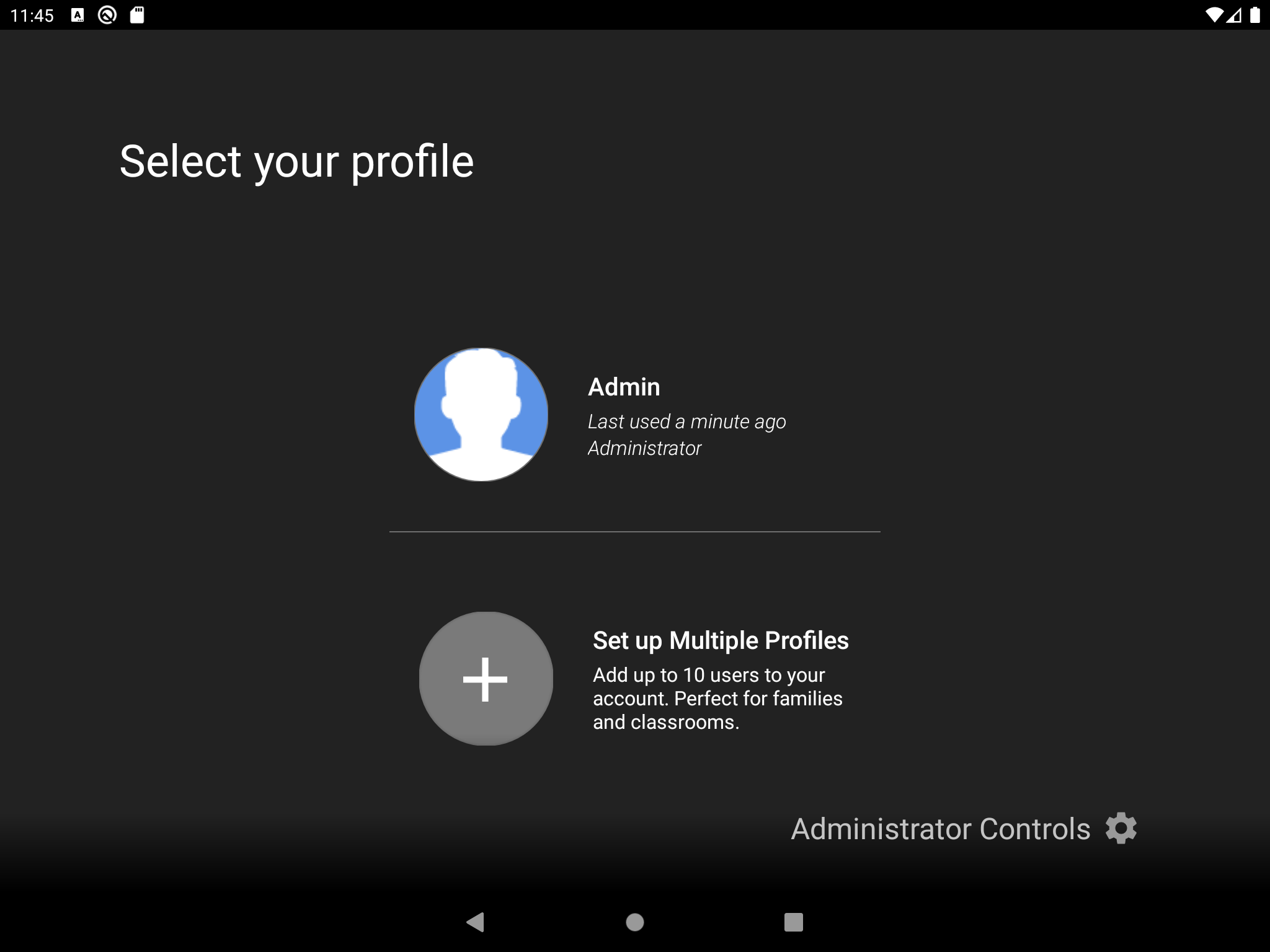| |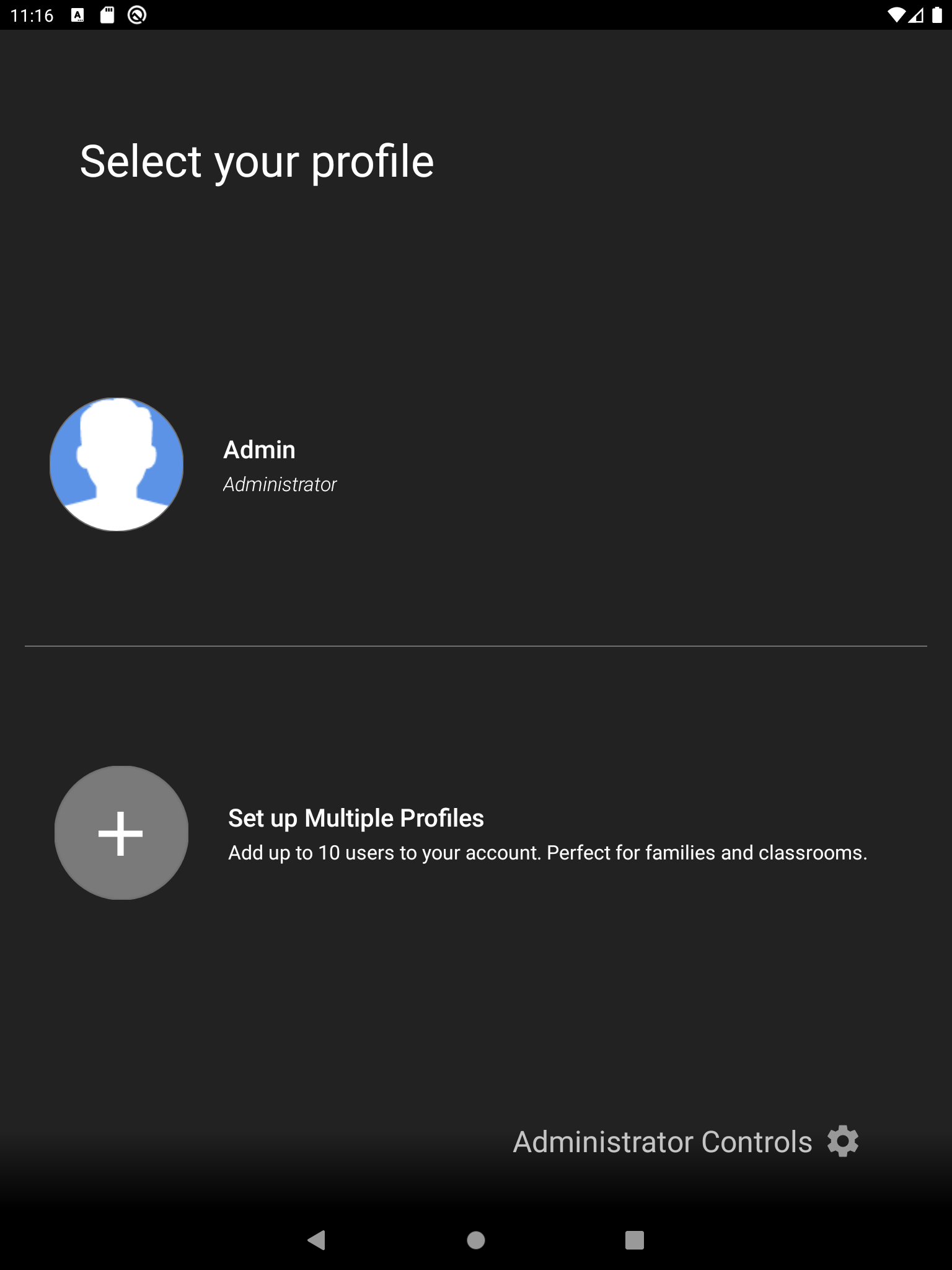|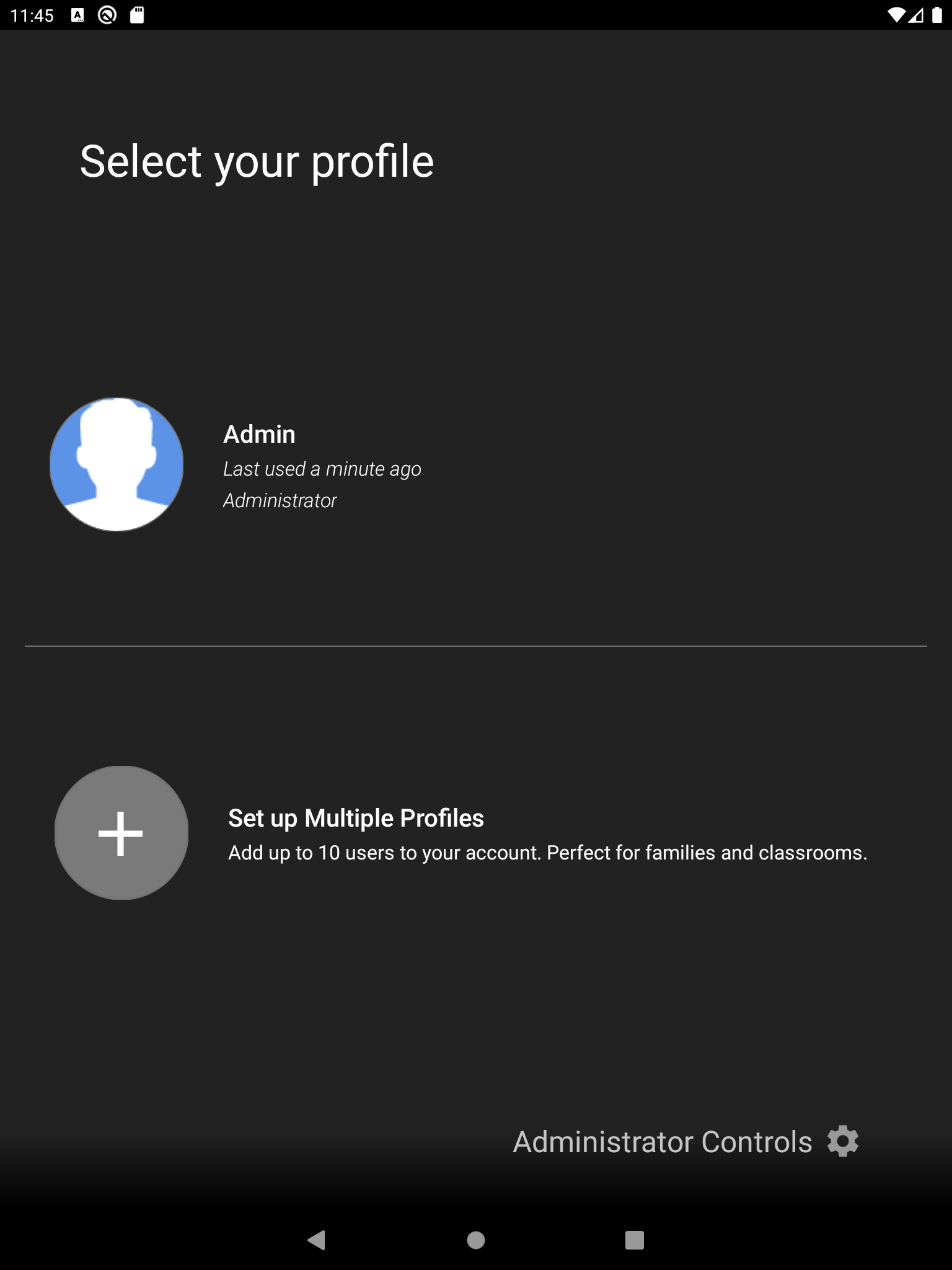| |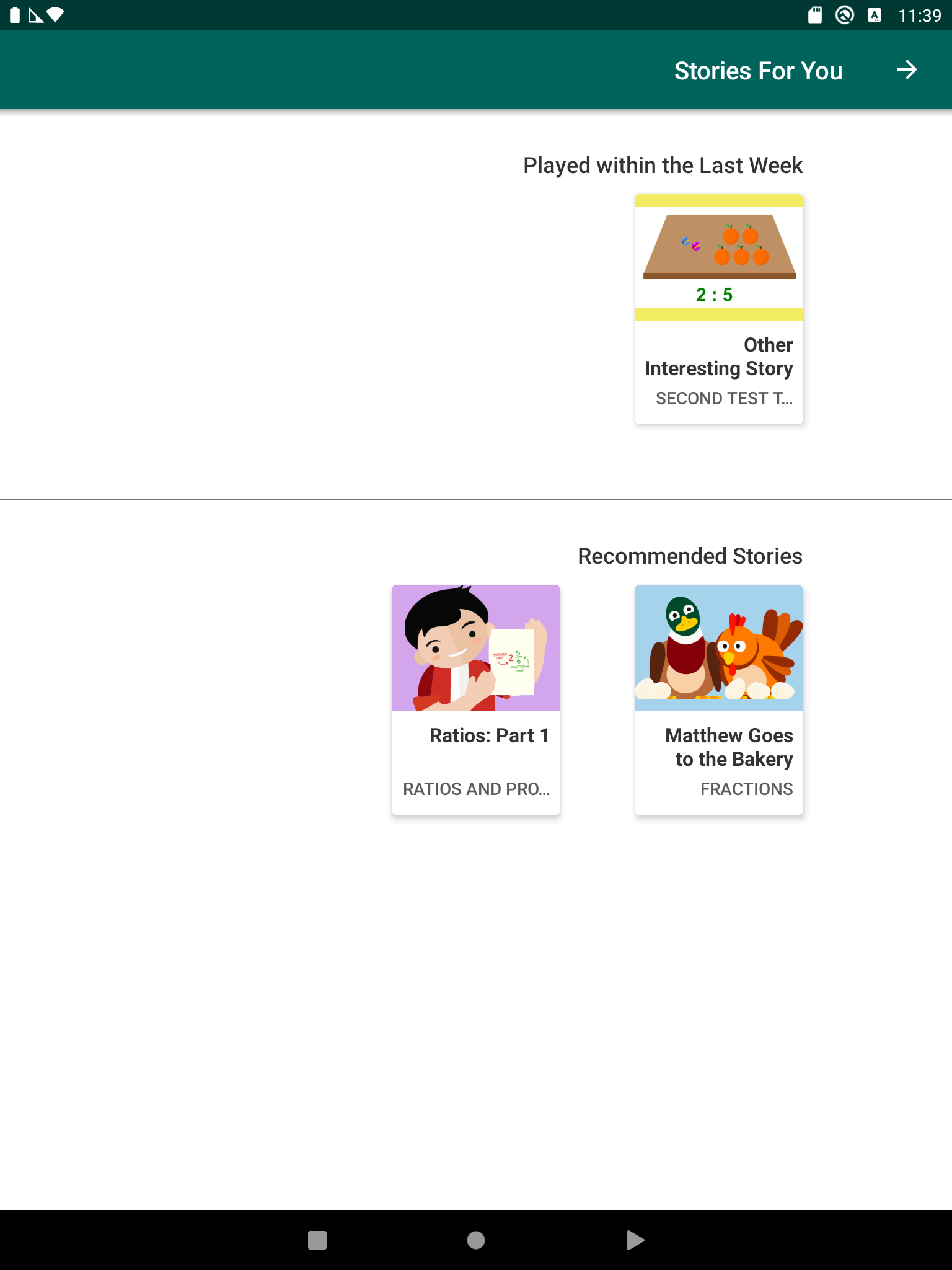|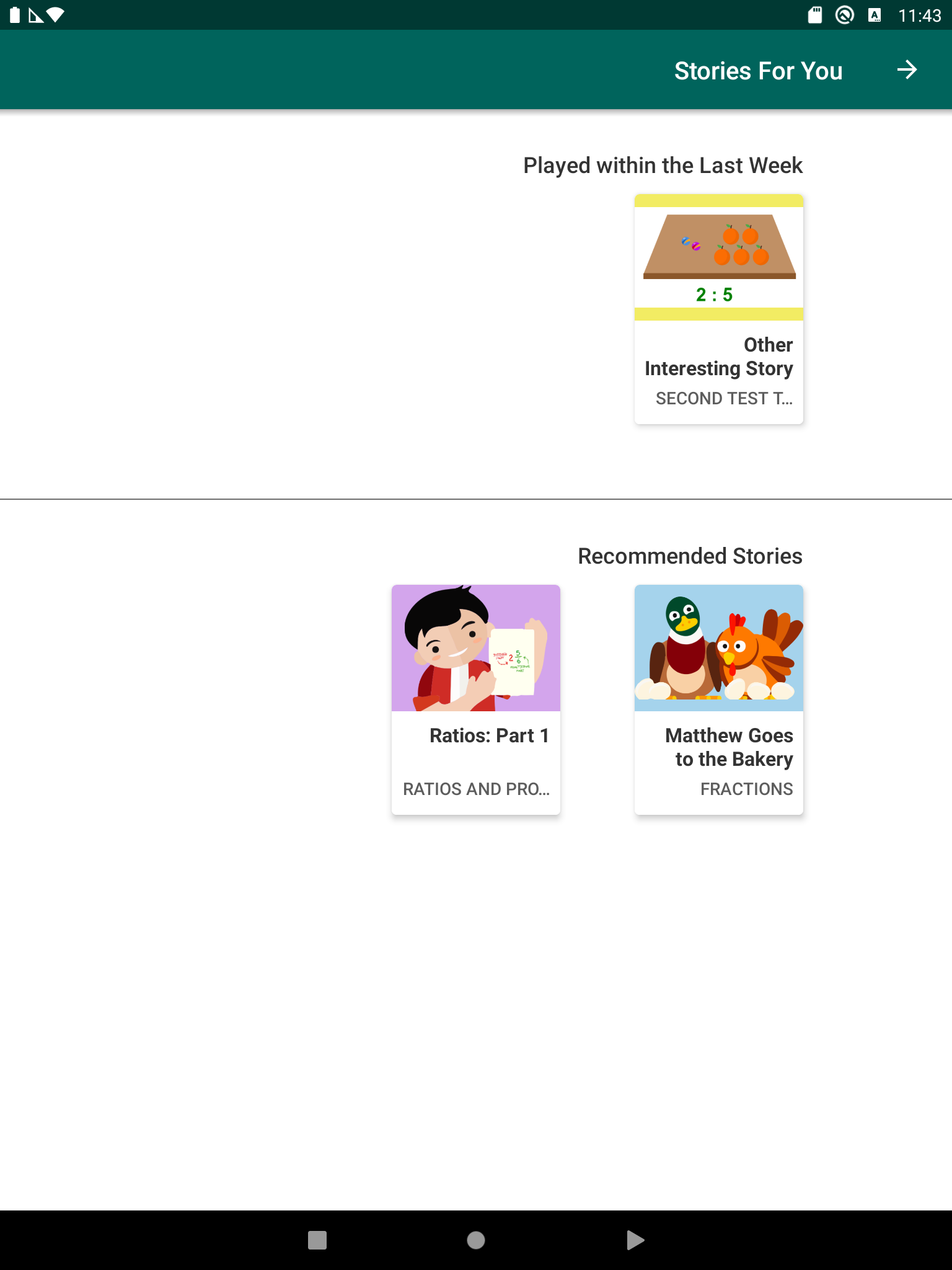| Test results: 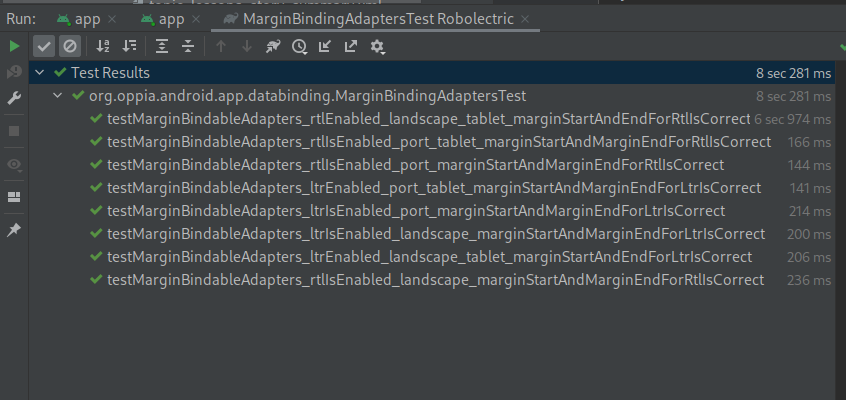 ## Essential Checklist <!-- Please tick the relevant boxes by putting an "x" in them. --> - [x] The PR title and explanation each start with "Fix #bugnum: " (If this PR fixes part of an issue, prefix the title with "Fix part of #bugnum: ...".) - [x] Any changes to [scripts/assets](https://github.com/oppia/oppia-android/tree/develop/scripts/assets) files have their rationale included in the PR explanation. - [x] The PR follows the [style guide](https://github.com/oppia/oppia-android/wiki/Coding-style-guide). - [x] The PR does not contain any unnecessary code changes from Android Studio ([reference](https://github.com/oppia/oppia-android/wiki/Guidance-on-submitting-a-PR#undo-unnecessary-changes)). - [x] The PR is made from a branch that's **not** called "develop" and is up-to-date with "develop". - [ ] The PR is **assigned** to the appropriate reviewers ([reference](https://github.com/oppia/oppia-android/wiki/Guidance-on-submitting-a-PR#clarification-regarding-assignees-and-reviewers-section)). ## For UI-specific PRs only <!-- Delete these section if this PR does not include UI-related changes. --> If your PR includes UI-related changes, then: - Add screenshots for portrait/landscape for both a tablet & phone of the before & after UI changes - For the screenshots above, include both English and pseudo-localized (RTL) screenshots (see [RTL guide](https://github.com/oppia/oppia-android/wiki/RTL-Guidelines)) - Add a video showing the full UX flow with a screen reader enabled (see [accessibility guide](https://github.com/oppia/oppia-android/wiki/Accessibility-A11y-Guide)) - Add a screenshot demonstrating that you ran affected Espresso tests locally & that they're passing --------- Co-authored-by: Adhiambo Peres <59600948+adhiamboperes@users.noreply.github.com>
- Loading branch information