-
Notifications
You must be signed in to change notification settings - Fork 0
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
Showing
1 changed file
with
217 additions
and
1 deletion.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1 +1,217 @@ | ||
# svg-paper | ||
# svg-paper | ||
|
||
[](https://travis-ci.com/ttskch/svg-paper) | ||
[](https://www.npmjs.com/package/svg-paper) | ||
[](https://www.npmjs.com/package/svg-paper) | ||
[](https://www.jsdelivr.com/package/npm/svg-paper) | ||
|
||
The world's most maintainable way to create paper-printable documents 🖨💘 | ||
|
||
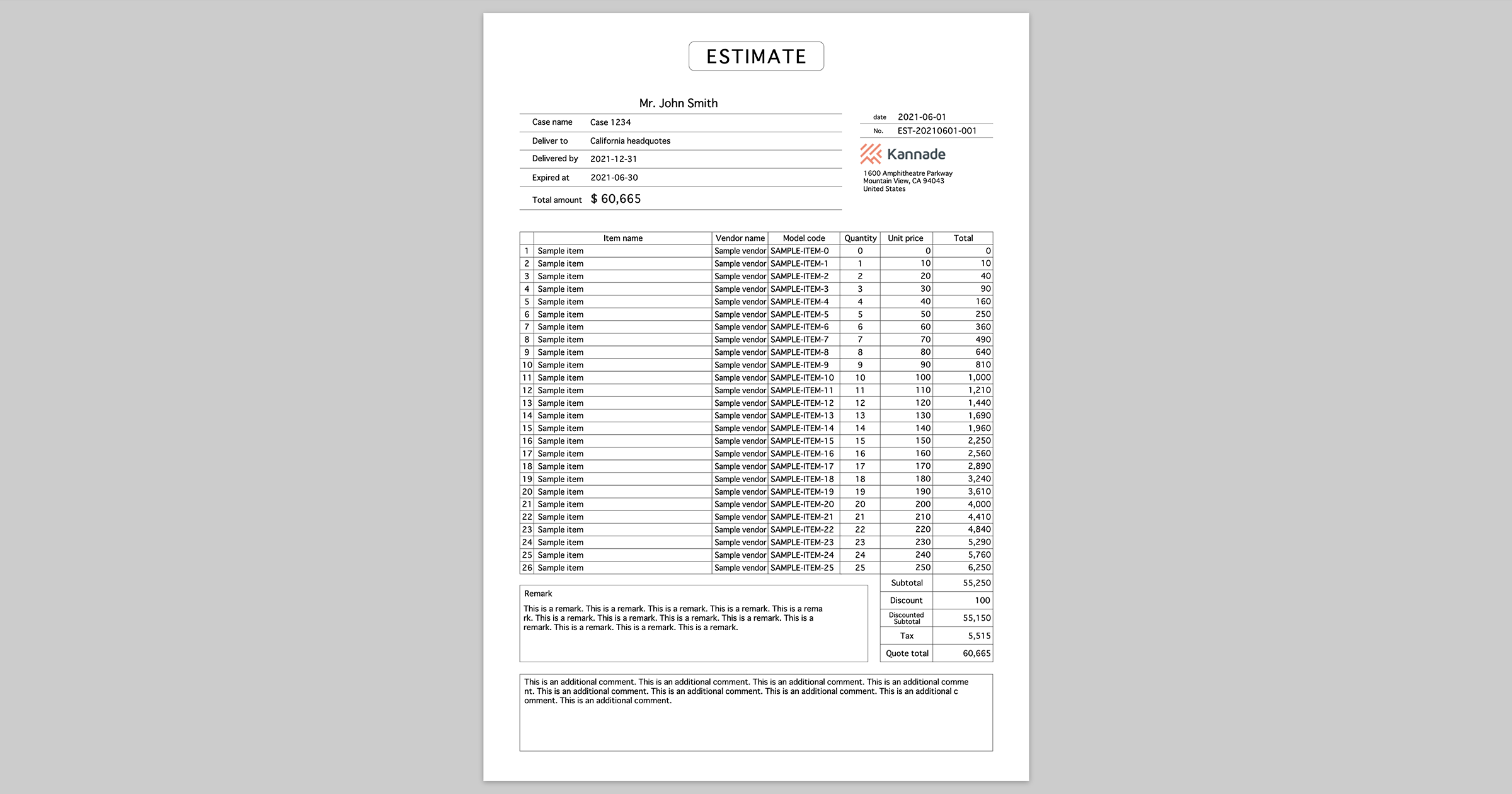 | ||
|
||
## Concepts | ||
|
||
You can print beautiful and maintainable paper documents by following steps: | ||
|
||
1. Design the document with [Adobe XD](https://www.adobe.com/products/xd.html), [Figma](https://www.figma.com/), or something | ||
1. Export it as SVG | ||
1. Embed SVG into your html and fix it with **svg-paper** | ||
1. Write a little CSS for previewing | ||
1. That's it 👍 | ||
|
||
## Installation | ||
|
||
### CDN | ||
|
||
```html | ||
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/svg-paper@0.0.0/dist/svg-paper.min.css"> | ||
<script src="https://cdn.jsdelivr.net/npm/svg-paper@0.0.0/dist/svg-paper.min.js"></script> | ||
``` | ||
|
||
### npm | ||
|
||
```bash | ||
$ npm install svg-paper | ||
``` | ||
|
||
## Basic usage | ||
|
||
```js | ||
import SvgPaper from 'svg-paper' | ||
// or | ||
// const SvgPaper = require('svg-paper') | ||
|
||
const paper = new SvgPaper() | ||
|
||
paper | ||
// replace placeholder to actual value | ||
.replace('%placeholder1%', 'Actual value 1') | ||
// ... and more | ||
|
||
// set max width to 1000 | ||
// in the other words, if actual width of the content is bigger than 1000 it shrinks automatically | ||
.adjustText('#selector1', 1000) | ||
|
||
// set max width to 800 and brings the element #_caseName to the center of 800 width area | ||
.adjustText('#selector2', 800, 'middle') | ||
|
||
// of course you can bring it to the end | ||
.adjustText('#selector3', 800, 'end') | ||
|
||
// automatically wrap or shrink actual content so that it fits within the specified area (600 x 300) | ||
.adjustTetxarea('#selector4', 600, 300) | ||
|
||
// you can pass some additional args | ||
.adjustTextarea('#selector5', | ||
600, // width | ||
300, // height | ||
1.2, // lineHeight : default is 1.2 times font-size | ||
0.5, // paddingX : default is 0.5 times font-size | ||
0.5, // paddingY : default is 0.5 times font-size | ||
false // nowrap : default is false. if true, content will not be wrapped | ||
) | ||
|
||
// finally, apply all replacing and adjusting to DOM | ||
.apply() | ||
``` | ||
|
||
Please see [test code](js/tests/functional.test.js) to learn more 👌 | ||
|
||
You also can see the live demo on your local by following steps: | ||
|
||
1. `git clone` this repo | ||
1. `npm install` | ||
1. `npm run test js/tests/functional.test.js` | ||
1. open `js/tests/output/real-world-paper-xd.html` in your browser | ||
|
||
### Tips: Hiding content before placeholders are replaced | ||
|
||
svg-paper replaces placeholders and adjust text/textarea after DOM loaded, so the content before replaced and adjusted will be shown on the screen for a moment 🤔 | ||
|
||
This problem is very easy to solve just by adding some "blinder" layer on the content and hide it after `.apply()` 👍 | ||
|
||
```html | ||
<body> | ||
<div id="blinder" style="width:100vw; height:100vh; background-color:#ccc"></div> | ||
<svg>...</svg> | ||
</body> | ||
``` | ||
|
||
```js | ||
paper.apply() | ||
|
||
document.querySelector('#blinder').style.display = 'none' | ||
``` | ||
|
||
|
||
## With non Node.js back-end | ||
|
||
If your back-end isn't Node.js, you can use svg-paper by passing replacements and text/textarea adjustment parameters to front-end as JSON string. | ||
|
||
### e.g. PHP and Twig | ||
|
||
```php | ||
// Controller | ||
public function paper(YourModel $model, YourPaperDefinition $paper) | ||
{ | ||
return $this->render('paper.html.twig', [ | ||
'svg' => $paper->getSvg(), | ||
'replacements' => $paper->getReplacements($model), | ||
'textAdjustments' => $paper->getTextAdjustments(), | ||
'textAreaAdjustments' => $paper->getTextAreaAdjustments(), | ||
]); | ||
} | ||
``` | ||
|
||
```php | ||
// YourPaperDefinition | ||
class YourPaperDefinition | ||
{ | ||
public function getSvg() | ||
{ | ||
return file_get_contents('/path/to/paper.svg'); | ||
} | ||
|
||
public function getReplacements(YourModel $model): array | ||
{ | ||
return [ | ||
'%placeholder1%' => 'Actual value 1', | ||
// ... and more | ||
]; | ||
} | ||
|
||
public function getTextAdjustments(): array | ||
{ | ||
return [ | ||
// selector => [args for SvgPaper.adjustText()] | ||
'#selector1' => [1000], | ||
'#selector2' => [800, 'middle'], | ||
'#selector3' => [800, 'end'], | ||
]; | ||
} | ||
|
||
public function getTextareaAdjustments(): array | ||
{ | ||
// selector => [args for SvgPaper.adjustTextarea()] | ||
return [ | ||
'#selector2' => [600, 300], | ||
// ... and more | ||
]; | ||
} | ||
} | ||
``` | ||
|
||
```twig | ||
{# paper.html.twig #} | ||
<!DOCTYPE html> | ||
<html> | ||
<head> | ||
<meta charset="utf-8"> | ||
<meta http-equiv="X-UA-Compatible" content="IE=edge"> | ||
<meta name="viewport" content="width=device-width, initial-scale=1, user-scalable=1"> | ||
<title>Paper</title> | ||
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/svg-paper@0.0.0/dist/svg-paper.min.css"> | ||
</head> | ||
<body class="{% block body_class %}{{ block('title') }}{% endblock %}"> | ||
{{ svg|raw }} | ||
<div data-replacements="{{ replacements|json_encode }}"></div> | ||
<div data-text-adjustments="{{ textAdjustments|json_encode }}"></div> | ||
<div data-textarea-adjustments="{{ textAdjustments|json_encode }}"></div> | ||
<script src="https://cdn.jsdelivr.net/npm/svg-paper@0.0.0/dist/svg-paper.min.js"></script> | ||
<script src="your-script.js"></script> | ||
</body> | ||
</html> | ||
``` | ||
|
||
```js | ||
// your-script.js | ||
const replacements = $('[data-replacements]').data('replacements') | ||
const textAdjustments = $('[data-adjustments]').data('text-adjustments') | ||
const textareaAdjustments = $('[data-adjustments]').data('textarea-adjustments') | ||
|
||
const paper = new SvgPaper() | ||
|
||
for (let [search, replacement] of Object.entries(replacements)) { | ||
paper.replace(search, replacement) | ||
} | ||
|
||
for (let [selector, args] of Object.entries(textAdjustments)) { | ||
paper.adjustText(selector, ...args) | ||
} | ||
|
||
for (let [selector, args] of Object.entries(textareaAdjustments)) { | ||
paper.adjustTextarea(selector, ...args) | ||
} | ||
|
||
paper.apply() | ||
``` | ||
|
||
## PDF Generation | ||
|
||
You can easily print to PDF by using [electron-pdf](https://github.com/fraserxu/electron-pdf). | ||
|
||
```bash | ||
$ npm install --global electron-pdf | ||
$ electron-pdf your-document.html your-document.pdf | ||
``` |